Understanding Coalescing and Logical OR in TypeScript: A Detailed Guide

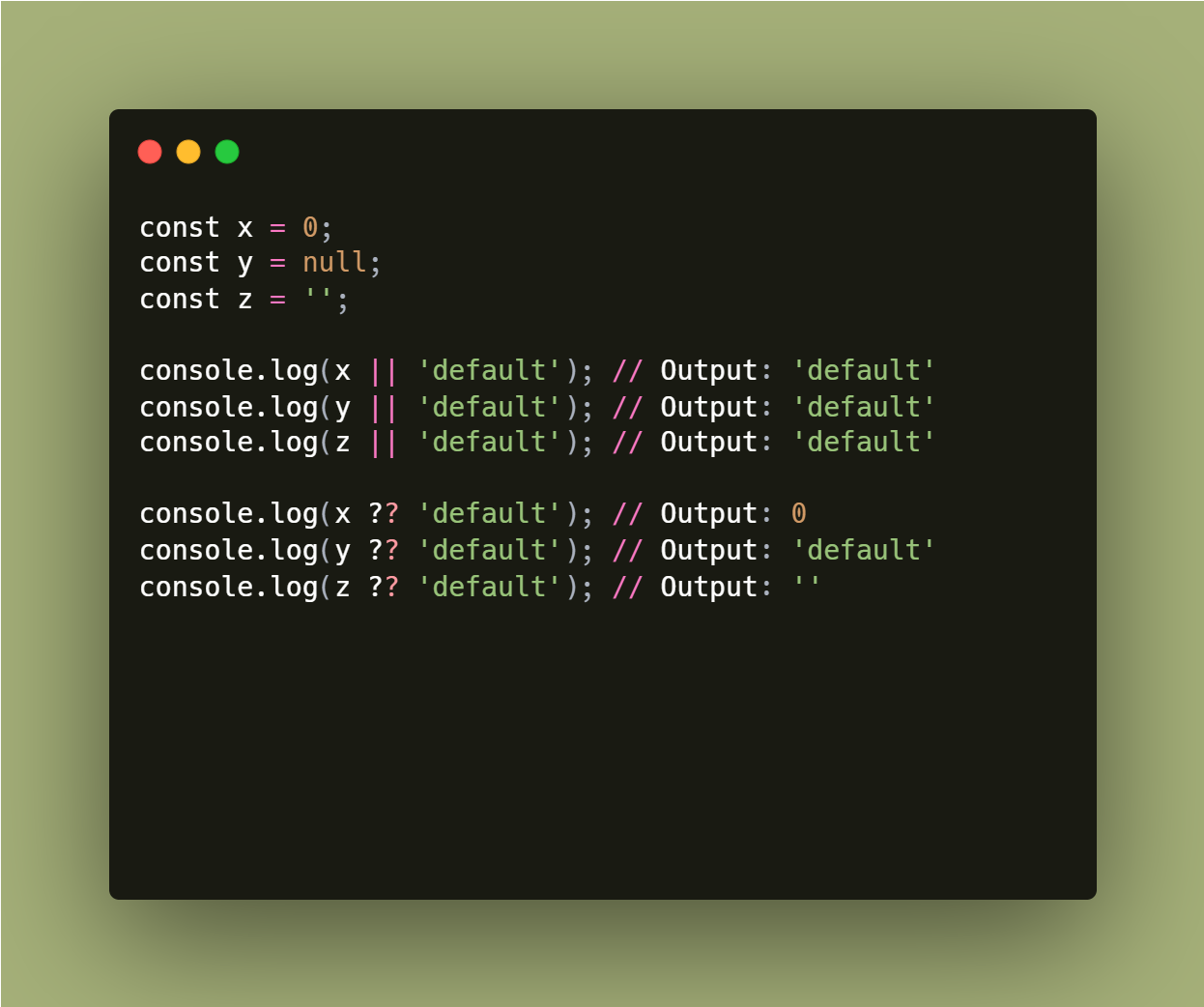
Introduction
In the world of TypeScript, understanding operators such as coalescing and logical OR is crucial for efficient coding. These operators provide a simple way to manage default values or to control the flow of your program based on certain conditions. In this blog, we will delve deeper into the concepts of coalescing and logical OR in TypeScript, with practical examples to enhance understanding.
The Nullish Coalescing Operator
The Nullish Coalescing Operator (??) in TypeScript is a logical operator that returns its right-hand side operand when its left-hand side operand is null or undefined, and otherwise returns its left-hand side operand. This can be particularly useful when you want to assign default values.
let x = null;
let y = x ?? 'default value';
console.log(y); // Output: 'default value'
In this example, since x is null, the variable y is assigned the 'default value'.
The Logical OR Operator
The Logical OR operator (||) returns its first truthy argument or the last argument if all are falsy. This is commonly used for setting default values as well, but it behaves differently from the nullish coalescing operator. It considers not just null or undefined, but also '', 0, NaN, and false as 'falsy' values.
let x = 0;
let y = x || 'default value';
console.log(y); // Output: 'default value'
In this example, even though x is defined, y gets the 'default value' because 0 is considered falsy in JavaScript.
Coalescing vs Logical OR
The main difference between the nullish coalescing operator and the logical OR operator in TypeScript is their treatment of 'falsy' values. While the logical OR operator treats '', 0, NaN, false, null, and undefined as falsy, the nullish coalescing operator only treats null and undefined as falsy.
let x = 0;
let y = x ?? 'default value';
console.log(y); // Output: 0
Here, y gets the value of x (which is 0), because with the nullish coalescing operator, 0 is not considered a falsy value.
Conclusion
In TypeScript, understanding the differences between the Nullish Coalescing Operator and the Logical OR Operator can significantly improve your coding efficiency. While they are similar in many ways, knowing when to use one over the other can significantly impact your code's behaviour. Remember, the Nullish Coalescing Operator treats only null and undefined as falsy, while the Logical OR Operator treats '', 0, NaN, false, null, and undefined as falsy.
Subscribe to my newsletter
Read articles from Kelvin John Domeh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Kelvin John Domeh
Kelvin John Domeh
Passionately curious about Software engineering. ๐ I spend time exploring new technologies and improving upon the ones I know. When I'm not studying for university, you'll find me building side projects that challenge me to learn and grow as a Tech Enthusiast. Join me as I share my journey and discoveries in the tech world.