Voice Search Integration in React: A Complete Tutorial
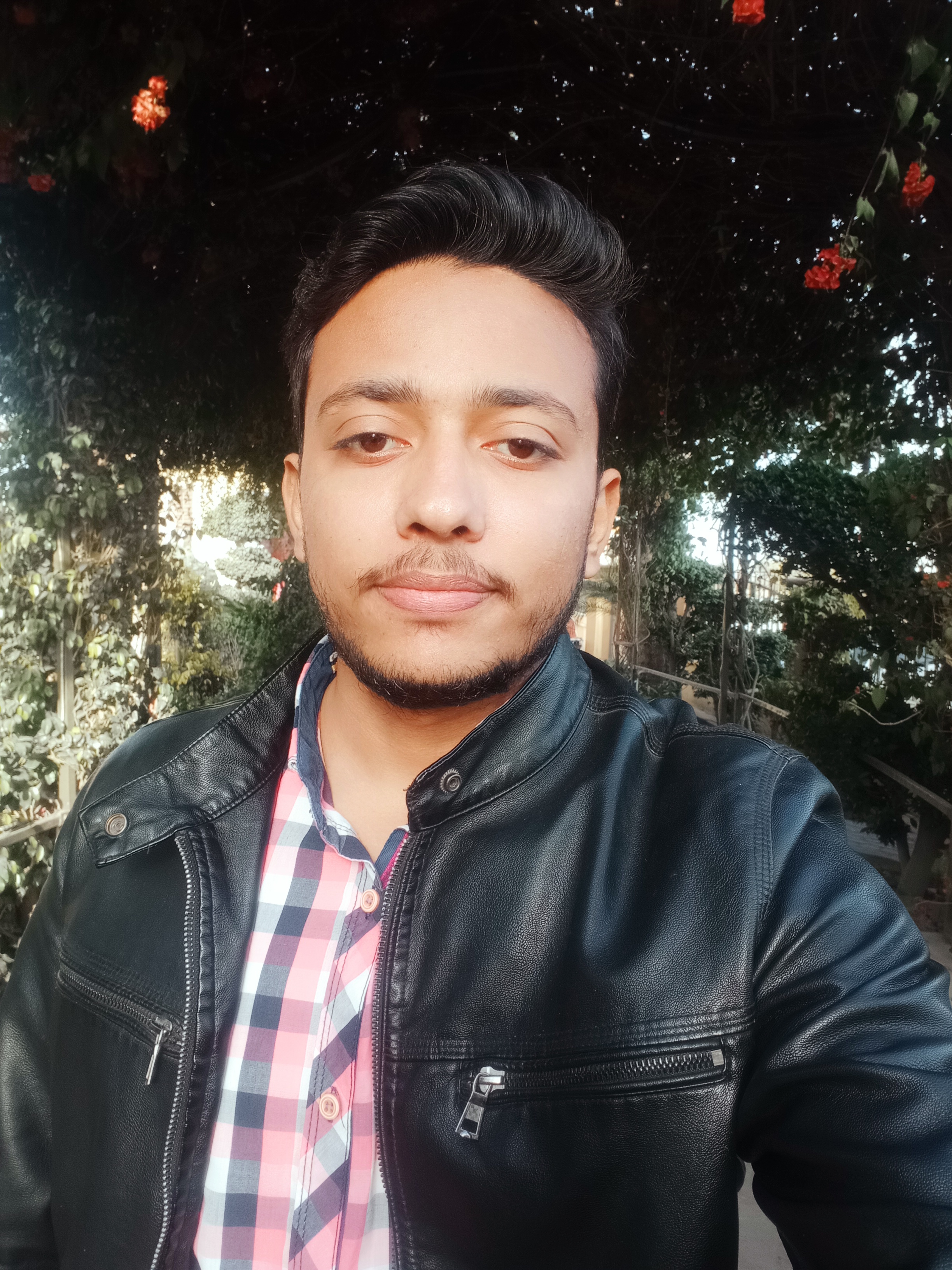
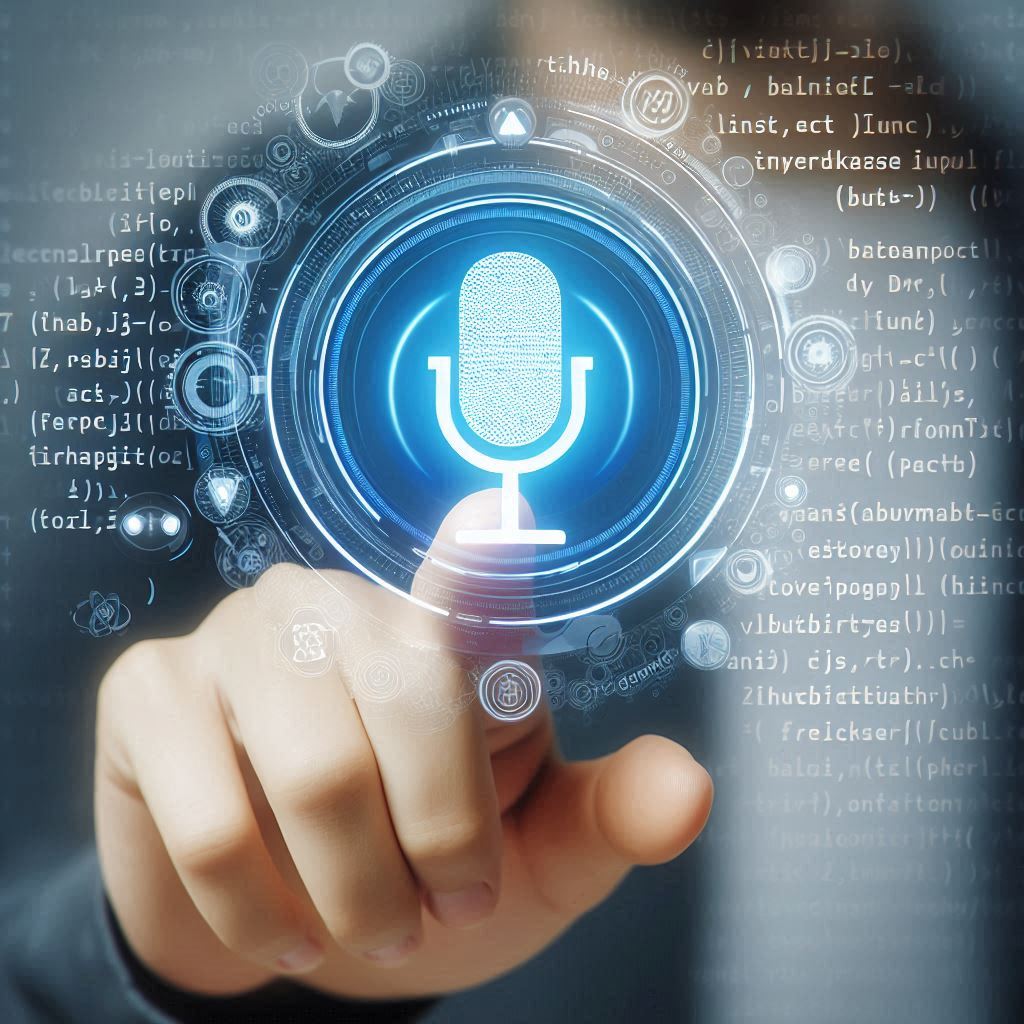
This blog post offers a step-by-step guide to adding voice search functionality to React applications using the Web Speech API. It explains how to set up a new React app, install the required dependencies, create a VoiceSearch component with the react-speech-recognition library, and integrate the component into your app. By the end, you'll have a working voice search feature that uses the browser's SpeechRecognition API.
In the era of smart devices, voice search has become a fundamental feature in applications. This blog post will guide you through the process of implementing voice search functionality in your React applications using the Web Speech API.
Prerequisites
Before we start, make sure you have the following:
Basic understanding of React and JavaScript.
Node.js and npm installed on your system.
A text editor, such as Visual Studio Code.
Step 1: Setting Up the React Application
First, let’s create a new React application using vite
:
yarn create vite voice-search-app --template react
or
npx create-vite voice-search-app --template react
Navigate into the project directory:
cd voice-search-app
Step 2: Installing Dependencies
We will use the react-speech-recognition
library, which provides a React Hook for the browser’s SpeechRecognition API. Install it using npm:
yarn add -D react-speech-recognition
or
npm install --save react-speech-recognition
Step 3: Implementing Voice Search
Now, let’s create a new component VoiceSearch.js
:
import React from 'react';
import SpeechRecognition, { useSpeechRecognition } from 'react-speech-recognition';
const VoiceSearch = () => {
const { transcript, listening } = useSpeechRecognition();
if (!SpeechRecognition.browserSupportsSpeechRecognition()) {
return <p>Sorry, your browser does not support speech recognition.</p>;
}
return (
<div>
<button onClick={SpeechRecognition.startListening}>Start</button>
<button onClick={SpeechRecognition.stopListening}>Stop</button>
<p>{listening ? 'Listening...' : 'Click "Start" to start listening'}</p>
<p>{transcript}</p>
</div>
);
};
export default VoiceSearch;
In this component, we use the useSpeechRecognition
hook to access the transcript
and listening
state. We also provide “Start” and “Stop” buttons to control the listening state.
Step 4: Using the Voice Search Component
Finally, let’s use our VoiceSearch
component in App.js
:
import React from 'react';
import VoiceSearch from './VoiceSearch';
function App() {
return (
<div className="App">
<h1>Voice Search Demo</h1>
<VoiceSearch />
</div>
);
}
export default App;
Conclusion
And that’s it! You’ve successfully implemented voice search functionality in your React app. Remember, the Web Speech API is still experimental and may not be fully supported in all browsers. Always check for browser compatibility before using it in production.
Happy coding!
Subscribe to my newsletter
Read articles from Muhammad Usman Tariq directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
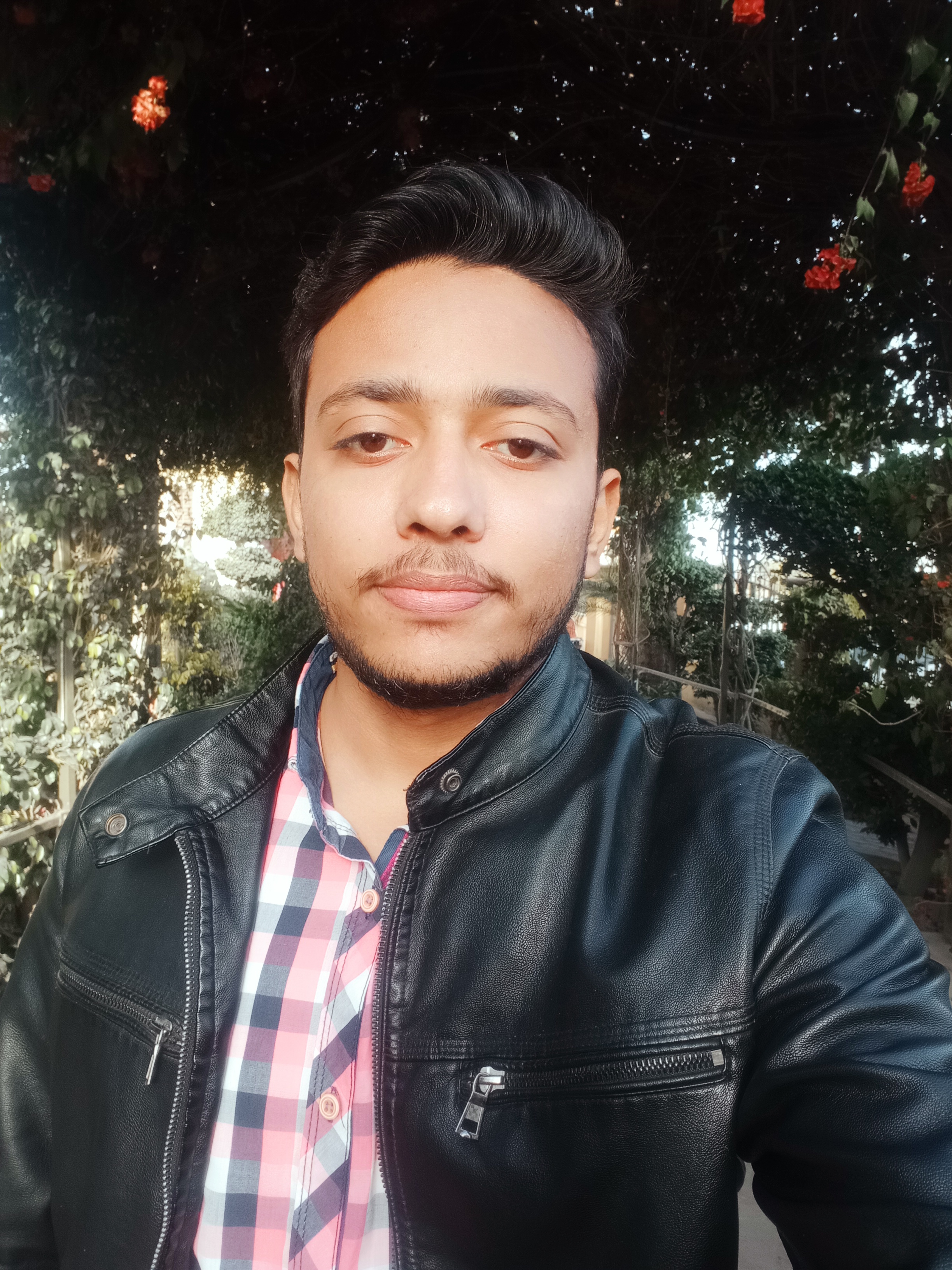
Muhammad Usman Tariq
Muhammad Usman Tariq
Hello, I'm Muhammad Usman Tariq, a dedicated and passionate Full Stack Developer. With a knack for problem-solving and an insatiable thirst for new knowledge, I am always on the lookout for innovative solutions to complex problems. My journey in the tech industry has been driven by my curiosity and the joy of turning ideas into reality. I have honed my skills in various technologies and programming languages, enabling me to build robust and scalable applications. I believe in the power of technology to transform lives and businesses, and I strive to be at the forefront of this transformation. My goal is to create software that not only meets business needs but also provides an exceptional user experience. In my spare time, I enjoy staying updated with the latest industry trends. I am always eager to learn and grow, and I welcome any opportunity that allows me to do so. Thank you for visiting my profile. Let's connect and create something amazing together!