Craft Dynamic Laravel Views: A Beginner's Guide to Blade Directives
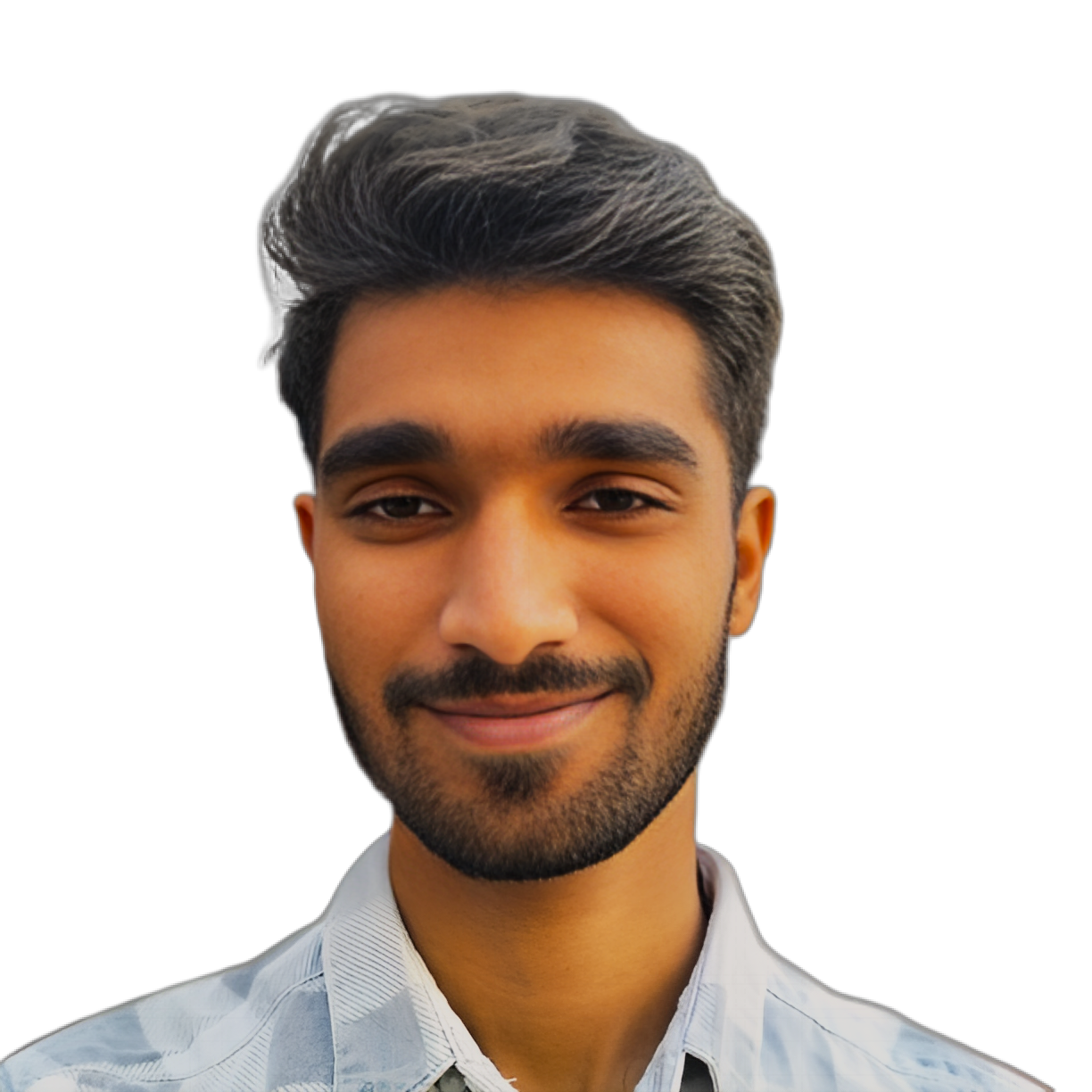
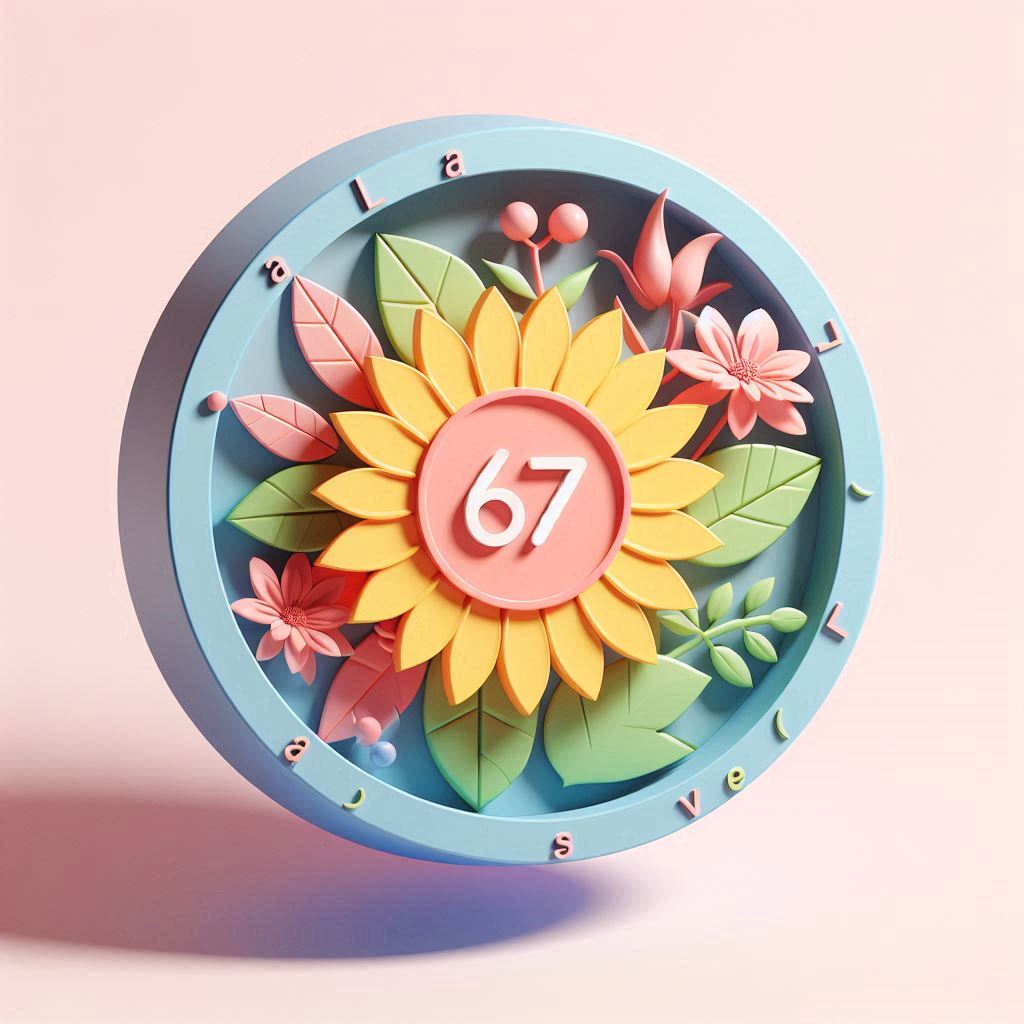
Hey there, fellow coders! Today, we're diving into the fantastic world of Laravel Blade directives, a game-changer for crafting clean and dynamic views in your Laravel applications. Buckle up and get ready to ditch clunky PHP code blocks and embrace a world of streamlined templating magic!
What are Blade Directives?
Imagine tiny wizards sprinkled throughout your Blade templates, each with a specific task. That's essentially what Blade directives are! These special helpers, starting with the "@" symbol, weave PHP logic seamlessly into your views. They offer a cleaner and more readable alternative to embedding raw PHP code blocks, keeping your templates organized and your codebase happy.
Unveiling the Blade Directive Toolbox
Blade offers a variety of directives to tackle common templating tasks. Let's explore some of the most frequently used ones:
- Conditional Rendering with
@if
,@else
, and@endif
These directives mimic their PHP counterparts, allowing you to control the flow of content based on conditions. Here's an example:
HTML
@if (count($products) > 0)
<ul>
@foreach ($products as $product)
<li>{{ $product->name }}</li>
@endforeach
</ul>
@else
<p>No products found!</p>
@endif
In this snippet, we check if there are any products in the $products
collection. If there are, we loop through them and display their names. Otherwise, a message indicating no products is displayed.
- Looping Through Collections with
@foreach
and@endforeach
Need to iterate over a collection of data? @foreach
and @endforeach
come to the rescue! This powerful duo simplifies the process of looping through data and displaying dynamic content for each item.
- Reusability Magic with
@include
Imagine having reusable template components that you can integrate into different views. That's the beauty of @include
! It allows you to define reusable code snippets in separate Blade files and then seamlessly include them wherever needed. This promotes code organization and reduces redundancy.
- Dynamic Sections with
@yield
and Layout Files
Ever wanted to create a master layout with defined content sections that you can populate dynamically across different views? @yield
makes it possible! You define content sections within a layout file using @yield('section-name')
, and then populate them with specific content in your views using @section('section-name')
. Think of it as building a flexible template framework!
Beyond the Basics: Exploring More Blade Directives
Blade offers a wider range of directives for various tasks, including:
@auth
and@guest
: Check for user authentication status@switch
and@case
: Handle multiple conditions@isset
and@empty
: Check if variables are set or empty
These directives further enhance your templating capabilities, allowing you to craft even more powerful and dynamic views.
Unleashing the Power of Blade Directives
Now that you've grasped the core concepts, let's see Blade directives in action! Here's a simple example demonstrating a product listing view:
HTML
<h1>Our Awesome Products</h1>
@if (count($products) > 0)
<ul>
@foreach ($products as $product)
<li>
<a href="{{ route('product.show', $product->id) }}">{{ $product->name }}</a> - ${{ $product->price }}
</li>
@endforeach
</ul>
@else
<p>No products available yet!</p>
@endif
This view displays a product listing with product names and prices. The @if
directive ensures the list is only displayed if products exist, while @foreach
loops through each product and displays its details.
Ready to Dive Deeper?
This blog post has just scratched the surface of the fantastic world of Blade directives. To truly master them, explore the Laravel documentation (https://arjunamrutiya.medium.com/mastering-laravel-blade-directives-a-step-by-step-guide-8fb36dbe59d1) and experiment with different directives. There are also plenty of online tutorials that delve deeper into specific use cases.
Remember, Blade directives are your allies in crafting clean, readable, and maintainable Laravel views. So, embrace them, unleash your creativity, and build stunning web applications!
Bonus Tip: Don't forget the power of comments! Adding comments to your Blade templates explains the purpose of different sections, making your code easier to understand for yourself and your teammates.
Happy coding!
Subscribe to my newsletter
Read articles from Asis Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
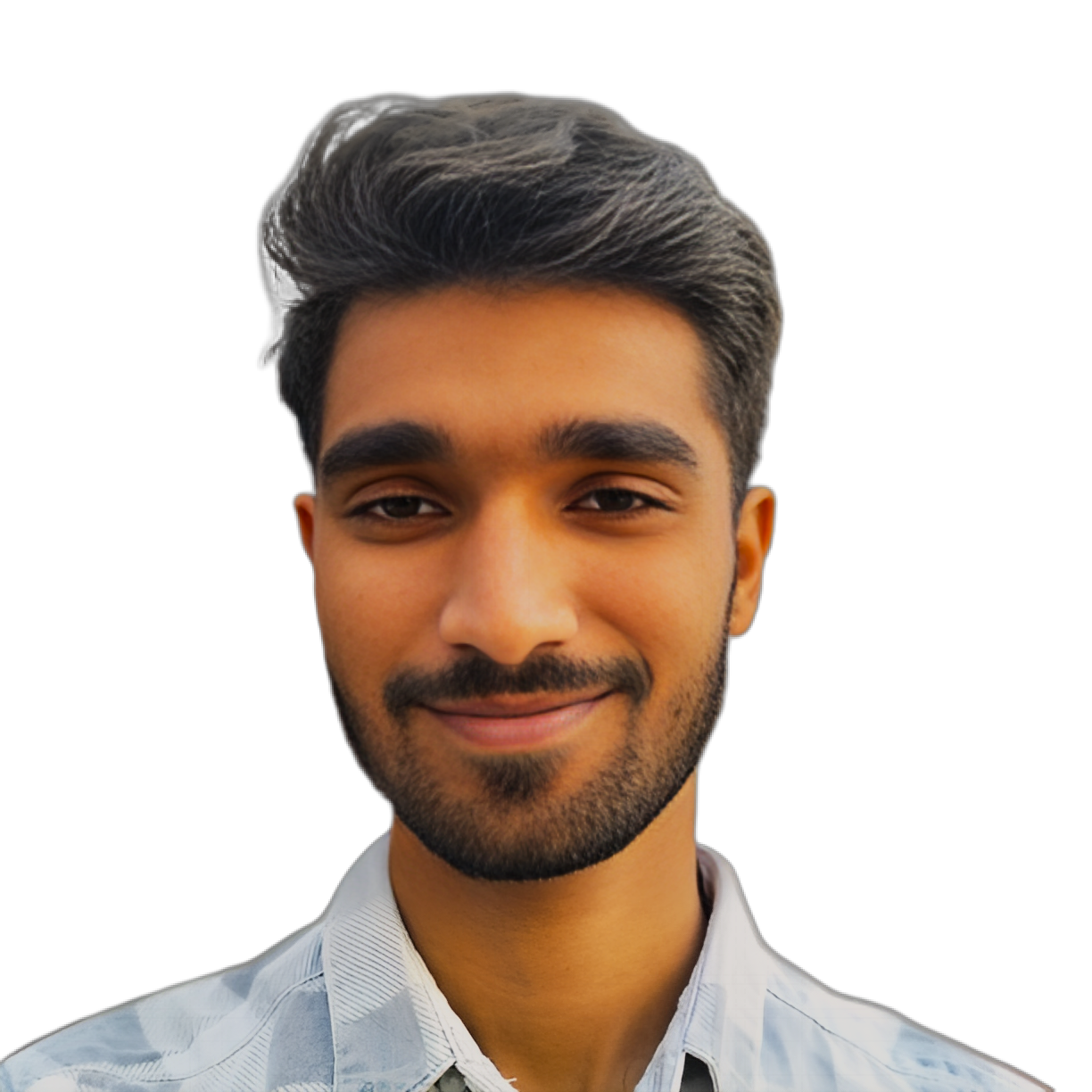
Asis Sharma
Asis Sharma
I'm an ambitious and motivated software developer, eager to apply my knowledge and skills to contribute to the development of innovative and cutting-edge software solutions. I'm well-versed in a variety of programming languages, including Javascript, C++, and Node.js, and have a understanding of object-oriented programming and algorithms. I'm passionate about developing and I am eager to learn and collaborate with others.