Understanding the Builder Design Pattern in Android Development
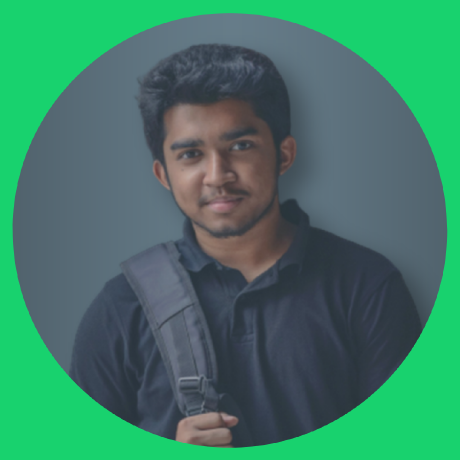
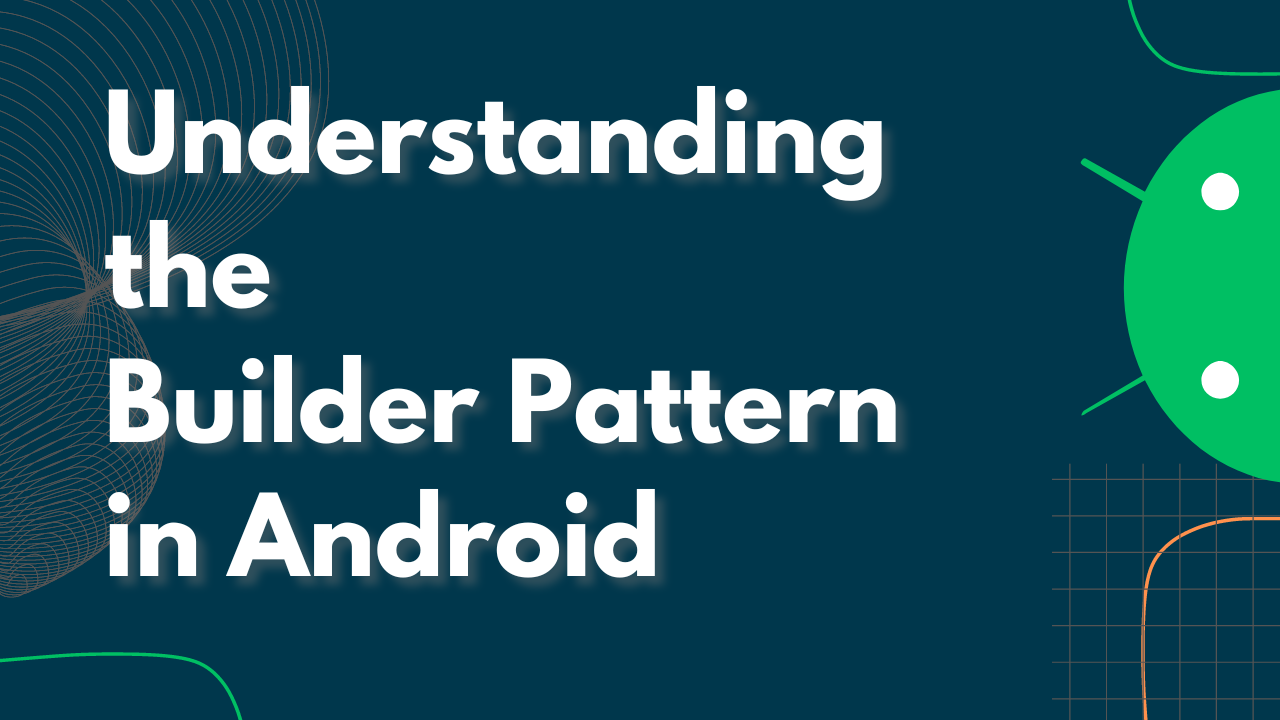
Design patterns are a vital part of software development, providing reusable solutions to common problems. Among them, the Builder design pattern is particularly useful in creating complex objects with numerous optional parameters. In Android development, where creating objects with multiple attributes is a frequent task, the Builder pattern can significantly simplify and enhance code readability and maintainability.
Introduction to Design Patterns
Design patterns are proven solutions to common problems encountered in software design. They provide a template that developers can use to address particular issues in their codebase. The primary goal of design patterns is to make code more efficient, maintainable, and understandable.
There are several categories of design patterns, including creational, structural, and behavioral. The Builder pattern falls under the creational category, focusing on the construction of objects in a flexible and readable manner.
What is the Builder Design Pattern?
The Builder pattern is a creational design pattern that allows for the step-by-step construction of complex objects. Unlike traditional constructors or static factory methods, the Builder pattern provides a clear and concise way to create objects with a variety of configurations without the need for multiple constructors or lengthy parameter lists.
Key Characteristics:
Fluent Interface: It typically uses a fluent interface, allowing method chaining for setting various properties of an object.
Immutable Object Creation: Often used in scenarios where the object to be constructed is immutable.
Readability and Maintainability: Enhances code readability and maintainability by avoiding a complex constructor with numerous parameters.
Why Use the Builder Pattern in Android?
In Android development, it’s common to encounter classes with a plethora of attributes. For instance, configuring a complex AlertDialog
or setting up a Notification
involves multiple optional parameters. The Builder pattern helps manage these configurations efficiently by:
Reducing Constructor Overloading: Avoiding the need for numerous constructors with different parameter combinations.
Enhancing Code Clarity: Making it easier to understand and maintain the code.
Promoting Immutability: Ensuring that the created object remains immutable, which is a best practice in Android to prevent accidental modifications.
Components of the Builder Pattern
The Builder pattern typically involves the following components:
Builder Class: Contains methods to set various properties and a
build()
method to create the object.Product Class: The complex object that is being constructed.
Director (Optional): Guides the construction process, ensuring that the builder creates a fully-configured object.
Implementing the Builder Pattern in Android
Step-by-Step Guide
Define the Product Class: This is the class of the object you want to create.
Create the Builder Class: This class will contain methods for setting each attribute and a
build()
method to construct the final object.Implement Fluent Methods: Each method in the Builder should return the builder itself to enable method chaining.
Example: Creating a Custom Dialog
Let’s create a custom Dialog
using the Builder pattern.
Define the Product Class & Builder Class
class CustomDialog private constructor(builder: Builder) { val title: String? = builder.title val message: String? = builder.message val isCancelable: Boolean = builder.isCancelable val customView: View? = builder.customView // Builder class class Builder { var title: String? = null private set var message: String? = null private set var isCancelable: Boolean = true private set var customView: View? = null private set fun setTitle(title: String) = apply { this.title = title } fun setMessage(message: String) = apply { this.message = message } fun setCancelable(isCancelable: Boolean) = apply { this.isCancelable = isCancelable } fun setCustomView(customView: View) = apply { this.customView = customView } fun build() = CustomDialog(this) } }
Here the
apply
function is used to return theBuilder
instance, enabling a fluent interface for chaining method calls.Using the Builder to Create an Object
val customView: View = // Initialize your custom view val dialog = CustomDialog.Builder() .setTitle("Alert") .setMessage("This is an alert message.") .setCancelable(true) .setCustomView(customView) .build()
Advantages and Disadvantages
Advantages
Improves Code Readability: The Builder pattern makes it easy to understand the construction of complex objects.
Prevents Inconsistent States: It ensures that the object is fully constructed before being used.
Promotes Immutability: The built object can be made immutable, preventing accidental changes.
Disadvantages
Overhead of Extra Code: Requires additional classes and code, which might be overkill for simple objects.
Increased Complexity: Can introduce unnecessary complexity for straightforward object construction.
Use Cases in Android
The Builder pattern is commonly used in Android for:
Creating Complex UI Components: Such as
AlertDialog
orNotification
.Constructing Config Objects: Like
Retrofit
orGlide
configurations.Building Domain Objects: In applications with complex business logic, where objects require multiple parameters.
Common Mistakes and How to Avoid Them
Ignoring Immutability: Failing to make the final object immutable can lead to unexpected changes.
Overusing the Pattern: Not every object needs a Builder; use it only for complex objects.
Neglecting Validation: Ensure that the builder validates input to prevent constructing invalid objects.
Conclusion
The Builder design pattern is a powerful tool in Android development, enabling developers to construct complex objects in a clear and maintainable manner. By encapsulating the construction process, it enhances code readability and helps prevent common pitfalls associated with traditional object creation methods.
Subscribe to my newsletter
Read articles from Emran Khandaker Evan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
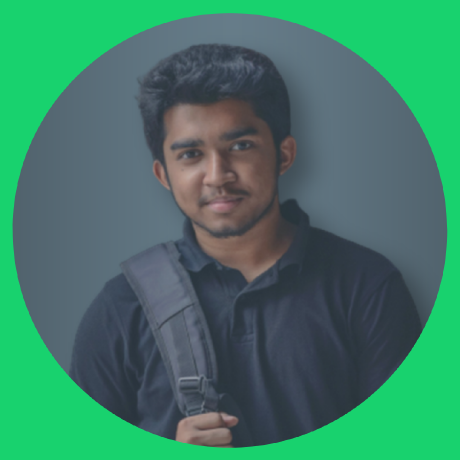
Emran Khandaker Evan
Emran Khandaker Evan
Software Engineer | Content Creator