Using Tailwind CSS with Next.js for Rapid UI Development
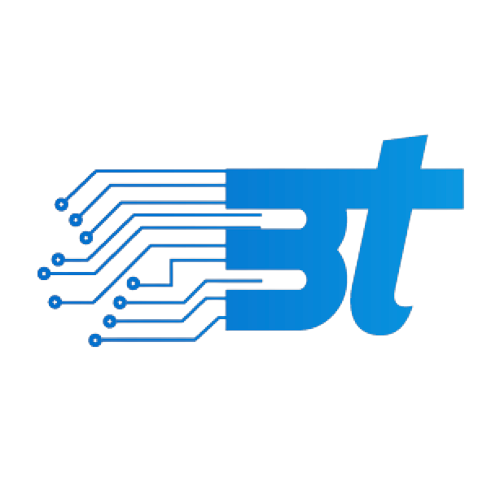
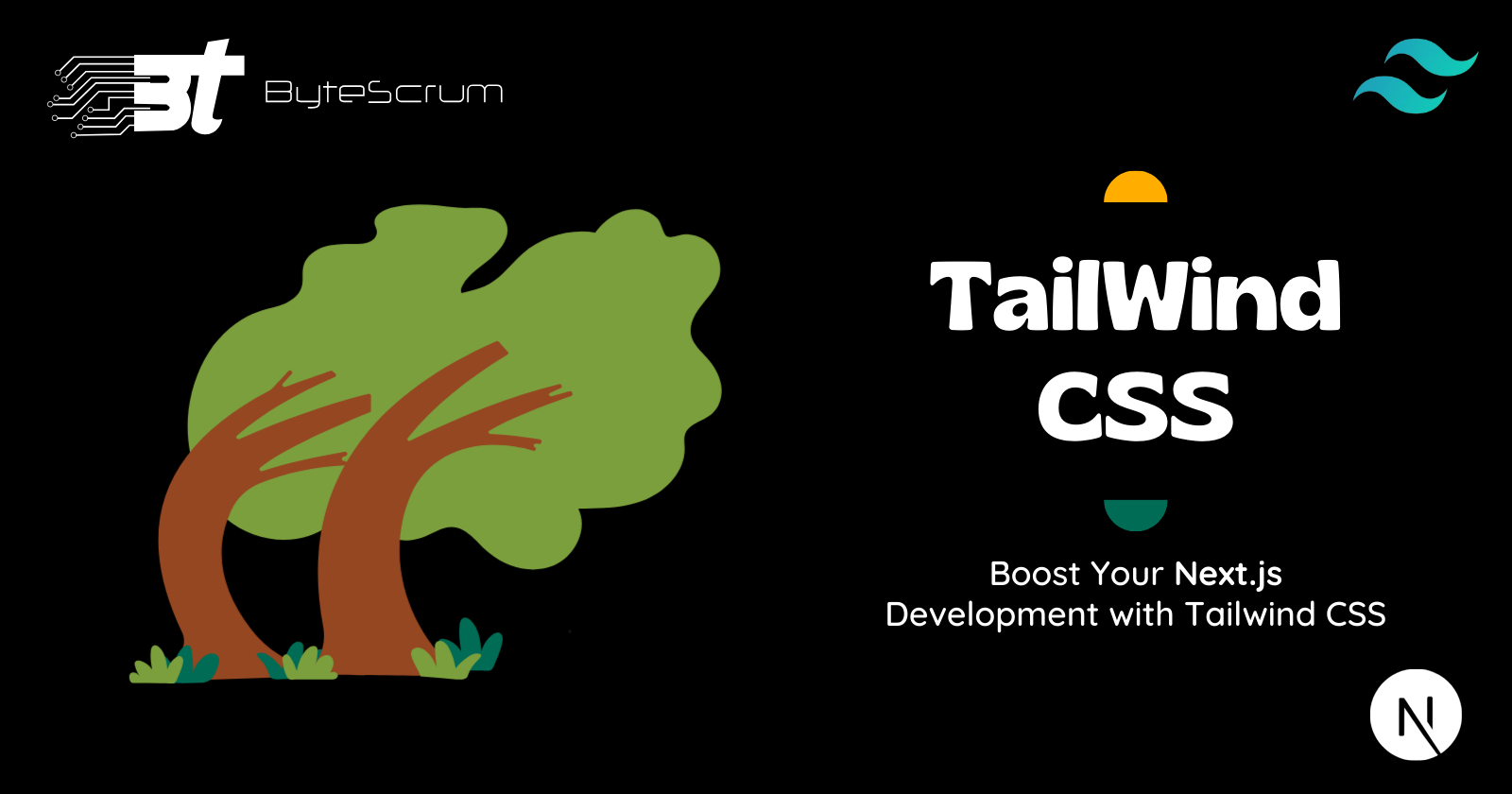
Tailwind CSS is a utility-first CSS framework that provides a highly customizable and efficient way to build modern UIs. When combined with Next.js, it offers a powerful setup for rapid UI development. This guide will walk you through integrating Tailwind CSS with Next.js and demonstrate how to use it effectively to build stylish and responsive web applications.
Why Tailwind CSS?
Utility-First Approach: Tailwind CSS provides low-level utility classes that allow you to build custom designs without writing CSS.
Highly Customizable: Easily customize your design system with Tailwind's configuration file.
Responsive Design: Built-in responsive utilities help you create responsive layouts effortlessly.
Developer Experience: Tailwind CSS offers an intuitive and productive workflow, speeding up the development process.
Setting Up Tailwind CSS with Next.js
Step 1: Create a New Next.js Project
First, create a new Next.js project if you don't have one already.
npx create-next-app my-nextjs-app
cd my-nextjs-app
Step 2: Install Tailwind CSS
Install Tailwind CSS, along with its peer dependencies, postcss
and autoprefixer
.
npm install tailwindcss postcss autoprefixer
Step 3: Initialize Tailwind CSS
Generate the Tailwind configuration files using the Tailwind CLI.
npx tailwindcss init -p
This command creates two configuration files: tailwind.config.js
and postcss.config.js
.
Step 4: Configure Tailwind CSS
Update your tailwind.config.js
file with the paths to all of your pages and components so Tailwind can tree-shake unused styles in production.
// tailwind.config.js
module.exports = {
content: [
'./pages/**/*.{js,ts,jsx,tsx}',
'./components/**/*.{js,ts,jsx,tsx}',
],
theme: {
extend: {},
},
plugins: [],
};
Step 5: Add Tailwind Directives to CSS
Create a CSS file (e.g., styles/globals.css
) and add the Tailwind directives for each of Tailwind’s layers.
/* styles/globals.css */
@tailwind base;
@tailwind components;
@tailwind utilities;
Then, import this CSS file in your _app.js
file.
// pages/_app.js
import '../styles/globals.css';
function MyApp({ Component, pageProps }) {
return <Component {...pageProps} />;
}
export default MyApp;
Building UI Components with Tailwind CSS
With Tailwind CSS set up, you can start building UI components using utility classes.
Example: Creating a Responsive Card Component
Create a new file components/Card.js
:
// components/Card.js
const Card = ({ title, description }) => {
return (
<div className="max-w-sm rounded overflow-hidden shadow-lg p-4 bg-white">
<div className="px-6 py-4">
<div className="font-bold text-xl mb-2">{title}</div>
<p className="text-gray-700 text-base">{description}</p>
</div>
</div>
);
};
export default Card;
Use the Card
component in a page:
// pages/index.js
import Card from '../components/Card';
const Home = () => {
return (
<div className="flex justify-center items-center min-h-screen bg-gray-100">
<Card title="Card Title" description="This is a description of the card." />
</div>
);
};
export default Home;
Example: Creating a Navigation Bar
Create a new file components/Navbar.js
:
// components/Navbar.js
import Link from 'next/link';
const Navbar = () => {
return (
<nav className="bg-blue-500 p-4">
<div className="container mx-auto flex justify-between items-center">
<Link href="/">
<a className="text-white text-lg font-semibold">Home</a>
</Link>
<div className="space-x-4">
<Link href="/about">
<a className="text-white">About</a>
</Link>
<Link href="/contact">
<a className="text-white">Contact</a>
</Link>
</div>
</div>
</nav>
);
};
export default Navbar;
Use the Navbar
component in your pages:
// pages/_app.js
import '../styles/globals.css';
import Navbar from '../components/Navbar';
function MyApp({ Component, pageProps }) {
return (
<>
<Navbar />
<Component {...pageProps} />
</>
);
}
export default MyApp;
Customizing Tailwind CSS
Tailwind's configuration file allows you to extend the default theme and add custom utilities, colors, spacing, and more.
Example: Adding Custom Colors
// tailwind.config.js
module.exports = {
content: [
'./pages/**/*.{js,ts,jsx,tsx}',
'./components/**/*.{js,ts,jsx,tsx}',
],
theme: {
extend: {
colors: {
customBlue: '#1DA1F2',
customGray: '#8899A6',
},
},
},
plugins: [],
};
Use the custom colors in your components:
// components/Card.js
const Card = ({ title, description }) => {
return (
<div className="max-w-sm rounded overflow-hidden shadow-lg p-4 bg-customBlue">
<div className="px-6 py-4">
<div className="font-bold text-xl mb-2 text-customGray">{title}</div>
<p className="text-gray-700 text-base">{description}</p>
</div>
</div>
);
};
export default Card;
Conclusion
For more information and advanced usage, check out the official Tailwind CSS documentation and the Next.js documentation. Happy coding!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
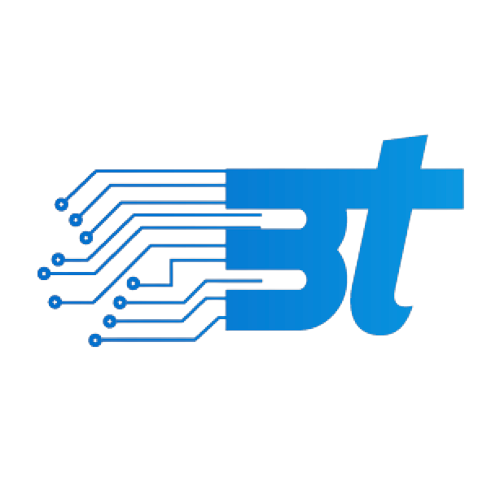
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.