How to Write a Basic Shell Script in Linux: A Step-by-Step Guide

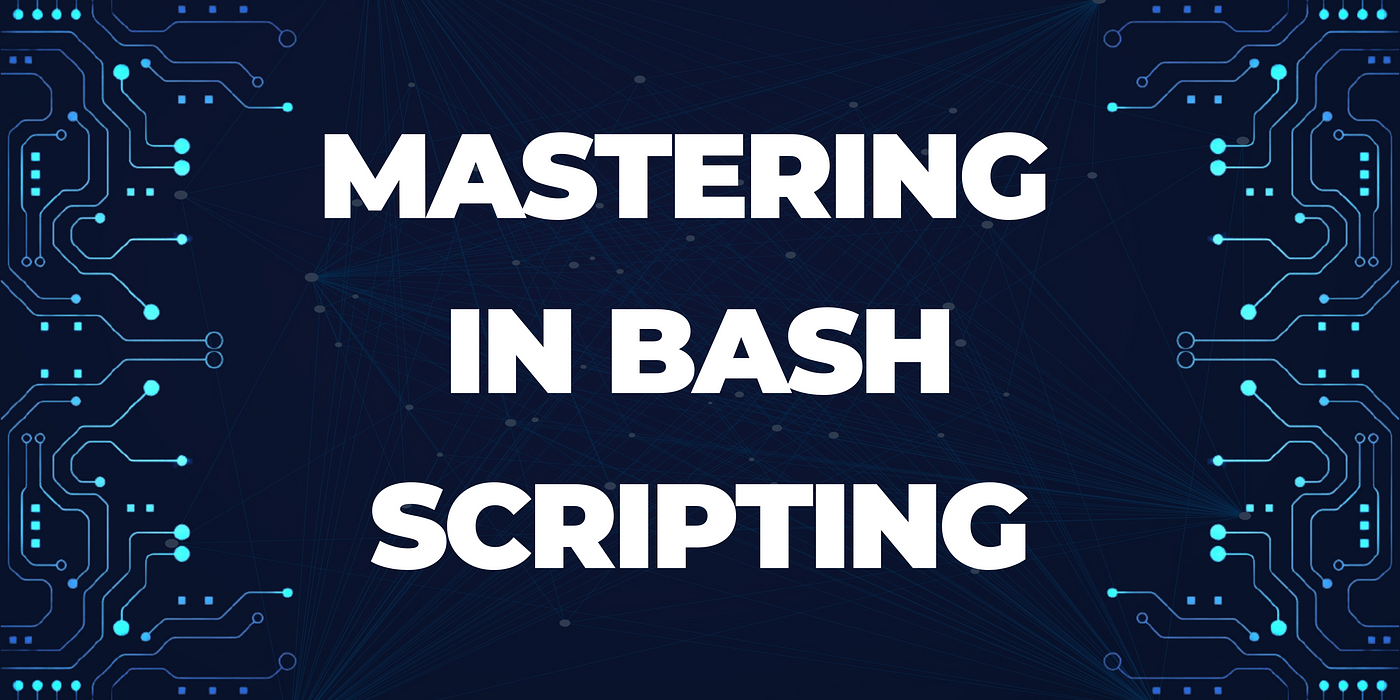
Writing a script in Linux typically involves creating a file with a list of commands that you want to execute. Here’s a step-by-step guide on how to write a basic shell script:
1. Choose a Text Editor
To write a shell script, you need a text editor. Linux offers several options:
nano
vi
vim
gedit
2. Create a New Script File
Create a new file with a .sh
extension. For example, you can create a file named myscript.sh
: nano myscript.sh
3. Add the Shebang Line
The first line of the script should be the shebang (#!
) followed by the path to the shell you want to use. For a Bash script, it would look like this: #!/bin/bash
4. Write Your Script
Add the commands you want to execute. Here’s an example script that prints the username and the current date and time:
#!/bin/bash
# Print the current user
echo "The current user is: $(whoami)"
# Print the current date and time
echo "The current date and time is: $(date)"
# List files in the current directory
echo "Listing files in the current directory:" ls -la
5. Save and Exit
Save the file and exit the text editor. In nano
, you can do this by pressing Ctrl+O
to write out the file, then Enter
to confirm, and Ctrl+X
to exit.
6. Make the Script Executable
To run the script, you need to make it executable. Use the chmod
command to change the file permissions: chmod +x myscript.sh
7. Run the Script
You can now run your script by typing ./
followed by the script name: ./myscript.sh
Example of a Complete Script
Here’s another example that checks if a directory exists and creates it if it doesn’t, then writes the username to a file within that directory:
#!/bin/bash
# Define the directory and file name
DIR="/tmp/mytestdir"
FILE="$DIR/username.txt"
# Check if the directory exists
if [ ! -d "$DIR" ]; then
# If not, create the directory
mkdir -p "$DIR"
echo "Directory $DIR created."
else
echo "Directory $DIR already exists."
fi
# Write the current user to the file
echo "The current user is: $(whoami)" > "$FILE"
echo "Username written to $FILE."
# Output the contents of the file
cat "$FILE"
Running the Script
Follow the same steps to create, save, and run this script. This script will:
Check if
/tmp/mytestdir
exists.Create the directory if it does not exist.
Write the current username to a file named
username.txt
inside that directory.Display the contents of
username.txt
.
Tips for Writing Shell Scripts
Comments: Use
#
to add comments to your script for better readability.Variables: Store reusable values in variables.
Conditionals: Use
if
,elif
, andelse
to make decisions.Loops: Use
for
,while
, anduntil
to repeat tasks.Functions: Define functions for reusable blocks of code.
Subscribe to my newsletter
Read articles from Mohi uddin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
