The Fundamentals of Sorting Algorithms: A Comprehensive Guide
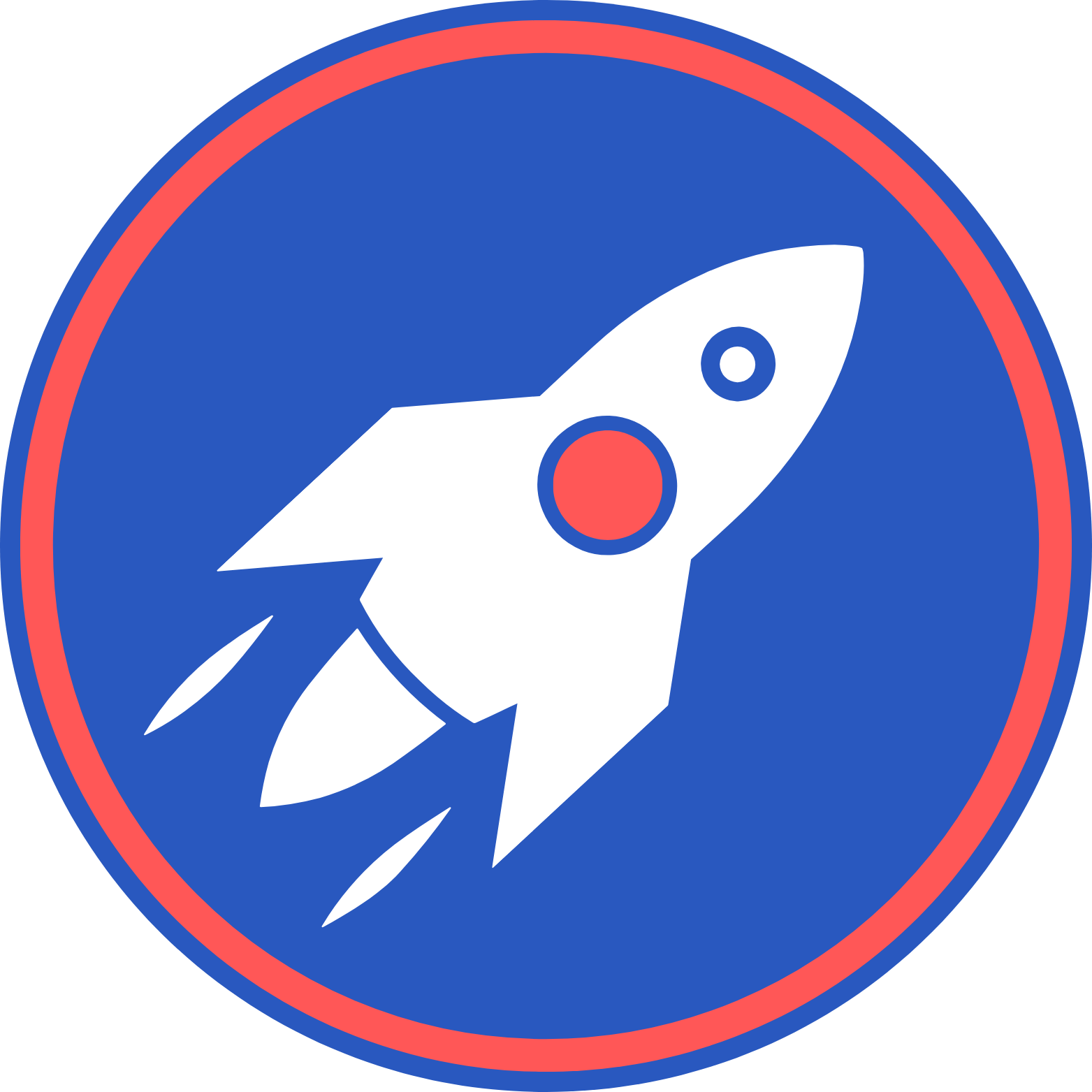
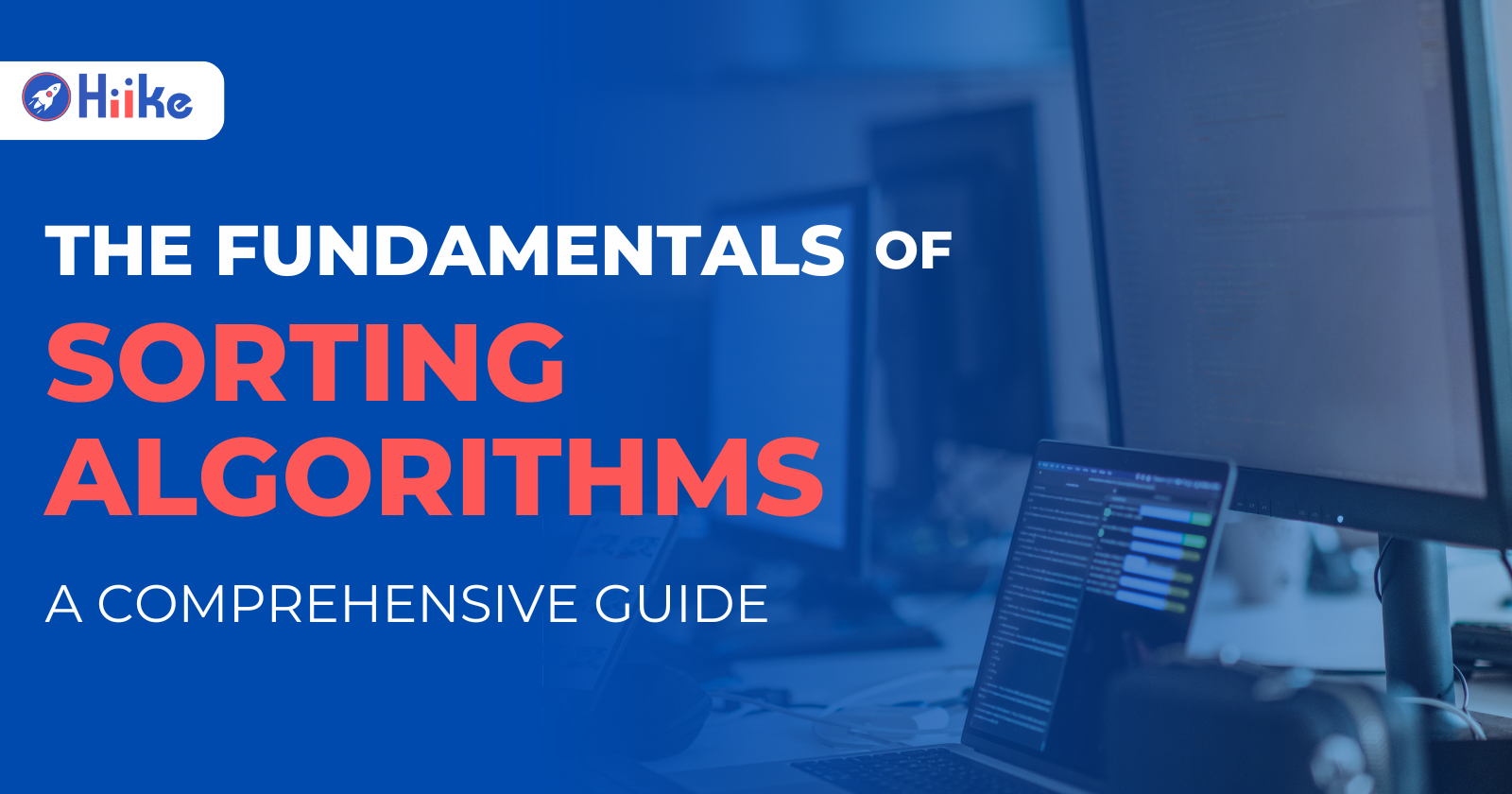
Sorting algorithms play a crucial role in computer science and programming, enabling us to organize data efficiently. In this comprehensive guide, we'll provide an overview of sorting algorithms, explain each algorithm in detail, present practical examples and exercises, compare their performance and efficiency, and explore their applications and use cases.
Overview of Sorting Algorithms
Sorting algorithms are algorithms that arrange elements of a list or array in a specific order, such as numerical or lexicographical order. They are fundamental to various computer science tasks and are used extensively in software development, database systems, and more. Sorting algorithms can be categorized based on their complexity, stability, and adaptability.
Detailed Explanation of Each Algorithm
1. Bubble Sort
Bubble Sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. It continues until no swaps are required, indicating that the list is sorted.
2. Selection Sort
Selection Sort divides the input list into two parts: a sorted sublist and an unsorted sublist. It repeatedly finds the minimum element from the unsorted sublist and swaps it with the first element of the unsorted sublist.
3. Insertion Sort
Insertion Sort builds a sorted list one element at a time by repeatedly taking an element from the unsorted part and inserting it into its correct position in the sorted part.
4. Merge Sort
Merge Sort is a divide-and-conquer algorithm that divides the input list into smaller sublists, sorts them recursively, and then merges the sorted sublists to produce a single sorted list.
5. Quick Sort
Quick Sort is another divide-and-conquer algorithm that selects a pivot element, partitions the list into two sublists (elements less than the pivot and elements greater than the pivot), and recursively sorts the sublists.
Practical Examples and Exercises
To solidify your understanding of sorting algorithms, let's consider a practical example of sorting an array of integers in ascending order using each algorithm. We'll provide step-by-step explanations and code samples for implementation. Additionally, we'll include exercises for you to practice and reinforce your knowledge.
Comparison of Performance and Efficiency
We'll compare the performance and efficiency of each sorting algorithm in terms of time complexity, space complexity, stability, and adaptability. Understanding the trade-offs and characteristics of different sorting algorithms is essential for selecting the most suitable algorithm for a given scenario.
Applications and Use Cases
Sorting algorithms find applications in various domains, including:
Database Management: Sorting records in a database table.
Algorithm Design: Building other algorithms that require sorted input.
Data Processing: Analyzing and organizing large datasets.
User Interfaces: Displaying data in sorted order in user interfaces.
Conclusion
Sorting algorithms are fundamental to computer science and programming, enabling efficient organization and manipulation of data. By understanding the fundamentals of sorting algorithms, practicing with practical examples and exercises, and exploring their applications and use cases, you can enhance your problem-solving skills and become a more proficient software engineer. Join Hiike's Top 30 Program and embark on a journey to master sorting algorithms and other essential concepts in data structures and algorithms!
Subscribe to my newsletter
Read articles from Hiike directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
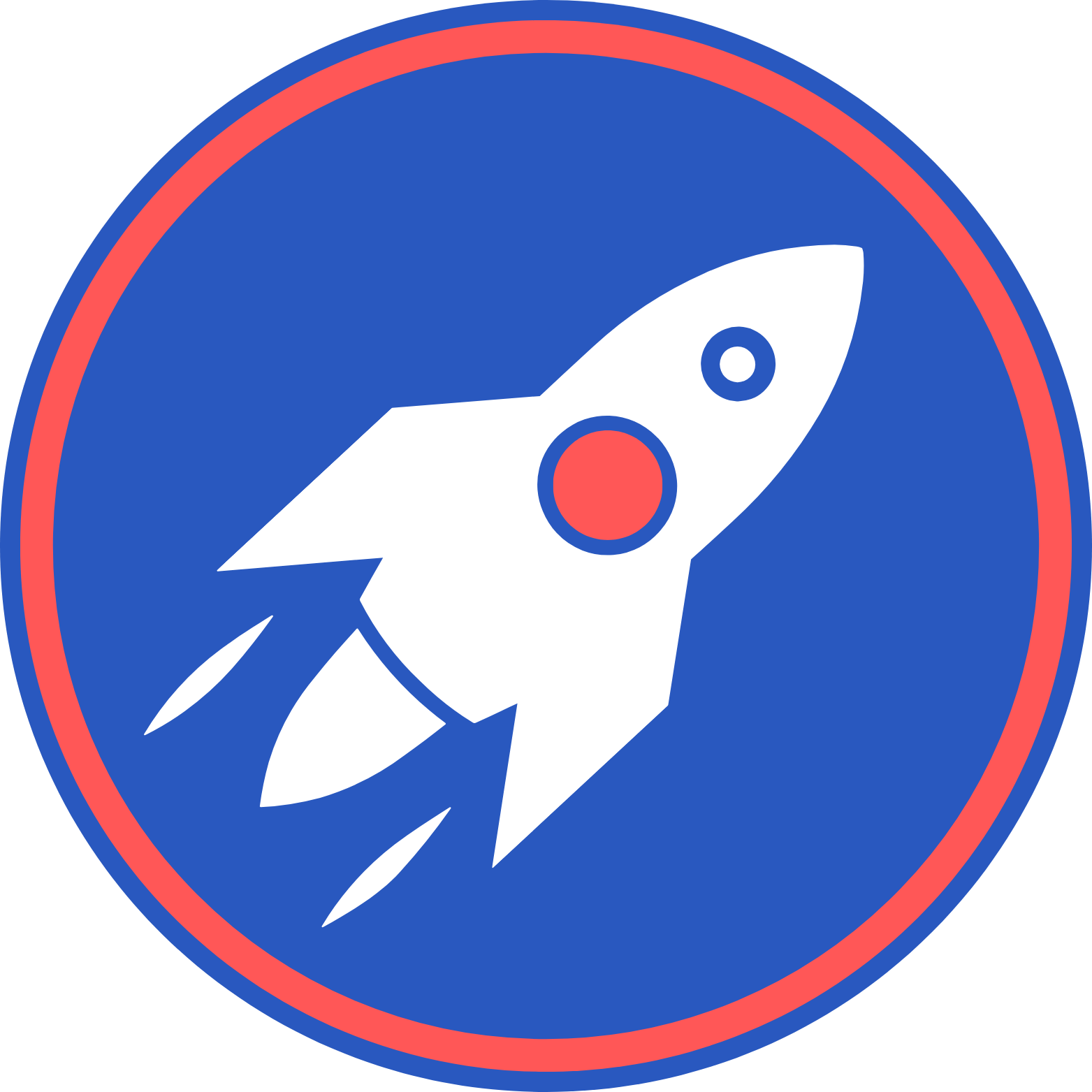
Hiike
Hiike
๐๐๐ข๐ข๐ค๐ - Crafting Your Tech Odyssey! ๐ Join Hiike for immersive learning, expert mentorship, and accelerated career growth. Our IITian-led courses and vibrant community ensure you excel in Data Structures, Algorithms, and System Design. Transform your tech career with Hiike today!