Real World ML: Early Stopping in Deep Learning: A Comprehensive Guide
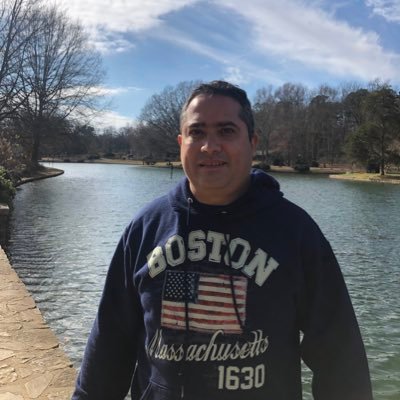
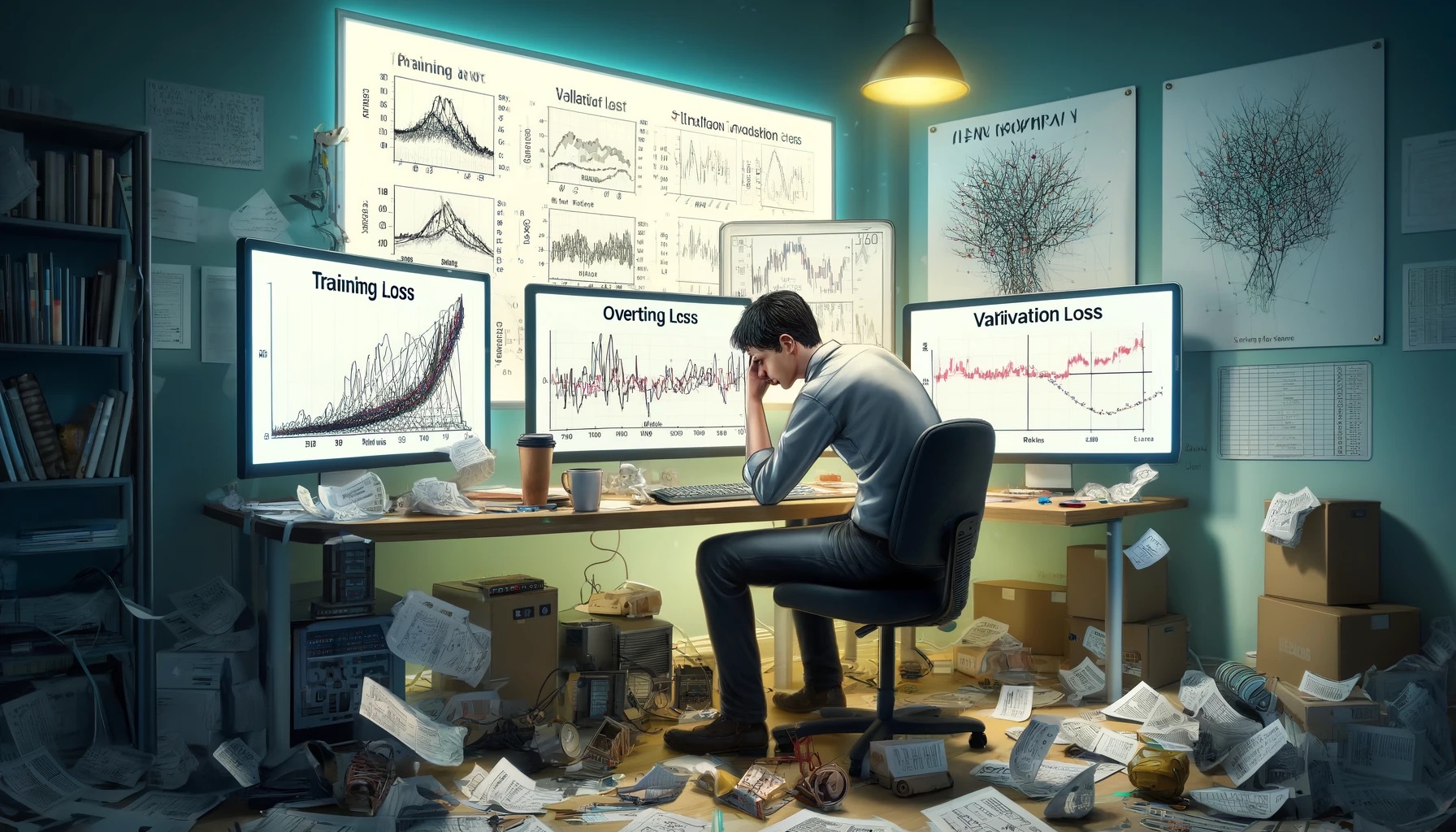
Have you ever spent days training a deep learning model, only to find out it performs poorly on new data?
This is a common frustration for many data scientists and machine learning engineers.
Imagine pouring countless hours into fine-tuning your neural network, only to realize it’s overfitting or underfitting.
The cost of wasted time and resources is immense, not to mention the emotional toll of seeing your hard work fail.
The good news? There’s a technique that can help: early stopping.
Early stopping halts the training process at the optimal point, preventing overfitting and ensuring your model generalizes well.
In this article, we’ll explore how early stopping works and how it can save you time and resources, making your deep learning models more effective and efficient.
The Problem of Overfitting and Underfitting
Deep learning models, particularly neural networks, are powerful tools for a variety of tasks.
When training a deep learning model, one of the primary goals is to achieve good generalization performance on unseen data.
However, two common pitfalls can hinder this objective: overfitting and underfitting.
Overfitting occurs when a model learns the training data too well, capturing noise and irrelevant patterns specific to the training set.
As a result, the model performs exceptionally well on the training data but fails to generalize to new, unseen examples.
On the other hand, underfitting happens when a model has a poor performance on both the training and test data.
Finding the right balance between overfitting and underfitting is crucial for building robust and reliable deep learning models.
Impact of Epochs on Overfitting and Underfitting
When a model trains for too many epochs, it starts to memorize the training data, including noise and outliers.
This memorization leads to poor performance on new, unseen data.
On the flip side, if a model trains for too few epochs, it may not learn the underlying patterns in the data, leading to underfitting.
Early stopping aims to find the sweet spot—the point at which the model performs best on the validation set.
The Mechanics of Early Stopping
Early stopping is a regularization technique that aims to find the optimal point at which to halt the training process of a deep learning model.
This technique uses a hold-out validation set and a performance metric, such as loss, to monitor the model’s progress.
During training, the model's performance is evaluated on both the training set and a separate validation set.
When the model's performance on the validation set stops improving and starts to degrade, early stopping triggers the halt of training.
Typically, the training loss decreases continuously, while the validation loss decreases initially but starts to increase once overfitting begins.
Early stopping aims to stop training at the inflection point where the validation loss is lowest.
Here's how early stopping works:
Split the available data into three sets: training, validation, and test sets.
Train the model on the training set for a certain number of epochs.
Make sure the best model is saved at each epoch, early stopping often involves checkpointing.
At each epoch, evaluate the model's performance on the validation set using a chosen metric (e.g., loss or accuracy).
If the model's performance on the validation set starts to worsen for a specified number of consecutive epochs (known as the patience), stop the training process.
Select the model checkpoint that achieved the best performance on the validation set as the final model.
Let's see the steps above in a code.
best_val_loss = float('inf')
patience = 5
patience_counter = 0
best_model = None
for epoch in range(num_epochs):
train_model(model, train_data)
val_loss = evaluate_model(model, val_data)
if val_loss < best_val_loss:
best_val_loss = val_loss
patience_counter = 0
best_model = model.save_weights()
else:
patience_counter += 1
if patience_counter >= patience:
break
model.load_weights(best_model)
By applying early stopping, we can prevent the model from overfitting to the training data and find the sweet spot where it generalizes well to unseen examples.
Benefits of Early Stopping
Early stopping offers several benefits in deep learning:
Regularization: Early stopping acts as a regularization technique by preventing the model from overfitting to the training data. It encourages the model to find a balance between fitting the training data and generalizing to unseen examples.
Computational Efficiency: By stopping the training process early, we can save computational resources and time. Instead of training the model for an excessive number of epochs, early stopping allows us to find the optimal stopping point efficiently.
Automated Tuning: Early stopping automates the process of determining the optimal number of training epochs. It eliminates the need for manual intervention and helps find the sweet spot for stopping the training.
Robustness: Early stopping makes the model more robust to variations in the training data and hyperparameters. It reduces the model's sensitivity to noise and outliers in the training set.
Considerations and Limitations
While early stopping is a powerful technique, there are a few considerations and limitations to keep in mind:
Validation Set Size: The effectiveness of early stopping depends on the quality and size of the validation set. If the validation set is too small or not representative of the true data distribution, early stopping may not provide accurate guidance.
Patience Setting: The choice of the patience parameter can impact the performance of early stopping. Setting the patience too low may lead to premature stopping, while setting it too high may result in unnecessary training. Experimenting with different patience values can help find the optimal setting.
Noisy Validation Loss: In some cases, the validation loss may exhibit noisy or fluctuating behavior, making it challenging to determine the optimal stopping point. Techniques such as smoothing the validation loss curve or using a moving average can help mitigate this issue.
Generalization Gap: Early stopping relies on the assumption that the validation set is representative of the true data distribution. If there is a significant difference between the validation set and the actual test set, the model's performance may not generalize well to the test set.
Implementing Early Stopping
Let’s consider a practical example using the MNIST dataset, a common benchmark for image classification.
from keras.datasets import mnist
from keras import Sequential
from keras.layers import Dense, Flatten
from keras.callbacks import EarlyStopping
# Load data
(x_train, y_train), (x_val, y_val) = mnist.load_data()
# Normalize data
x_train, x_val = x_train / 255.0, x_val / 255.0
# Define model
model = Sequential([
Flatten(input_shape=(28, 28)),
Dense(128, activation='relu'),
Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Set up early stopping
early_stopping = EarlyStopping(
monitor='val_loss',
patience=3,
restore_best_weights=True
)
# Train model
history = model.fit(
x_train, y_train,
epochs=50,
validation_data=(x_val, y_val),
callbacks=[early_stopping]
)
Conclusion
Early stopping is a powerful technique for training deep learning models.
It strikes a balance between underfitting and overfitting, ensuring the model generalizes well.
By monitoring the validation loss and halting training at the right moment, early stopping prevents overfitting and saves computational resources.
Implementing early stopping requires setting up a validation set, choosing a suitable performance metric, defining the patience parameter, and saving the best model checkpoint during training.
While early stopping offers benefits such as regularization, computational efficiency, and automated tuning, it is important to consider factors such as the validation set size, patience setting, and potential noisy validation loss.
By leveraging early stopping in your deep learning projects, you can build more robust and reliable models that strike a balance between fitting the training data and generalizing to unseen examples.
With the power of early stopping, you can optimize your models' performance and unlock the full potential of deep learning in solving complex real-world problems.
So, next time you train a deep learning model, consider incorporating early stopping to enhance your training process.
PS:
If you like this article, share it with others ♻️
Would help a lot ❤️
And feel free to follow me for articles more like this.
Subscribe to my newsletter
Read articles from Juan Carlos Olamendy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
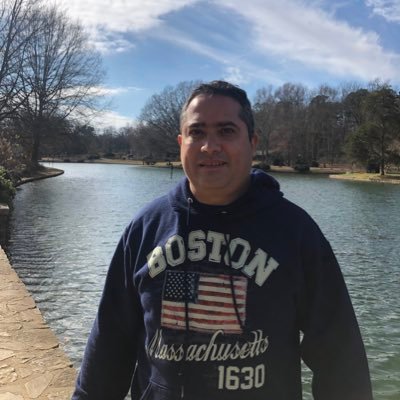
Juan Carlos Olamendy
Juan Carlos Olamendy
🤖 Talk about AI/ML · AI-preneur 🛠️ Build AI tools 🚀 Share my journey 𓀙 🔗 http://pixela.io