Day 66 - Terraform Hands-on Project - Build Your Own AWS Infrastructure with Ease using Infrastructure as Code (IaC) Techniques βοΈ
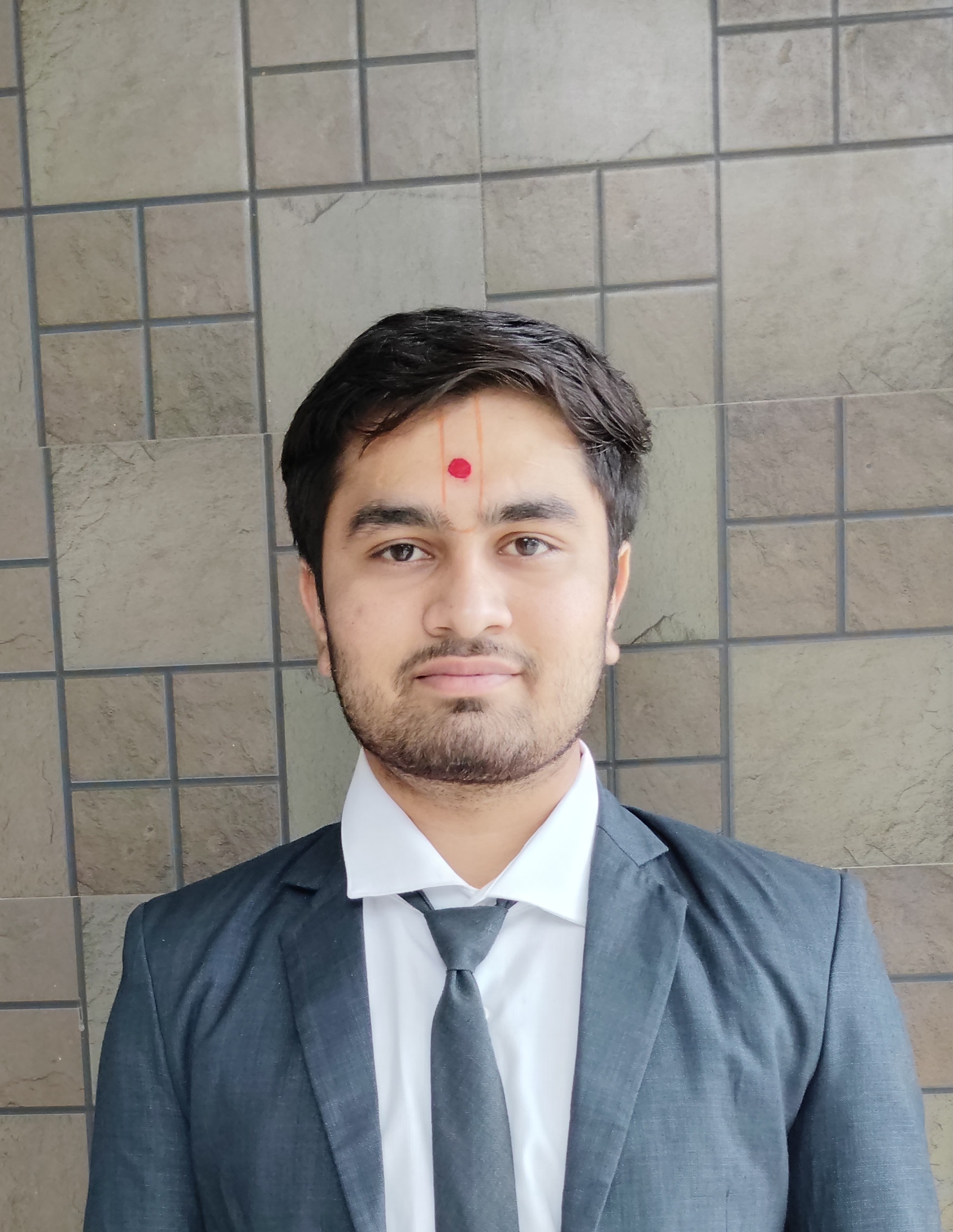
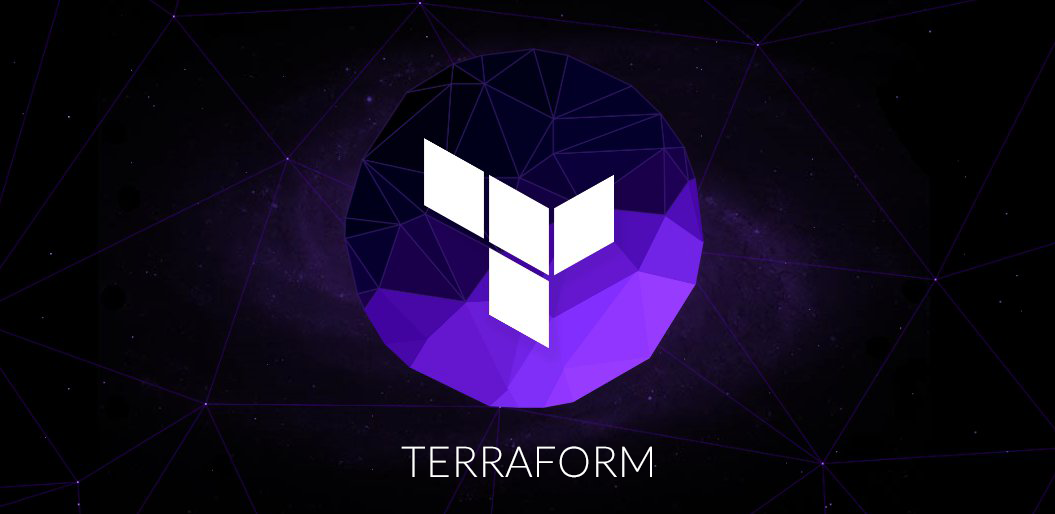
Let's dive into Day 66 of your 90 Days of DevOps Challenge. Today's focus is on a hands-on project using Terraform to build AWS infrastructure. This project will help you understand how to use Infrastructure as Code (IaC) techniques to manage AWS resources. Let's get started! π
Welcome back to your Terraform journey.
In the previous tasks, you have learned about the basics of Terraform, its configuration file, and creating an EC2 instance using Terraform. Today, we will explore more about Terraform and create multiple resources.
Prerequisites:
Terraform installed on your local machine
AWS CLI configured with your AWS credentials
Basic knowledge of Terraform and AWS
Install Terraform:
sudo apt-get update
sudo apt-get install -y gnupg software-properties-common curl
curl -fsSL https://apt.releases.hashicorp.com/gpg | sudo apt-key add -
sudo apt-add-repository "deb [arch=amd64] https://apt.releases.hashicorp.com $(lsb_release -cs) main"
sudo apt-get update
sudo apt-get install terraform
terraform -version
1. Create a VPC (Virtual Private Cloud) with CIDR block 10.0.0.0/16 π
Define the VPC Resource:
resource "aws_vpc" "main" { cidr_block = "10.0.0.0/16" tags = { Name = "main" } }
- This code block creates a VPC with the specified CIDR block and tags it with the name "main". π
Set the Terraform Version and AWS Region:
terraform { required_providers { aws = { source = "hashicorp/aws" version = "~> 4.16" } } required_version = ">= 1.2.0" } provider "aws" { region = "us-east-1" }
- Specifies the Terraform version and AWS region. βοΈ
Initialize and Apply Configuration:
terraform init terraform apply
terraform init
initializes the working directory. π οΈterraform apply
creates the VPC in your AWS account. π
Verify in AWS Console:
- Go to the VPC console and check if the VPC named "main" is created successfully. βοΈ
2. Create a Public Subnet with CIDR block 10.0.1.0/24 π‘
Step-by-Step:
Define the Public Subnet Resource:
resource "aws_subnet" "public_subnet" { vpc_id = aws_vpc.main.id cidr_block = "10.0.1.0/24" tags = { Name = "Public Subnet" } }
Creates a public subnet with the specified CIDR block in the VPC. ποΈ
Apply Configuration:
terraform apply
Runs the configuration to create the public subnet. οΏ½
Verify in AWS Console:
Go to the VPC console, then to Subnets, and check if the "Public Subnet" is created. β
3. Create a Private Subnet with CIDR block 10.0.2.0/24 π
Define the Private Subnet Resource:
resource "aws_subnet" "private_subnet" { vpc_id = aws_vpc.main.id cidr_block = "10.0.2.0/24" tags = { Name = "Private Subnet" } }
- Creates a private subnet with the specified CIDR block in the VPC. π‘
Apply Configuration:
terraform apply
Runs the configuration to create the private subnet. π
Verify in AWS Console:
Go to the VPC console, then to Subnets, and check if the "Private Subnet" is created. βοΈ
4. Create an Internet Gateway (IGW) and Attach it to the VPC π
Define the Internet Gateway Resource:
resource "aws_internet_gateway" "gw" { vpc_id = aws_vpc.main.id tags = { Name = "igw" } }
Creates an Internet Gateway and attaches it to the VPC. π
Apply Configuration:
terraform apply
Runs the configuration to create the Internet Gateway. π
Verify in AWS Console:
Go to the VPC console, then to Internet Gateways, and check if the Internet Gateway named "igw" is created and attached to the VPC. βοΈ
5. Create a Route Table for the Public Subnet and Associate It with the Public Subnet π€οΈ
Define the Route Table Resource:
resource "aws_route_table" "public" { vpc_id = aws_vpc.main.id route { cidr_block = "0.0.0.0/0" gateway_id = aws_internet_gateway.gw.id } tags = { Name = "route-table" } }
- Creates a route table for the VPC with a route to the Internet Gateway. πΊοΈ
Associate Route Table with Public Subnet:
resource "aws_route_table_association" "public" { subnet_id = aws_subnet.public_subnet.id route_table_id = aws_route_table.public.id }
Associates the route table with the public subnet. π
Apply Configuration:
terraform apply
- Runs the configuration to create and associate the route table. π
Verify in AWS Console:
Go to the VPC console, then to Route Tables, and check if the route table is created and associated with the public subnet. βοΈ
Route table routes with internet gateway.
Route table is associate with public subnet using terraform.
6. Launch an EC2 Instance in the Public Subnet π»
Define the Security Group Resource:
resource "aws_security_group" "ssh_access" { name_prefix = "ssh_access" vpc_id = aws_vpc.main.id ingress { from_port = 22 to_port = 22 protocol = "tcp" cidr_blocks = ["0.0.0.0/0"] } ingress { from_port = 80 to_port = 80 protocol = "tcp" cidr_blocks = ["0.0.0.0/0"] } } resource "aws_eip" "eip" { instance = aws_instance.web_server.id tags = { Name = "test-eip" } }
Creates a security group to allow SSH (port 22) and HTTP (port 80) access. π
Define the EC2 Instance Resource:
resource "aws_instance" "web_server" { ami = "ami-0ddda618e961f2270" instance_type = "t2.micro" key_name = "batch-6-key" subnet_id = aws_subnet.public_subnet.id vpc_security_group_ids = [ aws_security_group.ssh_access.id ] user_data = <<-EOF #!/bin/bash sudo apt-get update -y sudo apt-get install apache2 -y sudo systemctl start apache2 sudo systemctl enable apache2 echo "<html><body><h1>Welcome to my website!</h1></body></html>" > /var/www/html/index.html sudo systemctl restart apache2 EOF tags = { Name = "terraform-instance" } }
Launches an EC2 instance in the public subnet with the specified AMI, instance type, and security group. The
user_data
script installs Apache and sets up a basic website. πRun terraform apply to create the EC2 instance in your AWS account.
Apply Configuration:
terraform apply
- Runs the configuration to create the EC2 instance. π
Verify in AWS Console:
Go to the EC2 console and check if the instance named "terraform-instance" is created. βοΈ
7. Open the website URL in a browser to verify that the website is hosted successfully.ππ
By following these steps, you will successfully build and deploy AWS infrastructure using Terraform. Happy Terraforming! π
Interview Tips π‘
Be prepared to explain each Terraform resource and its purpose.
Understand the CIDR block notation and its significance in networking.
Highlight the importance of IaC and how Terraform helps in automating infrastructure.
That's it for today. You've successfully built an AWS infrastructure using Terraform! Keep practicing and happy Terraforming! π
Subscribe to my newsletter
Read articles from Nilkanth Mistry directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
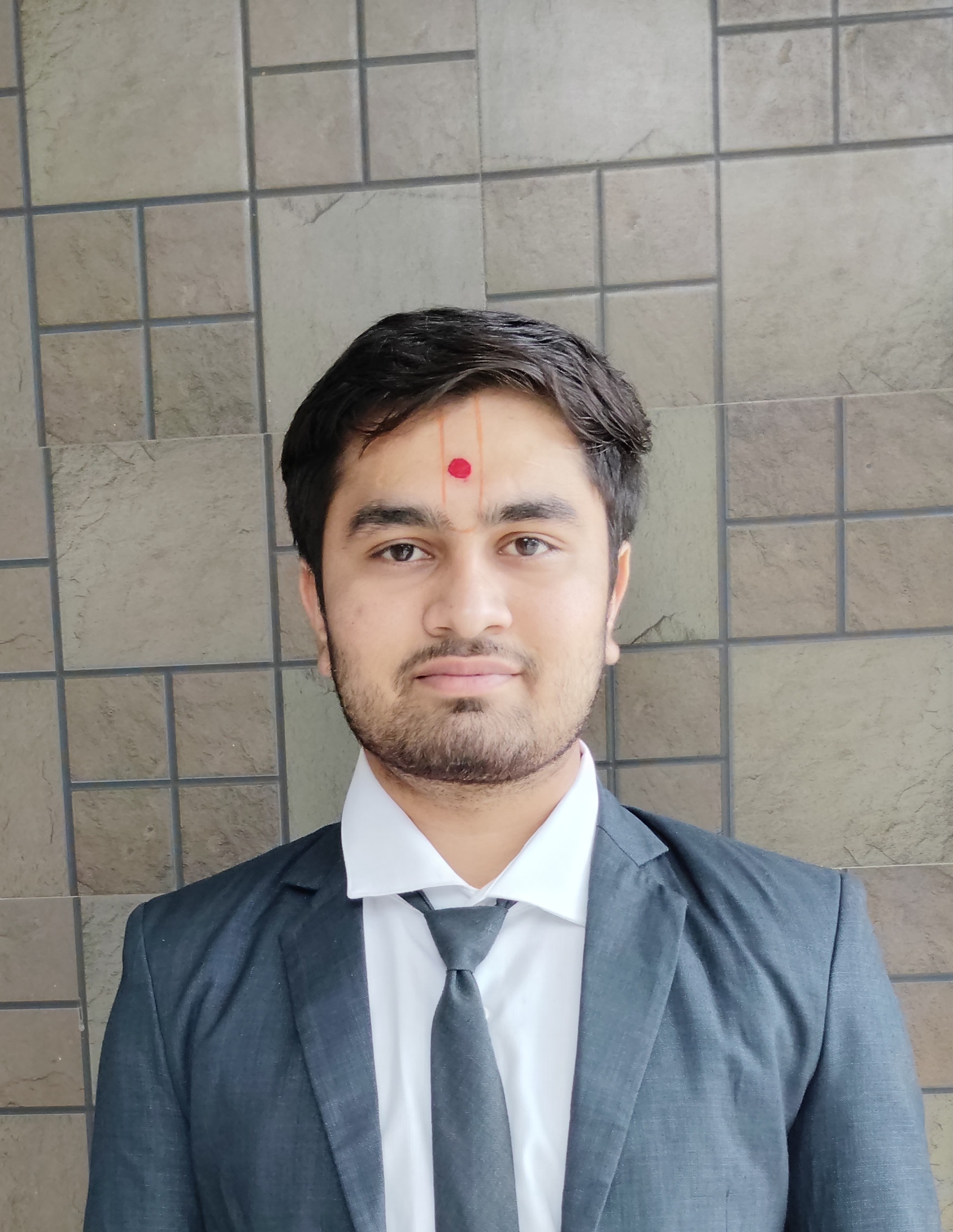
Nilkanth Mistry
Nilkanth Mistry
Embark on a 90-day DevOps journey with me as we tackle challenges, unravel complexities, and conquer the world of seamless software delivery. Join my Hashnode blog series where we'll explore hands-on DevOps scenarios, troubleshooting real-world issues, and mastering the art of efficient deployment. Let's embrace the challenges and elevate our DevOps expertise together! #DevOpsChallenges #HandsOnLearning #ContinuousImprovement