Creating an Engaging Animated Loader with HTML and CSS
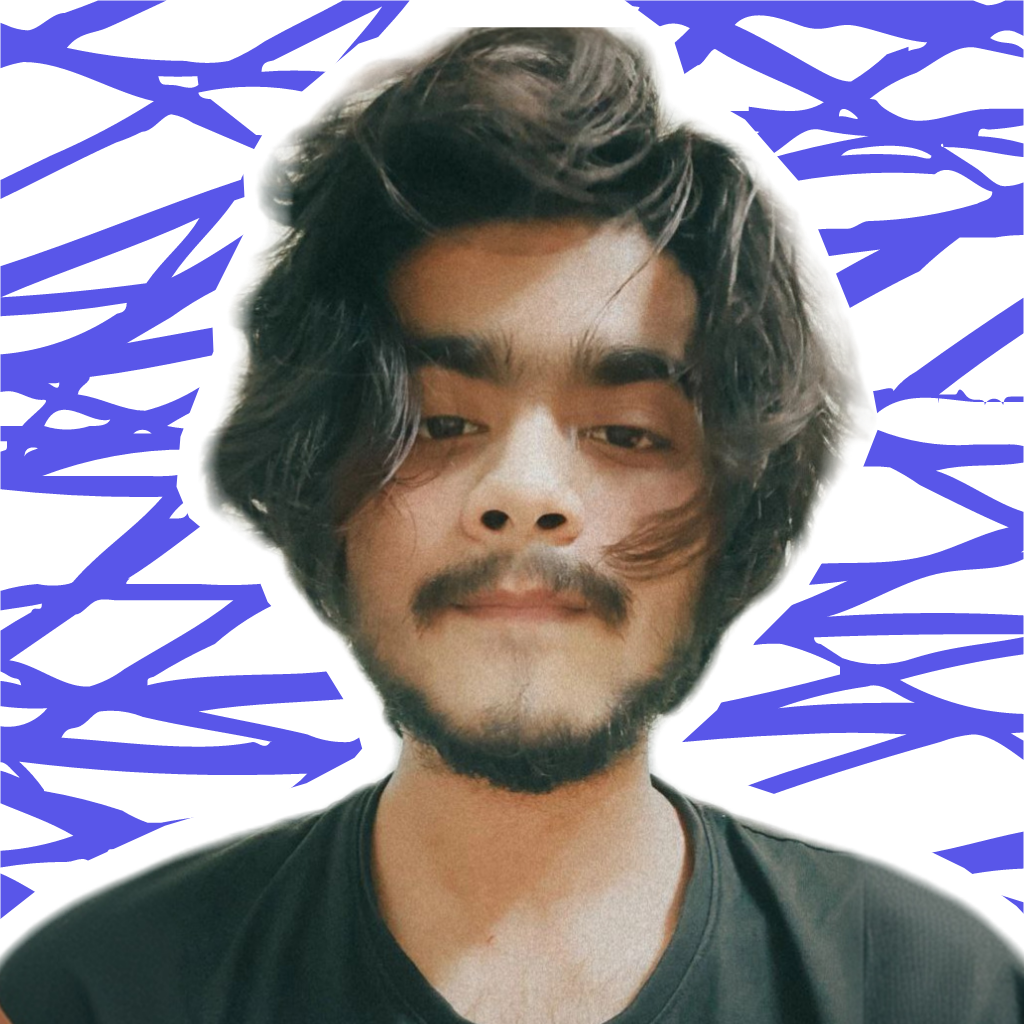
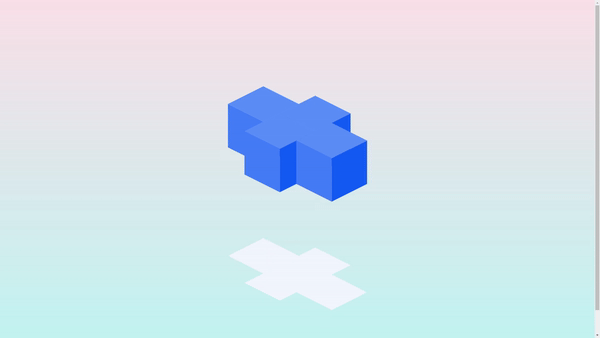
Introduction
Creating eye-catching web animations is a vital skill in web development. In this guide, we’ll walk through creating an animated loader using HTML and CSS. This project is part of day 50 of the #100DaysOfCode Challenge.
Step-by-Step Guide to Building the Animated Loader
Step 1: Setting Up the HTML Structure
To start, we need to create the basic HTML structure. This includes defining the document type, setting metadata, linking the CSS file, and creating the container for our animated boxes.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>Animated Loader</title>
</head>
<body>
<div class="boxes">
<div class="box">
<div></div>
<div></div>
<div></div>
<div></div>
</div>
<div class="box">
<div></div>
<div></div>
<div></div>
<div></div>
</div>
<div class="box">
<div></div>
<div></div>
<div></div>
<div></div>
</div>
<div class="box">
<div></div>
<div></div>
<div></div>
<div></div>
</div>
</div>
</body>
</html>
Step 2: Styling with CSS
Next, we’ll add CSS to style the body, container, and boxes. We’ll also define keyframes for the animations.
Styling the Body
The body will use flexbox to center the content and a gradient background for visual appeal.
body {
display: flex;
align-items: center;
justify-content: center;
min-height: 100vh;
background-image: linear-gradient(to top, #bef5f2 0%, #fbe1e9 100%);
}
Styling the Container
The container holds the boxes and applies initial 3D transformations.
.boxes {
--size: 32px;
--duration: 800ms;
height: calc(var(--size) * 2);
width: calc(var(--size) * 3);
position: relative;
transform-style: preserve-3d;
transform-origin: 50% 50%;
margin-top: calc(var(--size) * 1.5 * -1);
transform: rotateX(60deg) rotateZ(45deg) rotateY(0deg) translateZ(0px);
}
Styling the Boxes
Each box has specific dimensions and absolute positioning.
.boxes .box {
width: var(--size);
height: var(--size);
position: absolute;
transform-style: preserve-3d;
}
Step 3: Adding Animations
We’ll define keyframes to animate each box’s position.
Animation for the First Box
.boxes .box:nth-child(1) {
transform: translate(100%, 0);
animation: box1 var(--duration) linear infinite;
}
@keyframes box1 {
0%, 50% {
transform: translate(100%, 0);
}
100% {
transform: translate(200%, 0);
}
}
Animation for the Second Box
.boxes .box:nth-child(2) {
transform: translate(0, 100%);
animation: box2 var(--duration) linear infinite;
}
@keyframes box2 {
0% {
transform: translate(0, 100%);
}
50% {
transform: translate(0, 0);
}
100% {
transform: translate(100%, 0);
}
}
Animation for the Third Box
.boxes .box:nth-child(3) {
transform: translate(100%, 100%);
animation: box3 var(--duration) linear infinite;
}
@keyframes box3 {
0%, 50% {
transform: translate(100%, 100%);
}
100% {
transform: translate(0, 100%);
}
}
Animation for the Fourth Box
.boxes .box:nth-child(4) {
transform: translate(200%, 0);
animation: box4 var(--duration) linear infinite;
}
@keyframes box4 {
0% {
transform: translate(200%, 0);
}
50% {
transform: translate(200%, 100%);
}
100% {
transform: translate(100%, 100%);
}
}
Step 4: Styling Box Sides
Each side of the boxes has specific styles, including background color and transformations.
.boxes .box > div {
position: absolute;
width: 100%;
height: 100%;
background: var(--background);
transform: rotateY(var(--rotateY)) rotateX(var(--rotateX)) translateZ(var(--translateZ));
}
.boxes .box > div:nth-child(1) {
--background: #5C8DF6;
--top: 0;
--left: 0;
}
.boxes .box > div:nth-child(2) {
--background: #145af2;
--right: 0;
--rotateY: 90deg;
}
.boxes .box > div:nth-child(3) {
--background: #447cf5;
--rotateX: -90deg;
}
.boxes .box > div:nth-child(4) {
--background: #f2f6ff;
--top: 0;
--left: 0;
--translateZ: calc(var(--size) * 3 * -1);
}
Conclusion
By following these steps, you’ve created a visually appealing animated loader using HTML and CSS. This project not only enhances your web development skills but also adds a professional touch to your website. If you found this guide helpful, please consider supporting me so I can create more free prompts.
For the full source code, download it here. Contact me here.
Subscribe to my newsletter
Read articles from Aarzoo Islam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
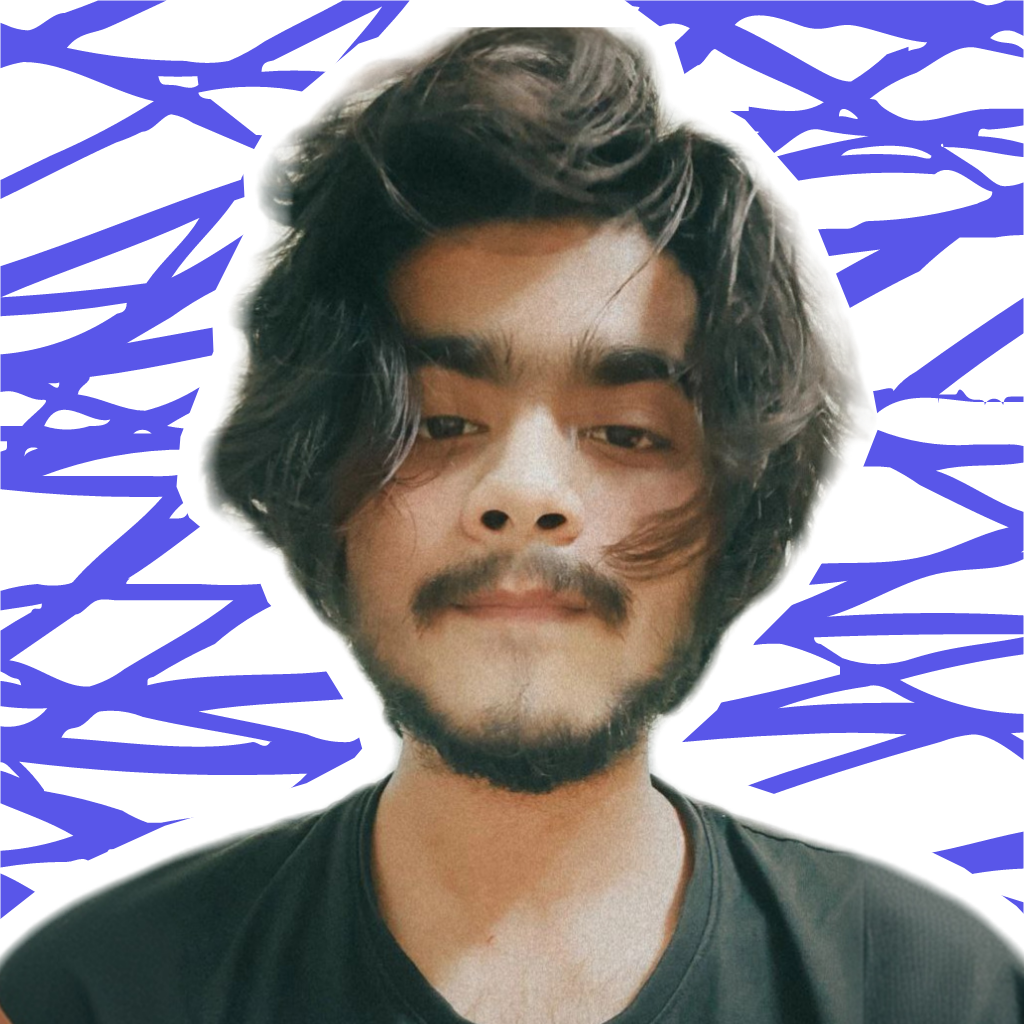
Aarzoo Islam
Aarzoo Islam
Founder of AroNus 🚀 | Full-stack web developer 👨🏻💻 | Sharing web development tips and showcasing projects 📂 | Transforming ideas into digital realities 🌟