Ultimate Next.js Tutorial for Beginners: From Setup to Deployment
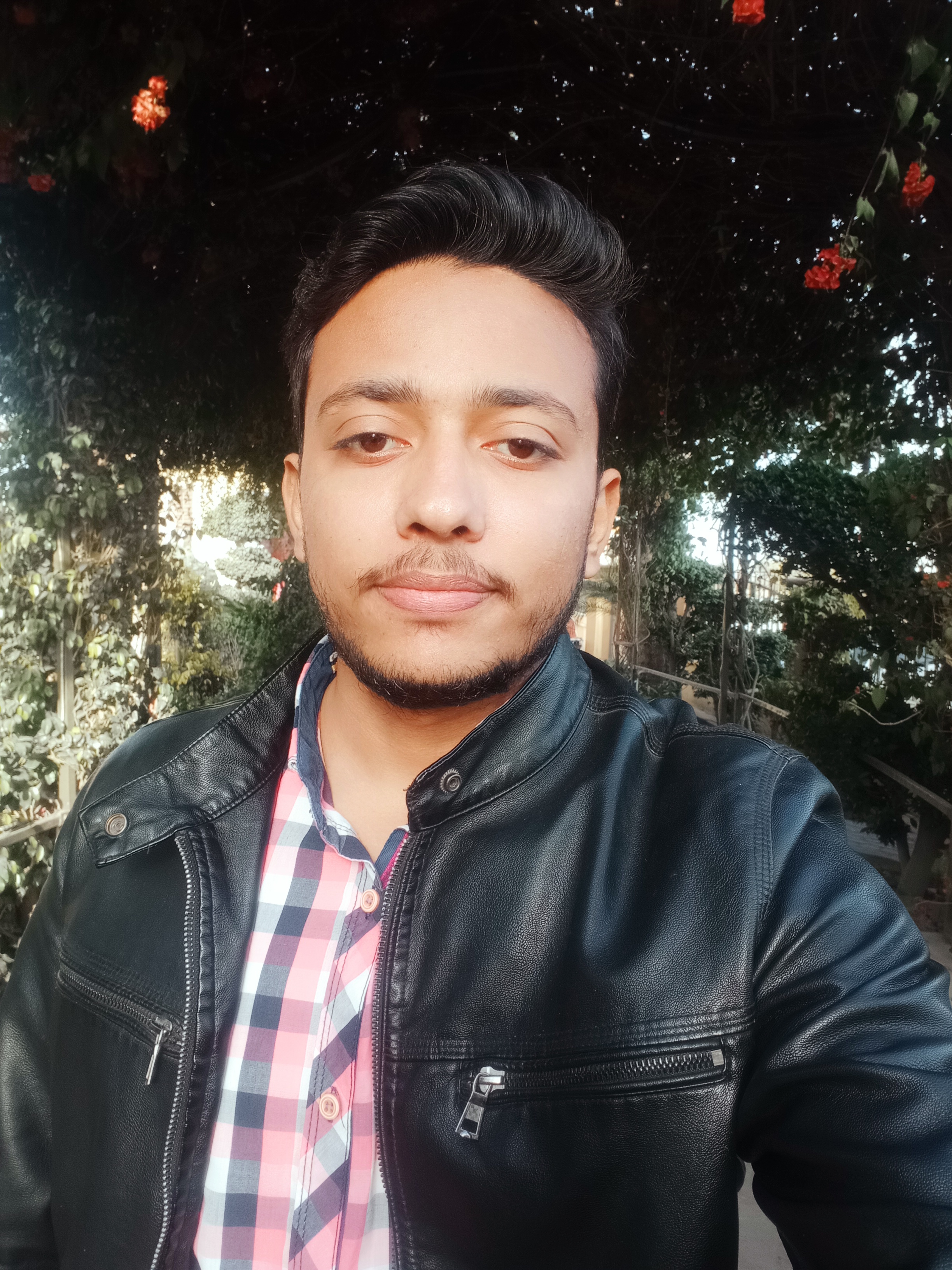
Table of contents

Next.js is an open-source React framework designed for front-end development, featuring powerful tools like server-side rendering (SSR) and static site generation (SSG) for enhanced performance and SEO.
SSR renders pages on the server before sending them to the client, ensuring faster load times and better SEO. SSG pre-renders pages at build time, allowing them to be served directly from a CDN, ensuring excellent performance and scalability.
Next.js also supports incremental static regeneration, enabling developers to update static content without a full rebuild. This makes Next.js a top choice for building high-performance, scalable, and SEO-friendly web applications. It's simply fantastic.
Prerequisites
Before we dive into Next.js, make sure you have the following:
node.js
andnpm
installed on your machine.Basic knowledge of
React
andJavaScript
orTypeScript
.A
Vercel
account. If you don’t have one, you can sign up for free.
Step 1: Kickstart Your Next.js Project
To create a new Next.js application, run the following command in your terminal:
yarn create next-app my-next-app
or
npx create-next-app@latest my-next-app
Now, Next.js will ask you some questions. Feel free to choose according to your preferences and press enter after each of your selection. In my case, I selected the following options:
Would you like to use TypeScript? » No / Yes
Would you like to use ESLint? » No / Yes
Would you like to use Tailwind CSS? » No / Yes
Would you like to use
src/
directory? » No / YesWould you like to use App Router? (recommended) » No / Yes
Would you like to customize the default import alias (@/*)? » No / Yes
This will create a new directory called my-next-app
with all the necessary files and dependencies.
Navigate into your project directory:
cd my-next-app
After running these commands, your folder structure should like this:
Step 2: Decoding the Next.js File Structure
After creating your project, you’ll notice several directories and files. The key ones are:
pages/
: Contains your application’s pages. Each JavaScript file under this directory corresponds to a route based on its file name.public/
: Static assets like images and fonts can be placed here.styles/
: Put your CSS files here.
The following file conventions are used to define routes and handle metadata in the app router.
layout | .js.jsx.tsx | Layout: root page |
page | .js.jsx.tsx | Page |
loading | .js.jsx.tsx | Loading UI: loading page |
not-found | .js.jsx.tsx | Not found UI: not found page |
error | .js.jsx.tsx | Error UI: error page |
global-error | .js.jsx.tsx | Global error UI: global error page |
route | .js.ts | API endpoint |
template | .js.jsx.tsx | Re-rendered layout |
default | .js.jsx.tsx | Parallel route fallback page |
The table provides information on Next.js file conventions, explaining which file names are used for specific purposes.
In Next.js:
Top-level folders
are used to organize your application's code and static assets.Top-level files
are used to configure your application, manage dependencies, run middleware, integrate monitoring tools, and define environment variables.
Step 3: Building Pages in Next.js
Creating a new page is as simple as adding a new file under the app/
directory. Here’s how you can create an about.js
page:
// app/page.js
import Link from "next/link";
export default function Home() {
return (
<div>
<h1>Welcome to My Next.js App</h1>
<Link href='/form'>Form Route</Link>
</div>
);
}
// app/form/page.js
"use client";
import { useState } from "react";
export default function Form() {
const [name, setName] = useState("");
const handleFormSubmit = () => {
fetch("/api/form", {
method: "POST",
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({ name }),
})
.then((response) => response.json())
.then((data) => {
console.log("Success:", data.message);
alert(data.message);
})
.catch((error) => {
console.error("Error:", error);
});
};
return (
<main>
<section className='container mx-auto'>
<h1 className='text-xl text-center font-bold text-black pb-8 pt-3'>
Send the input data to backend api
</h1>
<form
className='flex px-3 justify-center flex-wrap items-center gap-2'
onSubmit={handleFormSubmit}
>
<input
className={`outline-none border-2 min-w-[15rem] border-slate-200 max-sm:flex-1 flex-[0.75] h-14 rounded-md px-3`}
type='text'
placeholder='Please enter your name...'
onChange={(e) => setName(e.currentTarget.value)}
value={name}
name='name'
/>
<input
className={`bg-indigo-800 flex-[0.25] cursor-pointer hover:opacity-90 max-sm:flex-1 active:bg-indigo-900 rounded-md px-8 h-14 text-slate-100`}
type='submit'
value='Submit'
/>
</form>
</section>
</main>
);
}
Step 4: Seamless Navigation with Next.js Link Component
Next.js provides a Link
component to navigate between pages. Here’s how to use it:
// app/page.js
import Link from "next/link";
export default function Home() {
return (
<div>
<h1>Welcome to My Next.js App</h1>
<Link href='/form'>Form Route</Link>
</div>
);
}
Step 5: Crafting API Routes in Next.js
Next.js allows you to create API endpoints as part of your application. In Next.js API endpoints, you use POST
, GET
, PUT
, and DELETE
as function names and export them. However, make sure not to export them as default and always return the function; otherwise, the server will send an error.
Here’s a simple example where we get the username from the body and then send a response in the POST
method and a response in the GET
method:
// app/api/hello/route.js
// Handling POST Request
export async function POST(req) {
const body = await req.json();
/* Save it using mongodb or prisma or some other logic */
return Response.json({
message: `Hello ${body.name}!`,
});
}
// Handling GET Reqeuest
export async function GET() {
return Response.json({
message: "Next.js is amazing!"
});
}
Now, your folder structure should look like this:
Step 6: Effortless Deployment of Your Next.js App on Vercel
Deploying your Next.js app is straightforward with Vercel, the creators of Next.js. Simply push your code to a GitHub repository and import it into Vercel.
or
Install Vercel CLI
First, we need to install the Vercel
CLI (Command Line Interface). Open your terminal and run the following command:
npm i -g vercel
Login to Vercel
Next, log in to your Vercel account via the CLI. Run the following command and follow the prompts:
vercel login
Deploy Your Next.js Project
Navigate to your Next.js project directory using the cd
command if you are not in the root directory of your project:
cd path-to-your-nextjs-project
Then, deploy your project by simply running:
vercel
During the deployment process, Vercel CLI will ask you some questions. For most of them, you can use the default answers by pressing Enter
.
Visit Your Deployed Application
After the deployment is successful, Vercel CLI will provide a URL to your deployed application. Copy this URL and paste it into your web browser to see your Next.js application live!
Conclusion
In this guide, we have explored the fundamentals of Next.js, from setting up a project to understanding its file structure, creating pages, linking between them, creating API routes, and finally deploying the application using Vercel. With these steps, you are well-equipped to start building high-performance, scalable, and SEO-friendly web applications with Next.js. Happy coding!
Subscribe to my newsletter
Read articles from Muhammad Usman Tariq directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
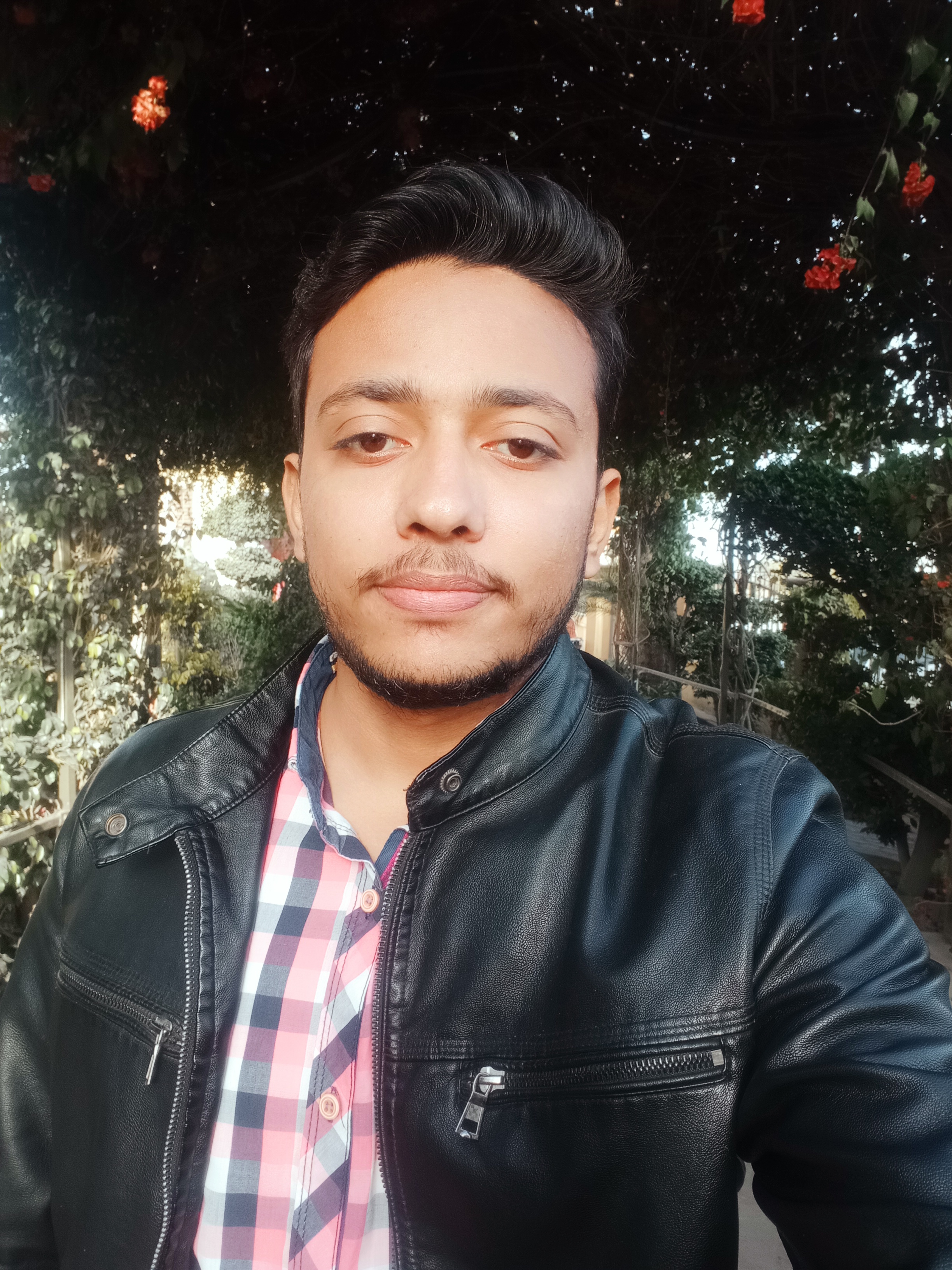
Muhammad Usman Tariq
Muhammad Usman Tariq
Hello, I'm Muhammad Usman Tariq, a dedicated and passionate Full Stack Developer. With a knack for problem-solving and an insatiable thirst for new knowledge, I am always on the lookout for innovative solutions to complex problems. My journey in the tech industry has been driven by my curiosity and the joy of turning ideas into reality. I have honed my skills in various technologies and programming languages, enabling me to build robust and scalable applications. I believe in the power of technology to transform lives and businesses, and I strive to be at the forefront of this transformation. My goal is to create software that not only meets business needs but also provides an exceptional user experience. In my spare time, I enjoy staying updated with the latest industry trends. I am always eager to learn and grow, and I welcome any opportunity that allows me to do so. Thank you for visiting my profile. Let's connect and create something amazing together!