Getting Started with C++ STL Containers: Part 1
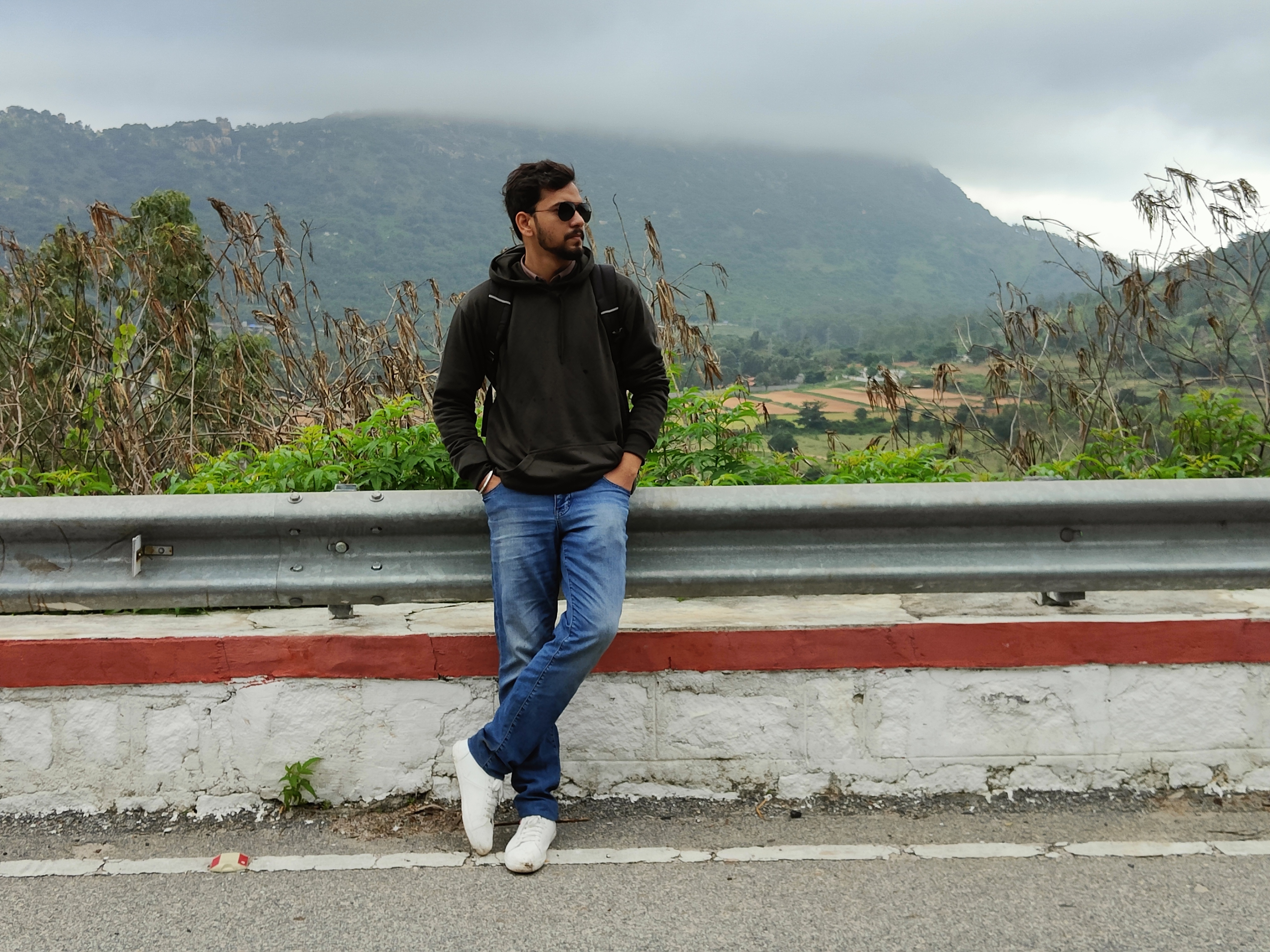
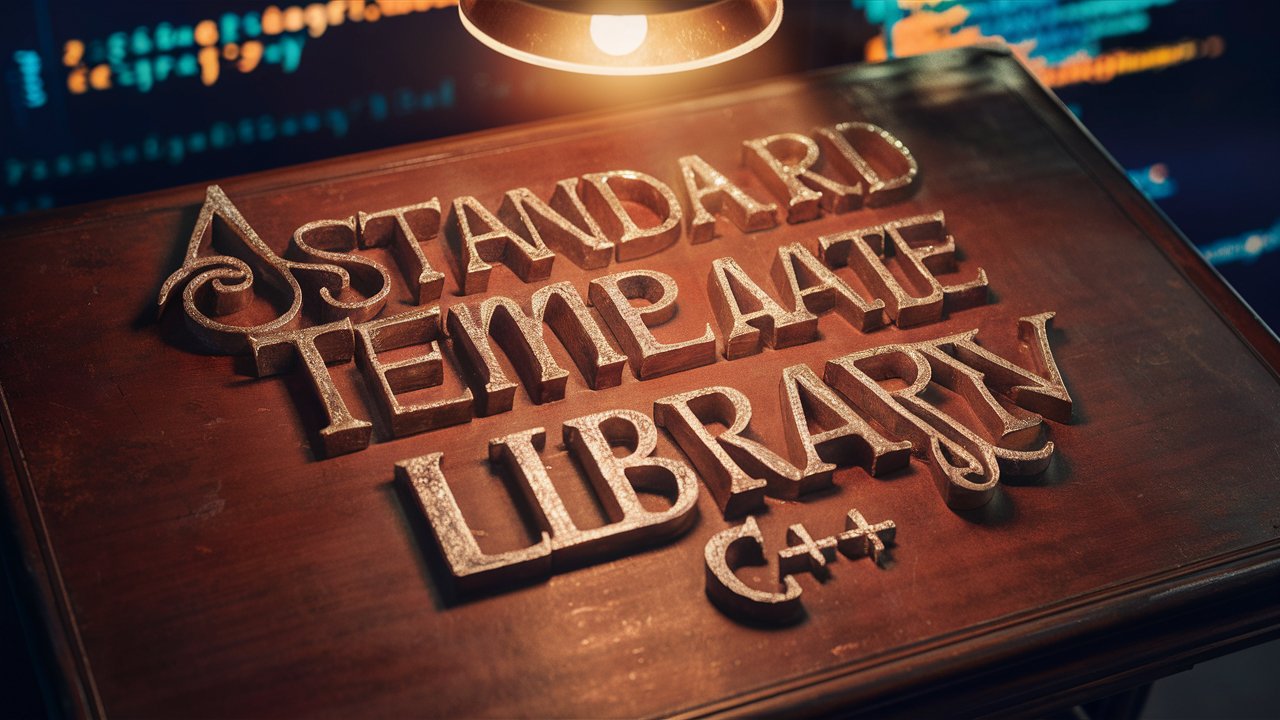
What is STL?
C++ STL stands for the "Standard Template Library". It is a powerful and extensive collection of template classes and functions in the C++ programming language that provides general-purpose classes and functions with templates that implement many popular and commonly used algorithms and Data Structures.
The C++ STL is an essential part of the C++ Standard Library, and it plays a crucial role in simplifying and accelerating C++ software development. It offers a wide range of data structures (containers) and algorithms that can be easily used in C++ programs, allowing developers to focus on solving specific problems rather than reinventing the wheel for common tasks.
Why do we need STL?
The Standard Template Library (STL) in C++ is essential for several reasons, providing numerous benefits that enhance programming efficiency, reliability, and performance. Here are some key reasons why the STL is important:
1. Predefined Data Structures and Algorithms
STL offers a wide range of commonly used data structures (like vectors, lists, stacks, queues, sets, and maps) and algorithms (like sort, search, and transform).
2. Code Reusability
STL components are generic and template-based, allowing them to be used with any data type. This promotes code reuse and reduces redundancy.
3. Efficiency and Performance
The implementations in STL are highly optimized for performance. Using STL ensures that the code is efficient and follows best practices for algorithmic complexity, which might be hard to achieve when implementing custom data structures and algorithms.
4. Consistency and Interoperability
STL provides a consistent interface across its different components, making it easier to learn and use. The consistency in naming conventions, iterator usage, and function interfaces helps in writing cleaner and more maintainable code.
5. Safety and Reliability
By leveraging STL, developers can focus on solving specific problems rather than worrying about implementing and debugging fundamental data structures and algorithms.
Common Components in STL:
Containers: vector, list, queue, stack, set, map, etc.
Algorithms: sort(), binary_search(), reverse() etc.
Iterators
Functors
We will go through each topic in detail, one by one.
1. Container:
Let's start with Container. The STL in C++ provides several container classes that store collections of objects. These containers are designed to be efficient and easy to use. They provide a standard way to store, retrieve, and manipulate data in various ways.
Vector:
A dynamic array that can grow and shrink in size.
Allows fast random access to the elements.
Insertion and removal of elements at the end is efficient.
Suitable for most scenarios when elements need to be stored in a linear sequence.
Member Functions:
begin(): Returns an iterator pointing to the first element in the vector.
end(): Returns an iterator pointing to the positions just after the last element in the vector.
Output:
size(): Returns the number of elements in the vector.
push_back(value): Adds an element to the end of the vector.
pop_back(): Removes the last element from the vector.
empty(): Checks whether a vector is empty or not.
at(index): Accesses the element at the specified index with bounds checking.
capacity(): Returns the number of elements that the vector can hold before needing to allocate more space.
reserve(n): Requests that the vector capacity be increased to at least n elements, potentially reducing the number of reallocations.
max_size(): Returns the maximum number of elements that the vector can hold due to system or library limitations.
front(): Accesses the first element in the vector.
back(): Accesses the last element in the vector.
insert(iterator position, value): Inserts a new element before the specified position in the vector.
erase(iterator first, iterator last): Removes one or more elements from the vector starting at the specified position.
clear(): Removes all elements from the vector, which are destroyed and leaves it with a size of 0.
swap(vector): Swaps the contents of the vector with those of another vector of the same type, including their sizes and capacities.
- 1D & 2D vector:
- list():
Doubly linked list that allows fast insertions and deletions from anywhere in the sequence.
No random access only sequential access.
Member Functions:
begin(): Returns an iterator pointing to the first element in the list.
end(): Returns an iterator pointing to the past-the-end element in the list.
front(): Accesses the first element in the list.
end(): Returns an iterator pointing to the past-the-end element in the list.
size(): Returns the number of elements in the list.
empty(): Checks if the list is empty or not.
clear(): Removes all elements from the list, that are destroyed, and leaves it with a size of 0.
push_back(value): Adds an element to the end of the list.
push_front(value): Adds an element to the beginning of the list.
pop_back(): Removes the last element from the list.
pop_front(): Removes the first element from the list.
remove(value): Removes all elements from the list that are equal to the specified value.
Queue():
Adapter Class that provides a First-In-First-Out (FIFO) data structure.
Implemented using other Containers (e.g., deque, list) as the underlying storage.
Member Function:
empty(): checks if the queue is empty(i.e., whether its size is 0).
size(): Returns the number of elements in the queue.
front(): Accesses the first element in the queue, which is the next element to be removed.
back(): Accesses the last element in the queue, which is the most recently added element.
push(value): Adds an element to the end of the queue.
pop(): Removes the first element from the queue.
swap(): Swaps the contents of the queue with those of another queue of the same type.
stack():
Adapter class that provides Last-In-First-Out(LIFO) Data Structure.
Implemented using other containers (e.g., vector, deque, list) as the underlying storage.
Member functions:
empty(): Checks if the stack is empty or not.
size(): Returns the number of elements in the stack.
top(): Accesses the top element of the stack, which is the most recently added element.
push(value): Adds an element to the top of the stack.
pop(): Removes the top element from the stack.
swap(): Swaps the content of the stack.
Deque:
Doubly ended Queue.
Similar to vectors but allow efficient insertion and removal at both ends.
Suitable when elements need to be inserted or removed frequently from the front and back.
Member function:
"Same as vector and list"
Priority queue():
Adapter class that provides a priority queue (heap).
Elements are stored in a way that allows the retrieval of the highest-priority element efficiently.
Member functions:
"Same as Stack and Queue"
NOTE:
max Heap - Maximum priority will be given to the highest element. The above code is of max heap.
min Heap - Maximum priority will be given to minimum element. The below code is of min heap.
Map():
Associative Container that stores key-value pairs.
Allows efficient retrieval and modification of values based on keys.
Keys are unique within the map.
Member Function:
find(key): Returns an iterator to the element with the given key, or end() if the key is not found.
count(key): Returns the number of elements with the specified key (1 or 0 since std::map does not allow duplicate keys).
"Rest all member function is same as stack"
NOTE: The above code can also work in an ordered map. All the elements will be in the sorted order based on keys.
Set():
Sorted collection of unique elements.
Elements are sorted in sorted order, and duplicates are automatically removed.
Provides efficient insertion, deletion, and search operations.
Member functions:
"Same as Map member functions"
Understanding these containers and their properties is crucial for efficient and effective C++ programming. Each container type offers distinct advantages and trade-offs, which can significantly impact the performance and maintainability of your code.
Subscribe to my newsletter
Read articles from Rishabh Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
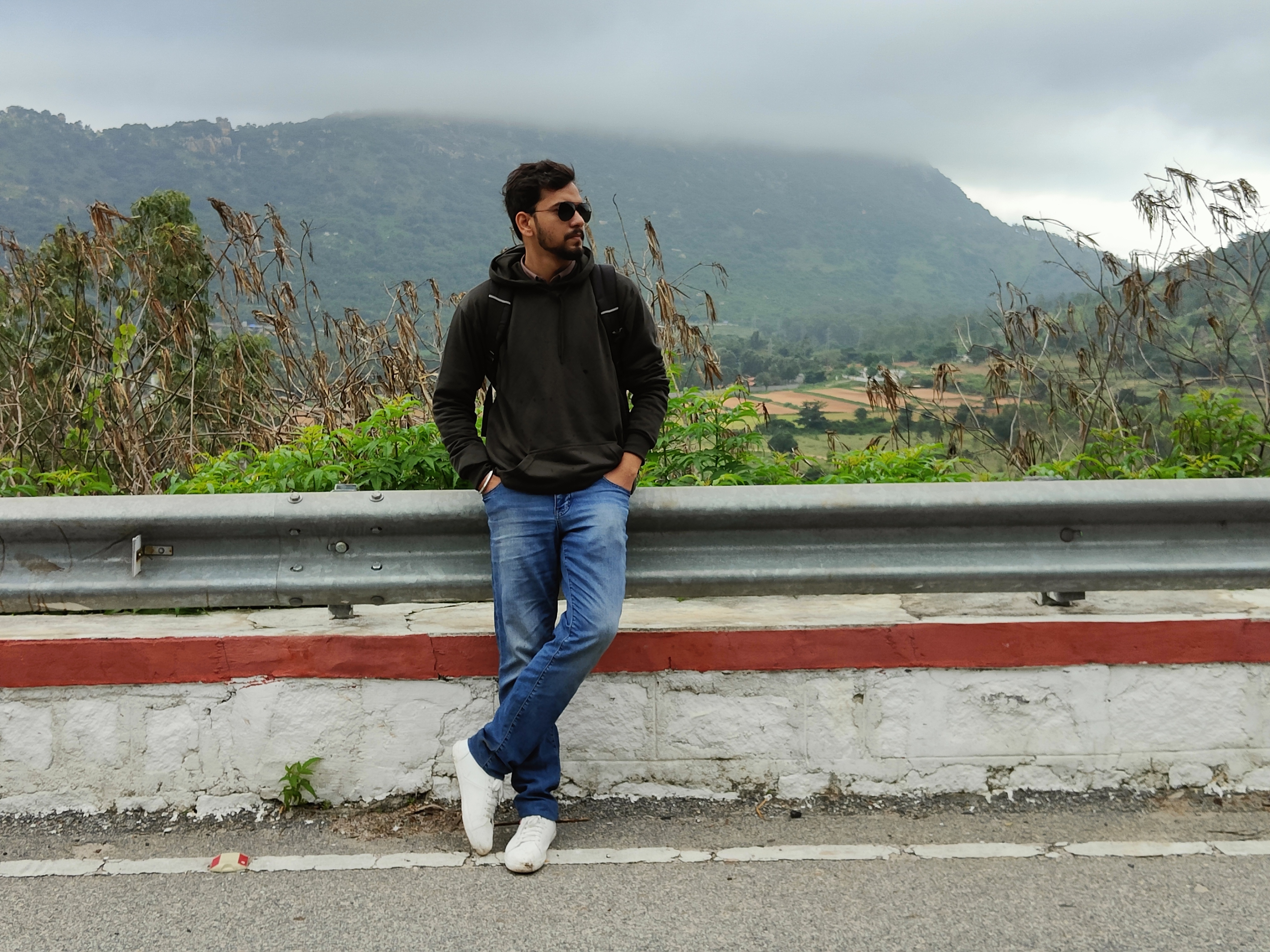