Understanding the Adapter Pattern in Android Development
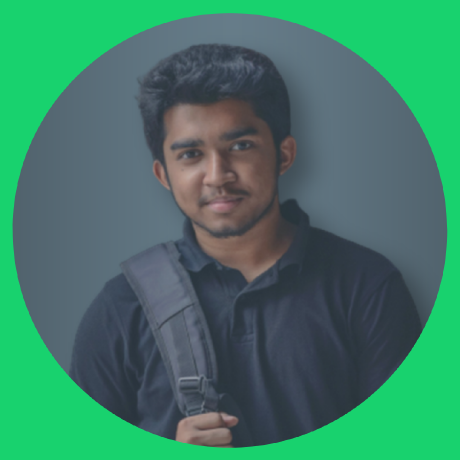
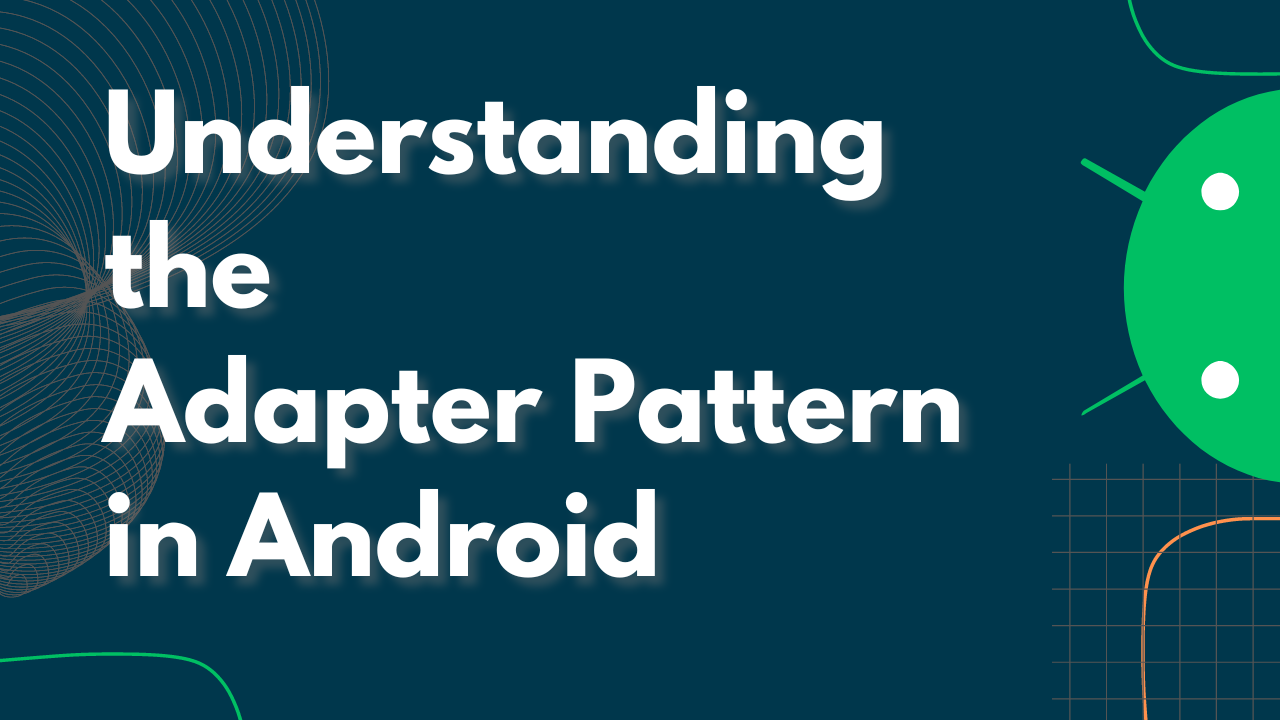
Introduction
In the world of software design, patterns are essential for solving recurring problems and ensuring robust architecture. The Adapter Pattern is a structural design pattern that enables classes with incompatible interfaces to work together. In Android development, the Adapter Pattern is particularly useful for creating flexible and reusable components that can adapt data from different sources to a common interface. In this blog, we'll explore the Adapter Pattern, its benefits, and its implementation in Android using Kotlin.
What is the Adapter Pattern?
The Adapter Pattern allows two incompatible interfaces to work together by wrapping an existing class with a new interface. It acts as a bridge, converting the interface of a class into another interface that a client expects. This pattern is especially useful for integrating new components into existing systems without changing their code.
Key Characteristics:
Interface Conversion: Converts the interface of a class into another interface clients expect.
Reusability: Promotes code reusability by allowing different classes to work together without modifying their code.
Flexibility: Makes it easy to introduce new types of data sources or components.
Benefits of the Adapter Pattern
Decoupling: Helps in decoupling the client from the specifics of the interface it uses, promoting loose coupling.
Code Reusability: Allows the reuse of existing classes and components with new interfaces.
Extensibility: Facilitates the addition of new data sources or components without affecting the existing codebase.
Flexibility: Enables easy adaptation to new interfaces or data formats.
Adapter Pattern in Android
In Android development, the Adapter Pattern is widely used for managing and displaying data in UI components such as ListView
, RecyclerView
, and ViewPager
. Adapters act as a bridge between the UI components and the data source, converting data items into viewable elements.
Common Use Cases:
RecyclerView Adapters: Convert data into
ViewHolder
items for efficient display in aRecyclerView
.ListView Adapters: Adapt data for
ListView
andGridView
components.ViewPager Adapters: Adapt pages for
ViewPager
to display a series of fragments or views.
Implementing the Adapter Pattern in Kotlin
RecyclerView Adapter
RecyclerView
is a powerful UI component for displaying large sets of data efficiently. The RecyclerView.Adapter
class is a key implementation of the Adapter Pattern in Android.
// Data class representing an item
data class User(val name: String, val age: Int)
// ViewHolder class
class UserViewHolder(view: View) : RecyclerView.ViewHolder(view) {
val nameTextView: TextView = view.findViewById(R.id.nameTextView)
val ageTextView: TextView = view.findViewById(R.id.ageTextView)
}
// Adapter class
class UserAdapter(private val userList: List<User>) : RecyclerView.Adapter<UserViewHolder>() {
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): UserViewHolder {
val view = LayoutInflater.from(parent.context)
.inflate(R.layout.item_user, parent, false)
return UserViewHolder(view)
}
override fun onBindViewHolder(holder: UserViewHolder, position: Int) {
val user = userList[position]
holder.nameTextView.text = user.name
holder.ageTextView.text = user.age.toString()
}
override fun getItemCount(): Int {
return userList.size
}
}
Custom ListView Adapter
While RecyclerView
is the preferred choice for most use cases, ListView
can still be useful for simpler scenarios. A custom adapter for ListView
can adapt data to be displayed in a list format.
// Data class representing an item
data class Product(val name: String, val price: Double)
// Custom adapter class
class ProductAdapter(context: Context, private val productList: List<Product>) : ArrayAdapter<Product>(context, 0, productList) {
override fun getView(position: Int, convertView: View?, parent: ViewGroup): View {
val view = convertView ?: LayoutInflater.from(context).inflate(R.layout.item_product, parent, false)
val product = productList[position]
val nameTextView = view.findViewById<TextView>(R.id.nameTextView)
val priceTextView = view.findViewById<TextView>(R.id.priceTextView)
nameTextView.text = product.name
priceTextView.text = String.format("%.2f", product.price)
return view
}
}
In this example, ProductAdapter
adapts a list of Product
objects to be displayed in a ListView
, converting each item into a view that displays the product's name and price.
Best Practices for Using Adapter Pattern
Separation of Concerns: Keep the adapter code focused on adapting data to the view, avoiding complex logic in the adapter.
ViewHolder Pattern: Always use the
ViewHolder
pattern withRecyclerView
to enhance performance and avoid unnecessary view inflation.Data Binding: Consider using data binding to reduce boilerplate code and improve maintainability.
Modular Design: Design adapters to be reusable and modular, allowing easy integration with different data sources or UI components.
Avoid Memory Leaks: Be cautious of context references in adapters to prevent memory leaks.
Conclusion
The Adapter Pattern is a vital design pattern in Android development, enabling the integration of different data sources and components into a cohesive application. By understanding and implementing this pattern using Kotlin, you can create flexible, reusable, and efficient adapters that enhance the user experience in your Android apps. Whether it's a RecyclerView
, ListView
, or ViewPager
, the Adapter Pattern is the key to effective data management and display.
Remember to follow best practices, focus on separation of concerns, and utilize tools like data binding to create robust and maintainable adapters. With these principles in mind, you'll be well-equipped to tackle any data-driven UI challenges in your Android development journey.
Feel free to leave comments or ask questions below. Happy coding!
Enjoyed this guide? Subscribe to our newsletter for more insightful content on Android development! ๐
Subscribe to my newsletter
Read articles from Emran Khandaker Evan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
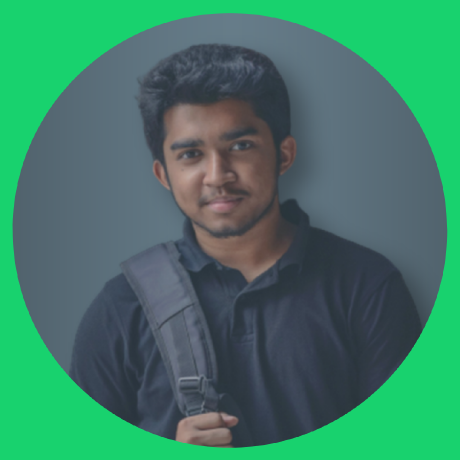
Emran Khandaker Evan
Emran Khandaker Evan
Software Engineer | Content Creator