Ultimate Git Cheat Sheet that Every Software Developer Needs
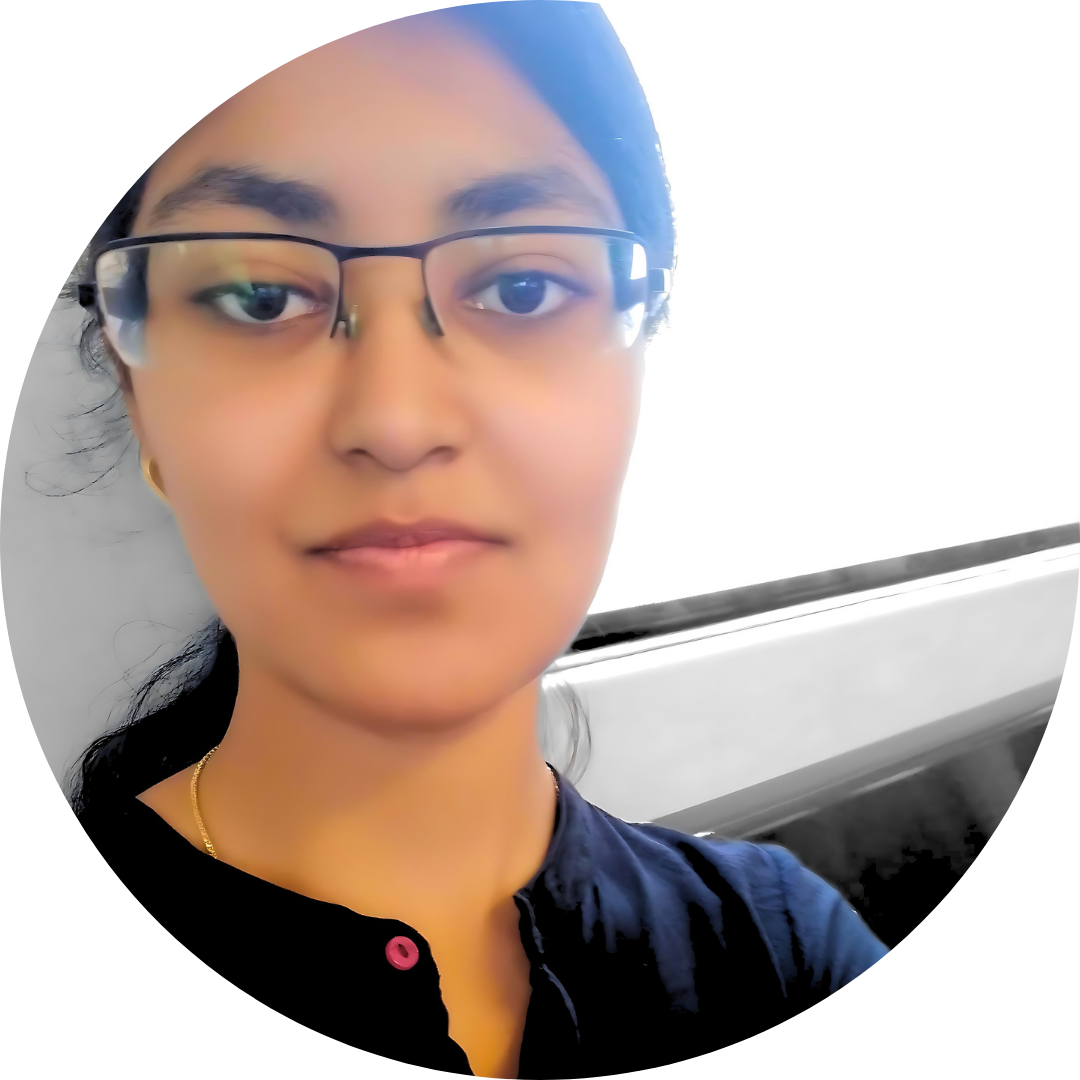

install git on your computer
use git bash as a terminal, it has unix command compatibility
After installing Git, verify the installation
git --version
You should see the installed version of Git after this command.
Configure Your Git Identity
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
This information will be used to know "who did a specific commit" in a collaborative environment
git config --global core.editor "code --wait"
this Specifies the default text editor that Git will use for commit messages
Verify Your Configuration
git config --global --list
/* used to verify if all configurations are saved */
cat .gitconfig
/*use above command in your home directory to view and
set all configurations*/
git initialisation in a repo
mkdir testdir
ls //checks if testdir is created
touch xyz.html //creates a new file -- use in gitbash
git init
/*git init creates a new .git directory which tracks changes in
our source code during development*/
ls -la
/*ls -la shows us all directories including hidden , useful to check
if .git dir is created */
git remote add origin <remote_repository_URL>
/* You typically do this once you start working on a project
so that when you push code it goes into this remote repo.*/
git remote -v //checks if remote is setup or not
.gitignore
this file specify which files and directories that should not be tracked by Git
This is particularly used to exclude
temporary files
build artifacts
files that are not relevant to the source code
files that should not be shared with others .
uses of gitlens extension in vscode
we can see who last modified a line of code and when
Provides a summary of recent changes directly above the affected code block
shows a file history,line history [shows all commits performed on a specific file/ line]
Compare your current branch with other branches
this allows developers to quickly find specific changes related to a bug fix or feature.
raising a pull request
git clone <repository-url>
git checkout master // switching to master branch
git checkout -b "branch_name" // creates new branch
the above code creates a new branch ,before creating new branch checkout to master [create new branch on existing branch only if you have such requirement]
usually branch names will be jira id's in companies
git branch //shows all existing branches
git checkout "branch_name"
/* takes us to an existing branch here do the required changes */
git add file1.html file2.txt /*adds specific files*/
git add . /*adds all files*/
git status /*shows if files are staged or not, or committed */
above code adds files to staging area
git commit -m "commit message"
git push --set-upstream <remote_name> <current_local_branch_name>
/*tells to which branch should git push code on a simple git push
command, this is one time setup for a branch*/
git push /*pushes code to upstream remote branch*/
git push <remote_name> <local_branch_name>:<remote_branch_name>
/*by using above command you can push code from your local branch
to a remote branch with a different name. communicate with your
team if you are pushing changes to a shared remote branch.*/
after pushing go to github and raise a pull request from your remote branch
git pull
its important to do git pull from origin master daily and solve merge conflicts that are due to latest commits on master, so that you can raise a conflict free pull-request, make sure you commit your code before doing a pull
git pull origin master
git pull
/* pulls from our current remote upstream branch,
use this if someone does changes to your branch */
git pull origin <other_remote_branch_name>
/* use this command to pull code from others branch */
git log
git log /*display a list of all commits in the current branch*/
git log --oneline /* Displays each commit on a single line*/
git reset
git reset --hard HEAD~1 /*undoing the most recent commit*/
git push --force /*removing the latest commit which is unwanted from
remote */
git reset --hard <hash>
explanation:
current commit history
A -- B -- C -- D (HEAD)
/*i dont want changes of C,D commits and want to remove them
permanantly */
/*Assume the hash for commit B is abcd1234*/
git reset --hard abcd1234
resulting commit history
A -- B (HEAD)
the above action is generally irreversible. Once you've reset hard and discarded changes, they cannot be recovered
git stash
Git stash is a powerful tool that allows you to temporarily shelve changes you've made in your working branch, allowing you to work on some other branch without committing changes or losing your work.
git stash
git stash pop /*apply the most recent stash to your working branch*/
git stash list /*shows all hashes*/
git stash apply@{2} /*apply a specific stash from the list*/
git stash drop stash@{1} /*removes a specific stash from the list*/
git stash clear /*clears the complete stash*/
git diff
git diff /*shows the difference between
the working branch and the staging area*/
git diff <any-prevcommit-hash> <currentcommit-hash> /*shows the
difference between 2 commits*/
git diff branch1..branch2 /*shows the difference between 2 branches*/
git checkout <commit-hash>
- it is used to inspect the state of your repository at a specific commit. This is useful for debugging or understanding the history of changes.
git checkout <commit-hash>
git checkout <commit-hash> -- myfile.txt
/*shows how a file is in a specific commit, do a checkout to master
once your research is done in a prev commit */
Subscribe to my newsletter
Read articles from prasanna pinnam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
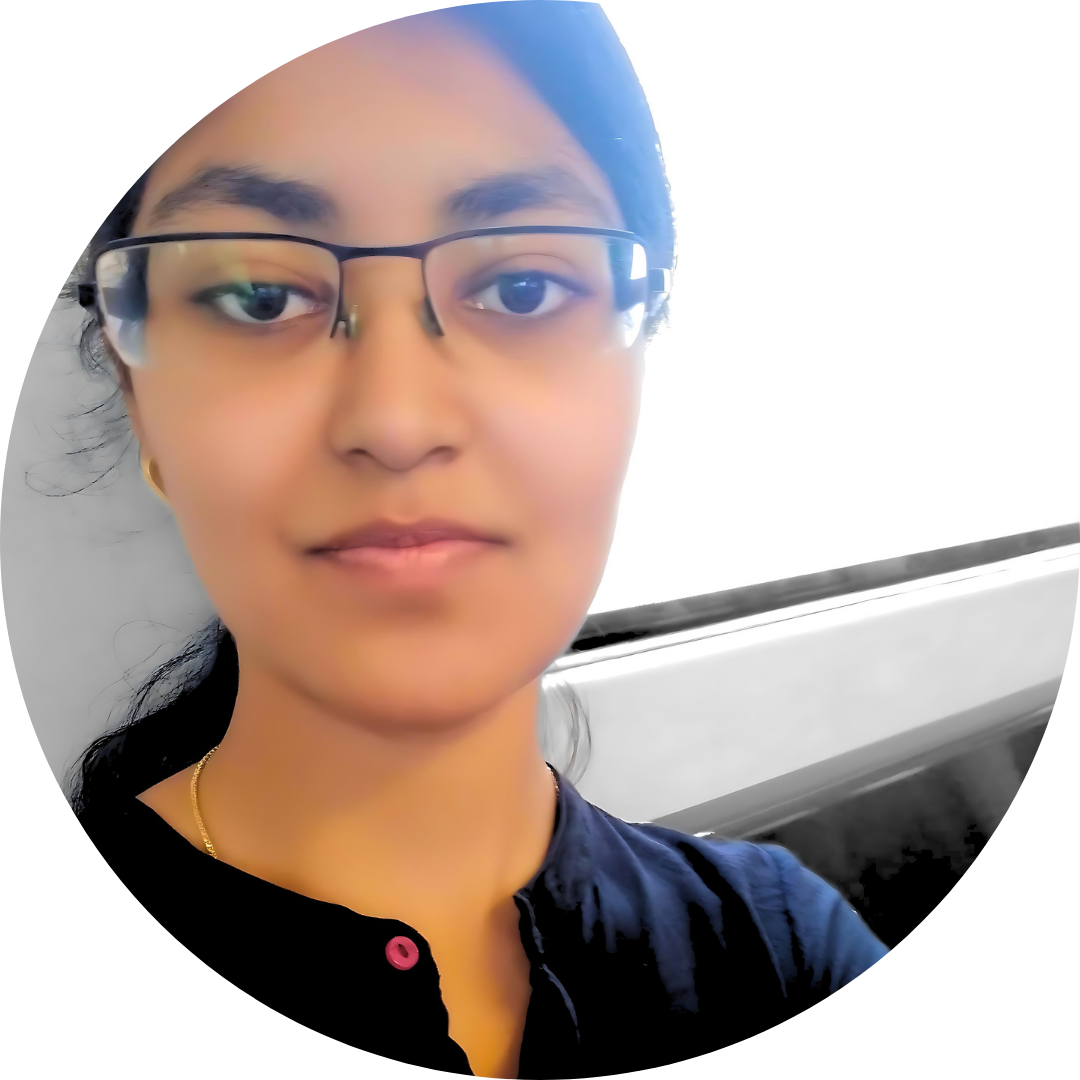