CSS Margin and Padding
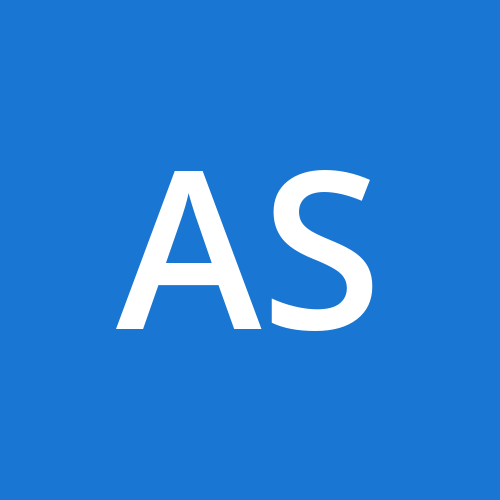
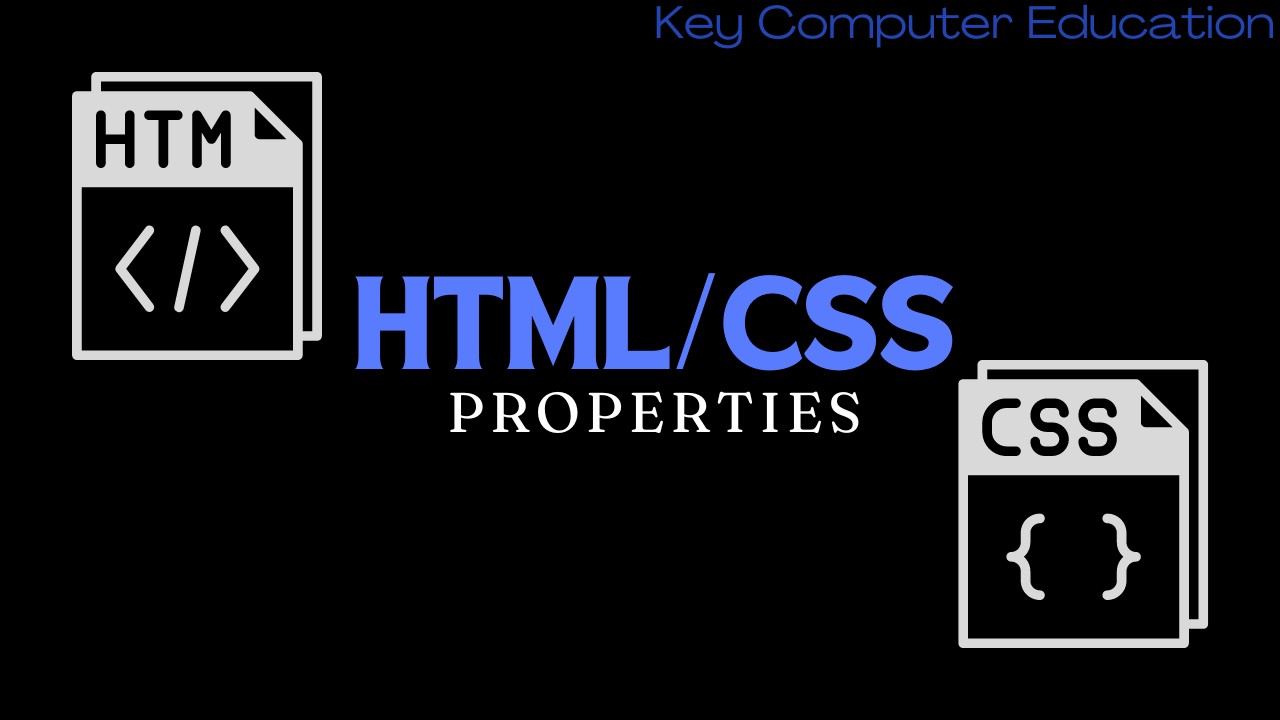
In CSS, margin
and padding
are properties used to create space around elements. However, they serve different purposes and affect elements differently.
Margin
Margin is the space outside the border of an element. It creates space between the element and its neighboring elements.
Properties
margin-top: Sets the top margin of an element.
margin-right: Sets the right margin of an element.
margin-bottom: Sets the bottom margin of an element.
margin-left: Sets the left margin of an element.
margin: A shorthand property to set all four margins at once.
div {
margin-top: 20px;
margin-right: 15px;
margin-bottom: 20px;
margin-left: 15px;
}
Or using shorthand:
div {
margin: 20px 15px 20px 15px; /* top right bottom left */
}
You can also use:
margin: 20px 15px; (top and bottom 20px, right and left 15px)
margin: 20px; (all sides 20px)
Padding
Padding is the space inside the border of an element, between the content and the border.
Properties
padding-top: Sets the top padding of an element.
padding-right: Sets the right padding of an element.
padding-bottom: Sets the bottom padding of an element.
padding-left: Sets the left padding of an element.
padding: A shorthand property to set all four paddings at once.
Example
div {
padding-top: 10px;
padding-right: 5px;
padding-bottom: 10px;
padding-left: 5px;
}
Or using shorthand:
div {
padding: 10px 5px 10px 5px; /* top right bottom left */
}
You can also use:
padding: 10px 5px; (top and bottom 10px, right and left 5px)
padding: 10px; (all sides 10px)
Differences and Use Cases
Margin affects the space outside the element. It can push other elements away from the element itself.
Padding affects the space inside the element. It increases the space between the element’s content and its border.
Visual Representation
Consider a box model for an element:
+-------------------------+
| margin |
| +---------------------+ |
| | border | |
| | +-----------------+ | |
| | | padding | | |
| | | +-------------+ | | |
| | | | content | | | |
| | | +-------------+ | | |
| | +-----------------+ | |
| +---------------------+ |
+-------------------------+
Content: The actual content of the element (text, images, etc.).
Padding: Space between the content and the border.
Border: Surrounds the padding (if defined).
Margin: Space outside the border, separating this element from others.
Practical Example
Suppose you have a <div>
element and you want to add spacing around it:
<!DOCTYPE html>
<html>
<head>
<style>
.container {
width: 300px;
height: 200px;
border: 1px solid black;
margin: 20px; /* Space outside the border */
padding: 10px; /* Space inside the border */
}
</style>
</head>
<body>
<div class="container">
This is a container with margin and padding.
</div></body>
</html>
In this example:
The
margin
will create a 20px space around the.container
element.The
padding
will create a 10px space inside the.container
element, between the border and the content.
Special Notes
Collapsing Margins: In certain cases, vertical margins (top and bottom) of adjacent elements collapse into a single margin, which is equal to the largest of the two margins. Padding does not collapse.
Auto Margin: You can use
margin: auto;
to center an element horizontally within its container.
Examples
1: Margin for Spacing Between Elements
<!DOCTYPE html>
<html>
<head>
<style>
.box {
width: 100px;
height: 100px;
background-color: lightblue;
margin: 20px; /* Adds space outside the box */
}
</style>
</head>
<body>
<div class="box"></div>
<div class="box"></div>
<div class="box"></div>
</body>
</html>
In this example, each .box
element will have a 20px margin on all sides, creating space between the boxes.
2: Padding for Spacing Inside an Element
<!DOCTYPE html>
<html>
<head>
<style>
.box {
width: 100px;
height: 100px;
background-color: lightblue;
padding: 20px; /* Adds space inside the box */
}
</style>
</head>
<body>
<div class="box">Content</div>
</body>
</html>
In this example, the .box
element will have a 20px padding on all sides, creating space between the content ("Content") and the border of the box.
3: Mixed Margins and Paddings
<!DOCTYPE html>
<html>
<head>
<style>
.outer {
width: 200px;
height: 200px;
background-color: lightgreen;
margin: 30px; /* Adds space outside the outer box */
}
.inner {
width: 100px;
height: 100px;
background-color: lightcoral;
padding: 10px; /* Adds space inside the inner box */
}
</style>
</head>
<body>
<div class="outer">
<div class="inner">Content</div>
</div>
</body>
</html>
In this example:
The
.outer
element has a margin of 30px, creating space outside of it.The
.inner
element has a padding of 10px, creating space inside of it between the content and the border.
4: Different Margin Values for Each Side
<!DOCTYPE html>
<html>
<head>
<style>
.box {
width: 100px;
height: 100px;
background-color: lightblue;
margin: 10px 20px 30px 40px; /* top right bottom left */
}
</style>
</head>
<body>
<div class="box"></div>
</body>
</html>
In this example, the .box
element will have different margins on each side:
10px on top
20px on the right
30px on the bottom
40px on the left
5: Different Padding Values for Each Side
<!DOCTYPE html>
<html>
<head>
<style>
.box {
width: 100px;
height: 100px;
background-color: lightblue;
padding: 5px 10px 15px 20px; /* top right bottom left */
}
</style>
</head>
<body>
<div class="box">Content</div>
</body>
</html>
In this example, the .box
element will have different paddings on each side:
5px on top
10px on the right
15px on the bottom
20px on the left
6: Centering an Element with Auto Margins
<!DOCTYPE html>
<html>
<head>
<style>
.container {
width: 400px;
height: 400px;
background-color: lightgray;
position: relative;
}
.center-box {
width: 100px;
height: 100px;
background-color: lightblue;
margin: auto; /* Center the box horizontally and vertically */
}
</style>
</head>
<body>
<div class="container">
<div class="center-box"></div>
</div>
</body>
</html>
In this example, the .center-box
will be centered horizontally and vertically within the .container
element. margin: auto;
ensures that the margins adjust automatically to center the element.
7: Collapsing Margins
<!DOCTYPE html>
<html>
<head>
<style>
.box {
width: 100px;
height: 100px;
background-color: lightblue;
margin-bottom: 20px;
}.container {
margin-top: 20px;
background-color: lightgreen;
padding: 10px;
}
</style>
</head>
<body>
<div class="box"></div>
<div class="container">Container</div>
</body>
</html>
In this example, the bottom margin of .box
and the top margin of .container
will collapse, resulting in only a 20px margin between them instead of 40px (since both are 20px and the larger one takes precedence).
Subscribe to my newsletter
Read articles from Akshima Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
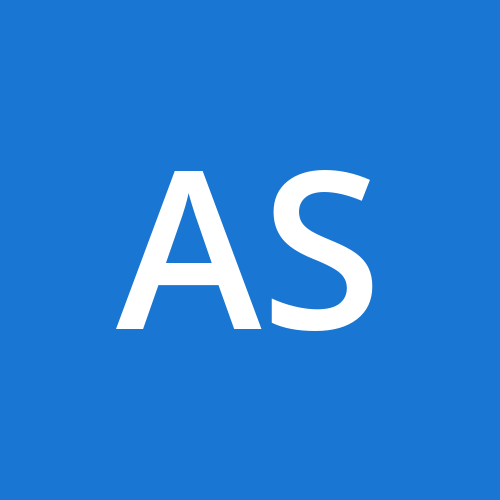