Basic User Authentication System in C
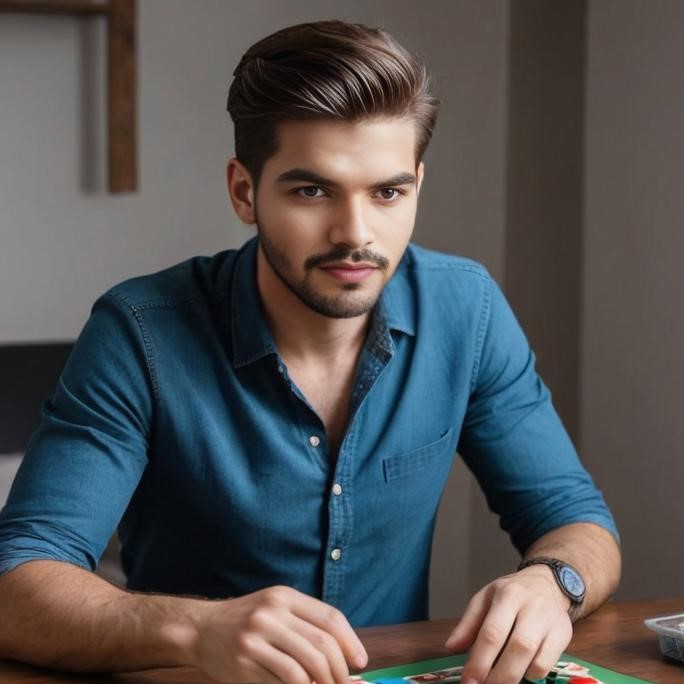
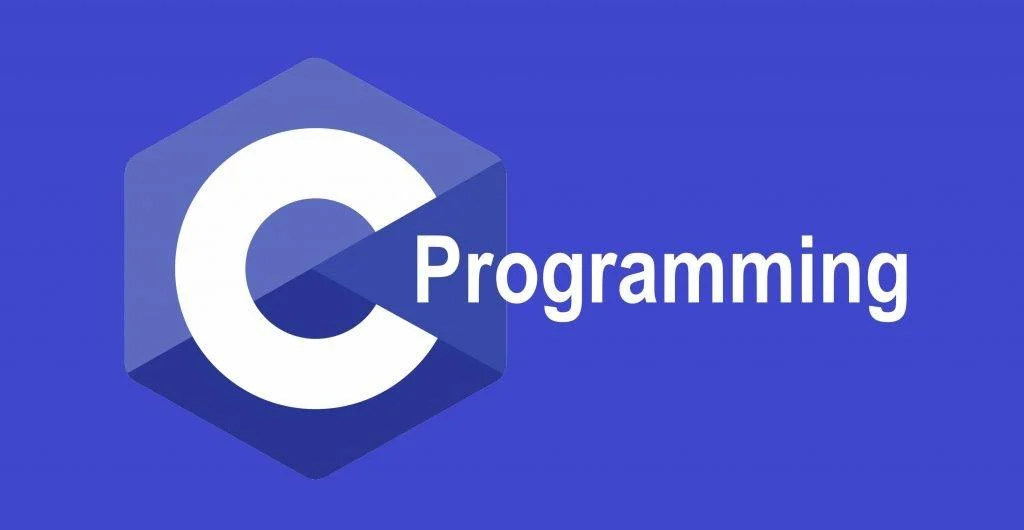
Introduction:
Introduce the concept of user authentication systems.
Mention the importance of user authentication in software applications.
Briefly describe the purpose and functionality of the code.
Code Overview:
Explain the purpose of each header file included (
stdio.h
,stdlib.h
,string.h
).Define the maximum number of users, maximum username length, and maximum password length using
#define
.Define a structure
User
to store user information (username and password).Declare an array of
User
structs to hold user data and a variablenumUsers
to track the number of registered users.
Functions:
Signup Function:
Check if the maximum user limit has been reached.
Prompt the user to enter a username and password.
Store the user's information in the array of users.
Increment
numUsers
and display a success message.
void signup() {
// Code for signup function
}
Login Function
Prompt the user to enter their username and password.
Iterate through the array of users to find a matching username and password.
If found, display a success message; otherwise, indicate a failed login.
int login() {
// Code for login function
}
Title: Building a Basic User Authentication System in C
Subtitle: A Beginner's Guide to Building a Secure User Authentication System in C
Introduction
Introduce the concept of user authentication systems.
Mention the importance of user authentication in software applications.
Briefly describe the purpose and functionality of the code.
Code Overview
Explain the purpose of each header file included (
stdio.h
,stdlib.h
,string.h
).Define the maximum number of users, maximum username length, and maximum password length using
#define
.Define a structure
User
to store user information (username and password).Declare an array of
User
structs to hold user data and a variablenumUsers
to track the number of registered users.
Functions
Signup Function
Check if the maximum user limit has been reached.
Prompt the user to enter a username and password.
Store the user's information in the array of users.
Increment
numUsers
and display a success message.
void signup() {
// Code for signup function
}
Login Function
Prompt the user to enter their username and password.
Iterate through the array of users to find a matching username and password.
If found, display a success message; otherwise, indicate a failed login.
int login() {
// Code for login function
}
Main Function:
Present a menu to the user with options for signup, login, and exit.
Handle user input using a do-while loop and a switch statement.
Call the appropriate functions based on the user's choice.
Explain
int main() {
int choice, accountNumber, toAccount;
float amount;
do {
printf("\nBanking System Menu:\n");
printf("1. Create Account\n");
printf("2. Deposit\n");
printf("3. Withdraw\n");
printf("4. Transfer\n");
printf("5. View Transactions\n");
printf("6. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
}
Conclusion:
Summarize the purpose and functionality of the code.
Highlight the importance of user authentication in software development.
Encourage further exploration and customization of the code for real-world applications.
Final Thoughts:
Share any additional resources or references related to user authentication in C.
Invite feedback and questions from readers.
Does this layout with the code functions presented in a more attractive format work for your blog post?
Subscribe to my newsletter
Read articles from Ashutosh Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
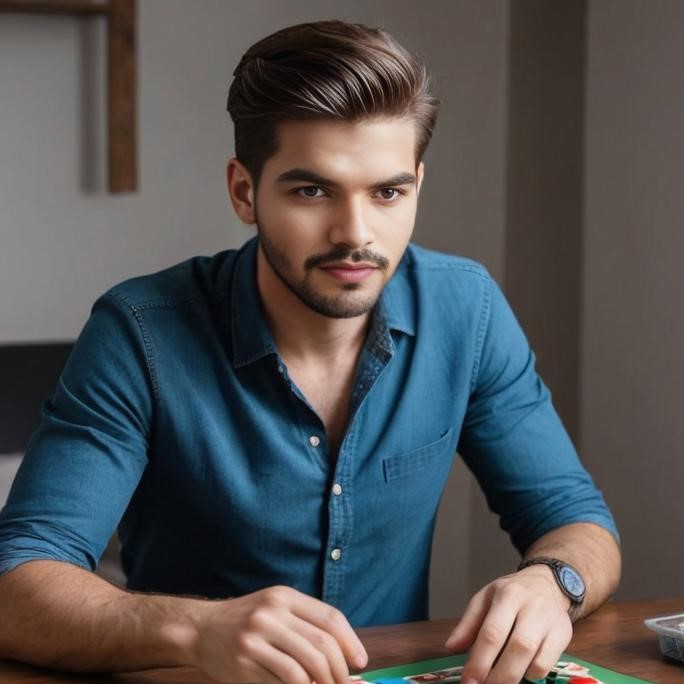
Ashutosh Kumar
Ashutosh Kumar
Creative Full Stack Web Developer & Designer specializing in crafting captivating websites & apps. With a focus on user-centric design, creative development, and effective collaboration, I can elevate brand presence with my design expertise. Till Now I have built 50+ web apps. Most importantly, I'm a reliable designer you can rely on for all your design needs. Currently Building DevDisplay - Paradise For Developers!