QueryQuest: RTK Made Simple πΊοΈ
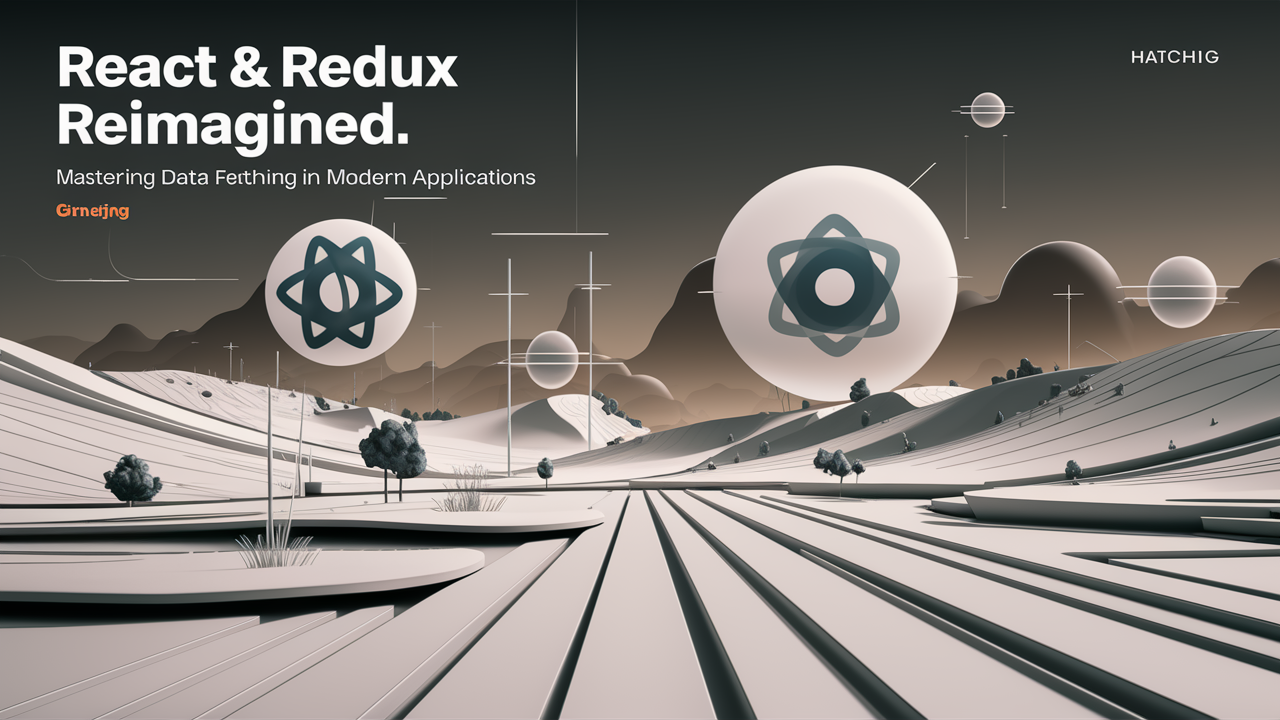
Hey everyone! π In one of my blogs, I delved into Redux Toolkit and explored how it makes your Redux experience a breeze. This time, Iβm excited to share a beginner's guide to a powerful add-on called RTK Query. Ready to dive in? Letβs go! π
RTK Query is an advanced data-fetching tool that can load data on your websites and make them more engaging. It's already included in the @reduxjs/toolkit package. If you're not familiar with @reduxjs/toolkit, be sure to check out my blog Simplifying Redux with Redux Toolkit for React π as it is expected that you know redux toolkit.
In this blog I will be using RTK query in react so we will begin using it in the src
folder where all the application's code is. However, you may wish to use it in similar folder setup of your choice.
Creating an API service
In the code above, we're creating an API slice using the createApi
method from Redux Toolkit. This is primarily boilerplate code and doesnβt require much configuration. In the baseUrl
property, we simply specify the main URL to which all endpoints will be attached. In this case, I'm using the base URL of Unsplash, a website for getting pictures πΈ.
In the endpoints
property, we define all our endpoints. It's recommended to define the entire API configuration in one place for better organization of endpoints and their respective data. This approach makes managing and maintaining your API structure much simpler and more efficient. π οΈ
Here, we're defining the getRandomPicture
property under which we specify the query
property, holding our endpoint. The query
property contains an arrow function that returns the endpoint for its respective purpose.
In the example above, our aim is to fetch a random picture. Hence, we define a /random
endpoint. When combined with the baseUrl
, it forms something like this: https://source.unsplash.com/random
. This endpoint will be used to make requests and fetch an image. πΈ
If you want to pass a specific category to your endpoint dynamically, you can modify the endpoint definition to accept a parameter for the category. Here's how you can do it:
Here, in this code we are passing a specific search term to the getImageBySearchTerm
endpoint which we are then embedding inside the particular search endpoint.
Also, you must have noticed that at the end of this code, I have exported two hooks. These hooks are automatically generated based on their respective endpoints, which will then be used in the functional components for rendering data. They basically have the word use
before the endpoint name (e.g getRandomImage
) followed by the word Query
at the end.
Creating a store πͺ
For using RTK query you would create the store in the same manner as you would create one for non API data. Just to recall, this is how you would do that
To define a reducer for an API slice, such as unsplashApi
, you can use the syntax [unsplashApi.reducerPath]: unsplashApi.reducer
.
In this context:
[unsplashApi.reducerPath]
refers to the key generated by thereducerPath
property of theunsplashApi
service.unsplashApi.reducer
refers to the reducer function associated with theunsplashApi
service.
Essentially, this syntax assigns the reducer function of the unsplashApi
service to its corresponding key, which is defined by its reducerPath
.
Additionally, at the end, we concatenate the default middleware (π οΈ) provided by RTK Query to the middleware of the unsplashApi
service. The default middleware performs tasks such as immutability checks, data fetching, and caching. By combining these with the unsplashApi
middleware, we ensure proper data updates and efficient data fetching for the API's data. This integration helps maintain the integrity and performance of the application's state management.
Integrating Functional Components with the Redux Store π¬
Again, we will follow the standard pattern for providing the Redux store to the rest of your React application component tree:
Using query in components
Now the only thing left to do is to actually use the query in our components for data fetching. Here's how we can do that,
Here data, error and isLoading are some properties that can be destructured from the query hook. It is important to note that all these variables internally work as state variables meaning that when their value changes, the particular component is re-rendered.
Some common properties are:
π¦ data: The fetched data.
β οΈ error: The error object, if any error occurred during the fetch.
β³ isLoading: Boolean indicating if the query is currently loading.
π isFetching: Boolean indicating if a fetch is currently happening.
β isSuccess: Boolean indicating if the query was successful.
β isError: Boolean indicating if the query resulted in an error.
π refetch: A function to manually refetch the data.
isLoading
and isFetching
serve similar purposes but with some differences:
β³ isLoading: This variable only reflects the initial state of the data query. It's
true
when the data is first loaded. It doesn't get updated when the data is refetched due to changes in search terms, component re-rendering, etc.π isFetching: On the other hand,
isFetching
always indicates the state of the query, whether it's the first time the data is fetched or the data is being refetched. It dynamically updates whenever a fetch is happening, providing real-time feedback on the fetch status.
And that's a wrap! I hope this guide has shed light on RTK Query, making data fetching and API calls a breeze in your React applications. Happy coding! π
Subscribe to my newsletter
Read articles from Aneesa Fatima directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by