Feature and Usage of Application.properties
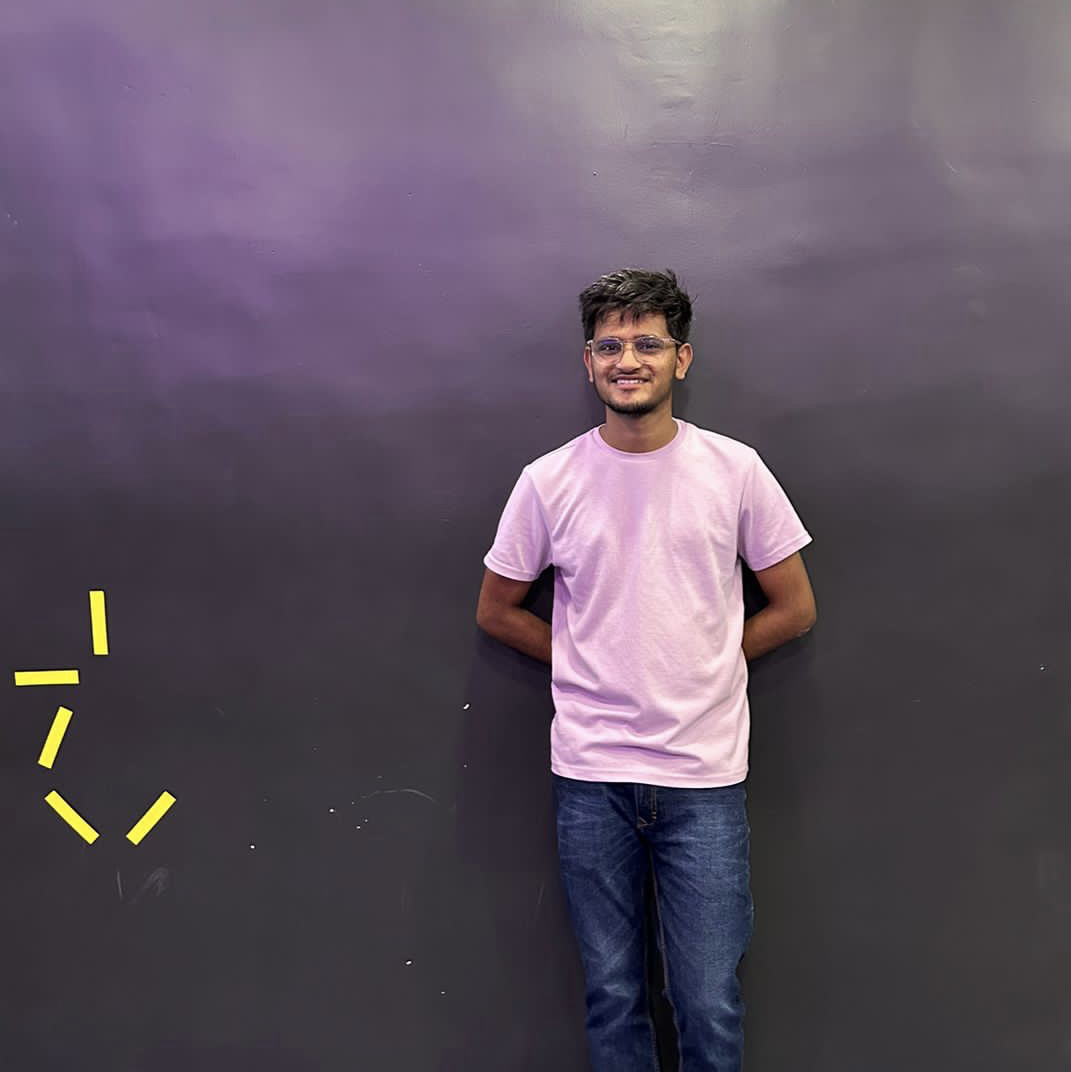
Certainly! Let's consider an example where we want to access custom properties defined in the application.properties
file. We'll demonstrate how to access these properties using the @Value
annotation and @ConfigurationProperties
approach.
Step-by-Step Example
1. Define Properties in application.properties
First, add some custom properties to your application.properties
file:
propertiesCopy code# application.properties
myapp.feature.enabled=true
myapp.default-role=USER
myapp.title=My Application
myapp.version=1.0.0
2. Access Properties Using @Value
You can directly inject these properties into your Spring components using the @Value
annotation.
javaCopy codepackage com.example.demo;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class MyComponent {
@Value("${myapp.feature.enabled}")
private boolean featureEnabled;
@Value("${myapp.default-role}")
private String defaultRole;
@Value("${myapp.title}")
private String title;
@Value("${myapp.version}")
private String version;
public void printProperties() {
System.out.println("Feature Enabled: " + featureEnabled);
System.out.println("Default Role: " + defaultRole);
System.out.println("Title: " + title);
System.out.println("Version: " + version);
}
}
3. Access Properties Using @ConfigurationProperties
For a more structured and type-safe approach, you can use the @ConfigurationProperties
annotation.
javaCopy codepackage com.example.demo;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.stereotype.Component;
@Component
@ConfigurationProperties(prefix = "myapp")
public class MyAppProperties {
private boolean featureEnabled;
private String defaultRole;
private String title;
private String version;
// Getters and setters
public boolean isFeatureEnabled() {
return featureEnabled;
}
public void setFeatureEnabled(boolean featureEnabled) {
this.featureEnabled = featureEnabled;
}
public String getDefaultRole() {
return defaultRole;
}
public void setDefaultRole(String defaultRole) {
this.defaultRole = defaultRole;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
public void printProperties() {
System.out.println("Feature Enabled: " + featureEnabled);
System.out.println("Default Role: " + defaultRole);
System.out.println("Title: " + title);
System.out.println("Version: " + version);
}
}
4. Enable Configuration Properties
Make sure to enable configuration properties in your Spring Boot application class.
SpringbootProjectApplication.java:
javaCopy codepackage com.example.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
@SpringBootApplication
@EnableConfigurationProperties(MyAppProperties.class)
public class SpringbootProjectApplication {
public static void main(String[] args) {
SpringApplication.run(SpringbootProjectApplication.class, args);
}
}
5. Use the Properties in Your Application
Finally, you can use these properties in your application logic. For example, let's use MyComponent
and MyAppProperties
to print the properties.
javaCopy codepackage com.example.demo;
import org.springframework.boot.CommandLineRunner;
import org.springframework.stereotype.Component;
@Component
public class DemoApplication implements CommandLineRunner {
private final MyComponent myComponent;
private final MyAppProperties myAppProperties;
public DemoApplication(MyComponent myComponent, MyAppProperties myAppProperties) {
this.myComponent = myComponent;
this.myAppProperties = myAppProperties;
}
@Override
public void run(String... args) throws Exception {
// Using @Value approach
myComponent.printProperties();
// Using @ConfigurationProperties approach
myAppProperties.printProperties();
}
}
Summary
In this example, we've demonstrated two approaches to accessing properties from the application.properties
file in a Spring Boot application:
Using the
@Value
annotation to inject individual properties.Using
@ConfigurationProperties
to bind a group of properties to a POJO (Plain Old Java Object).
Both approaches have their use cases, and you can choose one based on your specific needs. The @ConfigurationProperties
approach is generally preferred for complex configurations because it provides better type safety and is easier to manage.
Subscribe to my newsletter
Read articles from harsh sehrawat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
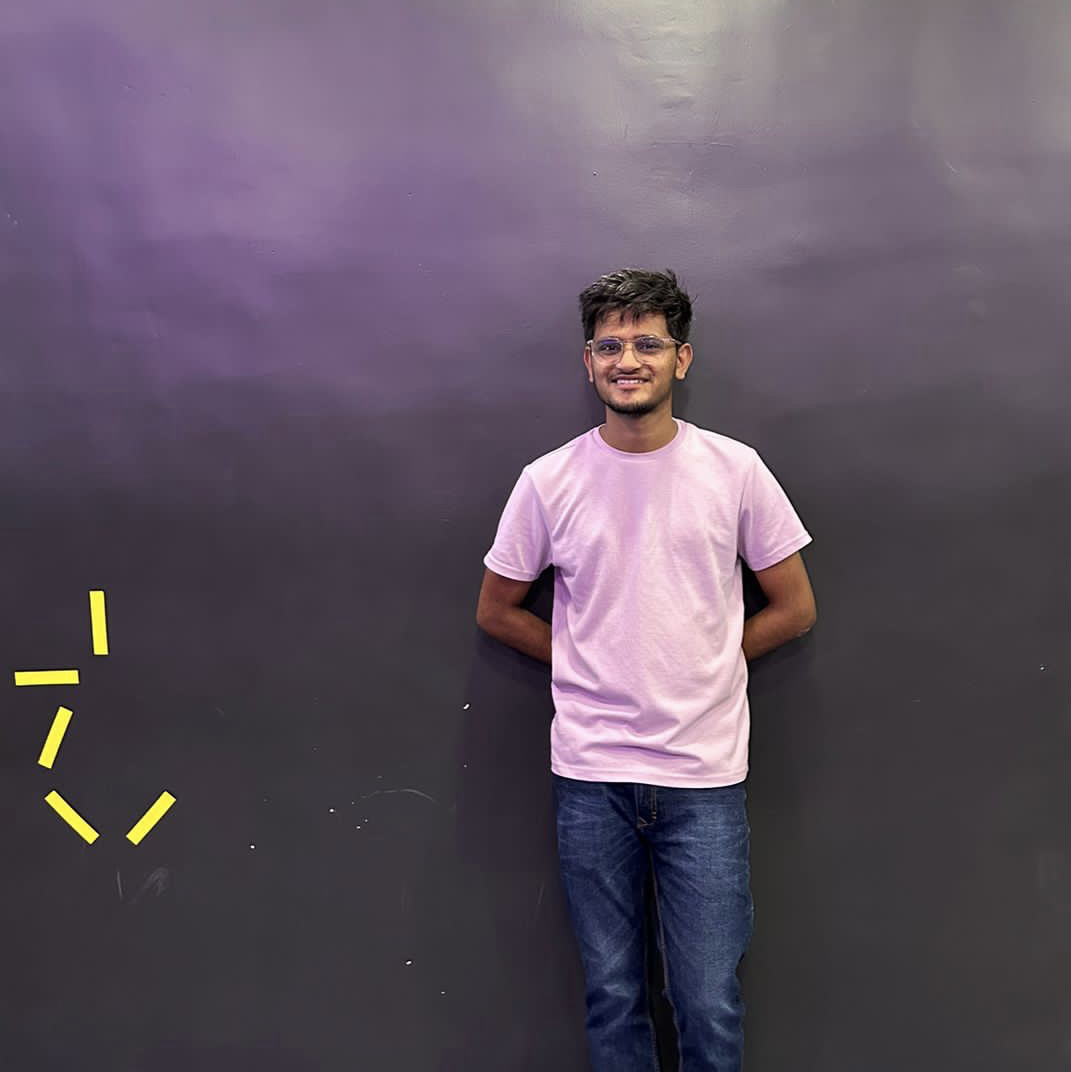
harsh sehrawat
harsh sehrawat
I am a engineering student who's determined to change the time ahead .