JavaScript Debouncing: Boost Performance & UX
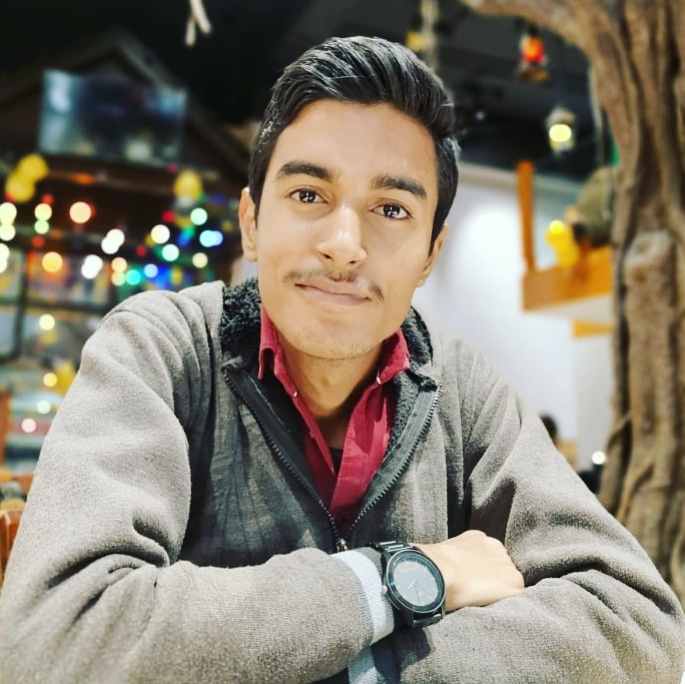
Table of contents
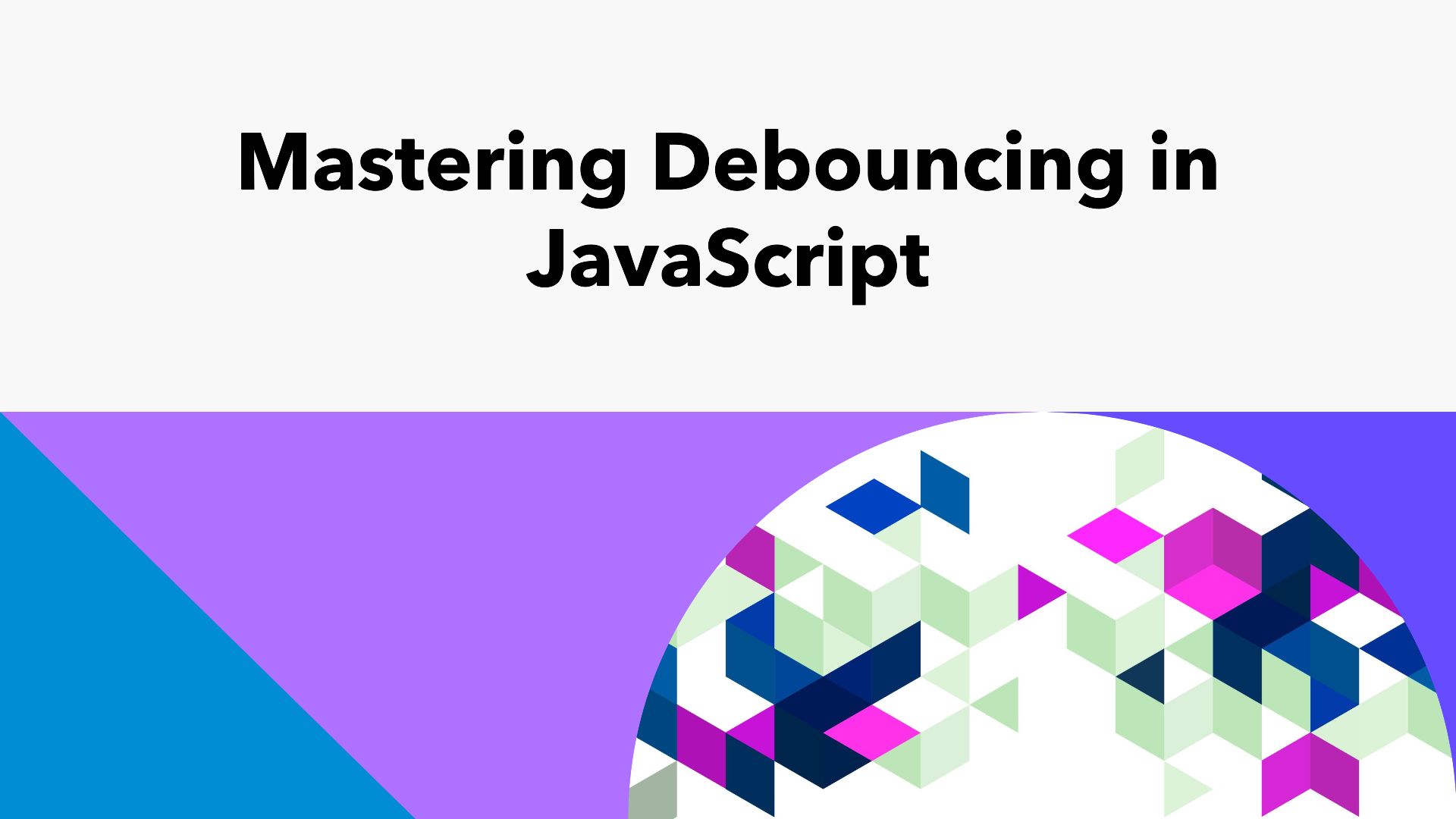
In the fast-paced world of web development, ensuring a smooth and responsive user experience is crucial. JavaScript, being a single-threaded language, can sometimes struggle with handling rapid user interactions or events. This can lead to performance bottlenecks and unintended behavior.
Debouncing is a powerful technique used in JavaScript to optimize performance and improve user experience by limiting the number of times a function is called in response to frequent events. It's particularly useful for scenarios like:
Search suggestions: As the user types in a search bar, you don't want to bombard the server with requests for every keystroke. Debouncing can delay the fetching of suggestions until the user stops typing for a certain period.
Window resize events: When the user resizes the window, you might want to update the layout. However, firing that function on every pixel change can be inefficient. Debouncing ensures the layout update happens only after the resize event stops (i.e., the user finishes resizing).
Scrolling events: Debouncing can be used to optimize infinite scroll functionality, where content is loaded as the user scrolls down. This prevents excessive calls while scrolling smoothly.
How Debouncing Works
Debouncing works by wrapping the function you want to optimize with additional logic. Here's a breakdown of the process:
Function Wrapping: You create a new function that acts as a wrapper around the actual function you want to debounce. This wrapper function controls the execution of the inner function.
Timer and Cancellation: Inside the wrapper function, a timer is introduced using
setTimeout
. This timer delays the execution of the wrapped function for a specific amount of time. Additionally, a variable is used to store the timer reference, allowing cancellation if needed.Event Handling: When the event that triggers the debounced function occurs (e.g., keypress, resize), the wrapper function is called.
Clear Existing Timer (Optional): If there's an existing timer running (meaning the function was called recently), it's cleared using
clearTimeout
. This prevents multiple executions within the delay period.Reset Timer: A new timer is set with the desired delay using
setTimeout
.Function Execution (After Delay): Once the timer expires (after the delay period), the wrapped function is finally executed.
Here's an example of how to implement debounce in JavaScript:
JavaScript
function debounce(func, delay) {
let timeout;
return function (...args) {
clearTimeout(timeout); // Clear existing timer (optional)
timeout = setTimeout(() => {
func.apply(this, args); // Execute function with arguments after delay
}, delay);
};
}
// Example usage
const resizeHandler = debounce(() => {
// Code to handle window resize
console.log("Window resized!");
}, 250); // Debounce with 250ms delay
window.addEventListener("resize", resizeHandler);
Use code with caution.
content_copy
In this example, the debounce
function takes the original function (func
) and the desired delay (delay
) as arguments. It returns a new function that wraps the original function and implements the debouncing logic. The resizeHandler
function demonstrates how to use the debounced function for window resize events, ensuring it's only called at most once every 250 milliseconds.
Benefits of Debouncing
By incorporating debouncing into your JavaScript code, you can achieve several benefits:
Improved Performance: By limiting function calls, debouncing prevents unnecessary computations and DOM manipulations, leading to a smoother user experience.
Resource Optimization: It helps avoid overwhelming the browser with too many function calls, especially for expensive operations. This can be particularly beneficial for mobile devices with limited resources.
Better User Experience: Debouncing prevents glitches or unintended behavior that might occur due to rapid function calls. For instance, in search suggestions, it prevents displaying outdated suggestions while the user is still typing.
Conclusion
Debouncing is a valuable technique for enhancing the performance and responsiveness of your web applications. By understanding how it works and implementing it effectively, you can create a more efficient and user-friendly experience for your visitors. So, the next time you're dealing with frequent events in JavaScript, consider using debouncing to optimize your code and ensure a seamless user experience.
Subscribe to my newsletter
Read articles from Abhishek Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
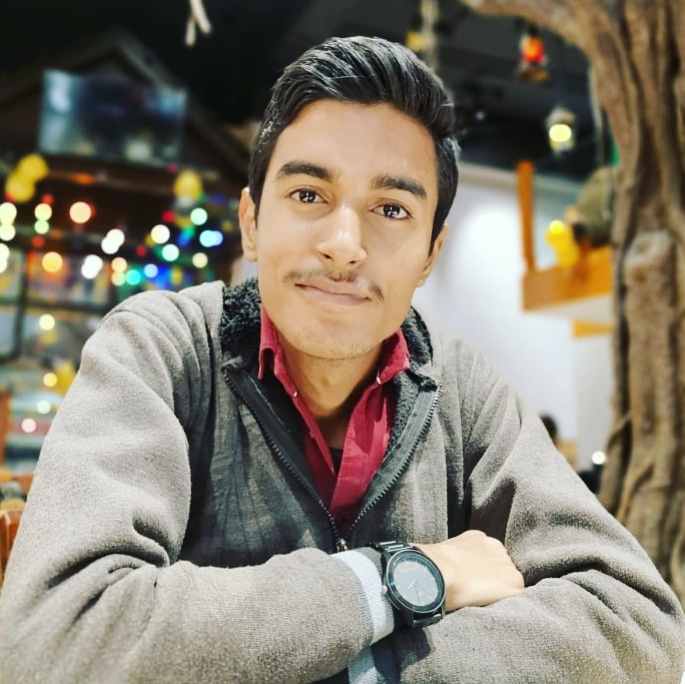
Abhishek Sharma
Abhishek Sharma
Abhishek is a designer, developer, and gaming enthusiast! He love creating things, whether it's building websites, designing interfaces, or conquering virtual worlds. With a passion for technology and its impact on the future, He is curious about how AI can be used to make work better and play even more fun.