How to Make an Animated Loader Using HTML and CSS
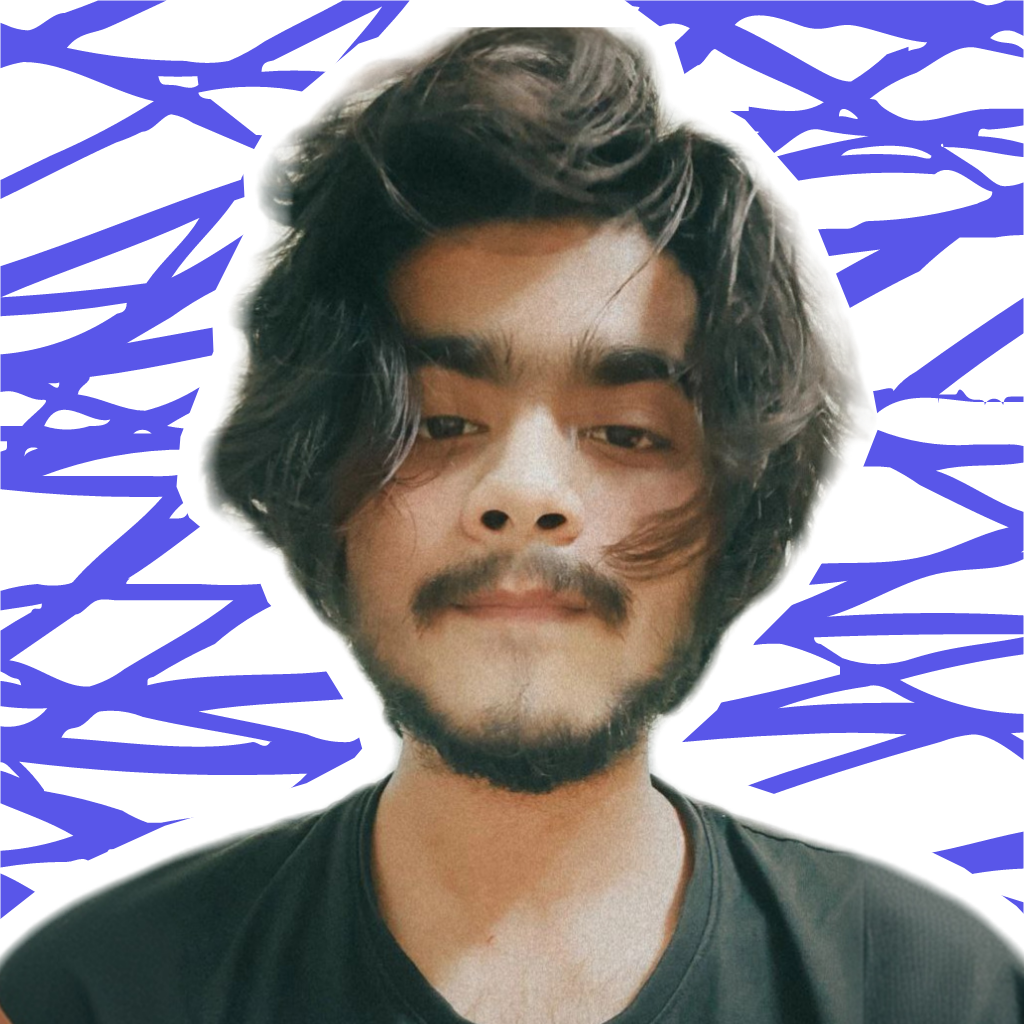
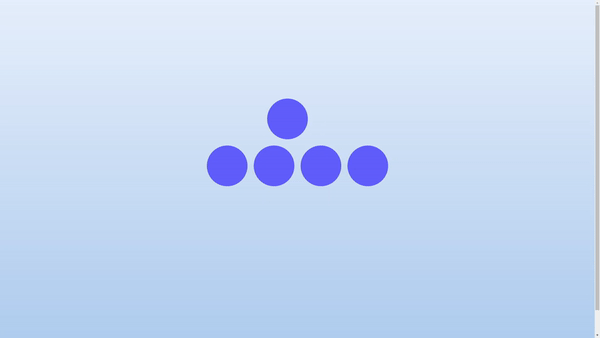
Introduction to Animated Loaders
Animated loaders are essential for improving user experience during webpage load times. They keep users engaged and informed that the site is processing their request. In this article, we'll walk through the process of creating a stylish animated loader using HTML and CSS. This project is part of Day 51 of the #100DaysOfCode Challenge.
Step 1: Setting Up the HTML Structure
To begin, we need to create the basic HTML structure for our animated loader. Here's the HTML code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>Animated Loader</title>
</head>
<body>
<div class="loadingspinner">
<div id="circle1"></div>
<div id="circle2"></div>
<div id="circle3"></div>
<div id="circle4"></div>
<div id="circle5"></div>
</div>
</body>
</html>
In this code, we set up a basic HTML document with a title and a link to an external CSS file. The <div>
elements inside the loadingspinner
container will serve as our animated circles.
Step 2: Styling the Loader with CSS
Next, we'll add the CSS to style the loader. Here's the CSS code:
body {
display: flex;
align-items: center;
justify-content: center;
min-height: 100vh;
background-image: linear-gradient(to top, #accbee 0%, #e7f0fd 100%);
}
.loadingspinner {
--circle: 26px;
--offset: 30px;
--duration: 2.4s;
--delay: 0.2s;
--timing-function: ease-in-out;
--in-duration: 0.4s;
--in-delay: 0.1s;
--in-timing-function: ease-out;
width: calc(3 * var(--offset) + var(--circle));
height: calc(2 * var(--offset) + var(--circle));
padding: 0px;
margin: 10px auto 30px auto;
position: relative;
}
.loadingspinner div {
display: inline-block;
background: #615EFC;
border: none;
border-radius: 50%;
width: var(--circle);
height: var(--circle);
position: absolute;
padding: 0px;
margin: 0px;
}
.loadingspinner #circle1 {
left: calc(0 * var(--offset));
top: calc(0 * var(--offset));
animation: circle1 var(--duration) var(--delay) var(--timing-function) infinite,
circlefadein var(--in-duration) calc(1 * var(--in-delay)) var(--in-timing-function) both;
}
.loadingspinner #circle2 {
left: calc(0 * var(--offset));
top: calc(1 * var(--offset));
animation: circle2 var(--duration) var(--delay) var(--timing-function) infinite,
circlefadein var(--in-duration) calc(1 * var(--in-delay)) var(--in-timing-function) both;
}
.loadingspinner #circle3 {
left: calc(1 * var(--offset));
top: calc(1 * var(--offset));
animation: circle3 var(--duration) var(--delay) var(--timing-function) infinite,
circlefadein var(--in-duration) calc(2 * var(--in-delay)) var(--in-timing-function) both;
}
.loadingspinner #circle4 {
left: calc(2 * var(--offset));
top: calc(1 * var(--offset));
animation: circle4 var(--duration) var(--delay) var(--timing-function) infinite,
circlefadein var(--in-duration) calc(3 * var(--in-delay)) var(--in-timing-function) both;
}
.loadingspinner #circle5 {
left: calc(3 * var(--offset));
top: calc(1 * var(--offset));
animation: circle5 var(--duration) var(--delay) var(--timing-function) infinite,
circlefadein var(--in-duration) calc(4 * var(--in-delay)) var(--in-timing-function) both;
}
This CSS styles the body to center the content both vertically and horizontally. It also sets a pleasant background gradient. The loadingspinner
class contains variables to control the size, spacing, and animation duration of the circles.
Step 3: Adding Keyframe Animations
Now, we need to define the animations for the circles. Here's the CSS code for the keyframes:
@keyframes circle1 {
0% {
left: calc(0 * var(--offset));
top: calc(0 * var(--offset));
}
8.333% {
left: calc(0 * var(--offset));
top: calc(1 * var(--offset));
}
100% {
left: calc(0 * var(--offset));
top: calc(1 * var(--offset));
}
}
@keyframes circle2 {
0% {
left: calc(0 * var(--offset));
top: calc(1 * var(--offset));
}
8.333% {
left: calc(0 * var(--offset));
top: calc(2 * var(--offset));
}
16.67% {
left: calc(1 * var(--offset));
top: calc(2 * var(--offset));
}
25.00% {
left: calc(1 * var(--offset));
top: calc(1 * var(--offset));
}
83.33% {
left: calc(1 * var(--offset));
top: calc(1 * var(--offset));
}
91.67% {
left: calc(1 * var(--offset));
top: calc(0 * var(--offset));
}
100% {
left: calc(0 * var(--offset));
top: calc(0 * var(--offset));
}
}
@keyframes circle3 {
0%, 100% {
left: calc(1 * var(--offset));
top: calc(1 * var(--offset));
}
16.67% {
left: calc(1 * var(--offset));
top: calc(1 * var(--offset));
}
25.00% {
left: calc(1 * var(--offset));
top: calc(0 * var(--offset));
}
33.33% {
left: calc(2 * var(--offset));
top: calc(0 * var(--offset));
}
41.67% {
left: calc(2 * var(--offset));
top: calc(1 * var(--offset));
}
66.67% {
left: calc(2 * var(--offset));
top: calc(1 * var(--offset));
}
75.00% {
left: calc(2 * var(--offset));
top: calc(2 * var(--offset));
}
83.33% {
left: calc(1 * var(--offset));
top: calc(2 * var(--offset));
}
91.67% {
left: calc(1 * var(--offset));
top: calc(1 * var(--offset));
}
}
@keyframes circle4 {
0% {
left: calc(2 * var(--offset));
top: calc(1 * var(--offset));
}
33.33% {
left: calc(2 * var(--offset));
top: calc(1 * var(--offset));
}
41.67% {
left: calc(2 * var(--offset));
top: calc(2 * var(--offset));
}
50.00% {
left: calc(3 * var(--offset));
top: calc(2 * var(--offset));
}
58.33% {
left: calc(3 * var(--offset));
top: calc(1 * var(--offset));
}
100% {
left: calc(3 * var(--offset));
top: calc(1 * var(--offset));
}
}
@keyframes circle5 {
0% {
left: calc(3 * var(--offset));
top: calc(1 * var(--offset));
}
50.00% {
left: calc(3 * var(--offset));
top: calc(1 * var(--offset));
}
58.33% {
left: calc(3 * var(--offset));
top: calc(0 * var(--offset));
}
66.67% {
left: calc
(2 * var(--offset));
top: calc(0 * var(--offset));
}
75.00% {
left: calc(2 * var(--offset));
top: calc(1 * var(--offset));
}
100% {
left: calc(2 * var(--offset));
top: calc(1 * var(--offset));
}
}
@keyframes circlefadein {
0% {
transform: scale(0.75);
opacity: 0.0;
}
100% {
transform: scale(1.0);
opacity: 1.0;
}
}
These keyframes define the movement and fade-in effects for each circle. The circle1
through circle5
animations move the circles in a specific path, while circlefadein
handles the initial fade-in effect.
Conclusion
By following these steps, you've created an animated loader using HTML and CSS. This loader enhances user experience by providing visual feedback during page load times. For the full source code, download here. If you have any questions or need further assistance, feel free to contact me.
Creating engaging and functional animations can significantly improve the user interface of your website. Keep experimenting and adding unique touches to your projects!
Subscribe to my newsletter
Read articles from Aarzoo Islam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
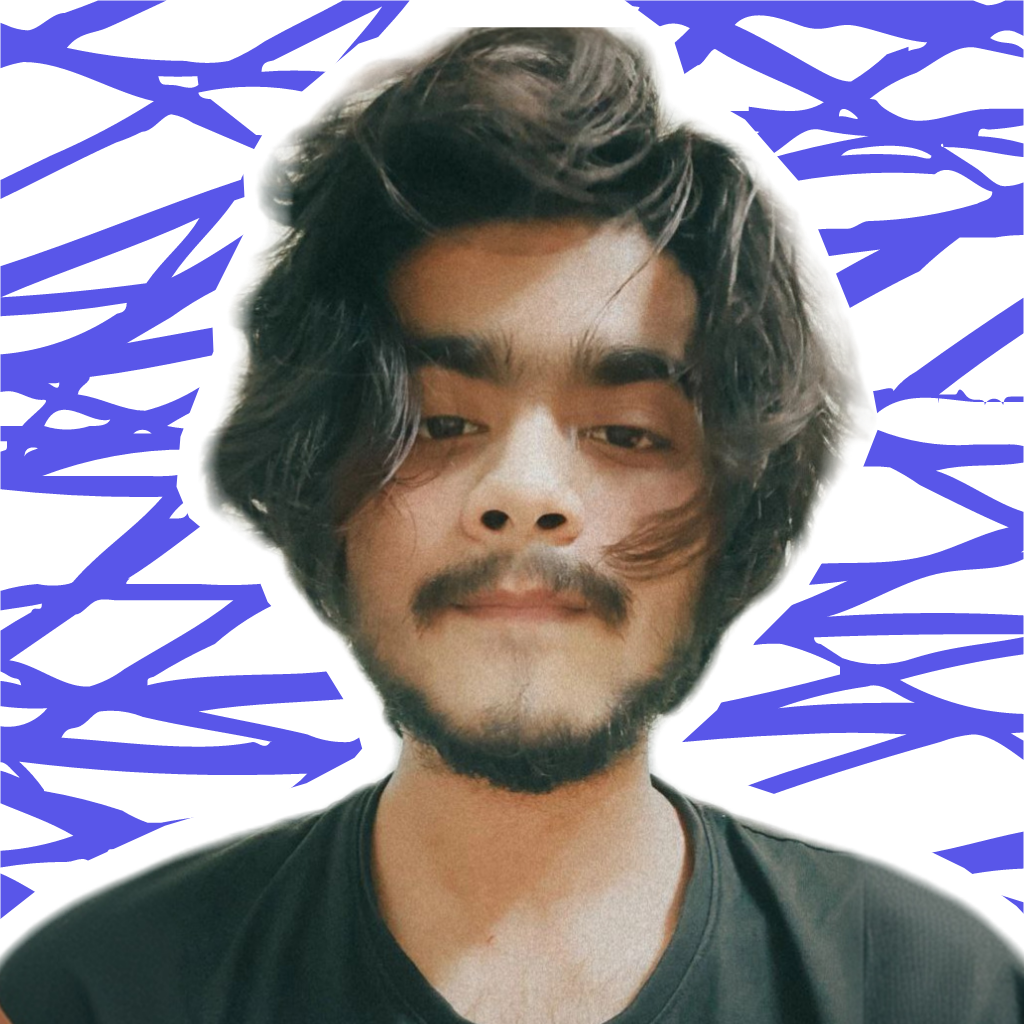
Aarzoo Islam
Aarzoo Islam
Founder of AroNus ๐ | Full-stack web developer ๐จ๐ปโ๐ป | Sharing web development tips and showcasing projects ๐ | Transforming ideas into digital realities ๐