Jumping Ball Progress
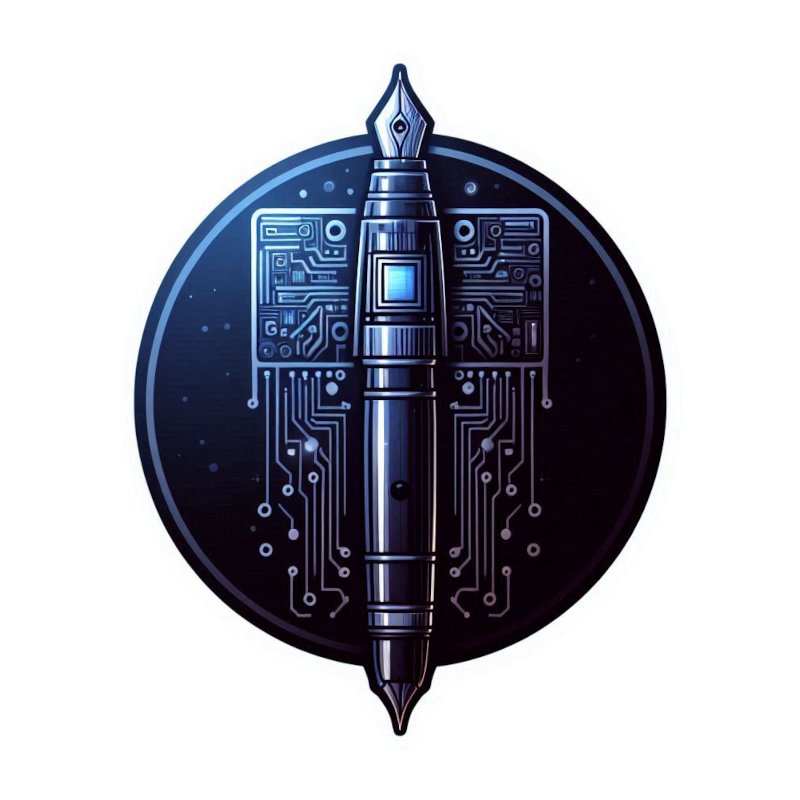
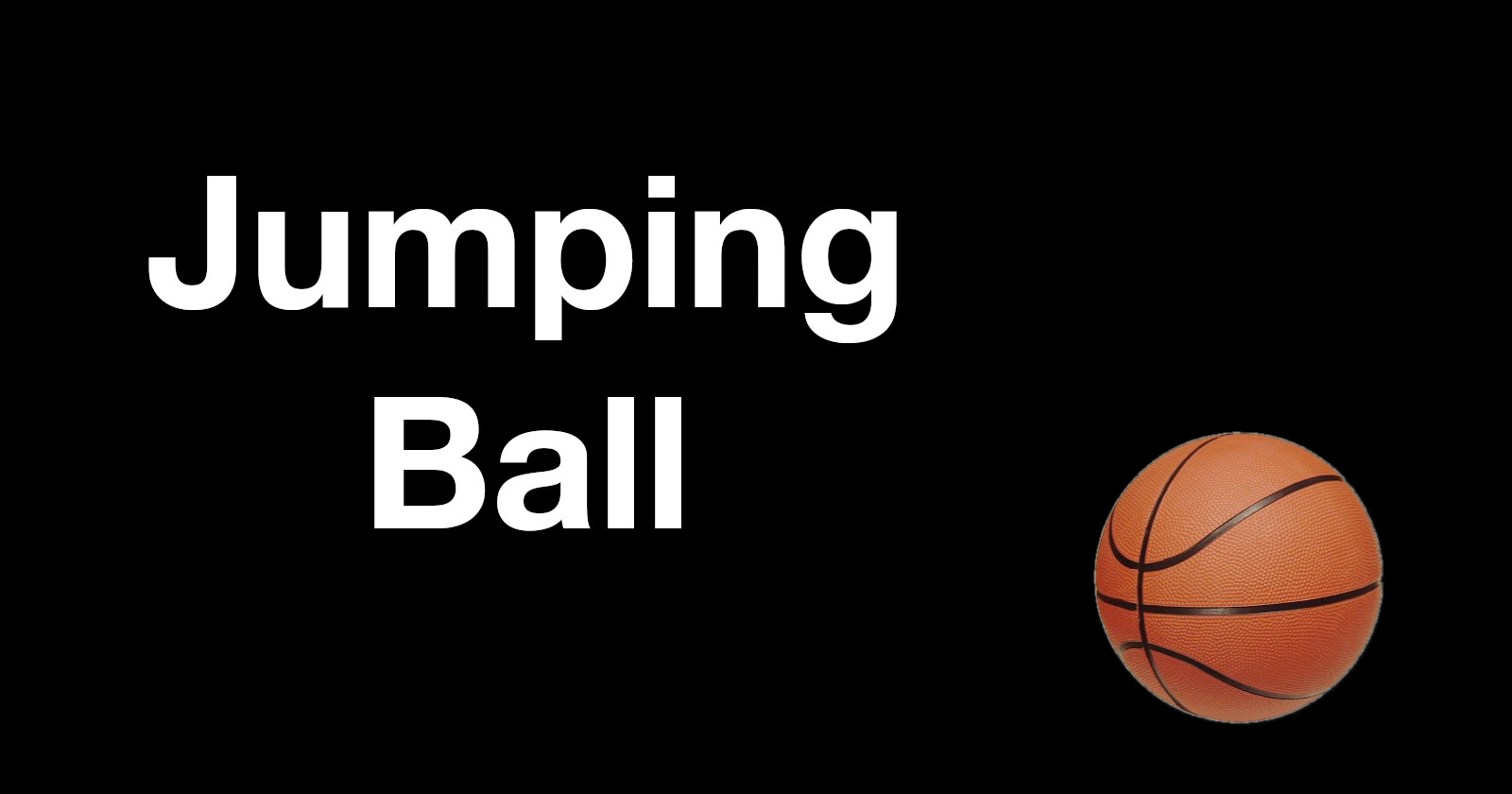
I finally figured out how to fix the font path crash. Using const
wasn't enough, as many crashes suddenly appeared. Using a char
array fixed the font path problem and also resolved a random segmentation fault I was experiencing.
Also, I discovered a really cool tool for dmg file creation. I updated the CMakeLists.txt file to move the dynamic libraries to the .app bundle and added an icon too!
The problem with char*
is that it points to the address of the first character and then the rest of the string can by found by going through each address after the first one until it meets a termination character. What does this might mean?
Since, the char*
variable is not an array and points to the first character, the rest of the string can be mutated without a reason. I converted the const char* location
to char location[1024]
array. I created a temporary char*
to assign the path that the FindResource
returns and then run it through a for loop to copy it.
// Resource struct
typedef struct Resource
{
char location[1024];
}Resource;
// Init app function
char *temp = "/Contents/Resources/fonts/Montserrat-VariableFont_wght.ttf";
for (int i = 0; i < strlen(temp); i++)
{
fontResource.location[i] = temp[i];
}
// The for loop doesn't add the termination character
// I had to add it "manually"
fontResource.location[strlen(temp)] = '\0';
To move the dylib files of each library inside the .app Frameworks folder, I used the same technique that I used for the assets that are being copied inside the .app bundle. The application does not search for the libraries inside the .app bundle. I need to find a way to fix this.
file(GLOB_RECURSE SDL_LIB_FILES "./modules/SDL/build/*.dylib")
file(GLOB_RECURSE SDL_TTF_LIB_FILES "./modules/SDL_ttf/build/*.dylib")
file(GLOB_RECURSE LIBLOCATE_LIB_FILES "./modules/cpplocate/build/*.dylib")
add_executable( ${PROJECT_NAME} MACOSX_BUNDLE ./src/main.c
./src/app.h ./src/app.c ./src/resourcelocator.h
./src/resourcelocator.c ./src/text.h ./src/text.c
./src/results.h ./src/results.c ./src/textbox.h
./src/textbox.c ./src/ball.h ./src/ball.c
./src/button.h ./src/button.c ${APP_FONT} ${MACOS_ICON}
${SDL_LIB_FILES} ${SDL_TTF_LIB_FILES} ${LIBLOCATE_LIB_FILES})
# include SDL library to the bundle
foreach(RESOURCE ${SDL_LIB_FILES})
file(RELATIVE_PATH RES_PATH_NAME "${CMAKE_CURRENT_SOURCE_DIR}" ${RESOURCE})
get_filename_component(NEW_FILE_PATH_NAME ${RES_PATH_NAME} DIRECTORY)
set_property(SOURCE ${RESOURCE} PROPERTY MACOSX_PACKAGE_LOCATION "Frameworks/${NEW_FILE_PATH_NAME}")
endforeach()
# include SDL ttf library to the bundle
foreach(RESOURCE ${SDL_TTF_LIB_FILES})
file(RELATIVE_PATH RES_PATH_NAME "${CMAKE_CURRENT_SOURCE_DIR}" ${RESOURCE})
get_filename_component(NEW_FILE_PATH_NAME ${RES_PATH_NAME} DIRECTORY)
set_property(SOURCE ${RESOURCE} PROPERTY MACOSX_PACKAGE_LOCATION "Frameworks/${NEW_FILE_PATH_NAME}")
endforeach()
# include cpplocate library to the bundle
foreach(RESOURCE ${LIBLOCATE_LIB_FILES})
file(RELATIVE_PATH RES_PATH_NAME "${CMAKE_CURRENT_SOURCE_DIR}" ${RESOURCE})
get_filename_component(NEW_FILE_PATH_NAME ${RES_PATH_NAME} DIRECTORY)
set_property(SOURCE ${RESOURCE} PROPERTY MACOSX_PACKAGE_LOCATION "Frameworks/${NEW_FILE_PATH_NAME}")
endforeach()
For the icon, I simply copied the resource to the Resources folder and added the icon as a property using CMakeLists.txt
file(GLOB MACOS_ICON "./packaging assets/img/Jumping_Ball_Logo.icns")
add_executable( ${PROJECT_NAME} MACOSX_BUNDLE ./src/main.c
./src/app.h ./src/app.c ./src/resourcelocator.h
./src/resourcelocator.c ./src/text.h ./src/text.c
./src/results.h ./src/results.c ./src/textbox.h
./src/textbox.c ./src/ball.h ./src/ball.c
./src/button.h ./src/button.c ${APP_FONT} ${MACOS_ICON}
${SDL_LIB_FILES} ${SDL_TTF_LIB_FILES} ${LIBLOCATE_LIB_FILES})
# Set macos Icon
file(RELATIVE_PATH RES_PATH_NAME "${CMAKE_CURRENT_SOURCE_DIR}" ${MACOS_ICON})
get_filename_component(NEW_FILE_PATH_NAME ${RES_PATH_NAME} DIRECTORY)
set_property(SOURCE ${MACOS_ICON} PROPERTY MACOSX_PACKAGE_LOCATION "Resources/${NEW_FILE_PATH_NAME}")
set_target_properties(${PROJECT_NAME} PROPERTIES MACOSX_BUNDLE_ICON_FILE "Contents/Resources/packaging assets/img/Jumping_Ball_Logo.icns")
For the dmg creation, I used a really cool that I found on GitHub. Special thanks to LinusU for the awesome tool!
Demonstration
Subscribe to my newsletter
Read articles from Chris Douris directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
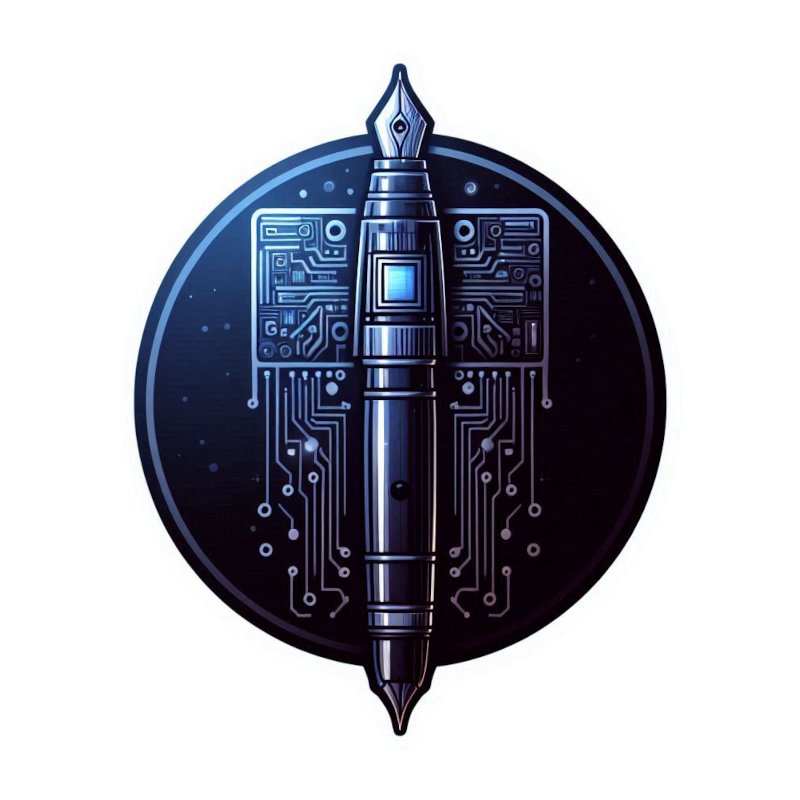
Chris Douris
Chris Douris
AKA Chris, is a software developer from Athens, Greece. He started programming with basic when he was very young. He lost interest in programming during school years but after an unsuccessful career in audio, he decided focus on what he really loves which is technology. He loves working with older languages like C and wants to start programming electronics and microcontrollers because he wants to get into embedded systems programming.