Array Questions Part — 2(CPP)
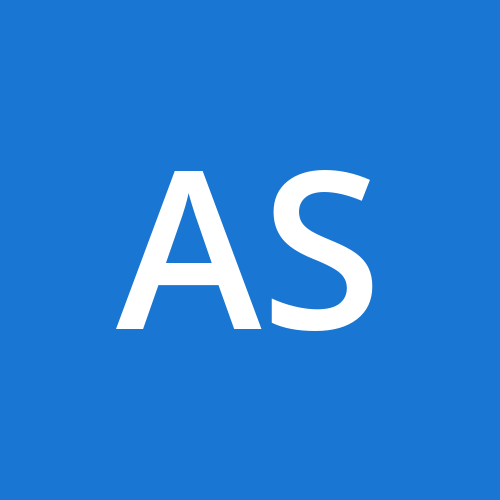
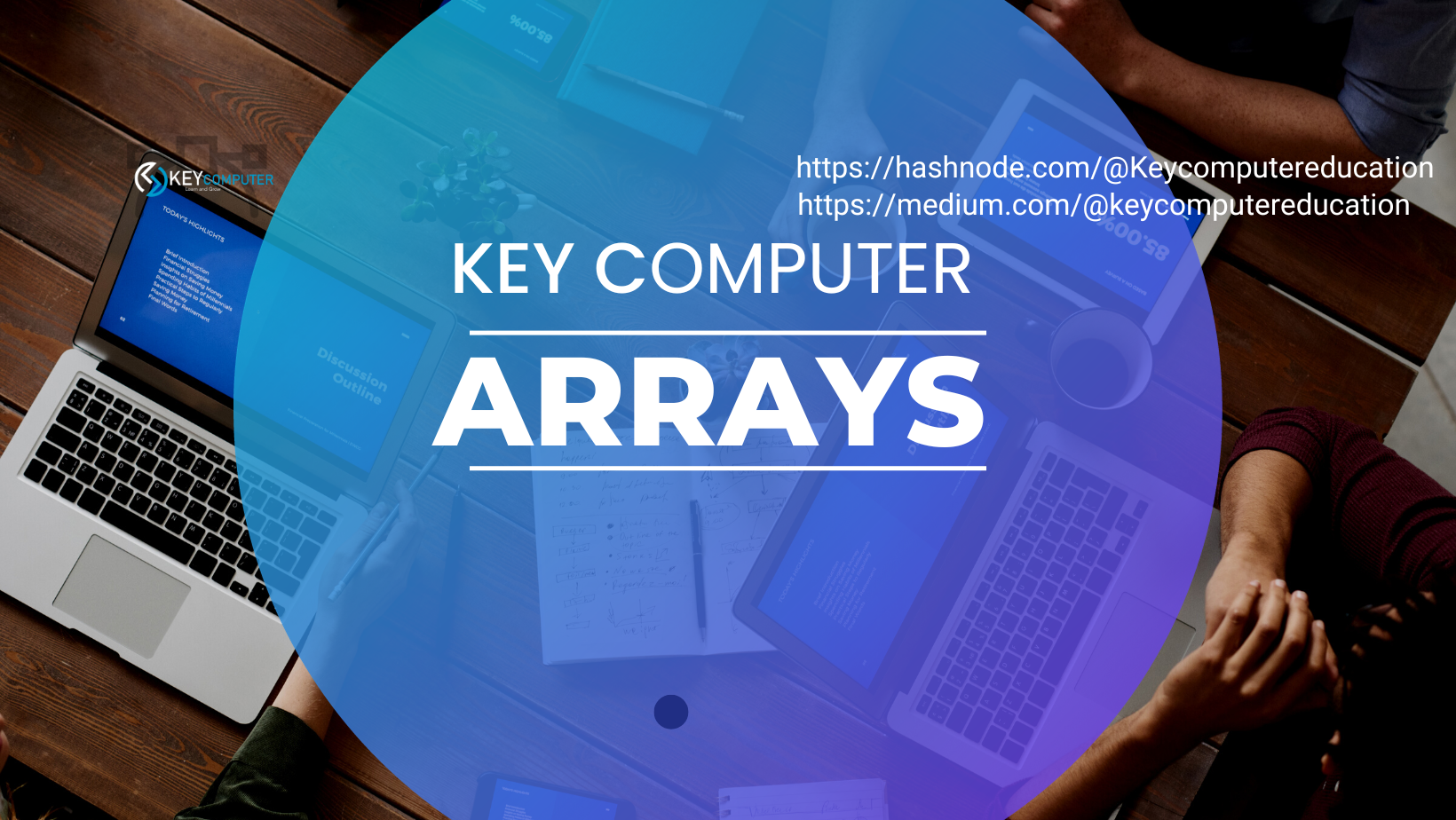
At least two greater elements
At least two greater elements | Practice | GeeksforGeeks
Given an array of N distinct elements, the task is to find all elements in array except two greatest elements in sorted…
Given an array of N distinct elements, the task is to find all elements in array except two greatest elements in sorted order.
Example 1:
Input :
a[] = {2, 8, 7, 1, 5}
Output :
1 2 5
Explanation :
The output three elements have two or
more greater elements.
Example 2:
Input :
a[] = {7, -2, 3, 4, 9, -1}
Output :
-2 -1 3 4
class Solution{
public:
vector<int> findElements(int a[], int n)
{
sort(a , a+n);
vector <int> v1;
for(int i=0;i<n-2;i++)
v1.push_back(a[i]);
return v1;
}
};
Print the left element
Print the left element | Practice | GeeksforGeeks
Given a array of length N, at each step it is reduced by 1 element. In the first step the maximum element would be…
Given a array of length N, at each step it is reduced by 1 element. In the first step the maximum element would be removed, while in the second step minimum element of the remaining array would be removed, in the third step again the maximum and so on. Continue this till the array contains only 1 element. And find the final element remaining in the array.
Example 1:
Input:
N = 7
A[] = {7, 8, 3, 4, 2, 9, 5}
Ouput:
5
Explanation:
In first step '9' would be removed, in 2nd step
'2' will be removed, in third step '8' will be
removed and so on. So the last remaining
element would be '5'.
class Solution{
public:
int leftElement(int a[], int n) {
// Your code goes here
sort(a, a+n);
int midindex ;
// even number of elements ->
if (n%2 ==0 )
midindex = n /2 -1 ;
else
midindex = n/2 ;
return a[midindex];
}
};
Fascinating Number
Fascinating Number | Practice | GeeksforGeeks
Given a number N. Your task is to check whether it is fascinating or not.Fascinating Number: When a number(should…
Given a number N. Your task is to check whether it is fascinating or not.
Fascinating Number: When a number(should contain 3 digits or more) is multiplied by 2 and 3, and when both these products are concatenated with the original number, then it results in all digits from 1 to 9 present exactly once.
Example 1:
Input:
N = 192
Output: Fascinating
Explanation: After multiplication with 2
and 3, and concatenating with original
number, number will become 192384576
which contains all digits from 1 to 9.
Example 2:
Input:
N = 853
Output: Not Fascinating
Explanation: It's not a fascinating
number.
vector<int>ans;
int n1=2*n;
int n2=3*n;
while(n>0){
ans.push_back(n%10);
n=n/10;
}
while(n1>0){
ans.push_back(n1%10);
n1=n1/10;
}
while(n2>0){
ans.push_back(n2%10);
n2=n2/10;
}
sort(ans.begin(),ans.end());
int sum=0;
for(int i=0;i<ans.size();i++){
if(ans[i]!=i+1){
sum++;
}
}
if(sum>0){
return false;
}
return true;
Average in a stream
Average in a stream | Practice | GeeksforGeeks
Given a stream of incoming numbers, find average or mean of the stream at every point.
Given a stream of incoming numbers, find average or mean of the stream at every point.
Example 1:
Input:
n = 5
arr[] = {10, 20, 30, 40, 50}
Output: 10.00 15.00 20.00 25.00 30.00
Explanation:
10 / 1 = 10.00
(10 + 20) / 2 = 15.00
(10 + 20 + 30) / 3 = 20.00
And so on.
class Solution{
public:
vector<float> streamAvg(int arr[], int n) {
// code here
vector<float> temp;
float sum = 0, avg;
for(int i=1;i<=n;i++)
{
sum = sum + arr[i-1];
avg = sum / i;
temp.push_back(avg);
}
return temp;
}
};
Compete the skills
Compete the skills | Practice | GeeksforGeeks
A and B are good friend and programmers. They are always in a healthy competition with each other. They decide to judge…
A and B are good friend and programmers. They are always in a healthy competition with each other. They decide to judge the best among them by competing. They do so by comparing their three skills as per their values. Please help them doing so as they are busy.
Set for A are like [a1, a2, a3]
Set for B are like [b1, b2, b3]
Compare ith skill of A with ith skill of B
if a[i] > b[i] , A’s score increases by 1
if a[i] < b[i] , B’s score increases by 1
if a[i] = b[i] , nothing happens
Example 1:
Input :
A = {4, 2, 7}
B = {5, 6, 3}
Output :
1 2
class Solution{
public:
void scores(long long a[], long long b[], int &ca, int &cb)
{
for(int i=0;i<3;i++)
{
if(a[i]<b[i]){
cb++;
}
else if(a[i]>b[i])
{
ca++;
}
}
}
};
C++ 2-D Arrays | Set-1
C++ 2-D Arrays | Set-1 | Practice | GeeksforGeeks
Given a 2-d array of integers having N*N elements, print the transpose of the array. Example 1: Input : arr[] =…
Given a 2-d array of integers having N*N elements, print the transpose of the array.
Example 1:
Input : arr[] = {{1,2,3}, {4,5,6},
{7,8,9}} and N = 3
Output : 1 4 7 2 5 8 3 6 9
Explanation:1 2 3 1 4 7
4 5 6 ----> 2 5 8
7 8 9 3 6 9
Transpose of array.
vector<vector<int>> transpose(int a[][M], int n)
{
vector<vector<int>>ans;
for(int i=0;i<n;i++)
{ vector<int>v;
for(int j=0;j<n;j++)
{
v.push_back(a[j][i]);
}
ans.push_back(v);
}
return ans;
}
Compete the skills
Compete the skills | Practice | GeeksforGeeks
A and B are good friend and programmers. They are always in a healthy competition with each other. They decide to judge…
A and B are good friend and programmers. They are always in a healthy competition with each other. They decide to judge the best among them by competing. They do so by comparing their three skills as per their values. Please help them doing so as they are busy.
Set for A are like [a1, a2, a3]
Set for B are like [b1, b2, b3]
Compare ith skill of A with ith skill of B
if a[i] > b[i] , A’s score increases by 1
if a[i] < b[i] , B’s score increases by 1
if a[i] = b[i] , nothing happens
class Solution{
public:
void scores(long long a[], long long b[], int &ca, int &cb)
{
for(int i=0;i<3;i++)
{
if(a[i]<b[i]){
cb++;
}
else if(a[i]>b[i])
{
ca++;
}
}
}
};
Subscribe to my newsletter
Read articles from Akshima Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
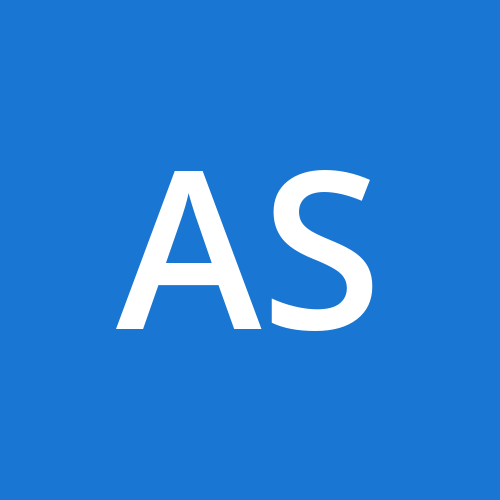