Understanding CRUD Operations: MERN Stack

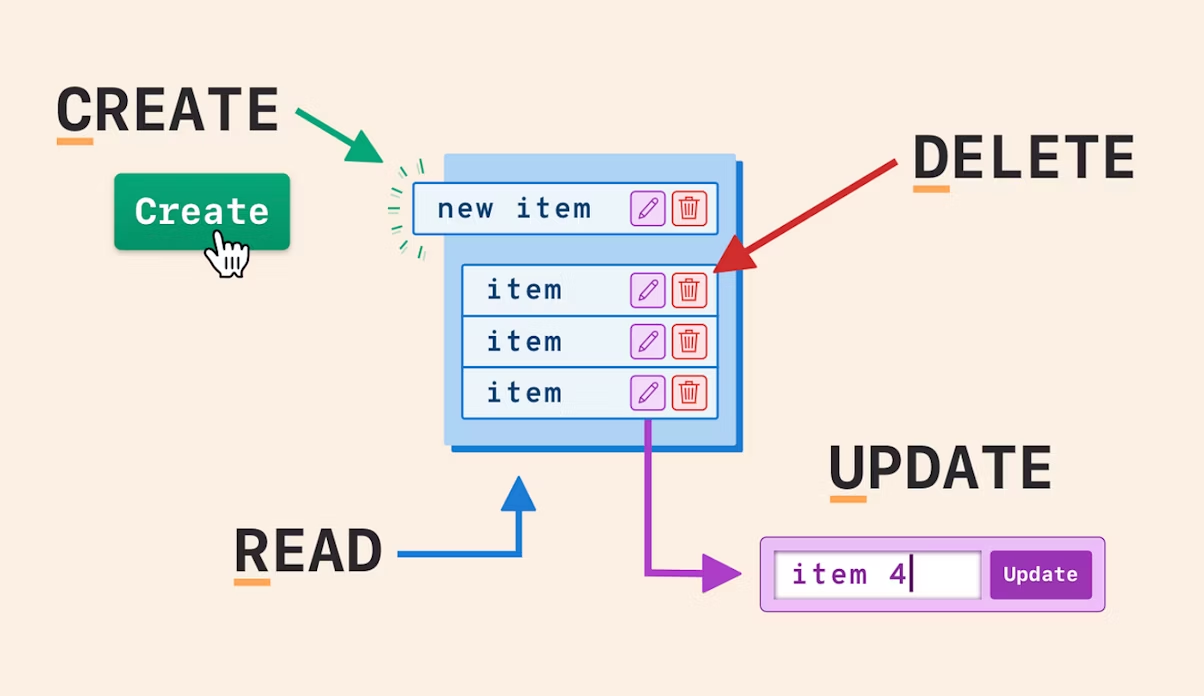
Introduction
CRUD operations form the backbone of any application that interacts with a database.
In the MERN stack (MongoDB, Express, React, Node.js), CRUD operations are essential for managing data effectively.
This blog will explore the importance of CRUD operations, how they are implemented in the MERN stack, and the advantages and disadvantages of using this stack.
What are CRUD Operations?
CRUD stands for "Create, Read, Update, and Delete".
These are the four basic operations required to manage data in a database:
Create: Adding new data to the database.
Read: Retrieving data from the database.
Update: Modifying existing data in the database.
Delete: Removing data from the database.
Importance of CRUD Operations
Fundamental Database Interactions: CRUD operations are the foundation of interacting with any database.
Data Management: They allow for efficient management and manipulation of data.
Consistency: Ensure consistent and reliable data processing.
CRUD Operations in the MERN Stack
The MERN stack combines MongoDB, Express, React, and Node.js to build robust web applications.
Here's a brief overview of how CRUD operations are implemented in the MERN stack:
1. Create
Example: Adding a new user
Backend (Node.js and Express):
javascriptCopy codeapp.post('/users', (req, res) => { const newUser = new User(req.body); newUser.save().then(() => res.status(201).send(newUser)); });
Frontend (React):
javascriptCopy codeconst addUser = async (user) => { await fetch('/users', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify(user) }); };
2. Read
Example: Fetching all users
Backend (Node.js and Express):
javascriptCopy codeapp.get('/users', (req, res) => { User.find().then(users => res.send(users)); });
Frontend (React):
javascriptCopy codeconst fetchUsers = async () => { const response = await fetch('/users'); const data = await response.json(); setUsers(data); };
3. Update
Example: Updating a user's information
Backend (Node.js and Express):
javascriptCopy codeapp.put('/users/:id', (req, res) => { User.findByIdAndUpdate(req.params.id, req.body, { new: true }) .then(updatedUser => res.send(updatedUser)); });
Frontend (React):
javascriptCopy codeconst updateUser = async (id, user) => { await fetch(`/users/${id}`, { method: 'PUT', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify(user) }); };
4. Delete
Example: Deleting a user
Backend (Node.js and Express):
javascriptCopy codeapp.delete('/users/:id', (req, res) => { User.findByIdAndDelete(req.params.id).then(() => res.status(204).send()); });
Frontend (React):
javascriptCopy codeconst deleteUser = async (id) => { await fetch(`/users/${id}`, { method: 'DELETE' }); };
Advantages of CRUD Operations in the MERN Stack
Efficiency: MERN stack allows for efficient handling of CRUD operations, making data management seamless.
Scalability: MongoDB provides excellent scalability options, accommodating large datasets.
Flexibility: With React on the frontend, the UI can be dynamically updated to reflect CRUD operations.
Performance: Node.js ensures high performance and scalability for backend operations.
Disadvantages of CRUD Operations in the MERN Stack
Complexity: Initial setup and configuration can be complex, especially for beginners.
Learning Curve: Developers need to be proficient in JavaScript and understand the nuances of each component of the MERN stack.
Debugging: Debugging issues across different components (MongoDB, Express, React, Node.js) can be challenging.
Why Choose CRUD Operations in the MERN Stack?
Full-Stack Solution: The MERN stack provides a comprehensive full-stack solution for building web applications.
JavaScript Everywhere: Using JavaScript across the entire stack (frontend and backend) simplifies development and streamlines the learning process.
Active Community: The MERN stack has a large and active community, providing ample resources and support.
Modern Development Practices: It incorporates modern development practices, making it suitable for contemporary web applications.
Conclusion
CRUD operations are essential for any application that interacts with a database.
The MERN stack offers a powerful and efficient way to implement these operations, leveraging the strengths of MongoDB, Express, React, and Node.js.
While there are some challenges, the advantages often outweigh the drawbacks, making the MERN stack a popular choice for developers.
Subscribe to my newsletter
Read articles from Rohan Shrivastava directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Rohan Shrivastava
Rohan Shrivastava
Hi, I'm Rohan, a B.Tech graduate in Computer Science (Batch 2022) with expertise in web development (HTML, CSS, JavaScript, Bootstrap, PHP, XAMPP). My journey expanded with certifications and intensive training at Infosys, covering DBMS, Java, SQL, Ansible, and networking. I've successfully delivered projects, including a dynamic e-commerce site and an Inventory Management System using Java. My proactive approach is reflected in certifications and contributions to open-source projects on GitHub. Recognized for excellence at Infosys, I bring a blend of technical proficiency and adaptability. Eager to leverage my skills and contribute to innovative projects, I'm excited about exploring new opportunities for hands-on experiences. Let's connect and explore how my skills align with your organization's goals.