Managing Azure Automation Runtime Environments via PowerShell

Table of contents
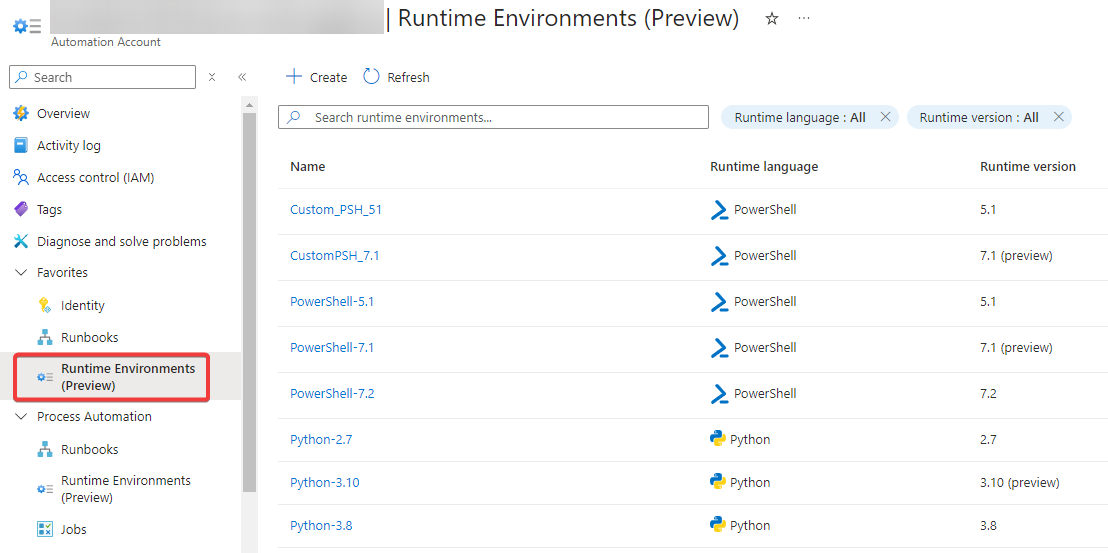
You may have heard about a new (currently in preview) feature called Runtime Environment.
The main features are:
You can have multiple custom runtime environments that can be used across numerous Runbooks (a.k.a. one runtime in multiple runbooks)
Testing of new module versions is super easy, you create a new runtime, import modules you want to test, switch to this runtime in your runbook, and see how it goes
Testing of the new Runtime language version is super easy
All of this can be automated via direct API calls
Today I will show you how to manage the whole Runtime Environment lifecycle including modules management through my PowerShell module AzureResourceStuff.
By the way, there is official documentation about managing Runtime Environments via API, but it lacks a lot of information, therefore I had to use web browser Developer Tools (F12) to get what I needed in most cases 😎
Before we begin
Enable Runtime Environments (Preview)
Open your testing Automation Account in the Azure web portal interface.
Manually switch to the new Runtime experience before you continue!
By the way, you can switch back using Switch to Old Experience
button any time.
Now when switching to the new experience, you should see a new menu Runtime Environments (Preview)
in your Automation Account left pane.
Now, we can create new runtimes, import modules, and more manually or using PowerShell commands, as shown below.
Install AzureResourceStuff module
To be able to use my PowerShell commands, you must first install AzureResourceStuff module from the PowerShell Gallery.
Install-Module AzureResourceStuff
Get-Command -Name automationRuntime -module AzureResourceStuff
Runtime environment functions
Now I show you a few basic actions you want to make with your Runtimes. Be sure to check functions help (via Get-Help
) to get more details and examples though!
Import-Module Az.Accounts
Connect-AzAccount
Set-AzContext -Subscription "<nameOfYourSubscription>"
Get all Runtime Environments
Get-AzureAutomationRuntime
Create a new Runtime Environment
$defaultPackage = @{
az = '8.0.0'
}
New-AzureAutomationRuntime -runtimeName 'CustomPSH_7.2' -runtimeLanguage 'PowerShell' -runtimeVersion '7.2' -defaultPackage $defaultPackage
Add a custom PSH module
Custom modules are imported from PSH Gallery or a ZIP file.
# import newest version of the 'CommonStuff' module (including all required dependencies) from the PowerShell Gallery
New-AzureAutomationRuntimeModule -moduleName 'CommonStuff' -runtimeName 'CustomPSH_7.2'
# import module archived in the selected zip file
New-AzureAutomationRuntimeZIPModule -moduleZIPPath "C:\DATA\helperFunctions.zip" -runtimeName 'CustomPSH_7.2'
Update a custom PSH module
# update 'CommonStuff' module to the newest version
Update-AzureAutomationRunbookModule -moduleName 'CommonStuff' -runtimeName 'CustomPSH_7.2'
# update/downgrade 'CommonStuff' module to the specified version
Update-AzureAutomationRunbookModule -moduleName 'CommonStuff' -moduleVersion '1.0.15' -runtimeName 'CustomPSH_7.2'
# update all custom modules to their newest version
Update-AzureAutomationRunbookModule -allCustomModule -runtimeName 'CustomPSH_7.2'
# replace existing module with the one saved in selected zip file
New-AzureAutomationRuntimeZIPModule -moduleZIPPath "C:\DATA\helperFunctionsv2.zip" -runtimeName 'CustomPSH_7.2'
Remove a custom PSH module
Remove-AzureAutomationRuntimeModule -moduleName 'CommonStuff' -runtimeName 'CustomPSH_7.2'
Get available default modules
Default modules are pre-built into every runtime. You select whether you want to use it (and which version) or don’t want to use it at all.
Get-AzureAutomationRuntimeAvailableDefaultModule
Set default module version
The default (built-in) modules are az
, azure cli
a.k.a. the ones selected from the dropdown menu in the Azure portal GUI.
$defaultPackage = @{
'azure cli' = '2.56.0'
}
# replace existing default modules with new setting (remove 'az' completely)
Set-AzureAutomationRuntimeDefaultModule -defaultPackage $defaultPackage -runtimeName 'CustomPSH_7.2' -replace
$defaultPackage = @{
'az' = '8.3.0'
'azure cli' = '2.56.0'
}
# replace existing default modules with new setting
Set-AzureAutomationRuntimeDefaultModule -defaultPackage $defaultPackage -runtimeName 'CustomPSH_7.2'
# remove all default modules
Set-AzureAutomationRuntimeDefaultModule -defaultPackage @{} -runtimeName 'CustomPSH_7.2'
Get Runtime selected default modules
Get-AzureAutomationRuntimeSelectedDefaultModule
Make Runtime Environment copy
Copy-AzureAutomationRuntime -runtimeName "CustomPSH_7.2" -newRuntimeName "CustomPSH_7.2_v2"
Remove Runtime Environment
Remove-AzureAutomationRuntime -runtimeName 'CustomPSH_7.2'
Runbook functions
Here are some of the functions related to Runbooks
Get Runbook script content
Get-AzureAutomationRunbookContent -runbookName someRunbook -ResourceGroupName Automations -AutomationAccountName someAutomationAccount
Set Runbook script content
$content = @'
Get-process notepad
restart-service spooler
'@
Set-AzureAutomationRunbookContent -runbookName someRunbook -ResourceGroupName Automations -AutomationAccountName someAutomationAccount -content $content
Get Runtime used in selected Runbook
Get-AzureAutomationRunbookRuntime
Set Runbook Runtime
Set-AzureAutomationRunbookRuntime
Set Runbook description
Set-AzureAutomationRuntimeDescription
Start Runbook test run using selected Runtime
Useful if you want to automate testing of the new Runtimes a.k.a. that your Runbook will successfully end with this new Runtime (modules) version, before the final assignment.
Start-AzureAutomationRunbookTestJob -runtimeName "CustomPSH_7.2_v2" -runbookName "ExchangeSetAuditSettings"
Stop Runbook test run
Stop-AzureAutomationRunbookTestJob
Get Runbook test run output
# get the output as array of string
Get-AzureAutomationRunbookTestJobOutput -runbookName "ExchangeSetAuditSettings" -justText 'Output', 'Warning', 'Error', 'Exception'
# get the output as array of objects
Get-AzureAutomationRunbookTestJobOutput -runbookName "ExchangeSetAuditSettings"
Get Runbook test run status
# get test run status
Get-AzureAutomationRunbookTestJobStatus -runbookName "ExchangeSetAuditSettings"
Subscribe to my newsletter
Read articles from Ondrej Sebela directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ondrej Sebela
Ondrej Sebela
I work as System Administrator for more than 10 years now and I love to make my life easier by automating work & personal stuff via PowerShell (even silly things like food recipes list generation).