Unlocking the Power of V8 Debug: Tips and Techniques for JavaScript Developers

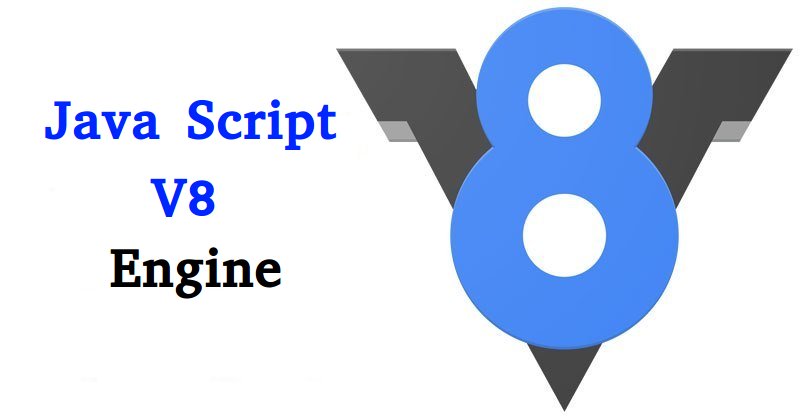
Understanding V8 and Its Debugging Tools
What is V8?
V8 is Google’s open-source high-performance JavaScript and WebAssembly engine, written in C++. It is used in Google Chrome and Node.js, among other projects. V8 compiles JavaScript directly to native machine code before executing it, which makes it fast and efficient.
Learn more about V8: https://v8.dev/docs
Types of Arrays in V8
V8 optimizes JavaScript arrays by categorizing them based on their content and usage. Understanding these types can help you write more efficient code:
Packed Arrays: Dense arrays with contiguous elements, which are highly optimized.
let packedArray = [1, 2, 3, 4, 5]; console.log(packedArray); // [1, 2, 3, 4, 5]
Holey Arrays: Arrays with gaps or holes, leading to less efficient memory usage.
let holeyArray = [1, , 3, , 5]; console.log(holeyArray); // [1, empty, 3, empty, 5]
Double Packed Arrays: Arrays that contain only double (64-bit floating-point) values.
let doublePackedArray = [1.1, 2.2, 3.3, 4.4]; console.log(doublePackedArray); // [1.1, 2.2, 3.3, 4.4]
SMI Arrays (Small Integer Arrays): Arrays containing small integers, optimized for integer arithmetic.
let smiArray = [1, 2, 3, 4, 5]; console.log(smiArray); // [1, 2, 3, 4, 5]
Semi-Packed Arrays: Arrays that are mostly packed but may have a few gaps.
let semiPackedArray = [1, 2, , 4, 'five']; console.log(semiPackedArray); // [1, 2, empty, 4, "five"]
Learn more about array types in V8: https://v8.dev/blog/elements-kinds
Getting Started with V8 Debug
Chrome DevTools Protocol
The Chrome DevTools Protocol is a set of APIs that allows you to inspect, debug, and profile JavaScript code running in V8. Chrome DevTools provides a rich interface to leverage these APIs, making it a powerful tool for debugging.
Node.js Inspector Protocol
Node.js includes a built-in debugger that uses the V8 Inspector Protocol. This allows you to debug server-side JavaScript applications effectively.
Key Features of V8 Debug
Breakpoints
Set breakpoints to pause execution at specific lines of code, functions, or events. This allows you to inspect the state of your application and understand its behavior at crucial points.
Call Stack Inspection
View the call stack to understand the sequence of function calls that led to the current execution point. This is useful for tracing the flow of your application and identifying where things might be going wrong.
Scope and Variables
Inspect local, closure, and global variables to see their current values. You can also modify variable values at runtime to test different scenarios.
Watch Expressions
Monitor specific expressions or variables to see how their values change over time. This helps in tracking down issues related to variable state changes.
Event Listener Breakpoints
Pause execution when specific types of events (e.g., DOM events, XHR events) are triggered. This is particularly useful for debugging asynchronous code.
Console and REPL
Use the interactive console to evaluate JavaScript expressions and interact with your running code. The Read-Eval-Print Loop (REPL) allows you to execute snippets of code and see the results immediately.
Profiling and Performance Monitoring
Profile your JavaScript execution to identify performance bottlenecks. Analyze memory usage and garbage collection events to optimize your application.
Using V8 Debug in Different Environments
Chrome DevTools
Chrome DevTools is integrated into the Chrome browser, providing a comprehensive suite of debugging and profiling tools for client-side JavaScript. You can access it via the browser menu: More Tools > Developer Tools
or by pressing F12
or Ctrl+Shift+I
.
Node.js
Node.js provides a built-in debugger that can be accessed via command-line options. Start Node.js with the --inspect
flag to enable the inspector:
node --inspect your-script.js
Use --inspect-brk
to pause execution on the first line of the script:
node --inspect-brk your-script.js
Connect to the debugger via Chrome DevTools by navigating to chrome://inspect
.
Integrated Development Environments (IDEs)
Many modern IDEs (e.g., Visual Studio Code, WebStorm) provide integrated support for V8 debugging. These IDEs typically use the Chrome DevTools Protocol or the Node.js Inspector Protocol, allowing you to set breakpoints, inspect variables, and step through code directly within the editor.
Using jsvu for Managing JavaScript Engines
jsvu
(JavaScript VU) is a command-line tool that helps you easily download and manage various JavaScript engines, including V8. It can be particularly useful for testing and debugging across different engine versions.
Installing jsvu
First, install jsvu
using npm:
npm install -g jsvu
Using jsvu to Manage V8
Once installed, you can use jsvu
to install the latest version of V8:
jsvu
Follow the prompts to download and set up the desired JavaScript engines. You can then run V8 directly from the command line:
v8
Learn more about jsvu
: https://github.com/GoogleChromeLabs/jsvu
Practical Tips and Techniques
Set Conditional Breakpoints: Use conditions to break only when certain criteria are met, reducing the noise and focusing on relevant issues.
Use the Console for Experimentation: Test snippets of code directly in the console to quickly identify issues and test fixes.
Profile Early and Often: Regularly profile your application to catch performance bottlenecks before they become critical.
Leverage Watch Expressions: Keep an eye on specific variables or expressions to understand how and when their values change.
Inspect Event Listeners: Debug event-driven code more effectively by pausing execution on specific events.
Conclusion
Unlocking the full potential of V8 Debug can significantly enhance your JavaScript development workflow. By leveraging the powerful tools and techniques available in Chrome DevTools and Node.js, you can optimize your code, quickly identify and fix issues, and ensure your applications run smoothly. Whether you are working on client-side or server-side JavaScript, mastering V8 Debug is a valuable skill for any developer.
Subscribe to my newsletter
Read articles from Naveen Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
