Getting started with generator functions in javascript.
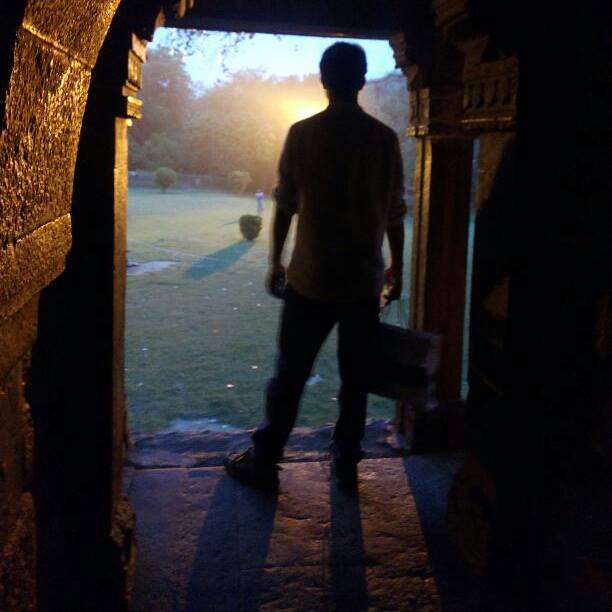
Generator functions in Javascript return a "Generator" Object.
And a generator in return is a type of iterator. We can say an object is iterator when it has a next method
someIterator.next() // {value:"some value",done: false)
someIterator.next() // {value:"some value",done: false)
someIterator.next() //{value:"some value",done: true)
we can create custom iterators ourselves,Generator functions are syntactic sugar way of creating iterators.Before we start seeing generator functions ,lets see an iterator in action
const dragons = ["Drogon", "Rhaegal","Viserion"];
const iterator = dragons[Symbol.iterator]();
console.log("iteraotor")
console.log(iterator.next())//{ value: "Drogon", done: false }
console.log(iterator.next())// { value: "Rhaegal", done: false }
console.log(iterator.next())// { value: "Viserion", done: false }
console.log(iterator.next()) //{ value: undefined, done: true }
As we can iterator object next function returns an object with two properties "value" and "done". done will be false untill we have iterated over the iterator.
now lets start creating iterators with generator functions.
1st step is generator function declaration
it's like usual function declarations but we tell Javascript that we are defining a generator function by using a star * with function e.g
const counter = function *() {
yield 1;
yield 2;
yield 3;
}
Yield Statement: The yield
keyword pauses the function and returns the current value of count
.
2 Create the generator object
to create generator object ,just call the generator function ,as we discussed above generator function returns "Generator" object
const counterGenerator = counter()
and voila!!! we have our iterator i.e generator Object . and we can call next on our iterator.
counterGenerator.next() // {value :1 ,done:false}
counterGenerator.next() // {value :2 ,done:false}
counterGenerator.next() // {value :3 ,done:false}
counterGenerator.next() // {value :undefined ,done:true}
once its done it's done. we will have to create new generator to loop over it again.
Now generator object confirms to iterator protocol and so we have the next() method on it. Generator object also confirms to iterable protocol which means we can use for of loop to loop over the object
const counterGenerator1 = counter();
for (let count of counterGenerator1) {
console.log("count", count);// 1 2 3
}
Note : Notice above we created a new generator object counterGenerator1 from the same generator function counter,we created a new generator object as counterGenerator was already iterated thru and was done .
This was short introduction to generators .there is a tonne of stuff which can be done using generators ,we can create infinite loops with out encountering stack over flow .
and then There are async generators , which conforms to both the async iterable protocol and the async iterator protocol. Async generator methods always yield Promise
objects.
We will learn more about async generators in upcoming blog .
Subscribe to my newsletter
Read articles from Ajay directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
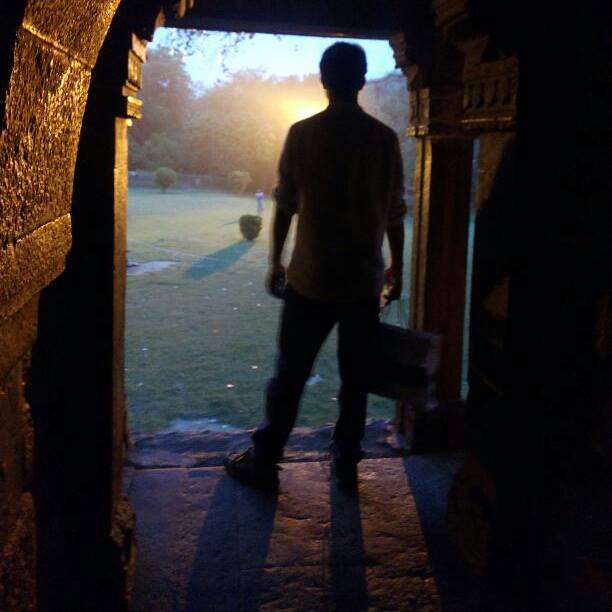
Ajay
Ajay
I am web developer from India, who loves everything related to web development, martial arts, writing, playing outdoor games and hanging out with honest minded folks