Redux Toolkit: A Simple Guide

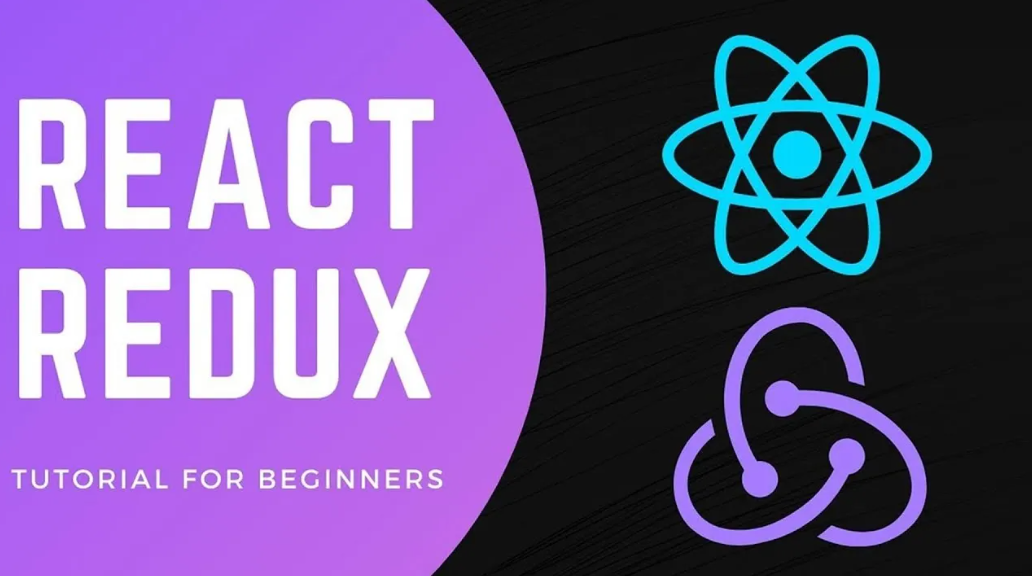
State management in large-scale React applications can become complex and unwieldy. Enter Redux Toolkit (RTK), a powerful solution that simplifies Redux development. If you're new to Redux or have struggled with its boilerplate code, RTK might be just what you need. In this blog, we'll explore what RTK is, its key features, and how to get started with it.
What is Redux Toolkit?
Redux Toolkit is the official, recommended way to write Redux logic. It provides a set of tools and best practices to simplify state management in your React applications. RTK is designed to address the common pain points of Redux, such as boilerplate code, complicated setup, and unnecessary complexity.
Key Features of Redux Toolkit
Simplified Configuration: RTK provides a
configureStore
function that simplifies the store setup process. It automatically sets up the Redux DevTools and includes sensible defaults for middleware.Reduced Boilerplate: With RTK, you can write less code to achieve the same functionality. It introduces
createSlice
, which combines reducers, actions, and action creators in one place.Built-in Thunk Middleware: RTK includes
redux-thunk
by default, making it easier to write asynchronous logic in your Redux actions.Immutability with Immer: RTK uses Immer under the hood, allowing you to write simpler and more readable immutable update logic.
Better Performance: RTK's best practices and simplified setup can lead to better performance and maintainability.
Getting Started with Redux Toolkit
Let's walk through the steps to get started with Redux Toolkit in a React application.
Step 1: Install Redux Toolkit
First, you'll need to install Redux Toolkit and React-Redux.
npm install @reduxjs/toolkit react-redux
Step 2: Create a Slice
A slice is a collection of Redux reducer logic and actions for a single feature of your app. Here's an example of creating a slice for managing a counter:
import { createSlice } from '@reduxjs/toolkit';
const counterSlice = createSlice({
name: 'counter',
initialState: { value: 0 },
reducers: {
increment: state => {
state.value += 1;
},
decrement: state => {
state.value -= 1;
},
reset: state => {
state.value = 0;
}
}
});
export const { increment, decrement, reset } = counterSlice.actions;
export default counterSlice.reducer;
Step 4: Provide the Store to Your React App
Wrap your application with the Provider
component from React-Redux, passing in the store.
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import App from './App';
import store from './store';
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
Step 5: Use Redux State and Actions in Components
Now, you can use the useSelector
and useDispatch
hooks to interact with the Redux state in your components.
import React from 'react';
import { useSelector, useDispatch } from 'react-redux';
import { increment, decrement, reset } from './counterSlice';
const Counter = () => {
const count = useSelector(state => state.counter.value);
const dispatch = useDispatch();
return (
<div>
<h1>{count}</h1>
<button onClick={() => dispatch(increment())}>Increment</button>
<button onClick={() => dispatch(decrement())}>Decrement</button>
<button onClick={() => dispatch(reset())}>Reset</button>
</div>
);
};
export default Counter;
Conclusion
Redux Toolkit streamlines the process of using Redux in your React applications. It reduces boilerplate, improves code readability, and includes powerful tools out of the box. By adopting RTK, you can manage your app's state more efficiently and focus on building features rather than wrestling with complex setup and configuration.
Give Redux Toolkit a try in your next project and experience the benefits of modern state management in React!
"If you found this helpful, please like, comment, and share! Have questions? Leave them in the comments below!"
Subscribe to my newsletter
Read articles from Yashaswi Sahu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
