C++ Algorithms and STL: Expert Guide - Part 2
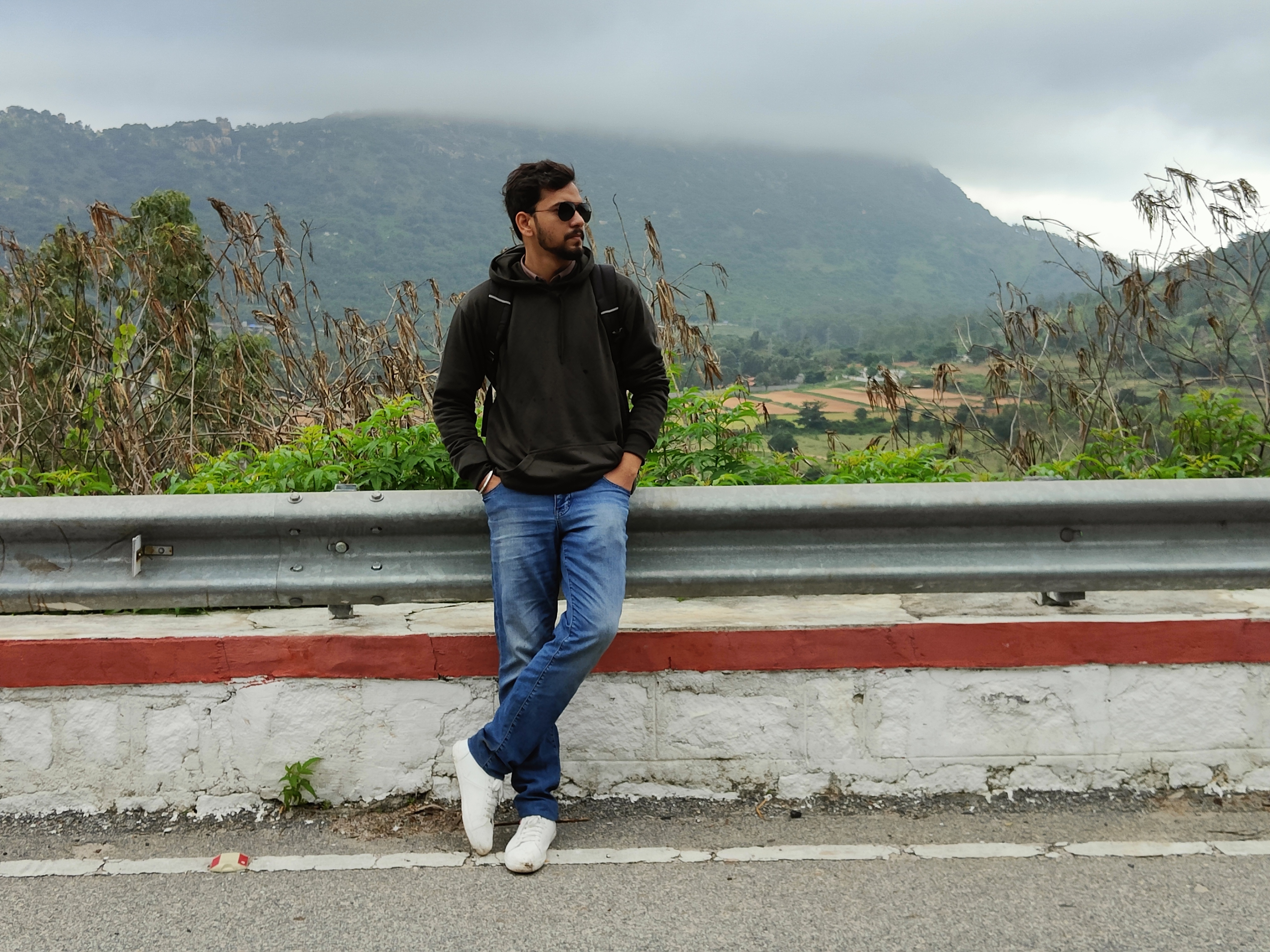
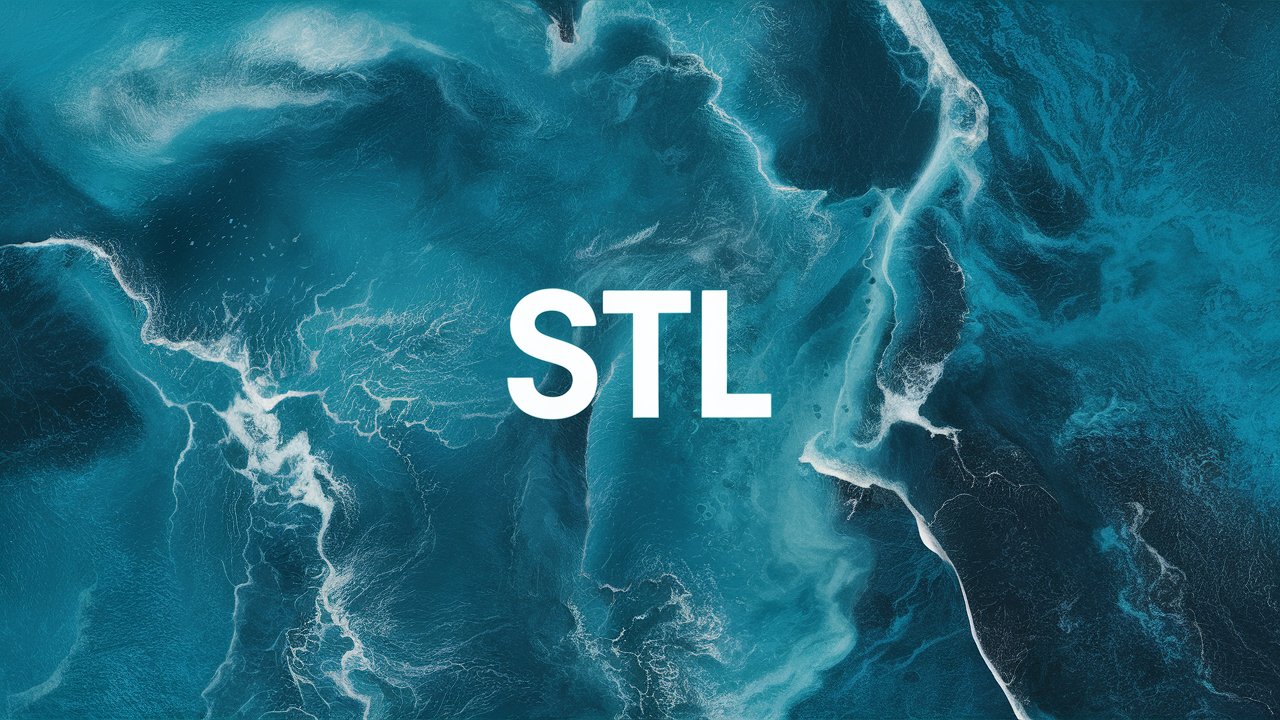
The C++ Standard Template Library(STL) includes a wide range of algorithms that operate on various container types(such as vectors, lists, sets, and maps) and provide essential functionality for data manipulation. These algorithms are defined in the <algorithm> header and are part of the STL's core functionality.
Iterators and Iterating Algorithms:
for_each: Applies a function to each element in a range.
find: Searches for a specific element in a range.
count: Count the occurrences of a value in a range.
count_if: Counts the elements that satisfy a given predicate.
sort: Sorts the element in a range in ascending order.
reverse: Reverses the order of elements in a range.
rotate: Rotates elements in a range.
unique: Removes duplicate elements from a sorted range.
partition: Divides elements in a range into two groups based on a predicate.
Numeric Algorithms:
accumulate: Computes the sum of elements in a range.
inner_product: Computes the inner product of two ranges.
partial_sum: Computes the partial sums of a range.
iota: Fills a range with incrementing values.
Searching and Finding Algorithm:
binary_search: Checks if a value exists in a sorted range.
lower_bound: Finds the first element greater or equal to a value in a sorted range.
upper_bound: Finds the first element greater than a value in a sorted range.
Min and Max Algorithms:
min(): Returns the smaller of two values.
max(): Returns the maximum of two values.
min_element(): Finds the smallest element in a range.
max_element(): Finds the largest element in a range.
Heap Algorithms:
make_heap(): Converts a range into a max-heap.
push_heap(): Inserts an element into a max-heap.
pop_heap(): Removes the largest element from a max-heap.
sort_heap(): Sorts a range that represents a max-heap.
Set Algorithms:
set_union(): Computes the union of two sorted ranges.
set_intersection(): Computes the intersection of two sorted ranges.
set_difference(): Computes the difference between two sorted ranges.
set_symmetric_difference(): Computes the symmetric difference of two sorted ranges.
Read my blogs in this series: [https://devrishabh.hashnode.dev/getting-started-with-c-stl-containers-part-1]
Subscribe to my newsletter
Read articles from Rishabh Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
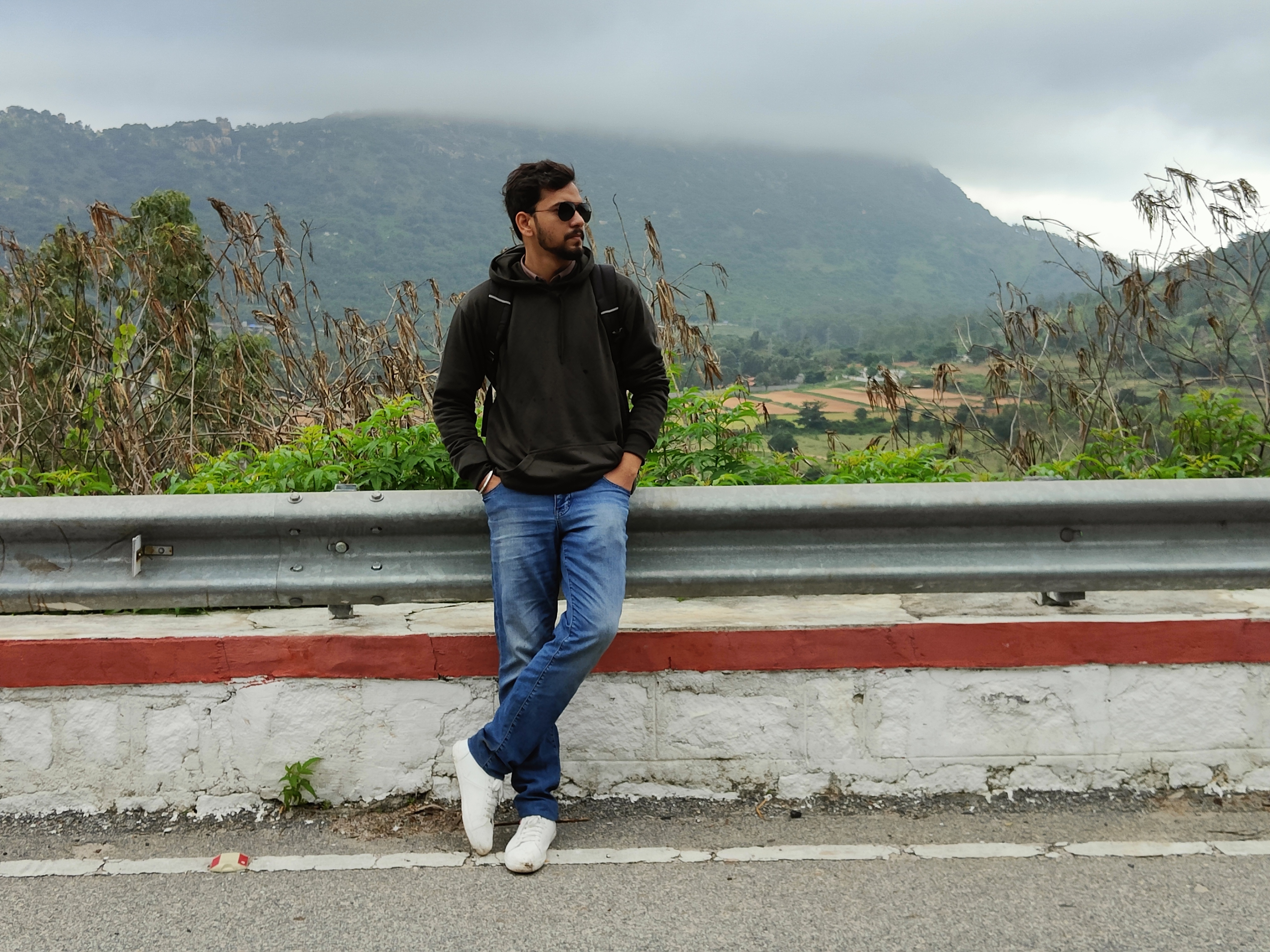