What’s New in React 19?
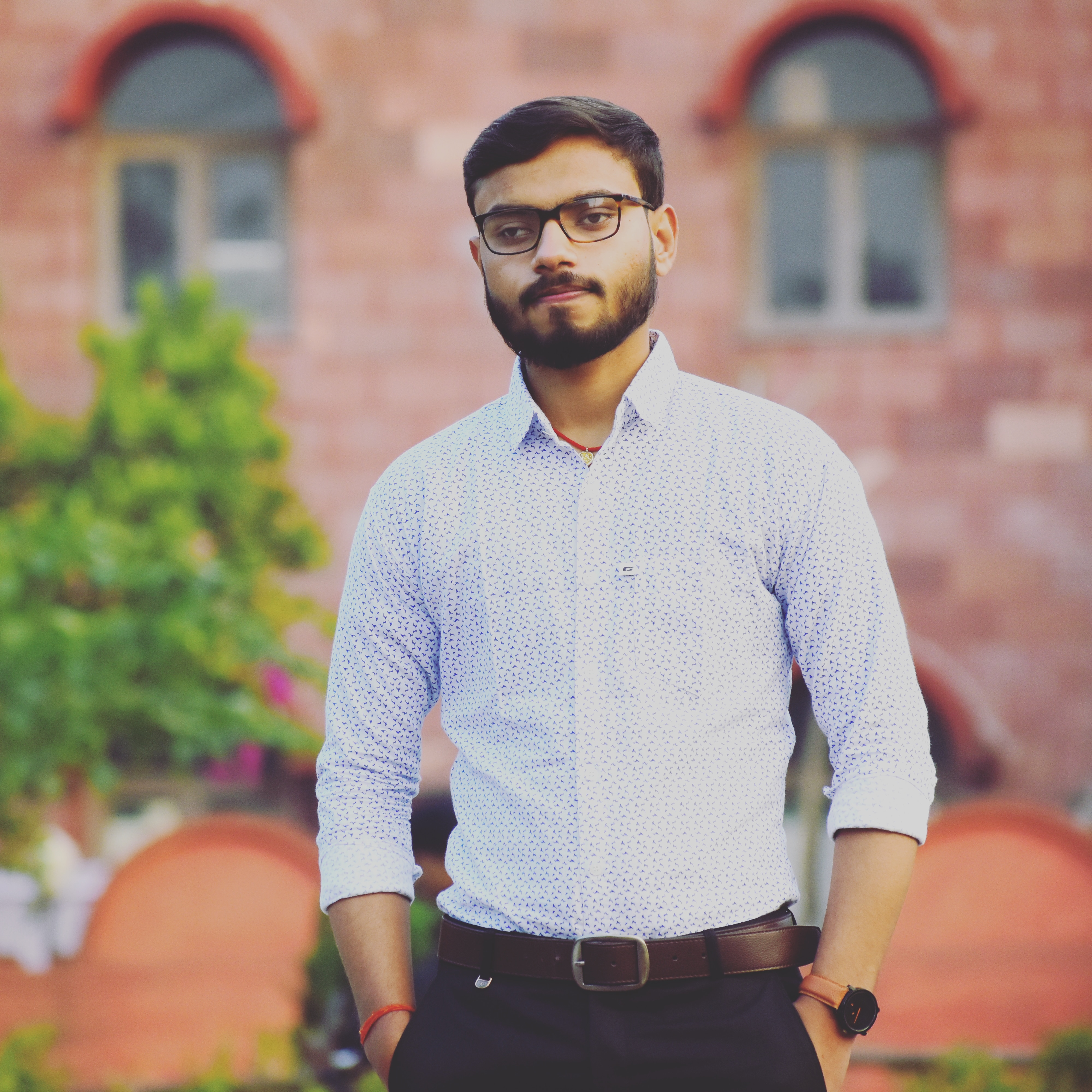
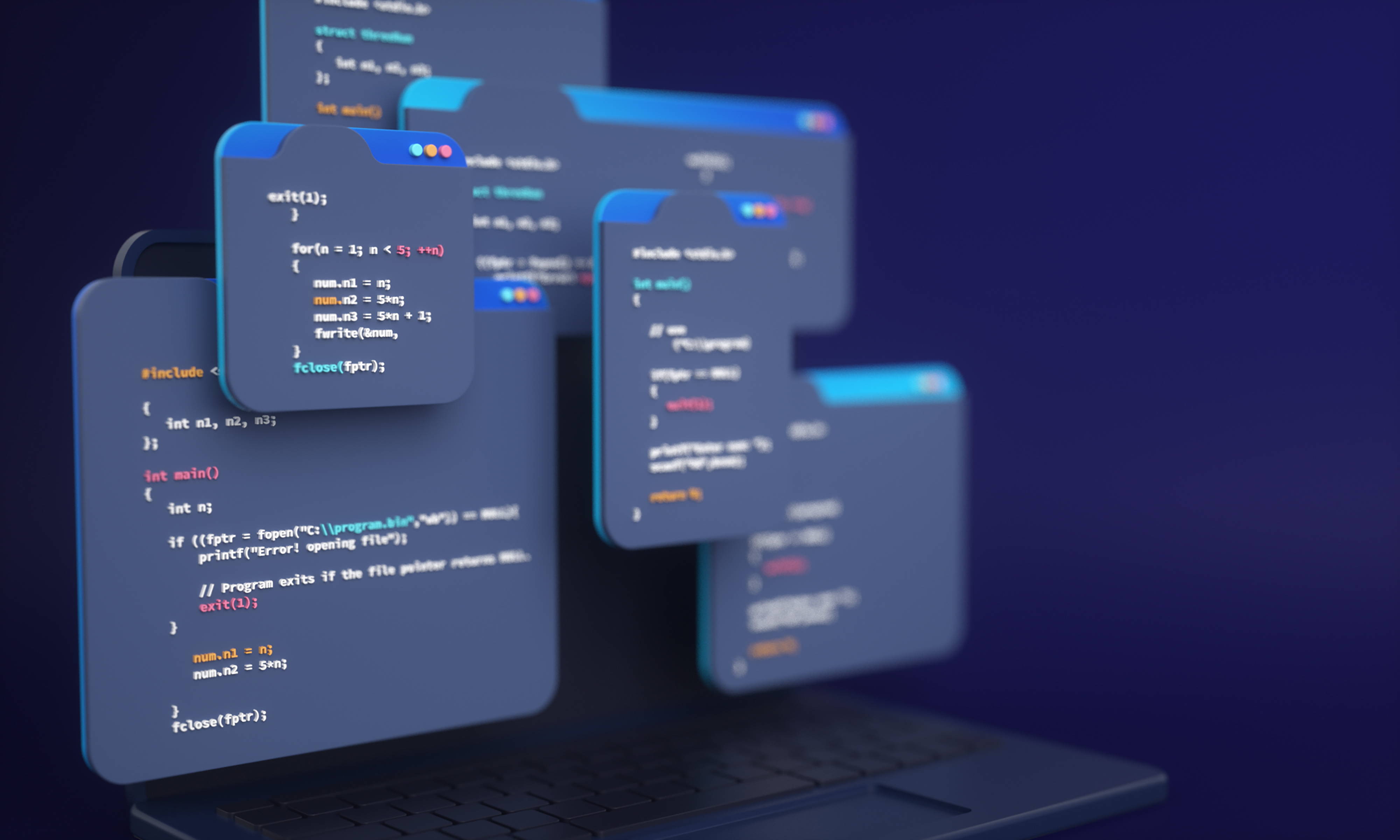
React 19 introduces several exciting features that make managing state, document metadata, handling errors, and working with asynchronous tasks easier for developers. These improvements aim to simplify development and optimize performance in React applications.
Introducing Exciting New Features in React 19! 🚀
Actions for Asynchronous Functions
With the new Actions feature, handling asynchronous requests becomes simpler. Actions automatically manage pending states, errors, and optimistic updates. By using useTransition
, developers can handle async operations more intuitively, keeping the UI responsive during data changes.
function UpdateName() {
const [name, setName] = useState("");
const [error, setError] = useState(null);
const [isPending, startTransition] = useTransition();
const handleSubmit = () => {
startTransition(async () => {
const error = await updateName(name);
if (error) {
setError(error);
return;
}
redirect("/path");
})
};
return (
<div>
<input value={name} onChange={(event) => setName(event.target.value)} />
<button onClick={handleSubmit} disabled={isPending}>
Update
</button>
{error && <p>{error}</p>}
</div>
);
}
Simplified Form Handling
The new <form>
Actions make form submissions easier. The useActionState
hook manages form states seamlessly, replacing the older useFormState
.
function ChangeName({ name, setName }) {
const [error, submitAction, isPending] = useActionState(
async (previousState, formData) => {
const error = await updateName(formData.get("name"));
if (error) {
return error;
}
redirect("/path");
return null;
},
null,
);
return (
<form action={submitAction}>
<input type="text" name="name" />
<button type="submit" disabled={isPending}>Update</button>
{error && <p>{error}</p>}
</form>
);
}
Optimistic Updates with useOptimistic
The useOptimistic
hook lets developers display changes immediately on the UI while the actual data updates in the background. This hook handles temporary UI states and reverts to the original state if there's an error.
function ChangeName({currentName, onUpdateName}) {
const [optimisticName, setOptimisticName] = useOptimistic(currentName);
const submitAction = async formData => {
const newName = formData.get("name");
setOptimisticName(newName);
const updatedName = await updateName(newName);
onUpdateName(updatedName);
};
return (
<form action={submitAction}>
<p>Your name is: {optimisticName}</p>
<p>
<label>Change Name:</label>
<input
type="text"
name="name"
disabled={currentName !== optimisticName}
/>
</p>
</form>
);
}
Accessing Form Status with useFormStatus
The useFormStatus
hook allows you to access form submission statuses directly, making it easier to design components that reflect the form's state without needing to pass props.
import { useFormStatus } from 'react-dom';
function SubmitButton() {
const { pending } = useFormStatus();
return <button type="submit" disabled={pending} />;
}
New Reading API: use
The new use
API helps read resources like promises and contexts during the render phase, supporting conditional reads and managing data dependencies more effectively.
import { use } from 'react';
function Comments({ commentsPromise }) {
const comments = use(commentsPromise);
return comments.map(comment => <p key={comment.id}>{comment}</p>);
}
function Page({ commentsPromise }) {
return (
<Suspense fallback={<div>Loading...</div>}>
<Comments commentsPromise={commentsPromise} />
</Suspense>
);
}
React Server Components and Server Actions🏗️
React 19 enhances performance with Server Components and Server Actions, optimizing resource usage by moving certain tasks to the server.
Server Components
Render components on the server, improving SEO and performance by reducing client-side work.
Server Actions
Allow client components to call server-side functions seamlessly, handled by the framework.
Other Improvements in React 19
Ref as a Prop
Function components can now use ref
as a prop without needing forwardRef
.
function MyInput({ placeholder, ref }) {
return <input placeholder={placeholder} ref={ref} />;
}
<MyInput ref={ref} />;
Diffs for Hydration Errors
Improved error reporting for hydration mismatches.
Context as a Provider
Use <Context>
instead of <Context.Provider>
, simplifying the API.
const ThemeContext = createContext('');
function App({ children }) {
return (
<ThemeContext value="dark">
{children}
</ThemeContext>
);
}
Cleanup Functions for Refs
Ref callbacks can now return cleanup functions for better resource management.
<input
ref={(ref) => {
// setup
return () => {
// cleanup
};
}}
/>
useDeferredValue
Initial Value
The useDeferredValue
hook now accepts an initial value, improving the handling of deferred state updates.
function Search({ deferredValue }) {
const value = useDeferredValue(deferredValue, '');
return <Results query={value} />;
}
When you set an initialValue, useDeferredValue uses it for the first render of the component. Then, it schedules another render with the deferred value happening in the background.
Enhanced Resource Support🤝
Document Metadata Support
Now, React 19 natively supports rendering document metadata tags like <title>
, <link>
, and <meta>
directly within components. This means you can easily manage metadata within your React apps without relying on external libraries like react-helmet. It ensures smoother compatibility across different rendering scenarios.
Stylesheets Support
Managing stylesheets becomes easier with React 19’s built-in support for both external and inline stylesheets. Components can now specify the priority of stylesheets, allowing React to handle their insertion order efficiently in the DOM. This is particularly useful for scenarios involving concurrent rendering and server-side rendering.
Async Scripts Support
React 19 enhances support for async scripts by enabling them to be rendered anywhere within the component tree. React ensures that async scripts are loaded only once, even if rendered by multiple components, preventing duplication and streamlining integration into React applications.
Support for Preloading Resources
New APIs introduced in React 19 enable developers to load and preload browser resources more efficiently. You can now prefetch DNS requests, preconnect to servers, and preload fonts, stylesheets, and scripts, improving overall resource loading efficiency and enhancing user experience, especially during initial page loads.
Support for Custom Elements
React 19 now fully supports custom elements, passing all tests on Custom Elements Everywhere. This update resolves past challenges by handling unrecognized props appropriately during both server-side and client-side rendering. This means you can seamlessly integrate custom elements into your React applications with confidence.
Enhanced Compatibility with Third-Party Scripts and Extensions
React 19 improves compatibility with third-party scripts and browser extensions during hydration, minimizing disruptions and errors for a smoother user experience.
Improved Hydration Compatibility
During hydration, React intelligently bypasses unexpected tags caused by third-party scripts instead of triggering re-render errors. This approach reduces potential disruptions stemming from external scripts and extensions, ensuring a smoother user experience overall.
Improved Error Reporting
Error reporting in React 19 is streamlined to provide clearer insights into component failures. Now, instead of cluttering the console with redundant messages, React logs a single error message with all the necessary information, making debugging easier and more efficient.
Conclusion
With streamlined state management, improved error handling, and simplified asynchronous operations, React 19 offers powerful features for web development. Server Components and Server Actions optimize performance, while enhanced compatibility and error reporting make React 19 a game-changer in the world of web development.
Subscribe to my newsletter
Read articles from Ayush Kumar Tiwari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
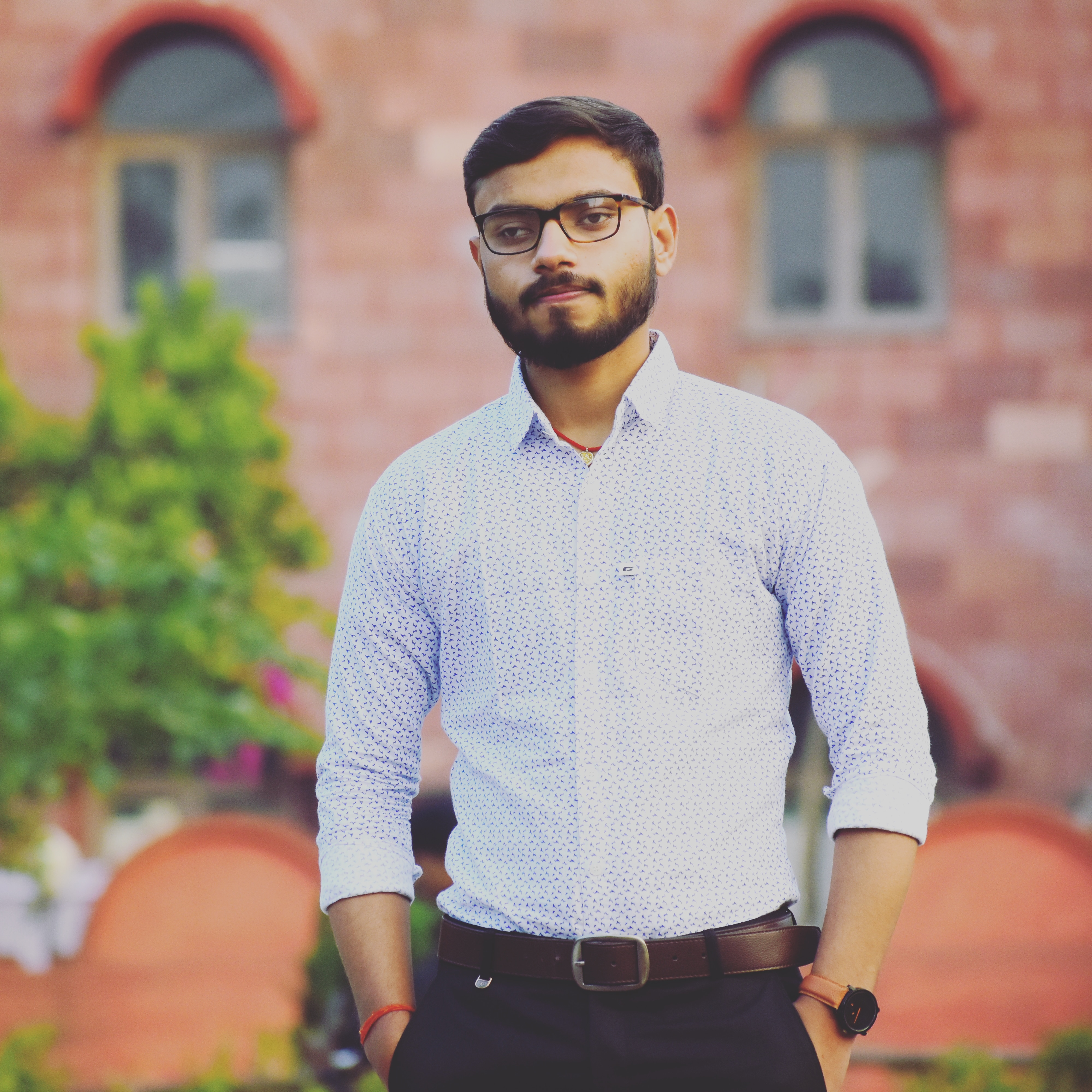