What is TypeScript?
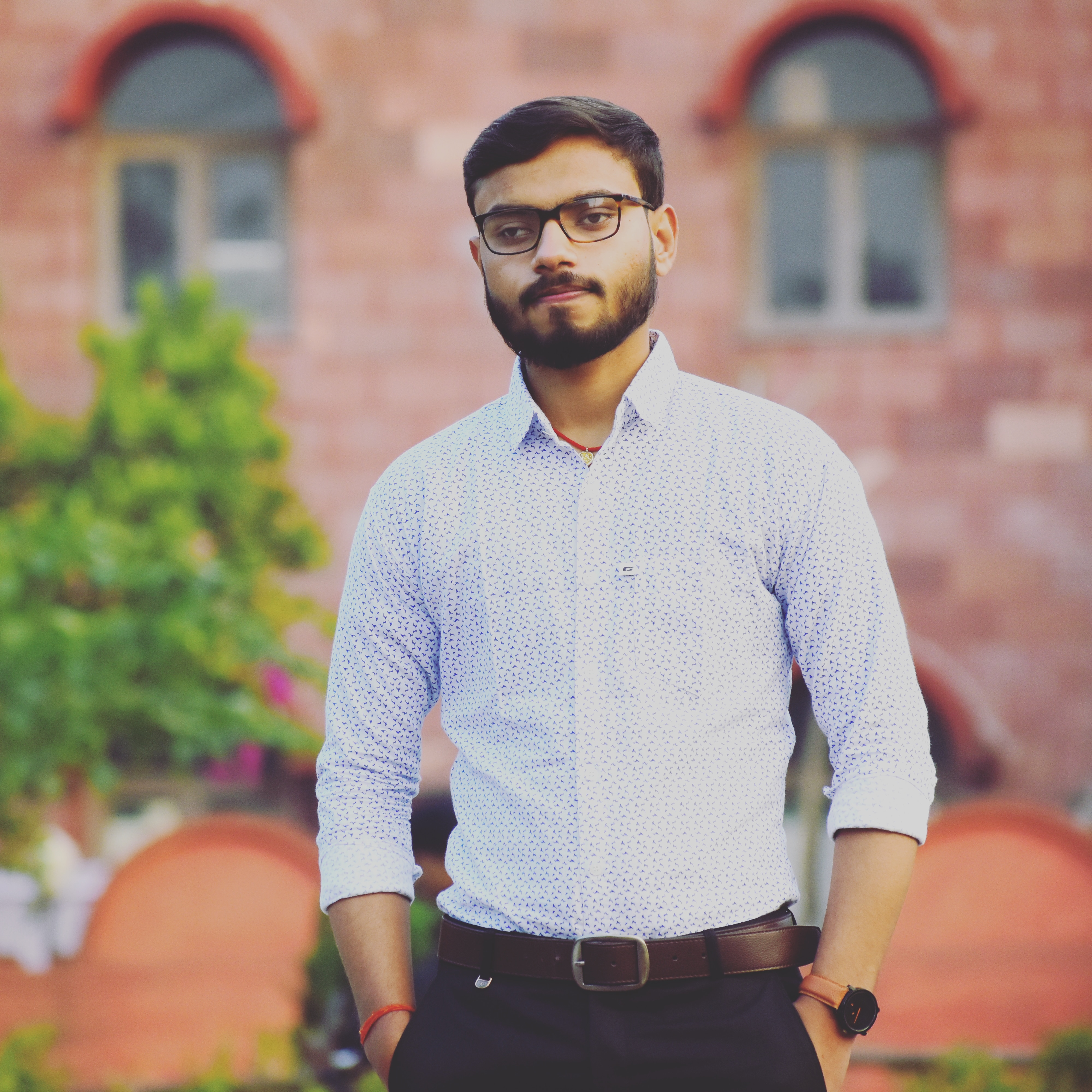
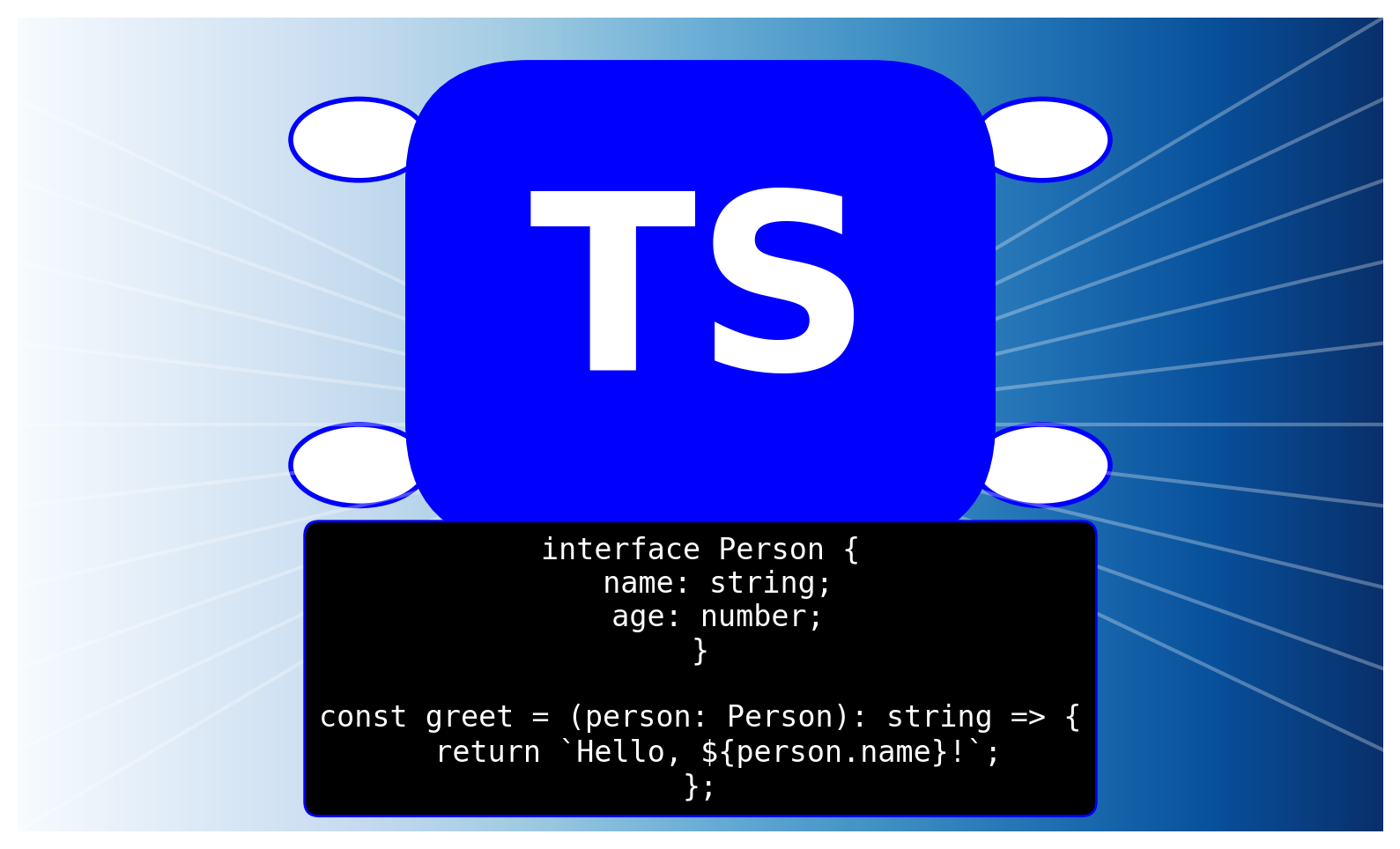
Overview
TypeScript is an object-oriented programming language developed by Microsoft in 2012. It is a superset of JavaScript, extending its functionality by adding data types, classes, and other object-oriented features along with type-checking capabilities. While JavaScript is ideal for small-scale applications, TypeScript is better suited for larger projects. It can be used to develop JavaScript applications for both server-side and client-side execution.
Understanding TypeScript
TypeScript can be likened to JavaScript, but with a stricter and more organized approach. If JavaScript is your easygoing companion, TypeScript is the disciplined soldier in your coding journey. While both are capable languages for web development, TypeScript adds a layer of structure and order, making it ideal for managing large-scale projects with confidence and efficiency. TypeScript programs include modules, functions, variables, comments, and expressions, much like any full-fledged programming language. TypeScript code is initially written and then compiled into plain JavaScript code using the TypeScript compiler. This compiled JavaScript can run in a browser. TypeScript files use the .ts
extension, in contrast to the .js
extension used by JavaScript files. The TypeScript code is converted to JavaScript using the tsc
or Babel compiler.
Example
To compile a TypeScript file named helloworld.ts
into JavaScript, you can use the following command:
$ tsc helloworld.ts
This will generate a helloworld.js
file.
Why Use TypeScript?
TypeScript is a strongly typed language backed by Microsoft, with an active developer community ensuring rapid evolution and frequent updates.
Benefits of TypeScript
Object-Oriented Programming Support: TypeScript supports classes, interfaces, inheritance, and other OOP concepts.
Compilation Error Checking: The TypeScript compiler identifies syntax errors during development.
Strong Static Typing: TypeScript’s static typing helps catch errors early in development.
Better Code Readability: Using classes and interfaces improves code organization, making it easier to maintain and debug.
Reliable: TypeScript catches type-related errors at compile time, ensuring more robust code.
Installing TypeScript Environment
To set up a TypeScript environment, follow these steps:
Install Node.js and npm: Download and install Node.js, which includes npm.
Install TypeScript Compiler: Open your terminal and run:
npm install -g typescript
- Verify Installation: Check the installation by running:
tsc --version
Now you can compile TypeScript files using the tsc
command.
Components of TypeScript
TypeScript is divided into three main layers, each containing several components:
Language
This includes TypeScript’s syntax, keywords, and type annotations.
TypeScript Compiler
The TypeScript compiler, named tsc
, converts TypeScript code into JavaScript. Browsers cannot directly execute TypeScript code, so it must be compiled to JavaScript first. The compiler can be installed locally using npm. Once installed, you can compile TypeScript files by running the tsc
command in the terminal.
Compiler Configuration
The TypeScript compiler is configured using the tsconfig.json
file. Below is an example configuration:
{
"compilerOptions": {
"declaration": true,
"emitDecoratorMetadata": false,
"experimentalDecorators": false,
"module": "none",
"moduleResolution": "node",
"noFallthroughCasesInSwitch": false,
"noImplicitAny": false,
"noImplicitReturns": false,
"removeComments": false,
"sourceMap": false,
"strictNullChecks": false,
"target": "es3"
},
"compileOnSave": true
}
TypeScript Language Services
These services provide useful information to editors and tools, enabling features like IntelliSense, automated refactoring, statement completion, signature help, code formatting, and outlining.
Addressing JavaScript’s Gaps
JavaScript is a loosely typed language, originally designed for client-side scripting. As JavaScript grew to include server-side programming with Node.js, it became more complex to manage. JavaScript lacks strong type checking, object orientation, and compile-time error checks, which are essential for robust server-side programming.
How TypeScript Fills These Gaps
TypeScript is a strongly typed superset of JavaScript, designed to fill the gaps and support large-scale application development. TypeScript standardizes projects, catches logical errors during development, and provides more confidence in code reliability.
Key Features of TypeScript
Compilation: TypeScript compiles code and identifies syntax errors during development, rather than at runtime.
Static Typing and Type Inference: TypeScript’s optional static typing and type inference system can deduce types, helping to eliminate type errors during compilation.
Enhanced IDE Support: TypeScript offers superior IDE support, improving the development experience with features like code completion and error highlighting.
Features of EcmaScript 6 (ES6)
ES6 introduced significant features, such as modules, classes, spread operators, and lambda functions. However, not all browsers support these features, so developers often use TypeScript to leverage them while ensuring compatibility with older environments.
Advantages and Disadvantages of TypeScript
Advantages
Runs on any browser or JavaScript engine after compilation.
Compatible with existing JavaScript libraries and frameworks.
Offers type checking at runtime.
Useful for large team collaborations.
Identifies compilation errors during development.
Easy to learn for JavaScript developers.
Syntax similar to backend languages like Java and Scala.
Disadvantages
Longer compilation times due to code conversion.
Lacks support for abstract classes.
Requires definition documents for external libraries.
Competitors
TypeScript’s main competitors are CoffeeScript and Dart. While CoffeeScript simplifies JavaScript, it lacks TypeScript’s static typing. Dart aims to replace JavaScript but struggles with interoperability. TypeScript excels in extending JavaScript with additional features and strong typing.
Conclusion
TypeScript enhances JavaScript with robust features for large-scale development. It addresses JavaScript’s shortcomings with strong typing, better IDE support, and compile-time error checking. With benefits like improved code readability and reliability, TypeScript is a powerful tool for modern web development.
Subscribe to my newsletter
Read articles from Ayush Kumar Tiwari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
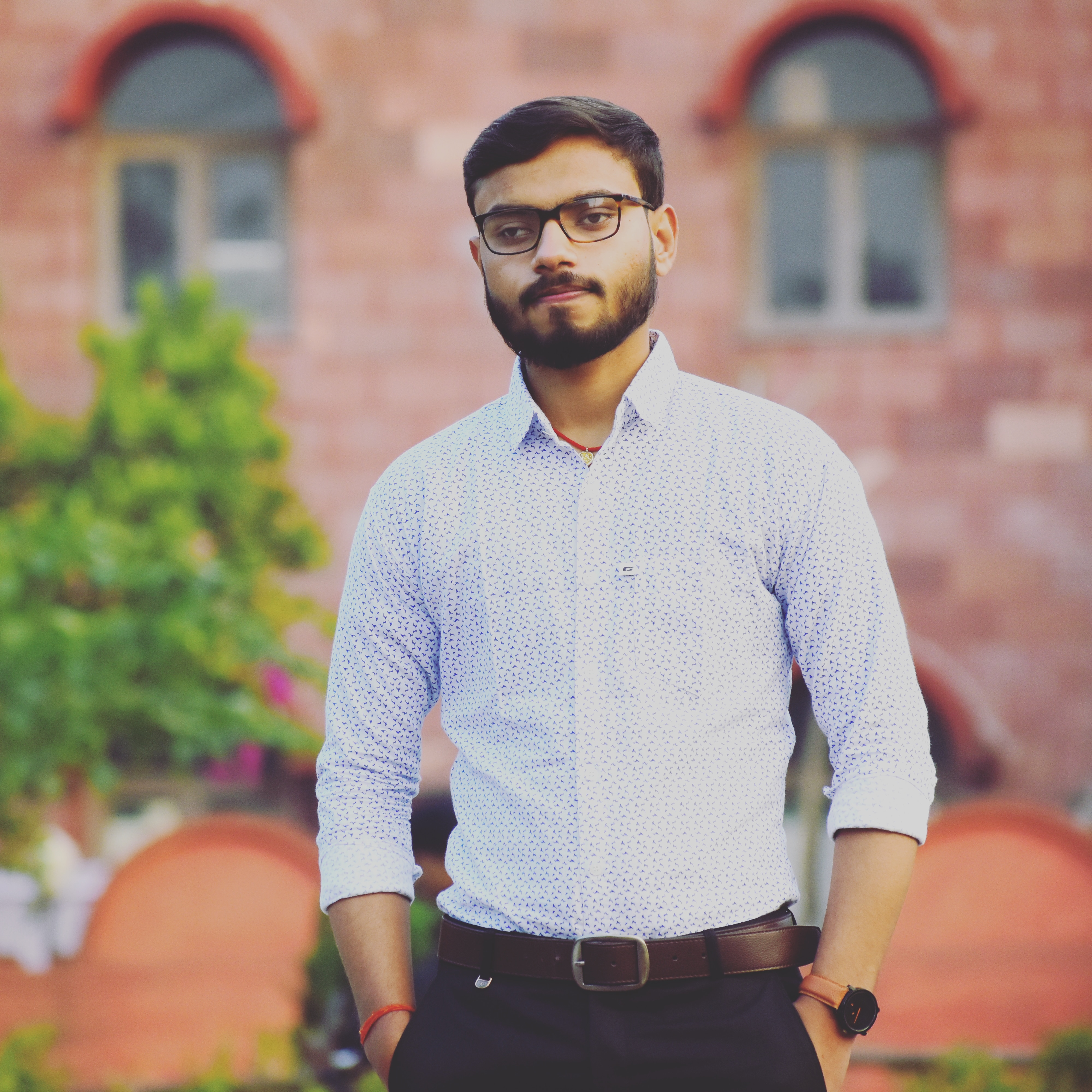