Avoiding unwrap() in Production Code: A Guide to Writing Robust Rust Applications

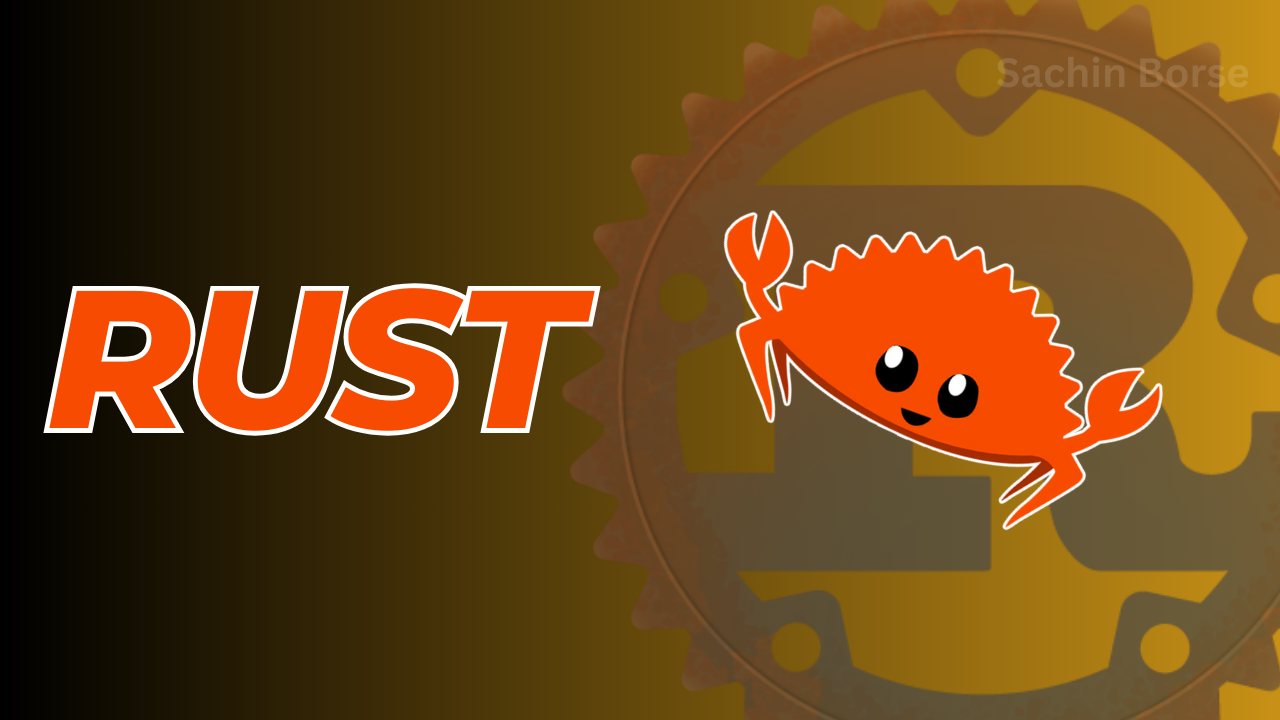
In the vast world of Rust programming, where safety and reliability are super important, there's a tricky function called unwrap()
. It might seem like a handy tool at first, but it can actually be a bit dangerous, especially in serious situations like when your app is live.
The Temptation of unwrap()
unwrap()
looks like a quick and easy way to get the good stuff out of an Option
or Result
without having to deal with any error messages. But here's the catch: if something unexpected happens, like if there's no value or if there's an error, unwrap()
makes your program freak out and crash.
Avoiding Crashes in Real Life
When your app is out there in the real world, crashing is not cool. It makes users sad, and it makes you look bad. Just one little unwrap()
in the wrong place can mess everything up.
Safer Ways to Handle Problems: match
, if let
, and ?
Thankfully, Rust gives us some safer ways to deal with errors. Instead of using unwrap()
, we can use things like match
, if let
, and the ?
symbol. These tricks help us handle errors without causing our app to blow up.
Using match
:
fn parse_number(input: &str) -> Result<i32, std::num::ParseIntError> {
match input.parse() {
Ok(num) => Ok(num),
Err(err) => Err(err),
}
}
Trying if let
:
fn parse_number(input: &str) -> Result<i32, std::num::ParseIntError> {
if let Ok(num) = input.parse() {
Ok(num)
} else {
Err(ParseIntError::new(ErrorKind::InvalidInput, "Invalid input"))
}
}
Using the ?
Symbol:
fn parse_number(input: &str) -> Result<i32, std::num::ParseIntError> {
let num = input.parse()?;
Ok(num)
}
Wrapping Up: Keeping Your Rust Code Strong
In the end, while unwrap()
might seem like a quick fix, it's not worth the risk in serious situations. By using safer methods like match
, if let
, and ?
, you can make sure your Rust apps stay strong and reliable, even when things get tough out there.
Subscribe to my newsletter
Read articles from Sachin Borse directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
