What is the main function in C++?

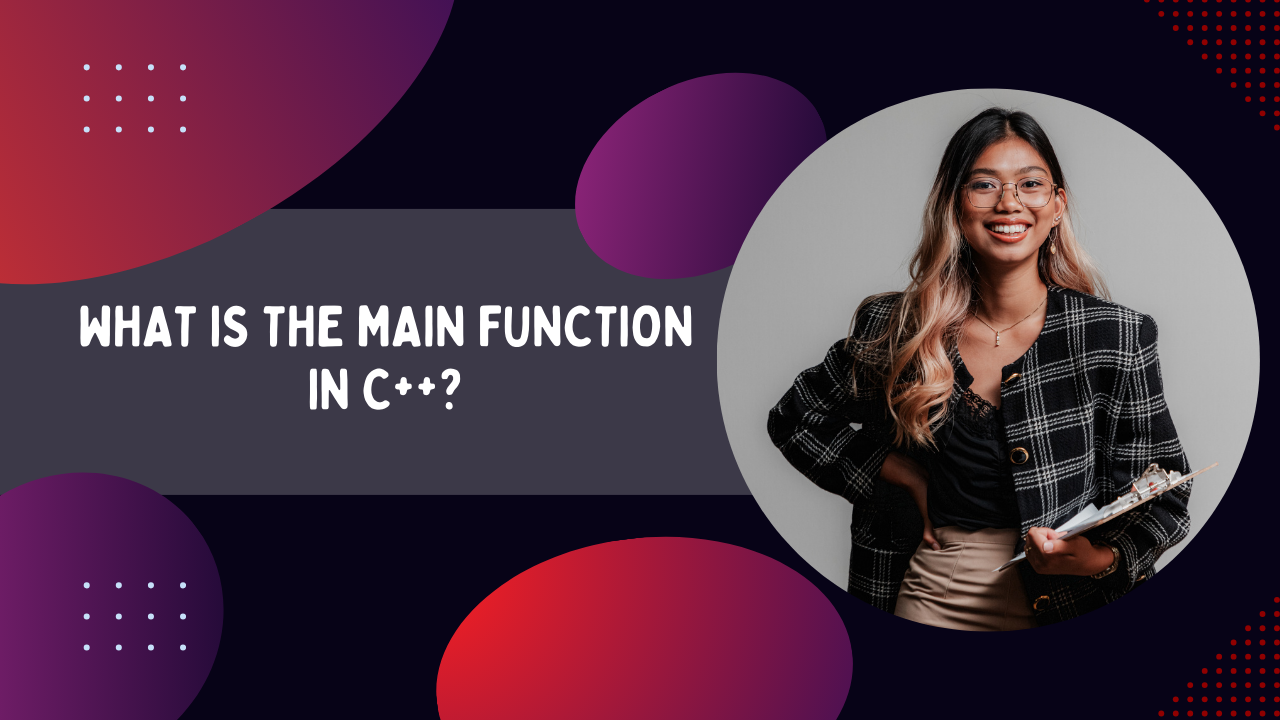
In the realm of programming languages, C++ stands tall as a powerhouse, revered for its versatility and efficiency. Central to the structure of any C++ program is the main function.
In this article, we delve into the intricacies of the main function, its significance, and how it operates within a C++ program. Additionally, we'll explore two fundamental concepts closely related to C++ programming: pointers and arrays.
What is the Main Function in C++?
In C++, the main function serves as the entry point for program execution. It is where the program begins its execution and where control is transferred after the program terminates. The main function has a specific signature:
int main() {
// Code statements
return 0;
}
Let's break down the components of the main function:
int: The return type of the main function is typically int, indicating that the function returns an integer value to the operating system upon termination. A return value of 0 conventionally indicates successful execution, while a non-zero value indicates an error.
main(): This is the function name. The parentheses indicate that it's a function, and the absence of parameters indicates that the main function doesn't accept any arguments.
Code statements: This is where the actual program logic resides. Here, you'll write the instructions that define the behavior of your program.
return 0: Finally, the return 0 statement signifies the end of the main function and indicates to the operating system that the program terminated successfully.
Pointers in C++
Pointers in C++ are the fundamental feature programming that allows you to work directly with memory addresses. A pointer is a variable that stores the memory address of another variable. It provides a way to access and manipulate data indirectly, by referencing the memory location where the data is stored.
Here's a basic example of a pointer declaration and usage:
int main() {
int num = 10;
int *ptr = # // Declaring a pointer and assigning the address of 'num'
cout << "Value of num: " << num << endl;
cout << "Address of num: " << &num << endl;
cout << "Value of num using pointer: " << *ptr << endl;
cout << "Address stored in pointer: " << ptr << endl;
return 0;
}
In this example, ptr is a pointer variable that stores the memory address of the variable num. By dereferencing the pointer using the * operator, we can access the value stored at that memory address.
C++ Arrays
C++ Arrays are another fundamental concept that allows you to store multiple elements of the same data type in contiguous memory locations. They provide a convenient way to work with collections of data, such as lists or sequences.
Here's a simple example of an array declaration and usage:
int main() {
int numbers[5] = {1, 2, 3, 4, 5}; // Declaring an array of integers
cout << "Elements of the array:" << endl;
for(int i = 0; i < 5; i++) {
cout << numbers[i] << " ";
}
cout << endl;
return 0;
}
In this example, we declare an array number with a size of 5 and initialize it with some values. We then use a for loop to iterate over the elements of the array and print them to the console.
Conclusion
The main function serves as the starting point for program execution in C++, while pointers and arrays are fundamental concepts that allow for efficient manipulation of data and memory. Understanding these concepts lays the foundation for mastering C++ programming and enables you to develop robust and efficient software solutions.
By grasping the intricacies of the main function, pointers, and arrays, you'll be well-equipped to tackle a wide range of programming challenges and unleash the full potential of C++.
Subscribe to my newsletter
Read articles from Anupam Srivastava directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
