Understanding STL Iterators in C++: Part 3
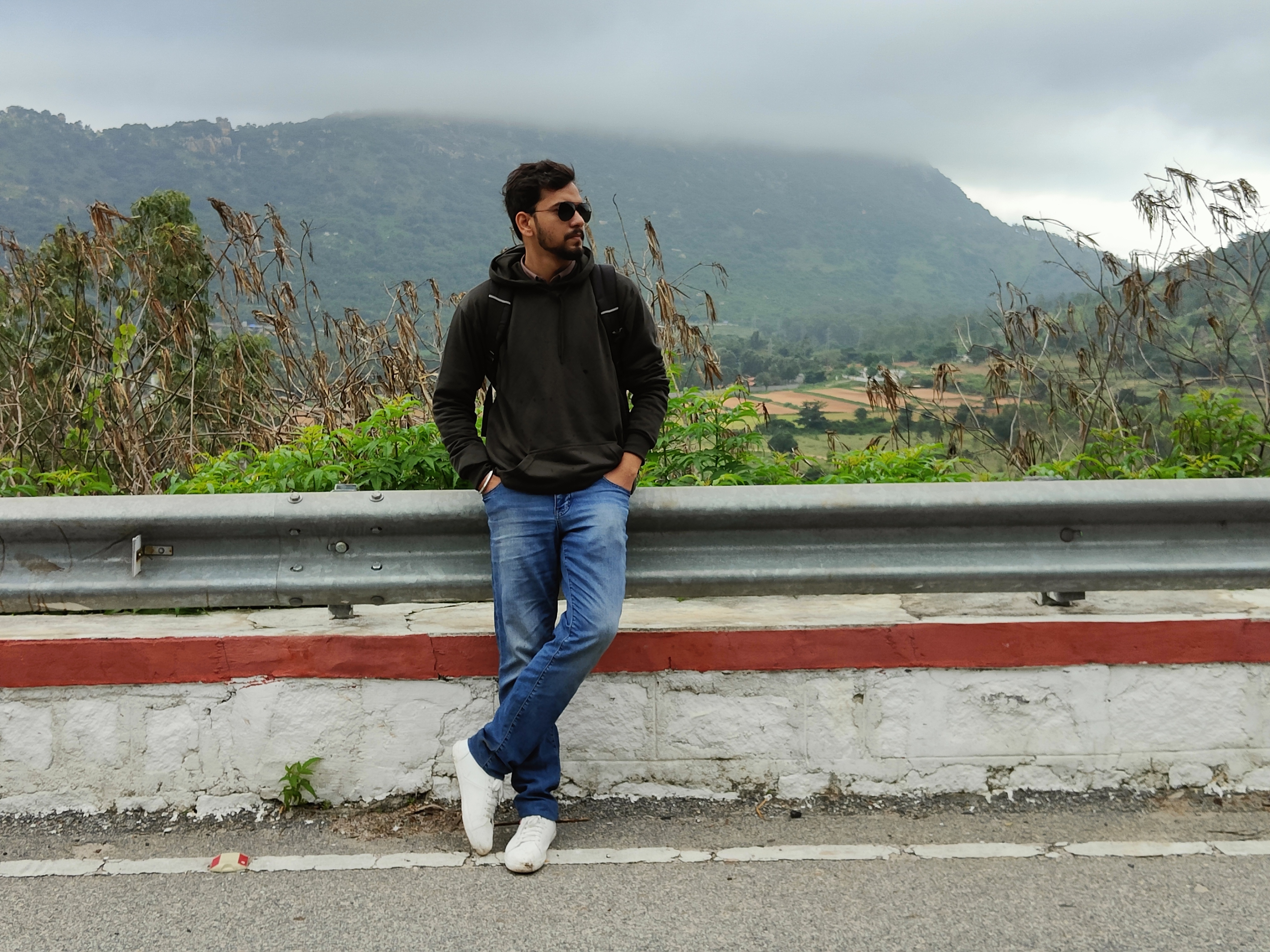
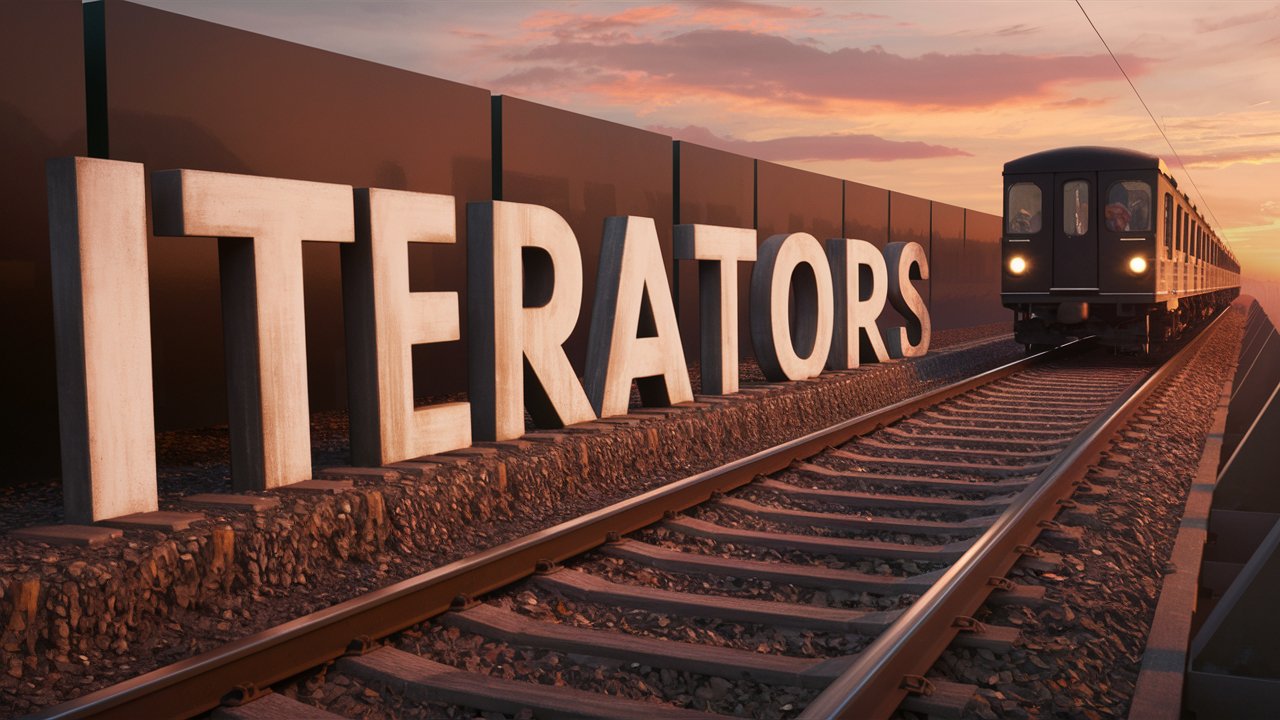
What are Iterators?
An Iterator is a pointer-like object representing an element's position in a container. It is used to iterate over elements in a container.
Suppose we have a vector named nums of size 4. Then, begin() and end() are member functions that return iterators pointing to the beginning and end of the vector respectively.
nums. begin(): points to the first element in the vector i.e., 0th index.
nums. begin() + i: points to the element at the ith index.
nums. end(): points to one element past the final element in the vector.
#include<iostream> #include<vector> using namespace std; int main() { //STL - Iterators vector<int> arr; arr.push_back(10); arr.push_back(20); arr.push_back(30); //traverse using iterator vector<int>::iterator it = arr.begin(); while (it != arr.end()) { cout << *it << " "; it++; } cout << endl; }
PS C:\Users\RISHABH KUMAR\OneDrive\Desktop\DSA\Iterators.cpp\output> & .\'iterator.exe' 10 20 30
Why use Iterators?
Working with algorithms: C++ has many ready-to-use algorithms like finding elements, sorting, and summing values. Iterators help you apply these algorithms to different types of data containers like arrays and lists.
Saving memory: Instead of loading a huge set of data all at once, iterators let you deal with one item at a time, which saves memory.
Uniform approach: Iterators allow you to interact with different kinds of data containers (like vectors or sets) in the same way. This makes your code more consistent and easier to manage.
Simpler Code: By using iterators, a lot of the repetitive details of going through data are taken care of, making your code cleaner and easier to read.
Iterator Operations:
*itr: Returns the element at the current position.
itr->m: Returns the member value m of the object pointed by the iterator and is equivalent to (*itr).m
++itr: Moves iterator to the next position
--itr: Moves the iterator to the previous position.
itr + i: Moves iterator by i positions
itr1 == itr2: returns true if the positions pointed by the iterator are the same.
itr1 != itr2: returns true if the positions pointed by the iterator are not the same
itr = itr1: Assigns the position pointed by itr1 to the itr iterator.
Iterator Types:
Input Iterator:
These Iterators can only be used for reading values from a container in a forward direction. They are typically used for algorithms that need to read data from a container, such as std::find or std::for_each.
//Create an input iterator to read values from cin istream_iterator<int> input_itr(cin);
Output Iterator:
These iterators can only be used for writing values to a container in a forward direction. They are less commonly used compared to other iterator types.
//create an output iterator to write integers to the console ostream_iterator<int> output_itr(cout, " ");
Forward Iterator:
These iterators combine the capabilities of both input and output iterators. They allow reading and writing values in a forward direction. Many container types, like lists, support forward iterators.
forward_list<in> nums{1,2,3,4}; //initialize an iterator to point //to the beginning of a forward list forward_list<int>::iterator it = nums.begin();
Bi-Directional Iterator:
These Iterators can move both forward and backward within a container. They are supported by containers like lists and double-ended queues (deques).
//initialize iterator to point the beginning of nums list<int>::iterator itr = nums.begin();
Random Access Iterator:
These Iterators offer full navigation capabilities, allowing you to move to any element within a container in constant time. Vectors, arrays, and deques provide random access iterators.
//create iterators to point to the first and the last elements vector<int>::iterator itr_first = vector.begin(); vector<int>::iterator itr_last = vector.end() - 1;
Also, read my blogs in this series: [https://devrishabh.hashnode.dev/]
Happy Coding!
Subscribe to my newsletter
Read articles from Rishabh Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
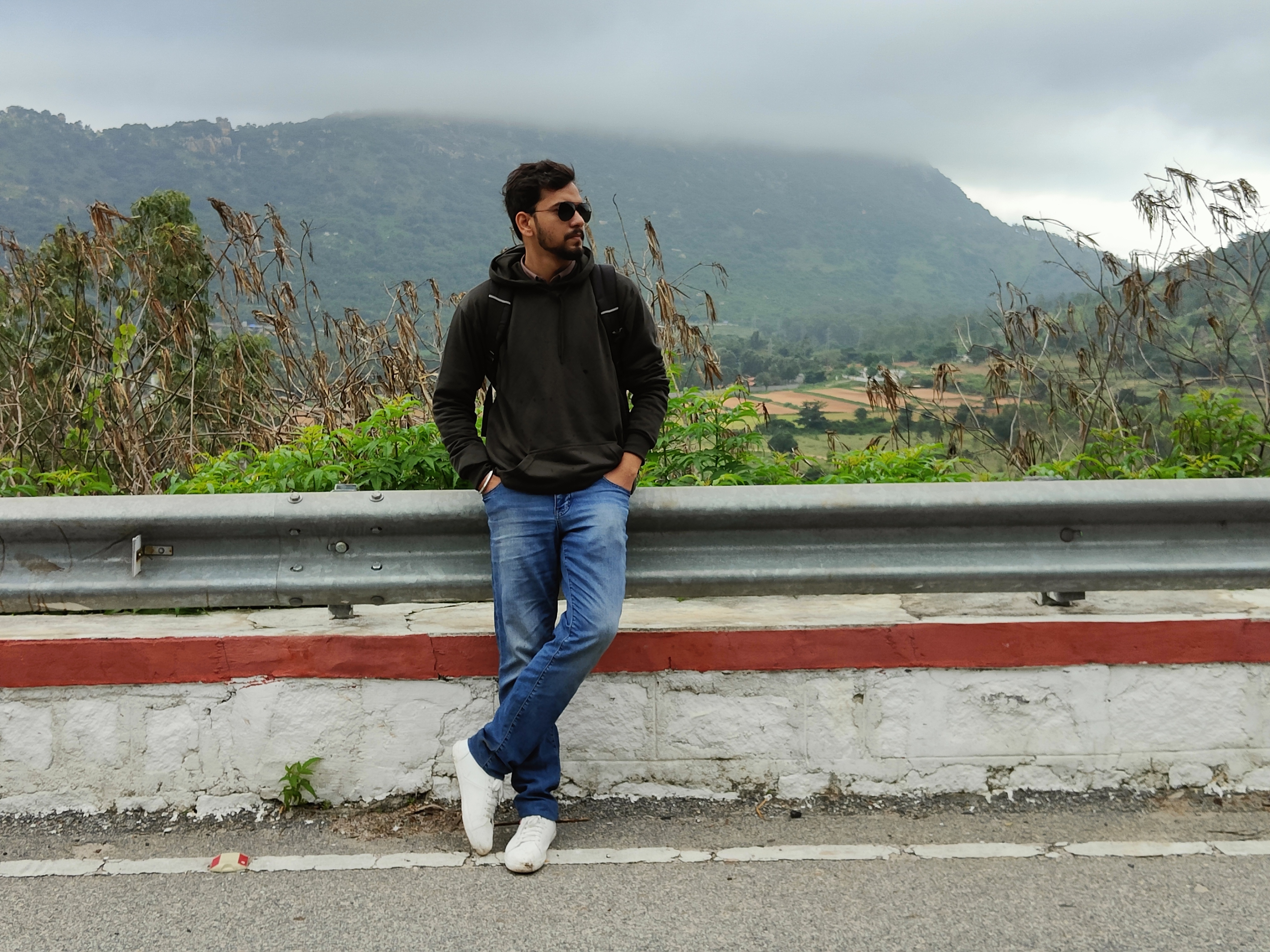