Optimizing Performance: JavaScript Slot Machine Code Optimization Strategies
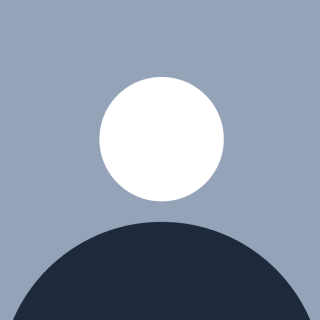
In the realm of web development, JavaScript performance optimization is crucial, especially when dealing with high-interactivity applications such as a Javascript slot machine code. Optimizing your JavaScript code can lead to a smoother user experience, reduced loading times, and improved performance across different devices and browsers. In this article, we will delve deep into advanced strategies for optimizing JavaScript code used in slot machine applications, ensuring your code runs efficiently and effectively.
Understanding the Basics of JavaScript Optimization
Before diving into advanced optimization techniques, it’s essential to understand the fundamentals of JavaScript performance. JavaScript, as a high-level, interpreted language, can sometimes introduce performance bottlenecks if not carefully managed. Key factors influencing JavaScript performance include memory management, execution context, asynchronous operations, and rendering processes.
1. Efficient Memory Management
Memory management is a cornerstone of JavaScript performance. Slot machine applications often involve numerous objects and animations, which can lead to significant memory consumption. Here’s how to manage memory effectively:
Garbage Collection Awareness: JavaScript uses an automatic memory management system known as garbage collection. However, understanding how and when garbage collection occurs can help you write more efficient code. Avoid creating unnecessary objects within loops or frequently called functions to reduce memory overhead.
Object Pooling: Reuse objects instead of creating new ones. For instance, in a slot machine game, reuse slot objects or animations to minimize memory allocation and deallocation costs.
Avoid Memory Leaks: Ensure that all event listeners are removed when they are no longer needed, and avoid retaining references to DOM elements or objects that are no longer in use.
2. Minimizing the Execution Context
JavaScript's execution context plays a critical role in performance. Every time a function is called, a new execution context is created. Reducing the number of context creations can significantly boost performance.
Function Inlining: Instead of calling small functions repeatedly, inline their logic where applicable. This reduces the overhead of context creation and improves execution speed.
Scope Reduction: Minimize the scope chain traversal by keeping the execution context as shallow as possible. Accessing variables in the global or parent scopes is slower than accessing local variables.
Closures Optimization: While closures are powerful, they can inadvertently cause performance issues if not used judiciously. Avoid excessive use of closures in performance-critical parts of your code.
3. Optimizing Asynchronous Operations
Asynchronous operations, such as animations and network requests, are integral to slot machine games. Optimizing these can lead to smoother gameplay and a better user experience.
Debouncing and Throttling: Use debouncing and throttling techniques to limit the rate at which your functions are executed, particularly in response to user interactions like clicks or scrolls.
Promises and Async/Await: Make use of modern asynchronous patterns like Promises and async/await to manage asynchronous operations more efficiently. These patterns can help avoid callback hell and improve the readability and maintainability of your code.
Web Workers: Offload heavy computations or non-UI tasks to Web Workers to keep the main thread responsive. This is particularly useful in slot machine games where complex calculations might be needed.
4. Enhancing Rendering Performance
Rendering performance is crucial in slot machine games where smooth animations and quick updates are essential.
Minimize Reflows and Repaints: Changes to the DOM can trigger reflows and repaints, which are costly operations. Batch DOM updates together and minimize direct manipulation of the DOM to improve rendering performance.
Use CSS for Animations: Wherever possible, use CSS animations instead of JavaScript-driven animations. CSS animations are typically more efficient as they can take advantage of GPU acceleration.
RequestAnimationFrame: For smoother animations, use the
requestAnimationFrame
method instead ofsetTimeout
orsetInterval
. This method synchronizes with the browser's refresh rate, resulting in more efficient rendering.
Advanced Techniques for JavaScript Optimization
For those looking to push the boundaries of JavaScript performance, especially in complex applications like slot machines, advanced optimization techniques can make a significant difference.
1. Code Splitting and Lazy Loading
Code Splitting: Divide your JavaScript code into smaller chunks that can be loaded on demand. This is particularly beneficial for large applications where not all code is needed immediately. Tools like Webpack can help automate code splitting.
Lazy Loading: Defer the loading of non-critical resources until they are actually needed. For example, in a slot machine game, you can lazy-load additional themes or features that aren’t required during the initial game load.
2. Minification and Compression
JavaScript Minification: Use tools like UglifyJS or Terser to remove whitespace, comments, and other unnecessary characters from your code, reducing its size and improving load times.
Gzip Compression: Enable Gzip compression on your server to reduce the size of JavaScript files sent over the network, improving download speed and reducing bandwidth usage.
3. Leveraging Modern JavaScript Features
ES6 and Beyond: Modern JavaScript (ES6+) includes many features that can improve performance, such as arrow functions, destructuring, and spread operators. Utilizing these features can lead to cleaner and more efficient code.
WebAssembly: For compute-intensive tasks, consider using WebAssembly. This binary instruction format is designed to be a compilation target for languages like C, C++, and Rust, allowing you to execute code at near-native speed.
4. Using Efficient Data Structures and Algorithms
Optimize Data Structures: Choose the right data structures for your application. For instance, use typed arrays for numerical data or Maps and Sets for better performance in lookup operations.
Algorithm Optimization: Review and optimize your algorithms for performance. Ensure that you are using the most efficient algorithms for sorting, searching, and other operations.
Monitoring and Debugging JavaScript Performance
Even the most optimized code can benefit from continuous monitoring and debugging. Here’s how to keep your slot machine application performing at its best:
1. Performance Profiling
Browser Developer Tools: Use tools like Chrome DevTools to profile your JavaScript performance. Analyze the execution time of functions, memory usage, and other performance metrics.
Lighthouse Audits: Google Lighthouse provides automated audits for performance, accessibility, and best practices. Use it to identify and address performance bottlenecks in your slot machine application.
2. Error Tracking and Logging
Real-time Error Tracking: Implement real-time error tracking using tools like Sentry or Rollbar to capture and analyze errors as they occur. This helps in quickly identifying performance issues that users might encounter.
Comprehensive Logging: Maintain detailed logs of application events and performance metrics. This can help in diagnosing issues and understanding the performance impact of different parts of your code.
3. Continuous Integration and Deployment
Automated Testing: Incorporate automated testing into your development workflow to catch performance issues early. Tools like Jest or Mocha can be used for unit testing, while tools like Cypress or Selenium can help with end-to-end testing.
Performance Budgets: Define performance budgets to ensure that your code stays within acceptable limits for load times, memory usage, and other critical metrics.
Conclusion
Optimizing JavaScript code for slot machine games requires a multifaceted approach. By focusing on memory management, execution context minimization, efficient asynchronous operations, and enhanced rendering performance, you can significantly improve the performance of your slot machine application. Additionally, leveraging advanced techniques such as code splitting, modern JavaScript features, and efficient data structures will further refine your application’s performance.
Subscribe to my newsletter
Read articles from Charlie Miller directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Charlie Miller
Charlie Miller
We Provide stands as a prominent worldwide provider of IT solutions, extending a range of services encompassing web and mobile app development, game development, and digital marketing. Backed by a proficient team and a bwell-established history of dispensing top-tier projects, we cater comprehensively to businesses of all magnitudes. Our inventive resolutions are meticulously fashioned to align with your distinct requisites, propelling you towards the accomplishment of your digital ambitions with precision. Embark on a journey through technology's prowess with unveil novel prospects for your enterprise.