Building a Random Quote Generator Using HTML, CSS, and JavaScript

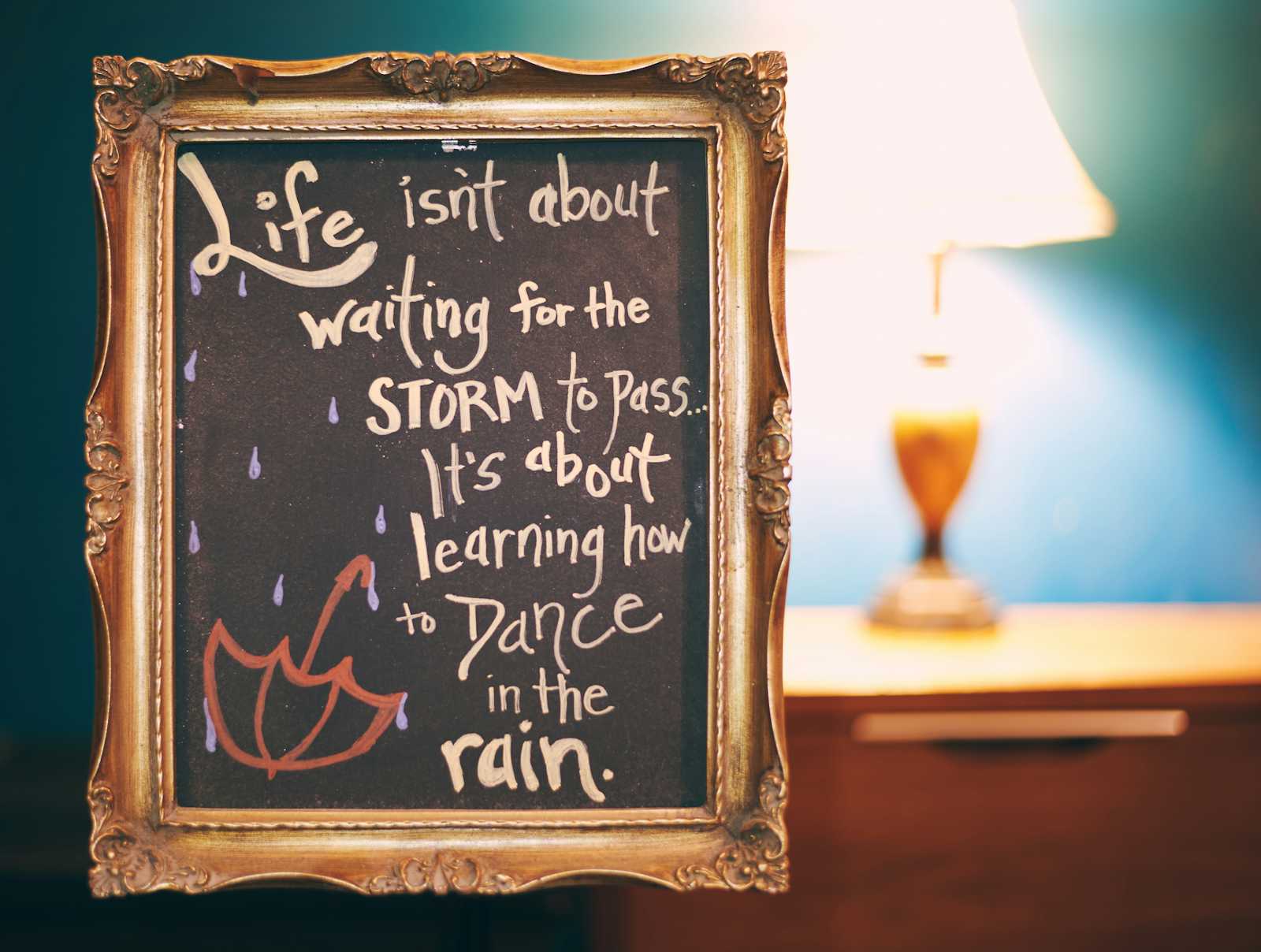
In this tutorial, we'll create a simple yet effective Random Quote Generator using HTML, CSS, and JavaScript. This project will randomly display quotes from an array when a button is clicked. Let's dive into the steps:
Step 1: Creating the HTML Structure
htmlCopy code<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Random Quote Generator</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="container">
<h1>Random Quote Generator</h1>
<div class="quote-container">
<h2>Quote of the Day</h2>
<div class="quote">
<span id="quoteText"></span>
</div>
<button id="generateButton">New Quote</button>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
Step 2: Styling with CSS
cssCopy code/* styles.css */
body {
font-family: Arial, sans-serif;
background-color: #f0f0f0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
}
.container {
text-align: center;
}
.quote-container {
background-color: #fff;
padding: 20px;
border-radius: 10px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
max-width: 600px;
margin: 0 auto;
}
.quote {
font-size: 24px;
margin: 20px 0;
}
#generateButton {
background-color: #007bff;
color: #fff;
border: none;
padding: 10px 20px;
font-size: 16px;
cursor: pointer;
border-radius: 5px;
}
#generateButton:hover {
background-color: #0056b3;
}
Step 3: Implementing the JavaScript Logic
javascriptCopy code// script.js
const quotes = [
"Live in the moment..!!",
"Do more of what you love..!!",
"You are perfect just the way you are :)",
"Live what you love <3",
// Add more quotes as needed
];
function generateRandomQuote() {
const randomIndex = Math.floor(Math.random() * quotes.length);
const quote = quotes[randomIndex];
document.getElementById("quoteText").textContent = quote;
}
document.getElementById("generateButton").addEventListener("click", generateRandomQuote);
generateRandomQuote();
Summary
In this tutorial, we've created a Random Quote Generator using HTML for structure, CSS for styling, and JavaScript for functionality. The project uses an array of quotes and generates a random quote each time the "New Quote" button is clicked.
Feel free to customize the design, add more quotes to the array, or enhance the functionality further based on your preferences. Have fun coding and exploring the world of web development!
Subscribe to my newsletter
Read articles from Shreya Berlikar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
