Singleton Pattern in Java

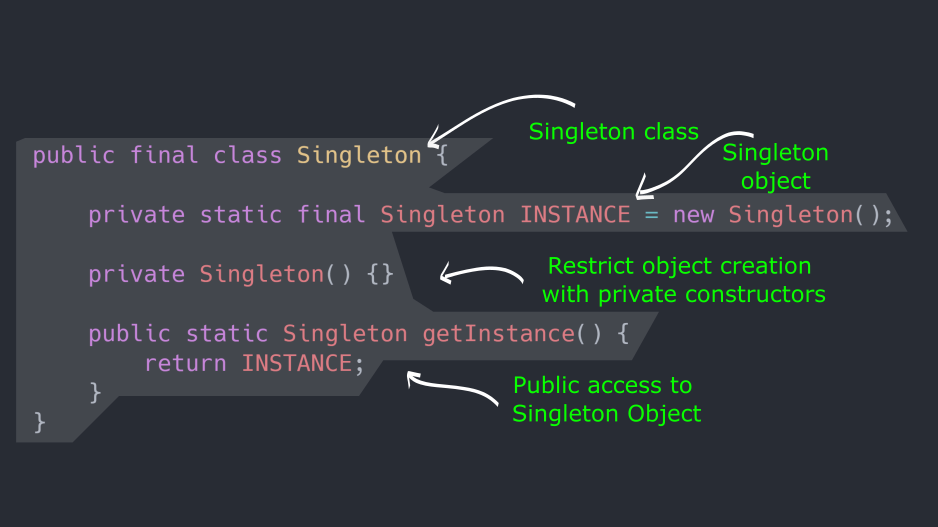
In software design, certain scenarios demand a single, globally accessible instance of a class. Whether it's managing a configuration, maintaining a connection pool, or logging activities, the Singleton design pattern in Java can be the perfect solution. This blog delves into the intricacies of the Singleton pattern, its implementations, and its applications in Java.
What is a Singleton Class?
A Singleton class ensures that a class has only one instance and provides a global point of access to that instance. This design pattern is particularly useful when exactly one object is needed to coordinate actions across the system.
Key Characteristics:
Single Instance: Only one instance of the class is created throughout the application's lifecycle.
Global Access: The instance is accessible globally, ensuring consistent behavior.
Implementing Singleton Pattern
There are several ways to implement a Singleton in Java, each with unique characteristics, advantages, and trade-offs.
1. Eager Initialization
In eager initialization, the instance is created at the time of class loading. This method is straightforward but may lead to resource wastage if the instance is never used.
public class Singleton {
private static final Singleton instance = new Singleton();
private Singleton() {
// Private constructor to prevent instantiation
}
public static Singleton getInstance() {
return instance;
}
}
2. Lazy Initialization
Lazy initialization defers the creation of the instance until it is needed. This approach conserves resources but is not thread-safe.
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
3. Thread-Safe Singleton (Synchronized Method)
To ensure thread safety in a multi-threaded environment, synchronization can be used. However, this approach can lead to performance overhead due to the cost of synchronization.
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static synchronized Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
4. Double-Checked Locking
Double-checked locking minimizes the performance overhead of synchronization by checking the instance twice. This method is more efficient than a fully synchronized method.
public class Singleton {
private static volatile Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
synchronized (Singleton.class) {
if (instance == null) {
instance = new Singleton();
}
}
}
return instance;
}
}
5. Bill Pugh Singleton Implementation (Initialization-on-demand holder idiom)
This implementation leverages the Java class loader mechanism to ensure thread-safe, lazy initialization without synchronization overhead.
public class Singleton {
private Singleton() {}
private static class SingletonHelper {
private static final Singleton INSTANCE = new Singleton();
}
public static Singleton getInstance() {
return SingletonHelper.INSTANCE;
}
}
When to Use Singleton Pattern?
The Singleton pattern is suitable for scenarios such as:
Logging: A single logger instance can be used to log messages across different parts of the application.
Configuration Management: One instance to manage application configurations ensures consistency.
Connection Pools: Managing database connections through a single instance to reuse connections.
Caching: A single instance to manage cached data for efficient data retrieval.
Advantages of Singleton Pattern
Controlled Access: Singleton ensures that there is controlled access to the sole instance.
Memory Efficiency: Reduces memory footprint by limiting the number of instances.
Global Access Point: Provides a global access point to the instance.
Disadvantages of Singleton Pattern
Global State: Can make unit testing difficult due to the presence of global state.
Multithreading Issues: Improper implementation in a multithreaded environment can lead to issues.
Tight Coupling: May lead to tighter coupling, making the system harder to modify and extend.
Conclusion
The Singleton pattern is a powerful tool in Java, ensuring a single instance of a class with global access. While it's beneficial in many scenarios, it's crucial to implement it correctly, especially in a multi-threaded environment, to avoid potential pitfalls. Understanding and leveraging the different ways to implement Singleton can significantly enhance the efficiency and maintainability of your Java applications.
Use the Singleton pattern judiciously and reap the benefits of a well-coordinated, resource-efficient application. Happy coding!
Subscribe to my newsletter
Read articles from Riyaz Nabiyulla directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Riyaz Nabiyulla
Riyaz Nabiyulla
Tech enthusiast and full-time worker ๐ Learning DSA in Java to unlock new coding adventures! โ Passionate about solving puzzles and embracing continuous learning. Let's connect and build a brighter tech future together!