How I Built a Real-Time Chat App in 24 Hours Using React and Firebase

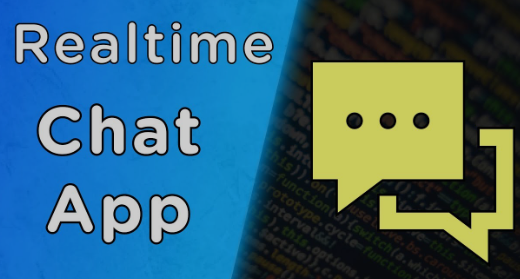
Introduction
Ever wondered how fast you can build a fully functional chat app? I took on the challenge to build one in just 24 hours using React and Firebase. In this article, I'll walk you through my journey, the obstacles I faced, and how I overcame them. Whether you're a seasoned developer or just starting, you'll find valuable insights and practical tips.
The Challenge
Setting the Stage
It all began on a Friday evening. With a weekend ahead and a burning desire to create something cool, I decided to build a real-time chat app. The goal was to complete it in 24 hours, using React for the frontend and Firebase for the backend.
Planning and Preparation
Tools and Technologies
Before diving in, I outlined the tools and technologies I’d use:
React: For building the user interface.
Firebase: For real-time database and authentication.
CSS Modules: For styling.
VS Code: As my code editor.
GitHub: To keep track of my code.
Initial Setup
Create React App:
I started by setting up the React project.
npx create-react-app real-time-chat-app
cd real-time-chat-app
Install Firebase:
Next, I installed Firebase to integrate it with my React app.
npm install firebase
Building the App
Step 1: Setting Up Firebase
I created a new Firebase project and set up the real-time database and authentication.
Create Firebase Project:
Go to the Firebase Console.
Click on "Add Project" and follow the setup instructions.
Add Firebase to Your App:
Register your app in the Firebase console.
Copy the Firebase config object and add it to your React project.
// src/firebase.js
import firebase from 'firebase/app';
import 'firebase/auth';
import 'firebase/firestore';
const firebaseConfig = {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_PROJECT_ID.firebaseapp.com",
projectId: "YOUR_PROJECT_ID",
storageBucket: "YOUR_PROJECT_ID.appspot.com",
messagingSenderId: "YOUR_MESSAGING_SENDER_ID",
appId: "YOUR_APP_ID"
};
firebase.initializeApp(firebaseConfig);
export const auth = firebase.auth();
export const firestore = firebase.firestore();
Step 2: Authentication
Setting up authentication using Firebase Authentication.
- Email and Password Authentication:
Enable Email/Password authentication in the Firebase Console.
Create signup and login components in React.
// src/components/Signup.js
import React, { useState } from 'react';
import { auth } from '../firebase';
const Signup = () => {
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const handleSubmit = async (e) => {
e.preventDefault();
try {
await auth.createUserWithEmailAndPassword(email, password);
} catch (error) {
console.error("Error signing up: ", error);
}
};
return (
<form onSubmit={handleSubmit}>
<input type="email" value={email} onChange={(e) => setEmail(e.target.value)} placeholder="Email" />
<input type="password" value={password} onChange={(e) => setPassword(e.target.value)} placeholder="Password" />
<button type="submit">Sign Up</button>
</form>
);
};
export default Signup;
Step 3: Real-Time Database
Configuring Firestore for real-time chat functionality.
Setting Up Firestore:
Enable Firestore in the Firebase Console.
Create a collection for messages.
// src/components/ChatRoom.js
import React, { useState, useEffect } from 'react';
import { firestore } from '../firebase';
const ChatRoom = () => {
const [messages, setMessages] = useState([]);
const [newMessage, setNewMessage] = useState('');
useEffect(() => {
const unsubscribe = firestore.collection('messages')
.orderBy('createdAt')
.onSnapshot(snapshot => {
const messages = snapshot.docs.map(doc => ({ id: doc.id, ...doc.data() }));
setMessages(messages);
});
return () => unsubscribe();
}, []);
const handleSendMessage = async (e) => {
e.preventDefault();
await firestore.collection('messages').add({
text: newMessage,
createdAt: firebase.firestore.FieldValue.serverTimestamp()
});
setNewMessage('');
};
return (
<div>
<div>
{messages.map(message => (
<div key={message.id}>
<p>{message.text}</p>
</div>
))}
</div>
<form onSubmit={handleSendMessage}>
<input value={newMessage} onChange={(e) => setNewMessage(e.target.value)} placeholder="Type a message" />
<button type="submit">Send</button>
</form>
</div>
);
};
export default ChatRoom;
Key Learnings
Challenges Faced
Time Management: Managing my time effectively was crucial. I divided the project into manageable chunks and set small deadlines.
Firebase Quirks: Encountered some unexpected behavior with Firestore which required digging into the documentation.
Tips for Success
Plan Ahead: Having a clear plan saved me a lot of time.
Stay Focused: Avoiding distractions helped me maintain momentum.
Utilize Resources: Stack Overflow and Firebase documentation were my best friends during this challenge.
Conclusion
Building a real-time chat app in 24 hours was a thrilling experience. It taught me a lot about rapid development and the power of React and Firebase. If you're up for a challenge, give it a try! I'd love to hear about your experiences and any improvements you make.
Call to Action: Have you built a similar app? What challenges did you face? Share your thoughts and experiences in the comments below!
Subscribe to my newsletter
Read articles from HEMANT directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

HEMANT
HEMANT
Hi 👋 this is ❤️🔥Hemant Katta💝, an 🛰️Electronics & Communication Engineer📡 🎓 graduate 2023 🎓.