Day 69 - Meta-Arguments in Terraform ๐
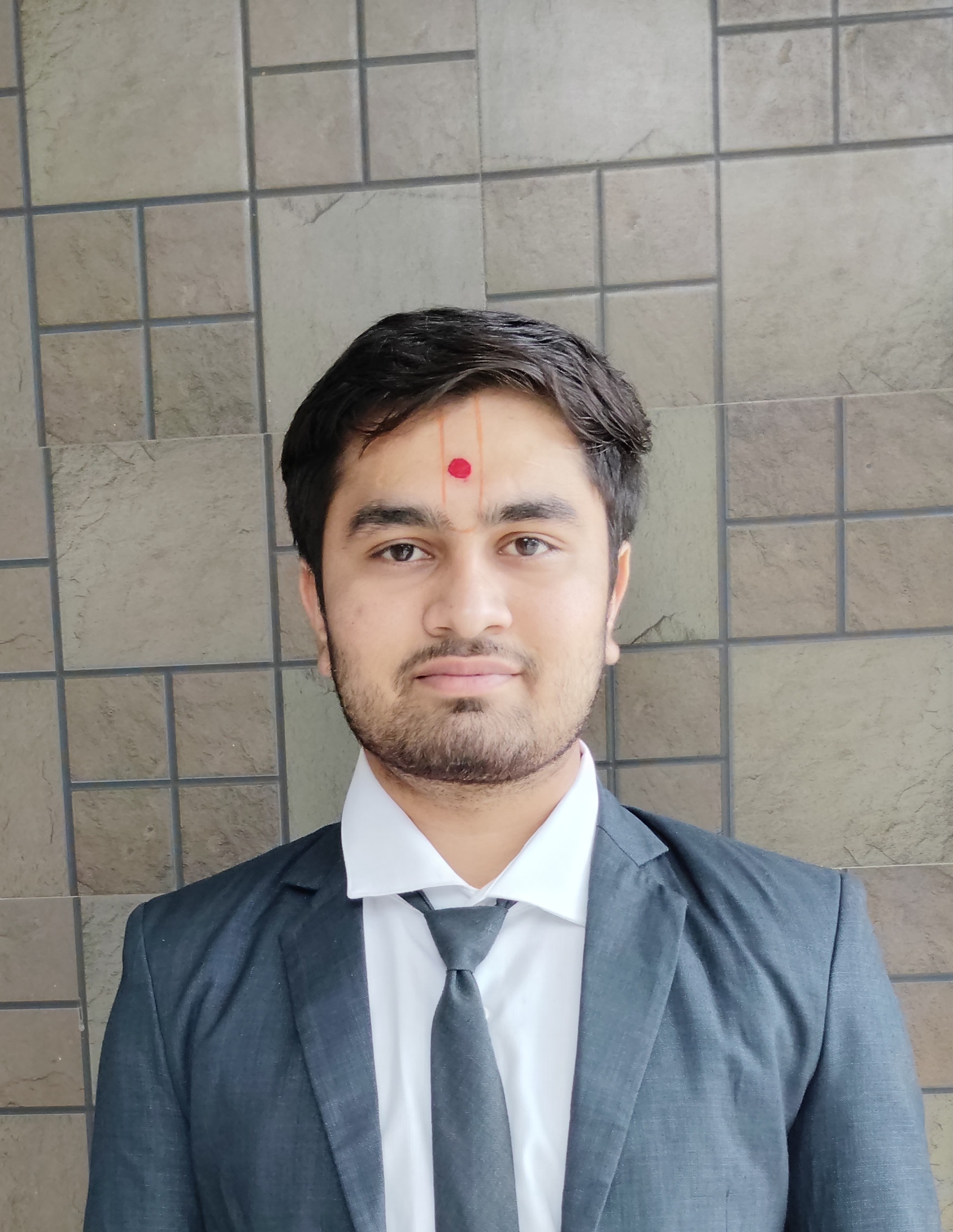
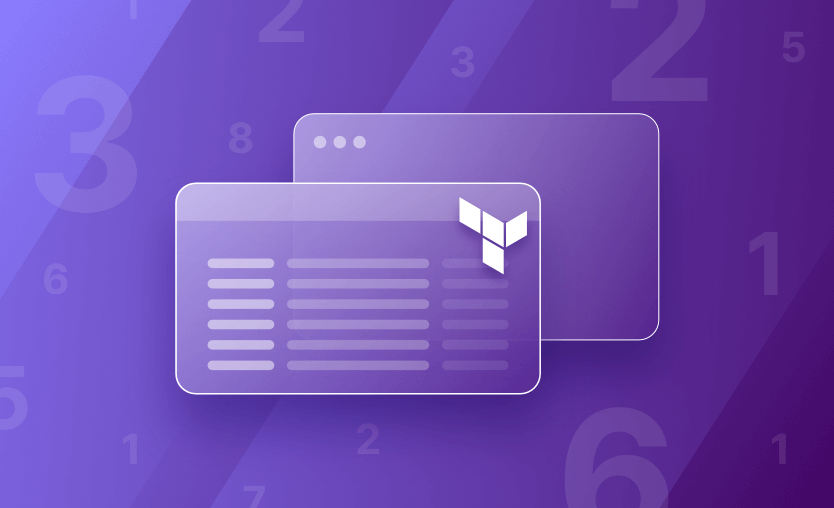
Welcome to Day 69 of the #90DaysOfDevOpsChallenge! ๐ Today, we dive into Meta-Arguments in Terraform. Meta-arguments like count
and for_each
are powerful tools that can help you manage multiple resources efficiently. Let's break down these concepts and walk through a hands-on example on AWS. ๐
Understanding Meta-Arguments in Terraform ๐ ๏ธ
When you define a resource block in Terraform, it specifies one resource by default. To manage several of the same resources without writing multiple blocks, you can use meta-arguments like count
and for_each
. These meta-arguments streamline your code and reduce overhead. ๐
Install Terraform:
sudo apt-get update
sudo apt-get install -y gnupg software-properties-common curl
curl -fsSL https://apt.releases.hashicorp.com/gpg | sudo apt-key add -
sudo apt-add-repository "deb [arch=amd64] https://apt.releases.hashicorp.com $(lsb_release -cs) main"
sudo apt-get update
sudo apt-get install terraform
terraform -version
Install AWS CLI:
sudo apt-get update
sudo snap install aws-cli --classic
aws --version
aws configure
Count ๐งฎ
The count
meta-argument accepts a whole number and creates that many instances of the specified resource. Each instance is managed independently. This is helpful for creating multiple identical resources. ๐ก
Example
terraform {
required_providers {
aws = {
source = "hashicorp/aws"
version = "~> 4.16"
}
}
required_version = ">= 1.2.0"
}
provider "aws" {
region = "us-east-1"
}
resource "aws_instance" "server" {
count = 4
ami = "ami-08c40ec9ead489470"
instance_type = "t2.micro"
tags = {
Name = "Server ${count.index}"
}
}
Initialize Terraform ๐
terraform init
Validate the Configuration โ
terraform validate
Apply the Configuration โก
terraform apply
Confirm with
yes
when prompted. ๐
For_Each ๐
The for_each
meta-argument is used when you need to create multiple resources with different values. Instead of a number, it accepts a map or a set of strings, iterating over each key-value pair. ๐
Example with Set of Strings
terraform {
required_providers {
aws = {
source = "hashicorp/aws"
version = "~> 4.16"
}
}
required_version = ">= 1.2.0"
}
provider "aws" {
region = "us-east-1"
}
locals {
ami_ids = toset([
"ami-08a0d1e16fc3f61ea",
"ami-04b70fa74e45c3917",
])
}
resource "aws_instance" "server" {
for_each = local.ami_ids
ami = each.key
instance_type = "t2.micro"
tags = {
Name = "Server ${each.key}"
}
}
Example with Map
locals {
ami_ids = {
"linux" : "ami-08a0d1e16fc3f61ea",
"ubuntu": "ami-04b70fa74e45c3917",
}
}
resource "aws_instance" "server" {
for_each = local.ami_ids
ami = each.value
instance_type = "t2.micro"
tags = {
Name = "Server ${each.key}"
}
}
for_each.tf
File
terraform {
required_providers {
aws = {
source = "hashicorp/aws"
version = "~> 4.16"
}
}
required_version = ">= 1.2.0"
}
provider "aws" {
region = "us-east-1"
}
# Define a local variable for count example
locals {
ami_ids_set = toset([
"ami-08a0d1e16fc3f61ea",
"ami-04b70fa74e45c3917",
])
}
resource "aws_instance" "server_set" {
for_each = local.ami_ids_set
ami = each.key
instance_type = "t2.micro"
tags = {
Name = "Server ${each.key}"
}
}
# Define a different local variable for for_each example
locals {
ami_ids_map = {
"linux" : "ami-08a0d1e16fc3f61ea",
"ubuntu": "ami-04b70fa74e45c3917",
}
}
resource "aws_instance" "server_map" {
for_each = local.ami_ids_map
ami = each.value
instance_type = "t2.micro"
tags = {
Name = "Server ${each.key}"
}
}
Initialize Terraform ๐
terraform init
Validate the Configuration โ
terraform validate
Apply the Configuration โก
terraform apply
Confirm with
yes
when prompted. ๐
Clean Up ๐งน To avoid unnecessary charges, destroy the resources after verification:
terraform destroy
Confirm with
yes
when prompted. ๐
Write About Meta-Arguments and Their Use in Terraform ๐
Meta-Arguments Overview
Meta-arguments in Terraform, such as count
and for_each
, allow you to manage multiple instances of resources efficiently. They reduce code duplication and make your Terraform configurations more maintainable and scalable. ๐ฑ
Count: Best for creating multiple identical resources. Accepts a number and iterates that many times. ๐ข
For_Each: Suitable for creating multiple resources with different values. Accepts a set or map and iterates over each element. ๐๏ธ
Using these meta-arguments can significantly simplify your infrastructure as code, making it easier to read, write, and maintain. โ๏ธ
Happy Learning! ๐
Embrace the power of Terraform meta-arguments and enhance your DevOps skills. Keep experimenting and happy coding! ๐
Subscribe to my newsletter
Read articles from Nilkanth Mistry directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
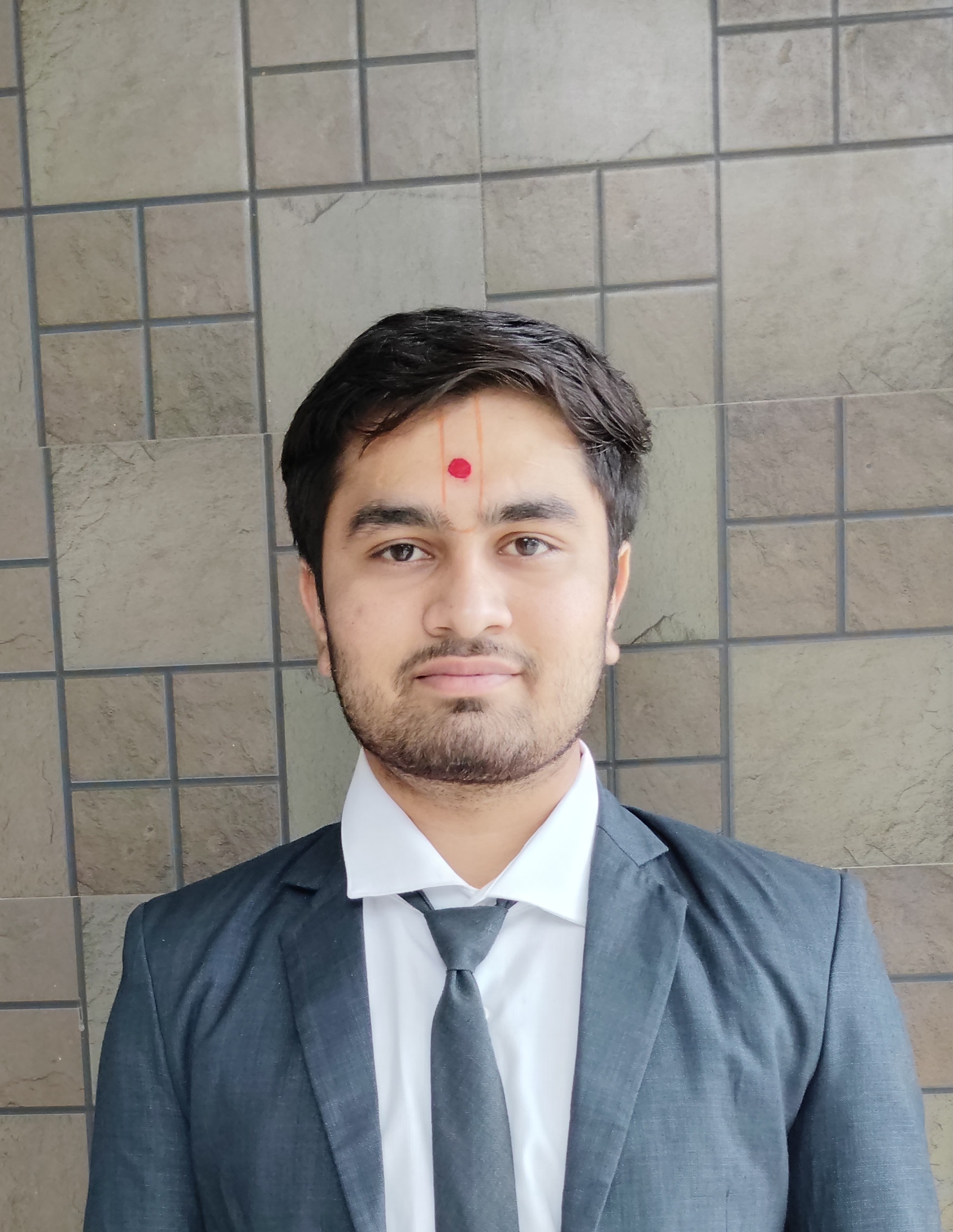
Nilkanth Mistry
Nilkanth Mistry
Embark on a 90-day DevOps journey with me as we tackle challenges, unravel complexities, and conquer the world of seamless software delivery. Join my Hashnode blog series where we'll explore hands-on DevOps scenarios, troubleshooting real-world issues, and mastering the art of efficient deployment. Let's embrace the challenges and elevate our DevOps expertise together! #DevOpsChallenges #HandsOnLearning #ContinuousImprovement