Python Lists: A complete and in-depth review

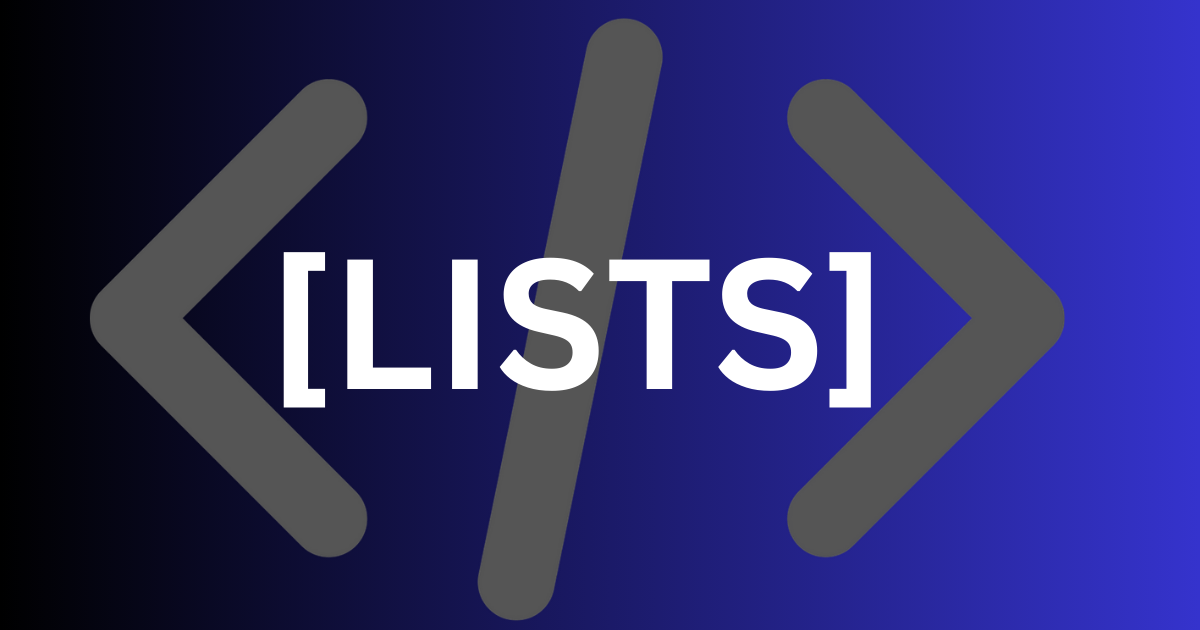
Python list is a data type used to store multiple items of the same variable. It is one of the four built-in data types in Python(Lists, Tuples, Sets & Dictionaries) used to store a collection(s) of data.
Features of Python Lists:
They are ordered
They are changeable
They allow duplicate values
They are indexed
Python lists are created using square brackets. The example below shows a variable x with its items presented in a list.
x = [4, 7, 8, 6, 1]
print(x)
If there’s one item in a list, end it with a comma:
x =[4,]
print(x)
The elements in the list are indexed with the first item(4) taking index 0.
Lists can accept different data types, e.g. integers, strings, and Boolean types.
X = [4, 7, 8, 6, 1] #integers
Y = ["tree", "house", "road"] #strings
z = [true, false] #Booleans
A single list can also hold different data types:
x = ["tree", 5, False] #different data types
We can use the print function to return the items in a list:
x = [4, 7, 8, 6, 1]
print (x)
Output: [4,7,8,6,1]
You can also print items at a specific index:
x = [4, 7, 8, 6, 1]
print(x[2])
Output: [8]
Removing an item in a Python list:
x = [4, 7, 8, 6, 1]
x.remove(8) #removes an item from a list
print(x). Output: [4, 7, 6, 1]
Adding an item to a Python list:
x = [4, 7, 8, 6, 1]
x.append(15) #adds an item to a list
print(x)
Output: [4,7,8,6,1,15]
Sorting items in a list:
#sort items in a list alphabetically or in ascending order.
x = [4, 7, 8, 6, 1]
y = ["tree", "house", "road"]
x.sort() print(x)
Output: [1,4,6,7,8]
y.sort() print(y)
output: ["house", "road", "tree"]
Performing calculations on items in a list:
x = [4, 7, 8, 6, 1]
print(x[0] + x[3]) #prints values at index 0 and 3
Output: [10]
. #You can add, subtract, multiply, or divide elements. simply change the operation symbol. you can also do similar calculations on elements from two different lists
Concatenating items in two different lists
X = [4, 7, 8, 6, 1]
Y = [9, 11, 2, 14, 5, 7, 1, 4]
print(X + Y)
Output: [4,7,8,6,1,9,11,2,14,5,7,1,4]
To print the matching items in the two different lists given do:
x = [4, 7, 8, 6, 1, 1]
Y = [9, 11, 2, 8,14, 5, 7, 1, 4]
match = [] #The new list where similar items are appended.
for i in x: #checks through the first list
if i in Y: #checks through the second list
match.append(i)
print(i)
Output: [1,1,4,8]
In order to print matching items in a list without duplicates do:
x = [4, 7, 8, 6, 1]
Y = [9, 11, 2, 8, 14, 5, 7, 1, 4]
match = []
for i in X:
if i in Y:
match.append(i)
print(set(match)
Output: [1, 4, 8]
#passing set through the output converts the list “match” into a Python set(does not accept duplicates) thus removing the repeated numbers.
Note: Ensure you have used the right indentation.
If you have any questions or additional comments, leave them below.
Subscribe to my newsletter
Read articles from Francis Onyach directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
