Exploring Buffers in Node.js: A Comprehensive Overview

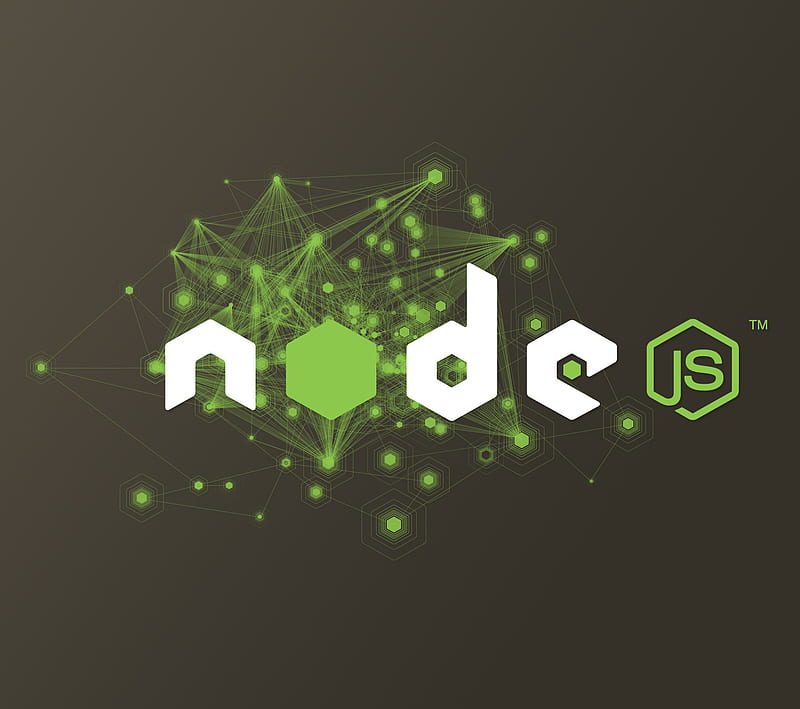
Welcome! If you’re into backend development, understanding buffers is essential. But before we get there, let's start with the basics: binary numbers, hexadecimal, and character encoding. By the end of this post, you'll have a solid grasp of buffers and how to use them in Node.js. Let’s get started!
The Basics: Binary Numbers and Hexadecimal
What Are Binary Numbers?
Think of binary numbers as the language of computers. Unlike us, who use a decimal system (0-9), computers use a binary system, which consists of only two digits: 0 and 1. Each digit in a binary number is called a "bit."
Example:
The binary number 1010
can be converted to a decimal number like this:
- (1×2^3)+(0×2^2)+(1×2^1)+(0×2^0)=8+0+2+0=10
So, 1010
in binary is 10
in decimal!
What Is Hexadecimal?
Hexadecimal, or hex for short, is another way to represent numbers. It’s base-16, meaning it uses sixteen symbols: 0-9 for values zero to nine and A-F for values ten to fifteen.
Example:
The hex number 1A
can be converted to a decimal number like this:
- (1×16^1)+(A×16^0)=(16+10)=26 (`A` stands for 10 in hex)
So, 1A
in hex is 26
in decimal!
Character Encoding: Bridging the Gap Between Humans and Machines
What Is Character Encoding?
Character encoding is how computers represent text. Since computers only understand binary, character encoding maps characters (like letters and symbols) to binary numbers.
Common Encodings
ASCII: Uses 7 bits to represent characters, covering basic English letters, digits, and some control characters. For example, the letter 'A' is
65
in decimal and01000001
in binary.UTF-8: A variable-width encoding that can represent every character in the Unicode character set. It's backward compatible with ASCII and is widely used on the web.
Example:
The character 'A' in different encodings:
ASCII:
65
(decimal) or41
(hex)UTF-8:
65
(decimal) or41
(hex)
Introduction to Buffers in Node.js
What Is a Buffer?
A buffer is like a container in Node.js that holds raw binary data. This is particularly useful for dealing with files, network streams, or any binary data.
Why Do We Need Buffers?
Efficiency: Handle raw binary data directly without converting to and from text.
Compatibility: Interact with binary data from external sources (like files and network protocols).
Performance: Reduce overhead by avoiding intermediate transformations.
Creating Buffers
In Node.js, buffers are created using the Buffer
class. Here are a few ways to create buffers:
- From an Array
const buf = Buffer.from([0x1, 0x2, 0x3, 0x4]); //The prefix "0x" is used to indicate that a number is written in hexadecimal (base-16) format.
console.log(buf); // <Buffer 01 02 03 04
- From a String
const buf = Buffer.from('Hello, World!', 'utf8');
console.log(buf); // <Buffer 48 65 6c 6c 6f 2c 20 57 6f 72 6c 64 21>
- Allocating a New Buffer
const buf = Buffer.alloc(10); // Allocates a buffer of 10 bytes
console.log(buf); // <Buffer 00 00 00 00 00 00 00 00 00 00>
Reading and Writing Data
Buffers let you read and write data in various formats:
- Writing to a Buffer
const buf = Buffer.alloc(10);
buf.write('Hello');
console.log(buf); // <Buffer 48 65 6c 6c 6f 00 00 00 00 00>
- Reading from a Buffer
const buf = Buffer.from('Hello, World!', 'utf8');
console.log(buf.toString('utf8')); // 'Hello, World!'
Manipulating Buffers
Buffers can be sliced and concatenated for flexible data manipulation:
- Slicing a Buffer
const buf = Buffer.from('Hello, World!', 'utf8');
const slicedBuf = buf.slice(0, 5);
console.log(slicedBuf.toString()); // 'Hello'
- Concatenating Buffers
const buf1 = Buffer.from('Hello, ');
const buf2 = Buffer.from('World!');
const concatenatedBuf = Buffer.concat([buf1, buf2]);
console.log(concatenatedBuf.toString()); // 'Hello, World!'
Buffer Methods
Here are some useful methods provided by the Buffer
class:
buf.toString(encoding)
: Converts the buffer data to a string using the specified encoding.buf.write(string, offset, length, encoding)
: Writes a string to the buffer.buf.slice(start, end)
: Returns a new buffer which is a slice of the original buffer.Buffer.concat(list, totalLength)
: Concatenates an array of buffer instances into a single buffer.
Practical Use Cases of Buffers
Reading Files
Buffers are commonly used when reading files in Node.js:
const fs = require('fs');
fs.readFile('example.txt', (err, data) => {
if (err) throw err;
console.log(data); // This is a buffer containing the file data
});
Handling Network Data
Buffers are also essential for handling data from network streams:
const net = require('net');
const server = net.createServer((socket) => {
socket.on('data', (data) => {
console.log(data); // This is a buffer containing the received data
});
});
server.listen(8080, '127.0.0.1');
Interacting with Binary Protocols
When dealing with binary protocols, buffers allow you to encode and decode messages efficiently:
const buf = Buffer.alloc(4);
buf.writeUInt32BE(0x12345678, 0);
console.log(buf); // <Buffer 12 34 56 78>
Conclusion
Buffers are a powerful feature in Node.js, enabling efficient handling of binary data. From reading files to processing network streams, buffers provide the necessary tools to work with raw binary data directly. By understanding the basics of binary and hexadecimal systems and character encoding, you can effectively use buffers in your Node.js applications to improve performance and compatibility with various data sources.
Subscribe to my newsletter
Read articles from Ashu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ashu
Ashu
Accomplished backend developer adept in a wide array of technologies including Node.js, Express.js, MongoDB, PostgreSQL, TypeScript, Redis, RESTful APIs, GraphQL, Docker, and comprehensive testing frameworks such as Mocha, Chai, and Jest. Well-versed in AWS services including EC2, S3 and experienced in leveraging cloud infrastructure for scalable backend solutions. Recognized for designing and implementing scalable, secure, and efficient solutions that drive business growth.