Mastering Authentication in Node.js: Implementing JWT with Express.js
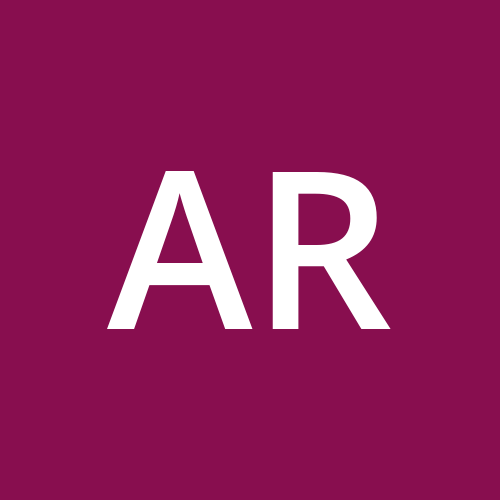
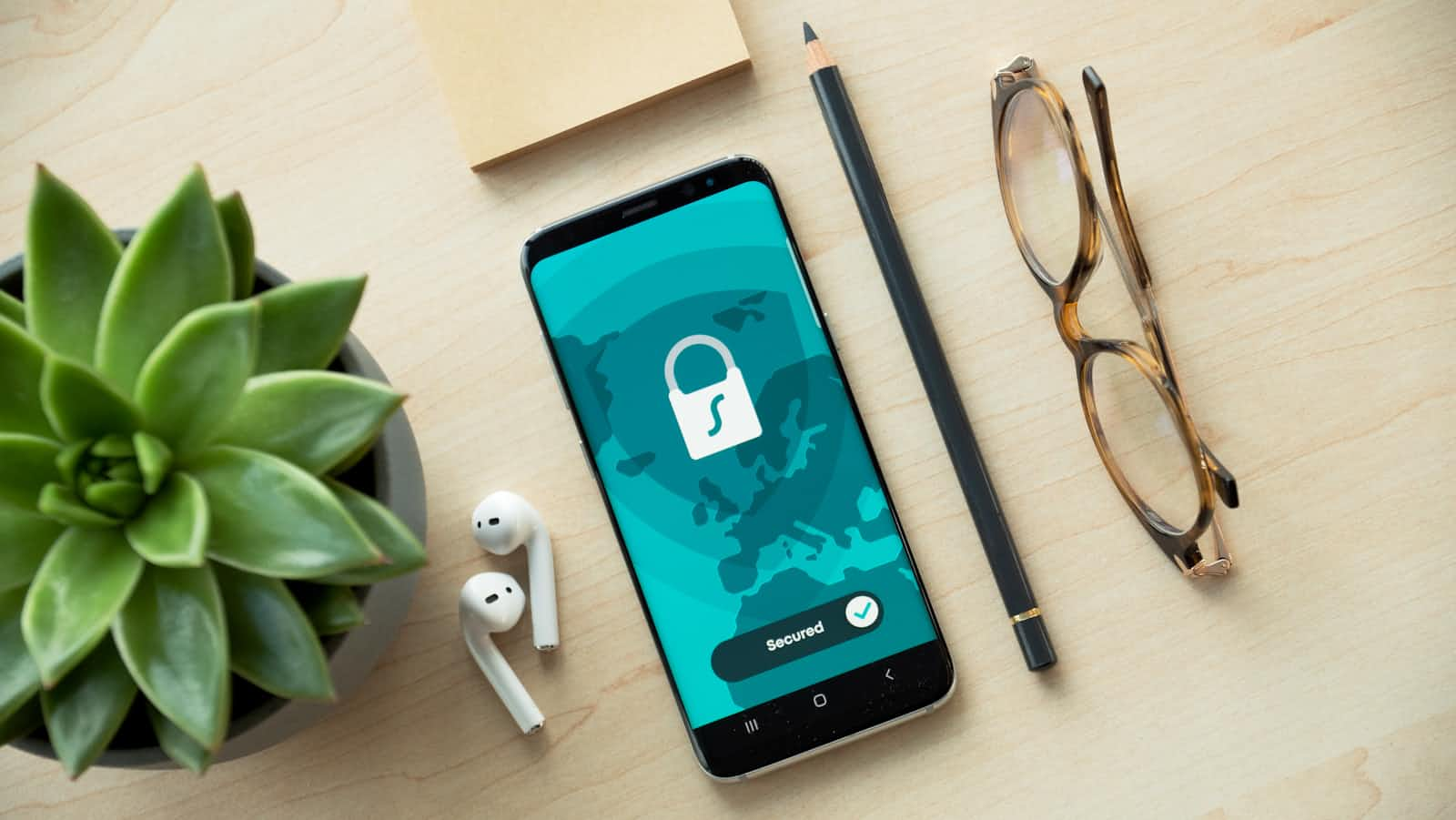
In the digital age, securing web applications is not just an option but a necessity. Whether you're handling sensitive user data, processing transactions, or managing private information, robust security measures are crucial to protect against unauthorized access and potential breaches. Authentication is the first line of defense in safeguarding your application, ensuring that only verified users can access certain features or data.
There are various methods to implement authentication in web applications, each with its own advantages and use cases. One of the most popular and effective methods is using JWT (JSON Web Token).
In this guide, we’ll explore how to set up JWT authentication in a Node.js application using Express.js. We will cover the basics of JWT, why it's a preferred method for authentication, and walk you through the process of integrating JWT into your application. By the end of this tutorial, you'll have a secure and efficient authentication system ready to use in your Node.js projects.
What is JWT?
JWT (JSON Web Token) is a compact, URL-safe token format used for securely transmitting information between a client and a server. This transmission typically occurs in the context of authentication and authorization. JWTs are designed to be both lightweight and efficient, making them suitable for a variety of scenarios.
JWTs consist of three parts:
Header:
- This section contains information about the type of token and the hashing algorithm used (such as
HS256
orRS256
).
- This section contains information about the type of token and the hashing algorithm used (such as
{
"alg": "HS256",
"typ": "JWT"
}
Payload:
- This section contains the claims, such as user information and additional data. Claims can include information like user ID, expiration time, and other metadata.
{
"sub": "1234567890",
"name": "John Doe",
"iat": 1516239022
}
Signature:
- Verifies that the sender of the JWT is who it says it is and ensures that the message wasn’t changed along the way. The signature is created by taking the encoded header, encoded payload, a secret key, and the specified algorithm.
(Simplified):
HMACSHA256(
base64UrlEncode(header) + "." +
base64UrlEncode(payload),
secret)
After creating the JWT, these parts are Base64Url encoded to form the final token, which looks like this: xxxxx.yyyyy.zzzzz
.
Example JWT Structure:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c
Why Use JWT for Authentication?
Stateless: JWTs are self-contained, meaning it doesn't require the server to store session information about each user between requests. Instead, all the information needed to verify a user’s identity is encoded directly in the token itself.
Scalable: JWTs are compact and efficient to transmit, and the server doesn’t need to track token information.
Secure: JWTs are signed to ensure integrity and can be encrypted to protect sensitive data.
Interoperable: JWTs follow an open standard, allowing easy integration across different systems and environments, making them versatile for various applications.
Setting Up JWT Authentication with Node.js and Express.js
Here’s how you can implement JWT authentication in a Node.js app with Express.js:
Initialize a New Node.js Project
First, create a new Node.js project:
mkdir jwt-auth-example cd jwt-auth-example npm init -y
Install Required Packages
You’ll need Express for the server and
jsonwebtoken
for handling JWTs:npm install express jsonwebtoken bcryptjs body-parser
Create the Basic Server Setup
Set up a basic Express server in a file called
server.js
:const express = require('express'); const bodyParser = require('body-parser'); const jwt = require('jsonwebtoken'); const bcrypt = require('bcryptjs'); const app = express(); const port = 3000; app.use(bodyParser.json()); app.listen(port, () => { console.log(`Server running on http://localhost:${port}`); });
Create User Authentication Routes
Add routes for user registration and login:
const users = []; // In-memory user store for example purposes // Register Route app.post('/register', (req, res) => { const { username, password } = req.body; const hashedPassword = bcrypt.hashSync(password, 8); const user = { username, password: hashedPassword }; users.push(user); res.status(201).send({ message: 'User registered successfully!' }); }); // Login Route app.post('/login', (req, res) => { const { username, password } = req.body; const user = users.find(u => u.username === username); if (!user) { return res.status(404).send({ message: 'User not found' }); } const passwordIsValid = bcrypt.compareSync(password, user.password); if (!passwordIsValid) { return res.status(401).send({ message: 'Invalid password' }); } const token = jwt.sign({ id: user.username }, 'your_jwt_secret', { expiresIn: 86400 // 24 hours }); res.status(200).send({ token }); });
Protecting Routes with JWT Middleware
Create middleware to protect routes:
function verifyToken(req, res, next) { const token = req.headers['x-access-token']; if (!token) return res.status(403).send({ message: 'No token provided' }); jwt.verify(token, 'your_jwt_secret', (err, decoded) => { if (err) return res.status(500).send({ message: 'Failed to authenticate token' }); req.userId = decoded.id; next(); }); } // Protected Route app.get('/protected', verifyToken, (req, res) => { res.status(200).send({ message: 'This is a protected route', userId: req.userId }); });
Testing Your API
Use tools like Postman to test your API:
Register a new user at
http://localhost:3000/register
.Log in with the user credentials at
http://localhost:3000/login
to get a JWT token.Access the protected route at
http://localhost:3000/protected
with the JWT token in the headers.
Best Practices for JWT Implementation
Use Strong Secret Keys: Generate long, complex keys and store them securely using environment variables or a key management system. Avoid hardcoding keys in your source code.
Store JWTs Securely: Use HTTP-only cookies for storing JWTs to protect against XSS attacks. Avoid localStorage or sessionStorage for sensitive tokens.
Set Expiration Time: Set short expiration times for JWTs (
exp
claim) to limit exposure if tokens are compromised. Use refresh tokens to maintain sessions.Validate Tokens Properly: Always validate the JWT’s signature and claims on the server. Use a reliable library to handle this process.
Implement Token Refresh: Use refresh tokens to extend user sessions securely, storing them in HTTP-only cookies and rotating them regularly.
Limit Token Scope: Include only essential claims in the JWT payload. Use roles or scopes to control access and avoid storing sensitive information.
Use HTTPS: Always transmit JWTs over HTTPS to protect them from being intercepted.
Handle Logout and Revocation: Provide a way to revoke tokens on logout. Use short-lived tokens or maintain a blacklist of revoked tokens.
Conclusion
Implementing JWT authentication in Node.js with Express.js enhances your application's security and scalability. By following these steps, you can build a robust authentication system that ensures only authorized users have access to protected resources. Start integrating JWT in your projects today and enjoy the benefits of secure, stateless authentication.
If you found this post helpful, please give it a like and share it with others who might benefit from it. Happy coding!
Subscribe to my newsletter
Read articles from Artur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
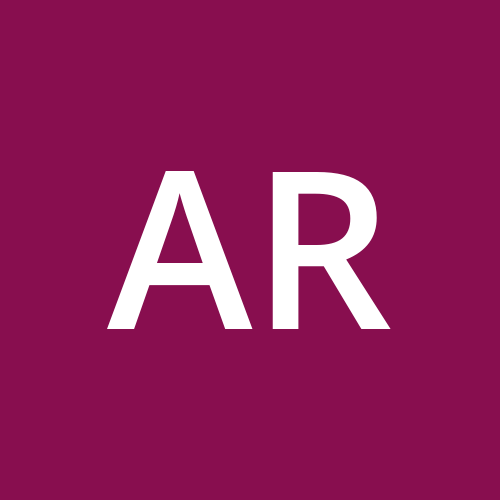