Collatz Conjecture Visualization using D3.js
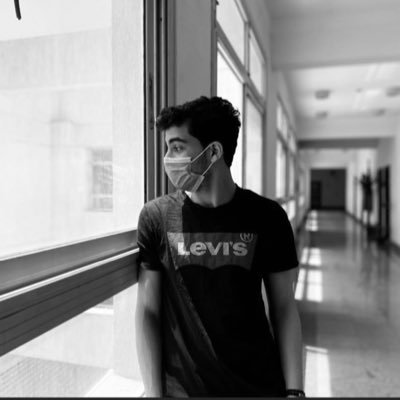
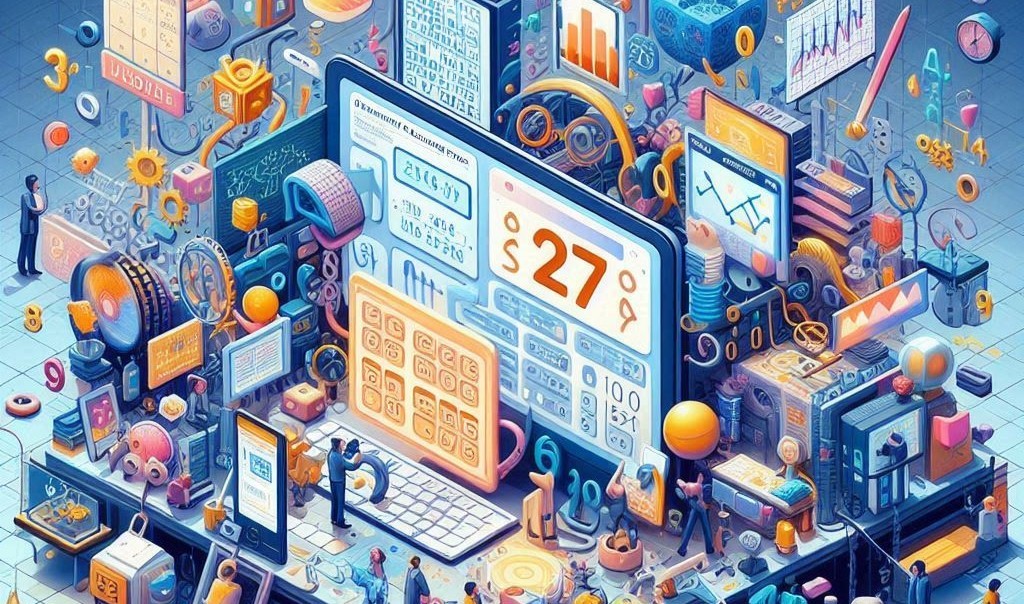
Introduction
I built fun project to try learning D3.js just for experimenting and enjoyment you can try it yourself from here:
Collatz Conjecture Simulation On Github Pages
I was browsing YouTube randomly for entertainment when I found a video recommendation on the topic we're discussing today: The Collatz Conjecture.
First, we should understand the difference between a conjecture, a theory, and a hypothesis, but unfortunately, that's not our main topic. However, I recommend the following videos to understand the difference:
Fact vs. Theory vs. Hypothesis vs. Law… EXPLAINED! (Be Smart Channel)
What is a Theorem, Corollary, Conjecture, Lemma, Axiom, and Proposition? (Quoc Dat Phung Channel)
Both videos are short and straightforward, but they are for general knowledge and not in-depth.
Conjecture Meaning
But briefly, for the part that concerns us, What is a conjecture?
A conjecture is simply when someone comes and says:
"Hey folks, there's something correct I've discovered that works perfectly."
So, we tried it, and it turned out to be true and working.
But when we asked him how he reached this conclusion?
he stood there and said:
"I don't know, it's just right this way, and we all tried it, and it worked out."
Simply put, we tried it and it turned out to be true, but we couldn't come up with a mathematical explanation or detailed formula to reach the solution or at least understand why it works this way.
Also, is it always working, or does it sometimes fail?
Are there any unexplored edge cases?
Additionally, For our case in this conjecture we don't have a consistent pattern, which is something I will explain later.
Collatz Conjecture
So let's understand what the Collatz Conjecture is and through it, you will understand what I mean.
One day, Collatz simply said:
Think of any number that comes to your mind and we will apply these two equations together:
If the number is even, we divide it by two: n/2
If it's odd, we multiply it by three and add one: 3n+1
For programming friends (Pseudo Code):
If even:
n=n/2
If odd:
n=3n+1
And we will repeat this process several times.
The surprise!
No matter what number you started with, if you apply these equations, the result will eventually be 1!
Let's give a small example:
Let's start with the number 6.
6 is an even number. We divide it by 2, it becomes 3.
3 is an odd number. We multiply it by 3 and add 1: (3*3)+1 = 10.
10 is an even number. We divide it by 2, it becomes 5.
5 is an odd number. We multiply it by 3 and add 1 = 16.
16 is an even number. We divide it by 2, it becomes 8.
8 is even, divide by 2, it becomes 4.
4 is even, divide by 2, it becomes 2.
2 is even, divide by 2, it becomes 1!
So, we started with 6, applied the equations, and ended up with 1!
Therefore, we can say our journey to reach the number 1 is:
- [6 - 3 - 10 - 5 - 16 - 8 - 4 - 2 - 1] which we can call "Sequence"
Try any number in the world and apply the same two equations, and you will eventually get 1!
Collatz Conjecture Applications
Knowing about the Collatz Conjecture is something for fun, general knowledge, or maybe treat it as a magic trick to amaze your friends.
However, using it as something reliable in practical applications is not going to happen for some simple reasons I won't delve into here.
but you can check this on Wikipedia to Understand the problem:
I'll take just one reason here: There is no consistent pattern to the outputs of this process.
The output varies greatly and randomly between different numbers.
To clarify:
the most famous number in this conjecture is 27.
If we take this number and apply the equations, we do reach the number 1, but after 111 steps.
This is the sequence to reach number 1:
[27, 82, 41, 124, 62, 31, 94, 47, 142, 71, 214, 107, 322, 161, 484, 242, 121, 364, 182, 91, 274, 137, 412, 206, 103, 310, 155, 466, 233, 700, 350, 175, 526, 263, 790, 395, 1186, 593, 1780, 890, 445, 1336, 668, 334, 167, 502, 251, 754, 377, 1132, 566, 283, 850, 425, 1276, 638, 319, 958, 479, 1438, 719, 2158, 1079, 3238, 1619, 4858, 2429, 7288, 3644, 1822, 911, 2734, 1367, 4102, 2051, 6154, 3077, 9232, 4616, 2308, 1154, 577, 1732, 866, 433, 1300, 650, 325, 976, 488, 244, 122, 61, 184, 92, 46, 23, 70, 35, 106, 53, 160, 80, 40, 20, 10, 5, 16, 8, 4, 2, 1.]
In contrast to the number 50, which is larger, we find the sequence:
[25, 76, 38, 19, 58, 29, 88, 44, 22, 11, 34, 17, 52, 26, 13, 40, 20, 10, 5, 16, 8, 4, 2, 1]
We reached 1 in just 24 steps.
So 27 took 111 steps to reach 1, while 50 took only 24 steps.
Simply put, there is no common pattern among all the numbers we might try to reach number 1.
Thus, how can we use something in computing with a random and unpredictable behavior?
Bro, We initialize pointers in C++, cause we are afraid of that an uninitialized pointer can point to any memory location, leading to undefined behavior if accessed.
The project "The Fun Part"
So, I think we can agree now that, this conjecture is just a game or something to have fun with.
So I simply decided to have fun with this project:
I decided to create a visualization of the behavior of numbers when applying these two equations.
I wrote the algorithm and made sure it works.
I created a nice UI (neither great nor terrible) but it serves the purpose.
I used the Javascript library called D3.js for visualizing the data.
The Goal Of The Project
The real goal of this project was to:
Review the basic concepts of Front-End: HTML – CSS – Javascript and DOM Manipulation – Bootstrap CSS since I'm not a big fan of the front-end development field, but basics are basics, and having knowledge in this field is important if you're working in web development, even if you work in back-end development.
Learn how to use the library p5.js, not because I need it for work but just for fun... but honestly, it was very complicated and I felt it wouldn't let me enjoy this small project (As I told you the goal of this project is building something fun).
So I decided to use D3.js, which is more user-friendly and faster to use and learn. I learned it in about two hours, got a ready-made template made by someone using the library, and started modifying it based on the functions I wrote and the data those functions return.
I put the chart code(The D3.js template with the modifications) in a function too.
And started calling the function called "visualize" in the other function in the algorithms file that is simply putting everything together .
And voila, the project Is completed.
You can see the code on Github here:
You can try the project yourself here:
Collatz Conjecture Simulation On Github Pages
Note: I uploaded it on Github pages (put any number in your mind, see how it behaves, play around to understand the concept, then check the code it's nice and small so have fun).
And if you liked this project, the explanation, and the article, then hit a like and share and follow me on LinkedIn from here:
See you in the next blog. 👋
Subscribe to my newsletter
Read articles from Mohamed Abusaif directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
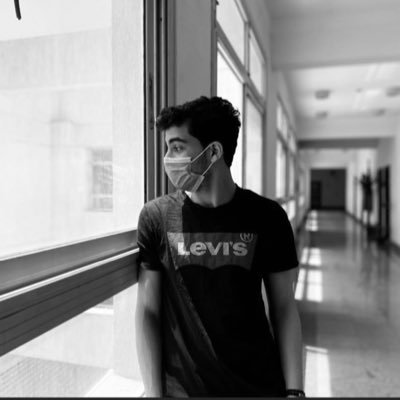
Mohamed Abusaif
Mohamed Abusaif
Back-End Developer, a Computer Science Graduate From Benha University, and a tech geek Who loves New Technology Topics. I love to try new stuff every day, gain new expertise, And expand my social network, Sharing knowledge about the stuff I learn everyday.