Starting With the Flask Web app

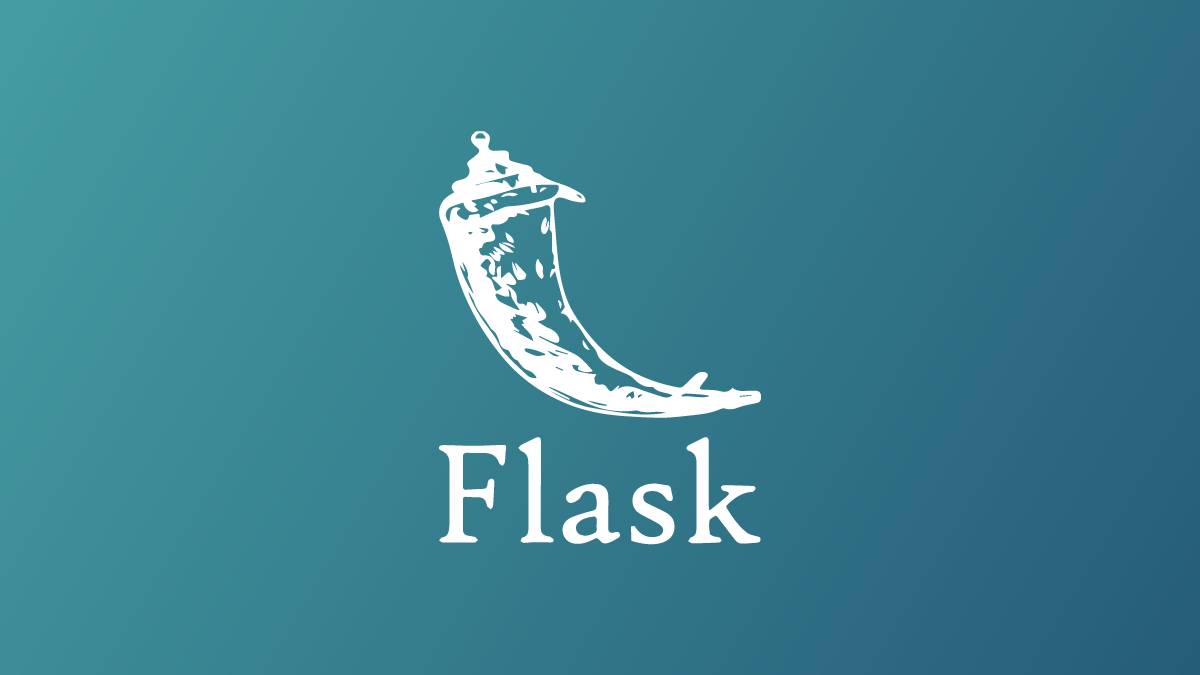
Flask is a Python framework that stands out for its simplicity and ease of use. While Python offers several frameworks for web app development, Flask holds a special place. Web apps are composed of two essential components: the front end (including templates and static files) and the back end (comprising the database and view route codes). Flask excels in its straightforward approach, as it keeps the file codes and formats neatly separated.
To grasp this distinction more clearly, let's compare Flask with other frameworks such as Django and FAST-API. Django possesses a more complex code structure and file management system. It is primarily divided into two parts: the app and the project. The app handles the back interface, whereas the project acts as a bridge between them. Similarly, like other web apps, Django also incorporates a front-end and a back-end. These sections contain code for different functions. Additionally, Django includes a code page that establishes connections with the URL path and a view page that facilitates communication between the backend and the front end. Furthermore, a settings page ensures proper configuration of settings.
Flask consists of a primary page called main.py that serves as a connection point between the backend and the front end. In addition to this, there are distinct sections dedicated to both the back end and front end. These encompass the Template section, which deals with the presentation of the web pages, the static file section, which handles the assets of the website, and finally, the database section where diverse data is stored.
Let's discuss this in a more detailed form.
Templates:
This section contains the different pages of the HTML that are the HTML codes containing pages. The pages like the homepage are called index.html, header.HTML, and foote.html. When creating a website, we often have multiple pages that serve different purposes. In addition to the main functional HTML pages, there might be other pages as well.
To effectively merge our Python code with the HTML code, we can rely on Jinja coding. Jinja coding acts as a bridge between the two and enables smooth integration.
from jinja2 import Template
# Define the template
template = Template("""
<h1>Hello, {{ name }}!</h1>
<p>You are {{ age }} years old.</p>
""")
# Render the template with data
rendered_html = template.render(name="Alice", age=25)
# Print the rendered HTML
print(rendered_html)
Jinja is a way to incorporate Python into your code, allowing you to generate output from your Python code and display it on an HTML page that can be viewed in a web browser on your local machine. i.e. http://localhost:8080
Statics:
This section contains all the essential resources that will be utilized within the project, including but not limited to images, CSS stylesheets, JavaScript files, and various other asset files. These components are integral to the project's functionality and visual presentation, ensuring a cohesive and engaging user experience. Including these resources signifies the beginning of the development process where the static assets are prepared and organized for later integration into the dynamic application framework.
Setting up the Environment:
Setting up the environment for a Flask project involves creating a virtual environment, installing Flask, and setting up the project structure. Here is a general guide to help you set up the environment for a Flask project:
Create a Virtual Environment:
Create a new directory for your project.
Open a terminal and navigate to the project directory.
Create a virtual environment using the following command
python -m venv venv
Activate the virtual environment.
On Windows
venv\Scripts\activate
On macOS and Linux
source venv/bin/activate
Install Flask:
While the virtual environment is activated, install Flask using pip:
pip install Flask
Project Structure:
Inside your project directory, create the following structure:
project_folder/ ├── venv/ ├── app/ │ ├── templates/ │ │ └── base.html │ ├── static/ │ │ └── style.css │ └── __init__.py ├── main.py └── requirements.txt
main.py:
This section includes the complete code that serves as a bridge between the template, the database, and the various code files used in the project.
Example code</>
# Import necessary libraries
From Flask import flask
import template_module
import database_module
import other_code_module
def main():
# Load or render the template
template_data = template_module.load_template()
# Connect to the database
db_con = database_module.connect_to_db()r
# Retrieve data from the database
data_from_db = database_module.fetch_data(db_con)
# Perform any necessary data processing or manipulation
processed_data = other_code_module.process_data(data_from_db)
# Pass the processed data to the template for rendering
output = template_module.render_template(template_data, processed_data)
# Display or save the final output
print(output)
if __name__ == "__main__":
main()
Subscribe to my newsletter
Read articles from sahil saurav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
