Python's Built In Modules: The Sys Module

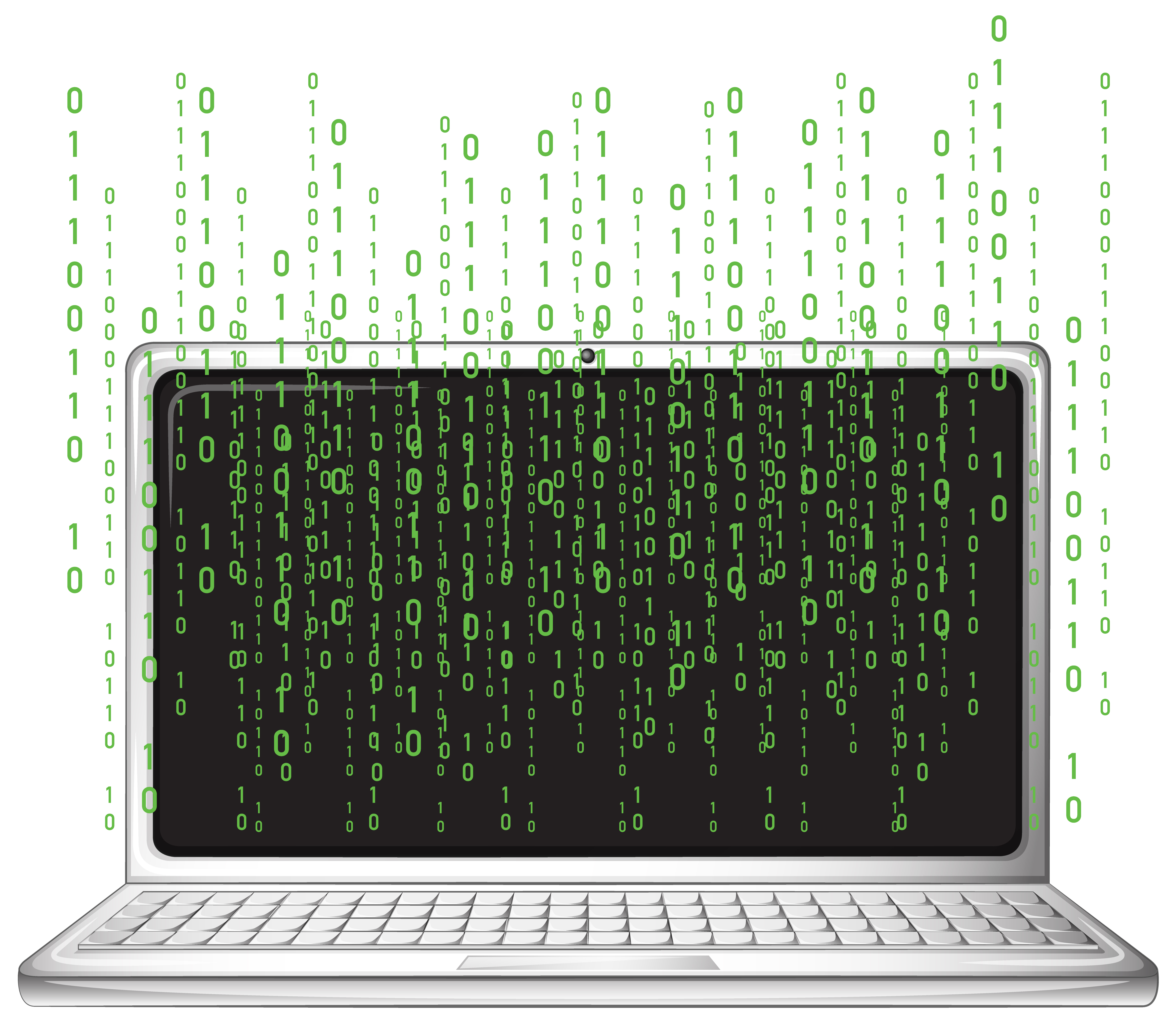
Aloha and karibu to another post in the Python built-in modules series. We would be looking at the sys module today. This would be a brief but informative one, so disfrutar!
The sys module is an awesome way to work with the Python runtime environment. Here, we would go through just a few examples so you’ll acquaint yourself with the module.
sys.argv
With this, we are dealing with a list of command line arguments that are passed to a python script. The first in this list is the name of the script while the others are the arguments supplied. You can use them in any way your code requires. Check out the example below:
import sys
print(f"'Laziness' is '{sys.argv[1]}' in the {sys.argv[2]} language")
The script above simply prints out a text with arguments supplied in the command line. Let’s see how to run and work with it.
C:\Users\lazyAmanda\Python Practice> python sysStuff.py kasala hausa
'Laziness' is 'kasala' in the hausa language
And that’s all it is. You simply supply the arguments in the terminal and see the results as so. Note that 'sysStuff' is the name of the file and would be the first in the list (i.e. sys.argv[0]).
sys.path
This may look familiar if you read my last post. This simply lists out the System’s Python path. You would also remember that the search path for all python modules can be found in this variable.
import sys
print(sys.path)
sys.version
Want to know what version of python you are using? It’s as simple as using the code below.
import sys
print(sys.version)
sys.getsizeof()
This just returns the size in bytes of an object.
import sys
someDigit = 30
print(sys.getsizeof(someDigit))
sys.getrecursionlimit()
Programs can crash for a number of reasons. Before you go off the rails speaking in tongues or strangling your rubber ducky to imagined death, it’ll be a good idea to consider exceeding the recursion limit a possible suspect. Knowing this can stop you from allowing the interpreter go into infinite recursion. You can however, increase the maximum recursion depth with sys.setrecursionlimit(). I would however not toy around with this unless I absolutely have to, which on average you may not have to.
import sys
print(sys.getrecursionlimit)
#You can also set the limit
sys.setrecursionlimit(1500)
sys.exit
This simply exits the program.
import sys
for i in range(6):
if i == 3:
sys.exit()
print(i)
sys.stdin and sys.stdout
If you’ve been coding a little while, stdin and stdout may stand out to you as implying standard input and standard output. sys.stdin is concerned with taking input directly from the command line and sys.stdout is about sending output to the command line. Check out the snippet below for a chat with the most annoying parrot in the command line. You can end the nightmare by typing “shut up!”
import sys
sys.stdout.write("An Intelligent Conversation\n\n")
for words in sys.stdin:
if "shut up!" in words:
sys.exit()
else:
print(f"parrota says: {words}")
Using the above sys module variables and functions, you may be able to create a simple command line program. Think up something creative. Here is an exhaustive list of a lot more of them you could look up when you need to. Tschüss!
ps...I make gifs now. :)
Subscribe to my newsletter
Read articles from Amanda Ene Adoyi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Amanda Ene Adoyi
Amanda Ene Adoyi
I am a Software Developer from Nigeria. I love learning about new technologies and talking about coding. I talk about coding so much that I genuinely think I could explode if I don't . I have been freelance writing for years and now I do the two things I love the most: writing and talking about tech.