Write your first React Native App with me (Part 1)
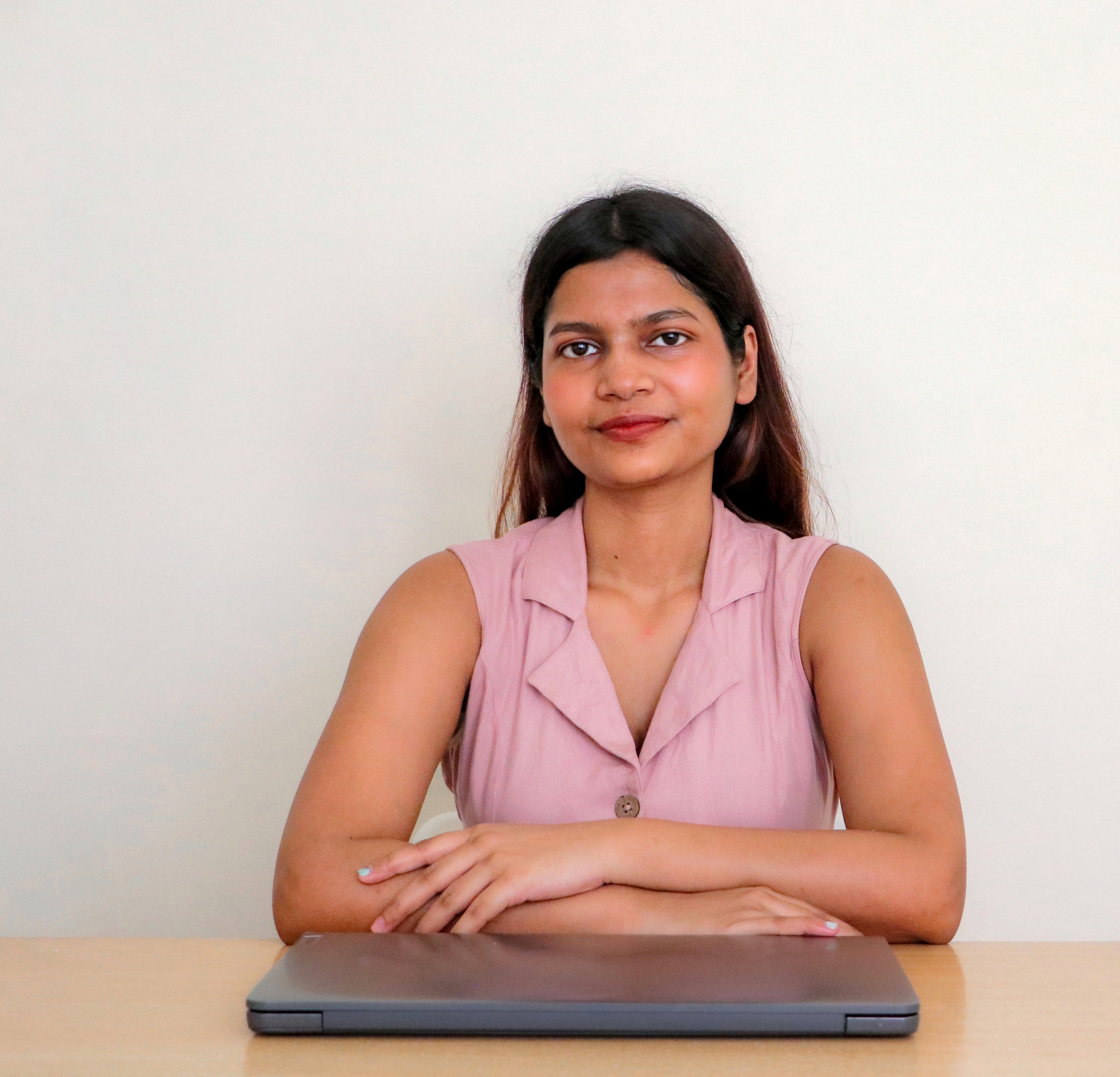

Let me make your life easy and give you choices that you have while creating your first React Native App. My advice is to pick one of these and stick to it rather than switching and trying to learn everything because then you will be able to master none.
Choice 1: Expo
Expo is a production-grade React Native Framework. Expo provides developer tooling that makes developing apps easier, in turn your life easier, by providing features like file-based routing, a standard library of native modules, and much more.
It is very easy to use. You don't need to do much setup or configuration. But it in a long run it makes your life harder as you don't really have much control over behind the scene processes.
Also, Expo uses React Native CLI in the backend. You can consider Expo as a wrapper around RN CLI to make your life easier by providing you some easy features.
Choice 2: React Native CLI
Personally, I prefer React Native CLI because let's be real if you want to create a high performant app with complete control you would have to learn CLI in the future. So why not start with it. And let me tell you it's damn easy. Only thing you will struggle with is the initial Setup for the Production grade application. But don't worry I will help you with that in this and next few articles.
Choice 3: You don't have one
Pick from the above two choices.
As you have read above, I prefer React Native CLI and I will be using only that. So, if you are leaning towards using Expo then fell free to find some other articles because there are some great one out there, just not from me.
Tech Stack
Let me introduce you to what you will need to make your App.
Typescript (Programming Language)
Nodejs, React, java openJDK, Android Studio (Basic Software Setup)
React Native CLI (Software)
React Navigation (Routing)
Stylesheet or NativeWind (UI Designing)
Redux or Zustand (State Management)
Axios or TanStack Query (Data Fetching)
Async storage or Watermelon DB or Supabase (Data Storage)
PowerSync (Syncing local and Remote data)
Reanimated (Animation)
Flipper or React Native debugger (Debug)
Bugsnag (Error Reporting)
Programming Language: Typescript
You might be wondering why I didn't say JavaScript. It's because I want you to have perfect habits from the starting. Typescript is basically an extension to JavaScript in which you have to always mention the data types while writing the code. This leads to least number of bugs. Trust me you will appreciate my advice later during debugging stage.
So, you need to learn JavaScript first. And then you will learn Typescript. And you will only write your React Native apps using Typescript.
Software: React Native CLI
You will use React Native CLI because it's just better.
Where should you learn it from? Documentation. React Native has one of the best documentations because they are highly simple. And if you don't like reading documentation then you should start liking it because you want to succeed in your software development career, right?
Reading documentation and implementing things using it is one of the best skills you will ever develop because there will always be some new software or package coming and there won't be tutorials available on it. So, you decide what kind of developer you want to be - the one who waits for others to explain things to them or the one who is proactive and learns by themselves by reading documentation. In the industry, you already know which kind of people excel.
Also, you need to learn the basic fundamentals of React like components, JSX, props, arrow functions, Higher order components, state, etc. Most of these concepts will be used in your React Native application.
Basic Software Environment Setup Documentation
Routing: React Navigation
You would have multiple screens in your app, and you would want to go from one screen to the other. For this purpose, you would use React Navigation. It is a library that provides all the features that you might need for different kinds of navigations such as Stack, Drawer, Bottom Tabs, etc.
React Navigation documentation Link
UI Designing: Stylesheet or NativeWind
Stylesheet is the default thing that is used to style any react native component. Stylesheet is to React Native, what CSS is to website development. I have been using stylesheet because it is very easy to use and quite powerful actually.
NativeWind is an alternate that you can use for styling in react native. Native wind uses Tailwind CSS as scripting language to create a universal style system for React Native. You should go for native wind if you plan to create mobile application as well as a website for it because then you will basically be using Tailwind CSS for both. Make your life easier and choose wisely for your app. Don't follow anything that someone else is preaching. Only you can make best decisions for you.
State Management: Redux or Zustand
You need to learn at least one state management tool if you want to survive in this industry. I suggest you learn both if time permits.
Redux is most widely used state management tool and most companies use this. But it is quite complex to implement. Zustand is relatively new but is becoming popular due to its ease of use and lightweight implementation that results in faster state updates and fewer re-renders.
Redux | Zustand |
More complex | Simple |
Steeper | Easier |
Centralized Store | Decentralized store with hooks |
Unidirectional data flow with actions and reducers | Direct updates with hooks |
Performance can be optimized, but potentially slower for smaller apps | Generally faster due to simplicity |
Large ecosystem with mature developer tools (Redux DevTools) | Smaller ecosystem, limited dedicated tools (integrates with React DevTools) |
Larger applications with complex state management | Smaller to medium-sized applications, projects that value simplicity |
Personally, I have used redux most of my development life but I have made few apps in Zustand and I think I will use it more.
Data Fetching: Axios or TanStack Query
If you are coming from web development world you might be familiar with axios already. Axios is a promise-based HTTP client for node.js and the browser for making basic API requests.
If I have to choose, I will always choose TanStack Query over Axios any day. React Query (TanStack Query) is used for data fetching where you just tell it to get your data and how fresh you need it to be, and the rest is automatic. It handles caching, background updates and stale data out of the box with zero-configuration.
For simple needs, Axios might be sufficient. For complex data fetching with caching and background updates, TanStack Query is the suitable option.
Data Storage: Async storage or Watermelon DB or Supabase
For data storage, the choice will always depend on the kind of requirements you have. There is never a right database for all apps. Some of the choice that we have is async storage, MMKV, watermelon DB, supabase.
I alternate between these data storage solutions as per my requirements. Currently I am leaning towards Supabase, which is a Firebase alternative because I have a requirement for cloud-based data storage solution.
AsyncStorage is ideal for storing small amounts of data like user preferences or settings. It is lightweight, unencrypted, and asynchronous key-value storage system that enables developers to store and retrieve data on the device's local storage.
Watermelon DB is also a great SQLite-based option. It is quite light weight. It allows storing structured data and provides offline capabilities. It's a good choice for apps requiring local persistence and faster data access compared to AsyncStorage.
MMKV has been really popular in the last year. It is the fastest key-value storage for React Native. It provides features like encryption, multiple instances, customizable storage location, fully synchronous calls, etc.
So, in the last year there has been a rising trend of local-first apps which basically means that your data should be available on your mobile device first and all the syncing to the cloud storage should happen in the background. For this I prefer using Supabase with PowerSync.
For syncing local and remote database: PowerSync
PowerSync is a Postgres<>SQLite sync layer and it's the only option I can recommend to you. Because that's the only one I like.
You can control which data is synced with which users. Define sync rules to control which buckets of data should sync to which users, using SQL statements.
Animation: Reanimated
React Reanimated simplifies creating smooth, performant animations in your React Native apps. With a declarative approach, you define what you want to animate, and Reanimated handles the complex calculations for stunning visuals at 60 fps and beyond, making your app feel responsive and engaging.
Debugging: Flipper or React Native Debugger
Flipper is a desktop platform that offers built-in tools for inspecting logs, UI structure, and network traffic, along with a vast plugin ecosystem to tackle specific debugging challenges. From Redux to memory usage, Flipper lets you see your app's inner workings and fix issues faster.
React Native Debugger is a standalone app for debugging React Native apps. It is based on official Remote Debugger and provide more functionality. It Includes React Inspector from react-devtools-core, Redux DevTools and Apollo Client DevTools.
Both are great tools but personally I prefer Flipper.
Error Reporting: BugSnag
BugSnag is used to report JavaScript and native errors. You can even see full stack trace. On iOS platforms, BugSnag can even detect terminations of your app by the operating system due to the device overheating.
Bugsnag react native integration guide
So by reading the above article if you think that it's a lot then let me tell you it is basic production grade setup. And you might need to add or remove few things as per your requirements. I suggest that you don't get scared by the number of things there are to learn. Rather dedicate 2 hours daily, 1.5 hours to implement react native code and half an hour to learn more about it. I suggest that you do this for next 30 days regularly and see what happens. Don't come to me saying it's tough. Honestly, I don't really care if you don't want to put effort in learning. I only care if you do the work and then come to me for some help.
FYI: If you were triggered by any of my comments then it's a you problem not a me problem.
Thanks, and Regards
JM
Subscribe to my newsletter
Read articles from Jyoti Maurya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
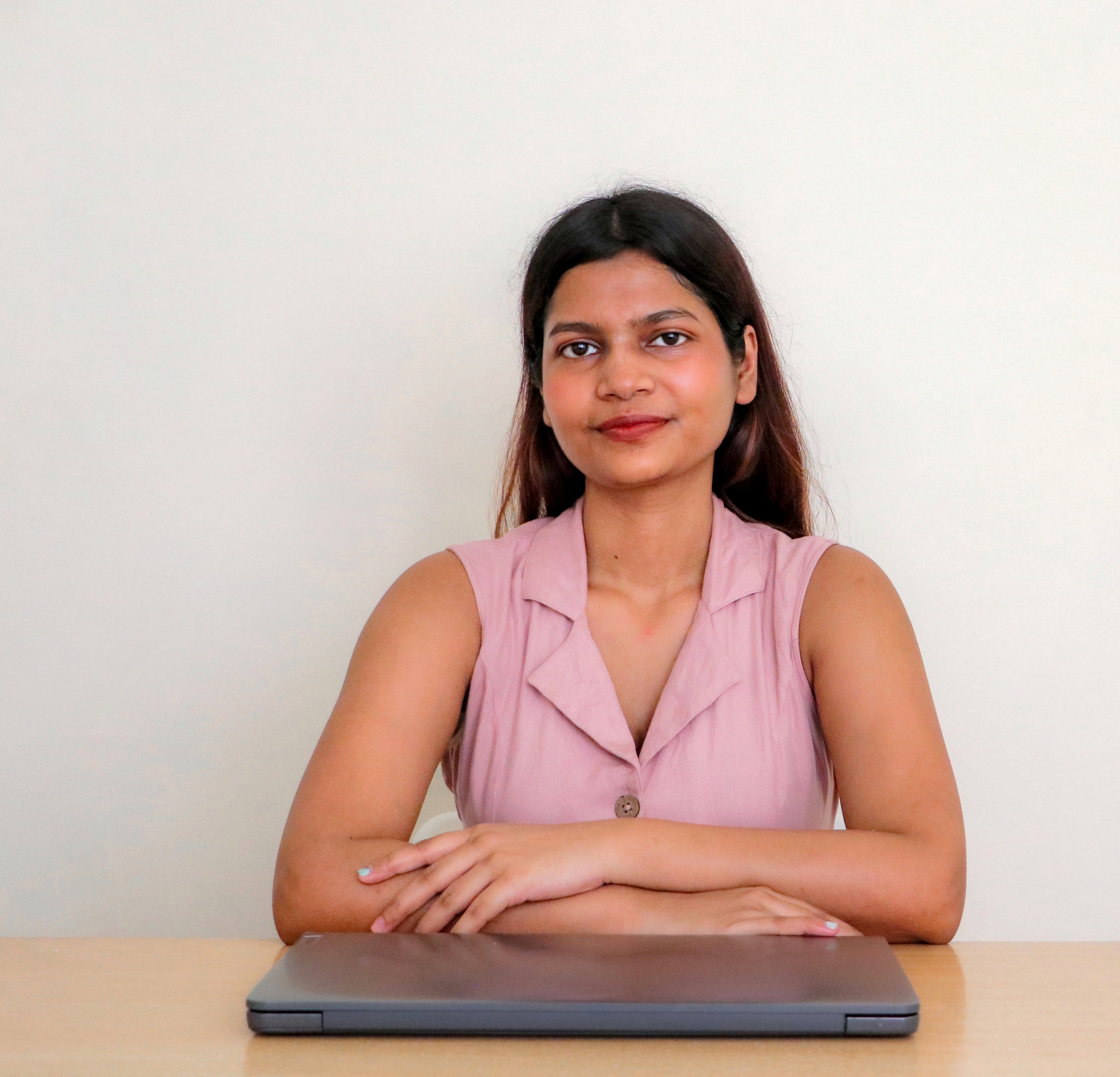
Jyoti Maurya
Jyoti Maurya
I create cross platform mobile apps with AI functionalities. Currently a PhD Scholar at Indira Gandhi Delhi Technical University for Women, Delhi. M.Tech in Artificial Intelligence (AI).