Angular Series: Key Concepts in a Flash
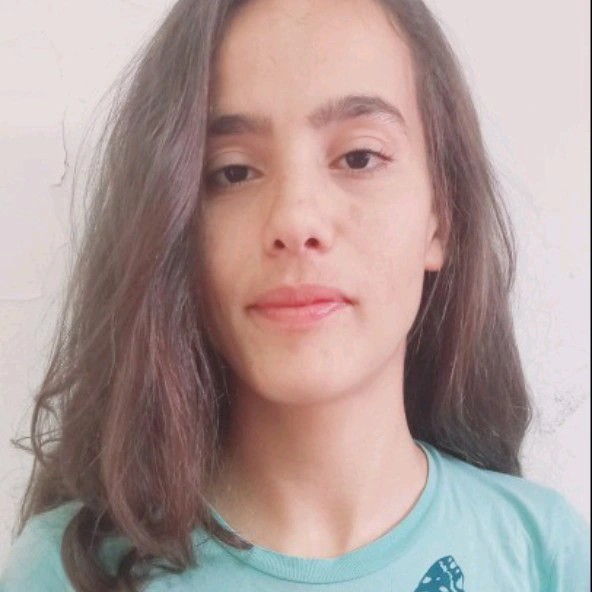
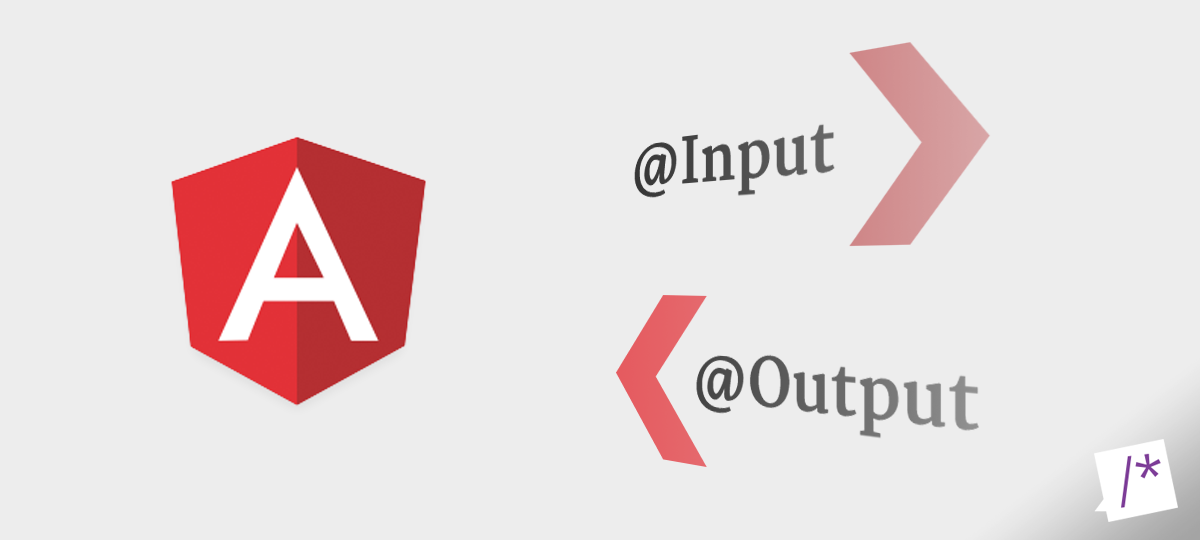
@Input()
and @Output()
allow Angular to share data between the parent context and child components.
"Think of
@Input()
and@Output()
like ports or doorways—@Input()
is the doorway into the component allowing data to flow in while@Output()
is the doorway out of the component, allowing the child component to send data out."
In this first part, we will explore what the @Input() property is and how to use it. The explanation will be illustrated with an example to ensure an in-depth understanding. The next blog post will tackle the second part, which concerns the @Output() property.
1- @Input() in Angular: How It used
In simple terms, the @Input() property allows a parent component to pass data to its child components This facilitates communication between the parent and child components.
1- At first, we decorate the property with @Input()
in the child component :
import { Component, Input } from '@angular/core'; // import Input
export class childComponent {
@Input() inputData: string;
//@Input() property can have any type, such as number, string, boolean, or object.
}
2- Then, use the child component's selector, here <app-child-component>
, as a directive within the parent component template. Next, bind the parent component's property to the child's @Input
property.
<app-child-component [inputData]="greeting"></app-child-component>
3- In the parent component class, parent.component.ts
: we assign explicitly static value to "greeting".
export class ParentComponent {
greeting = 'HI';
}
4- Finally, use interpolation to insert data in childComponent template; sent from the parentcomponent.
<p>
hello, I am child component. My parent said : {{inputData}}
</p>
@Input('startDay') campaignRequestStartDay : string;
OnChanges
and @Input()
According to the official Angular Doc, OnChanges
is specifically designed to work with properties that have the @Input()
decorator.
Another example ..
Here is the app component class and we have a BestTennisWoman variable defined in the class set to true.
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'input decorator';
BestTennisWoman = true;
}
Here is the app template, where we have the childcomp as a child component of the app component. We pass the boolean variable into it, depending on which button is clicked.
<h1>Vote for the best tenniswoman in 2023</h1>
<button (click)="BestTennisWoman=true">ONS JABEUR</button>
<button (click)="BestTennisWoman=false">IGA SWIATEK</button>
<app-childcomp [bestPlayer]='BestTennisWoman'></app-childcomp>
Here is childcomp.component.ts in which bestPlayer variable is docorated with the Input propriety.
import { Component, OnInit, Input } from '@angular/core';
@Component({
selector: 'app-childcomp',
templateUrl: './childcomp.component.html',
styleUrls: ['./childcomp.component.css']
})
export class ChildcompComponent implements OnInit {
@Input() bestPlayer: boolean;
constructor() { }
ngOnInit(): void {
}
}
This is the childComp template. After binding the value of BestPlayer, which is set by the app component using the ngIf directive, an appropriate message is displayed.
<h2> This is the best tennisWoman </h2>
<h4 *ngIf='!bestPlayer; else elseBlock'> IGA is the best tennisWomen in 2023</h4>
<ng-template #elseBlock><h4> ONS is the best tennisWomen in 2023</h4></ng-template>
Subscribe to my newsletter
Read articles from Meriem Trabelsi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
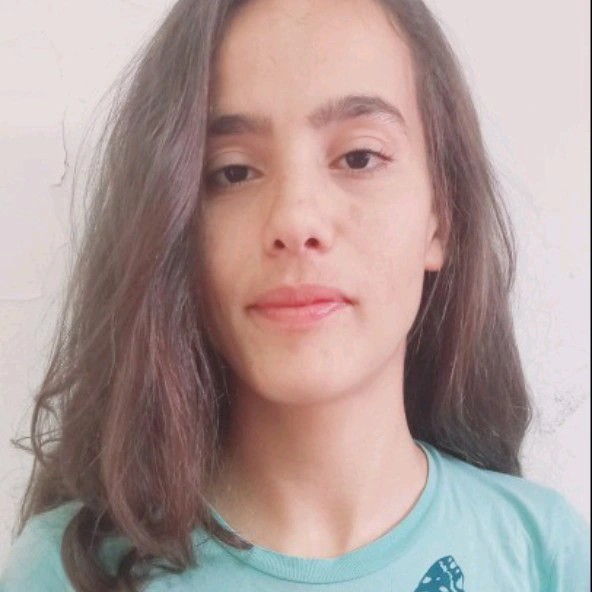
Meriem Trabelsi
Meriem Trabelsi
I am a curious, ambitious young woman passionate about the IT world 🙂. Acutually, I am software Engineering Student @ISI. More motivated to discover new technologies. I am interested in project management and the different approaches, business intelligence, web/mobile development, ISTQB (software testing) and so on ..