Methods of document object
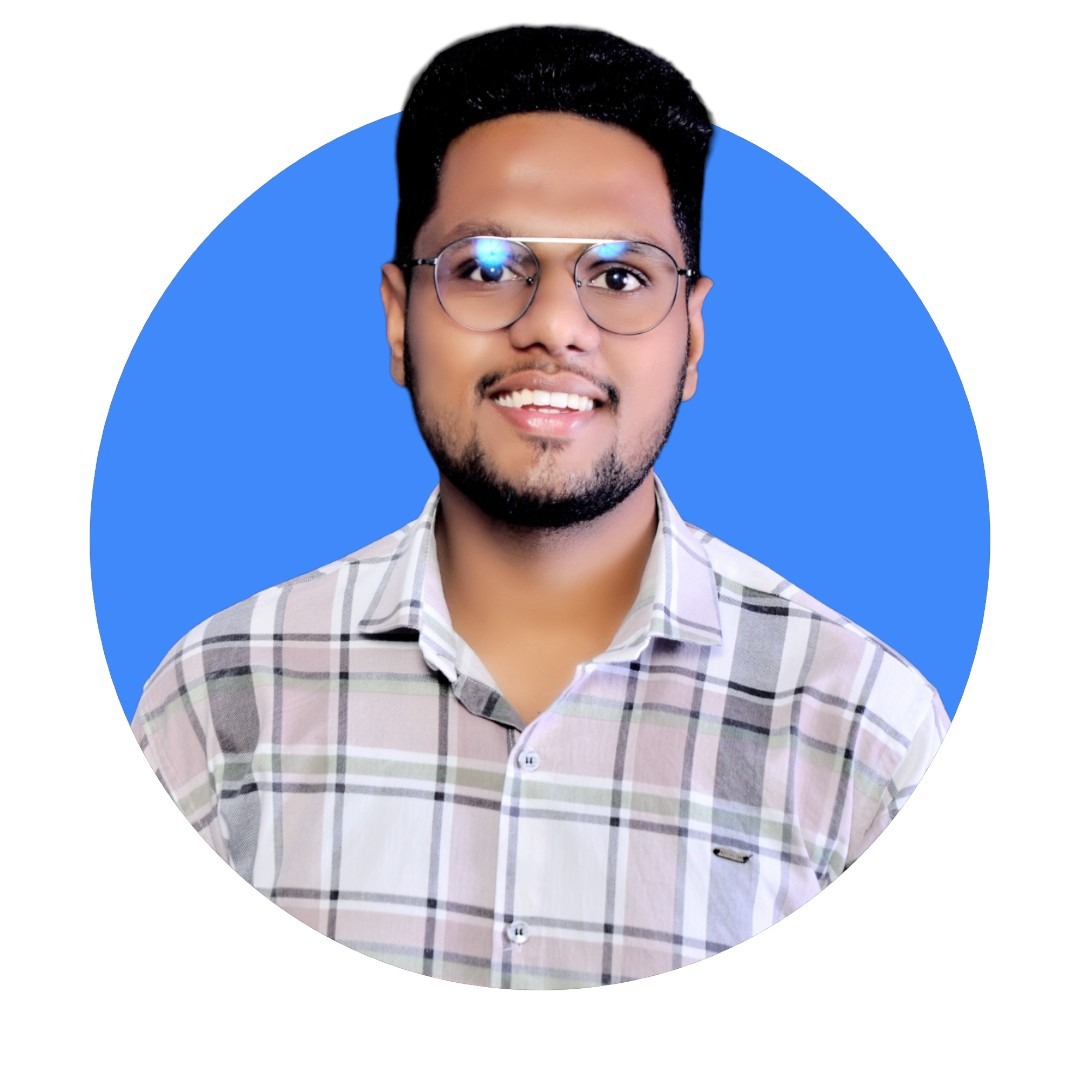
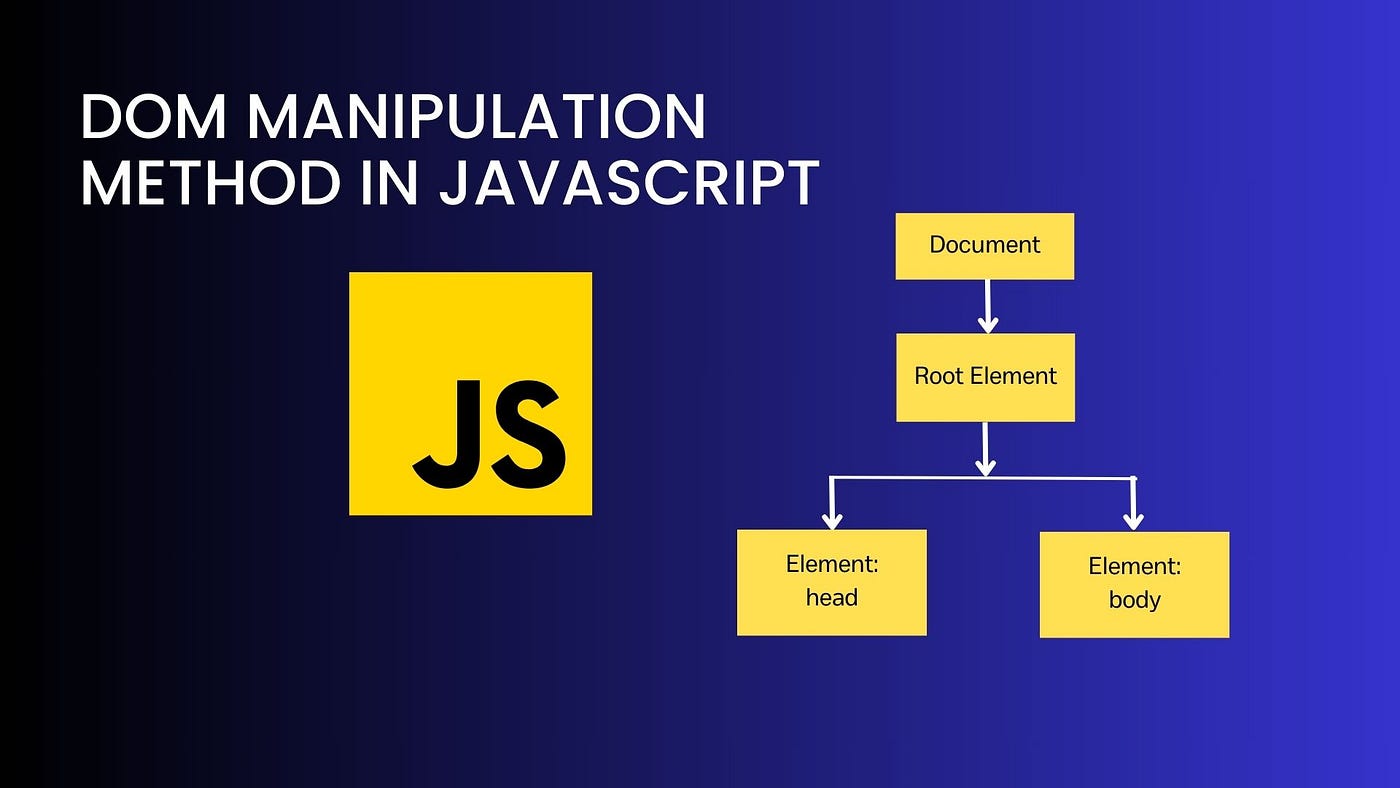
document.getElementById() method :
The getElementById() method returns an element with a specified value.
The getElementById() method returns
null
if the element does not exist.The getElementById() method is one of the most common methods in the HTML DOM.
It is used almost every time you want to read or edit an HTML element.
Syntax :
document.getElementById(elementID)
Example :
Get the element with the specified id:
document.getElementById("demo");
Get the element and change its color:
const myElement = document.getElementById("demo");
myElement.style.color = "red";Or just change its color:
document.getElementById("demo").style.color = "red";
document.getElementsByName() method :
The document.getElementsByName() method returns all the element of specified name.
The syntax of the getElementsByName() method is given below:
- document.getElementsByName("name")
Example of document.getElementsByName() method :
<script type="text/javascript">
function totalelements()
{
var allgenders=document.getElementsByName("gender");
alert("Total Genders:"+allgenders.length);
}
</script>
<form>
Male:<input type="radio" name="gender" value="male">
Female:<input type="radio" name="gender" value="female">
<input type="button" onclick="totalelements()" value="Total Genders">
</form>
Or Display Alert Box Which Value is Total Genders:2
document.getElementsByTagName() method :
The document.getElementsByTagName() method returns all the element of specified tag name.
The syntax of the getElementsByTagName() method is given below:
- document.getElementsByTagName("name")
Example of document.getElementsByTagName() method :
In this example, we going to count total number of paragraphs used in the document. To do this, we have called the document.getElementsByTagName("p") method that returns the total paragraphs.
<script type="text/javascript">
function countpara(){
var totalpara=document.getElementsByTagName("p");
alert("total p tags are: "+totalpara.length);
}
</script>
<p>This is a pragraph</p>
<p>Here we are going to count total number of paragraphs by getElementByTagName() method.</p>
<p>Let's see the simple example</p>
<button onclick="countpara()">count paragraph</button>
And Display Alert Box Which Value is total p tags are: 3
Javascript - innerHTML :
The innerHTML property can be used to write the dynamic html on the html document.
It is used mostly in the web pages to generate the dynamic html such as registration form, comment form, links etc.
Javascript - innerText :
The innerText property can be used to write the dynamic text on the html document.
Here, text will not be interpreted as html text but a normal text.
It is used mostly in the web pages to generate the dynamic content such as writing the validation message, password strength etc.
JavaScript Form Validation :
It is important to validate the form submitted by the user because it can have inappropriate values. So, validation is must to authenticate user.
JavaScript provides facility to validate the form on the client-side so data processing will be faster than server-side validation.
Most of the web developers prefer JavaScript form validation.
JavaScript Form Validation Example :
In this example, we are going to validate the name and password. The name can’t be empty and password can’t be less than 6 characters long.
<html>
<body>
<script>
function validateform(){
var name=document.myform.name.value;
var password=document.myform.password.value;
if (name==null || name==""){
alert("Name can't be blank");
return false;
}else if(password.length<6){
alert("Password must be at least 6 characters long.");
return false;
}
}
</script>
<body>
<form name="myform" method="post" action="http://www.javatpoint.com/javascriptpages/valid.jsp" onsubmit="return validateform()" >
Name: <input type="text" name="name"><br/>
Password: <input type="password" name="password"><br/>
<input type="submit" value="register">
</form>
</body>
</html>
JavaScript email validation :
We can validate the email by the help of JavaScript.
There are many criteria that need to be follow to validate the email id such as:
email id must contain the @ and . character
There must be at least one character before and after the @.
There must be at least two characters after . (dot).
Example:
<html>
<body>
<script>
function validateemail()
{
var x=document.myform.email.value;
var atposition=x.indexOf("@");
var dotposition=x.lastIndexOf(".");
if (atposition<1 || dotposition<atposition+2 || dotposition+2>=x.length){
alert("Please enter a valid e-mail address \n atpostion:"+atposition+"\n dotposition:"+dotposition);
return false;
}
}
</script>
<body>
<form name="myform" method="post" action="http://www.javatpoint.com/javascriptpages/valid.jsp" onsubmit="return validateemail();">
Email: <input type="text" name="email"><br/>
<input type="submit" value="register">
</form>
</body>
</html>
Whats Next?
Javascript OOPS
Subscribe to my newsletter
Read articles from Aditya Gadhave directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
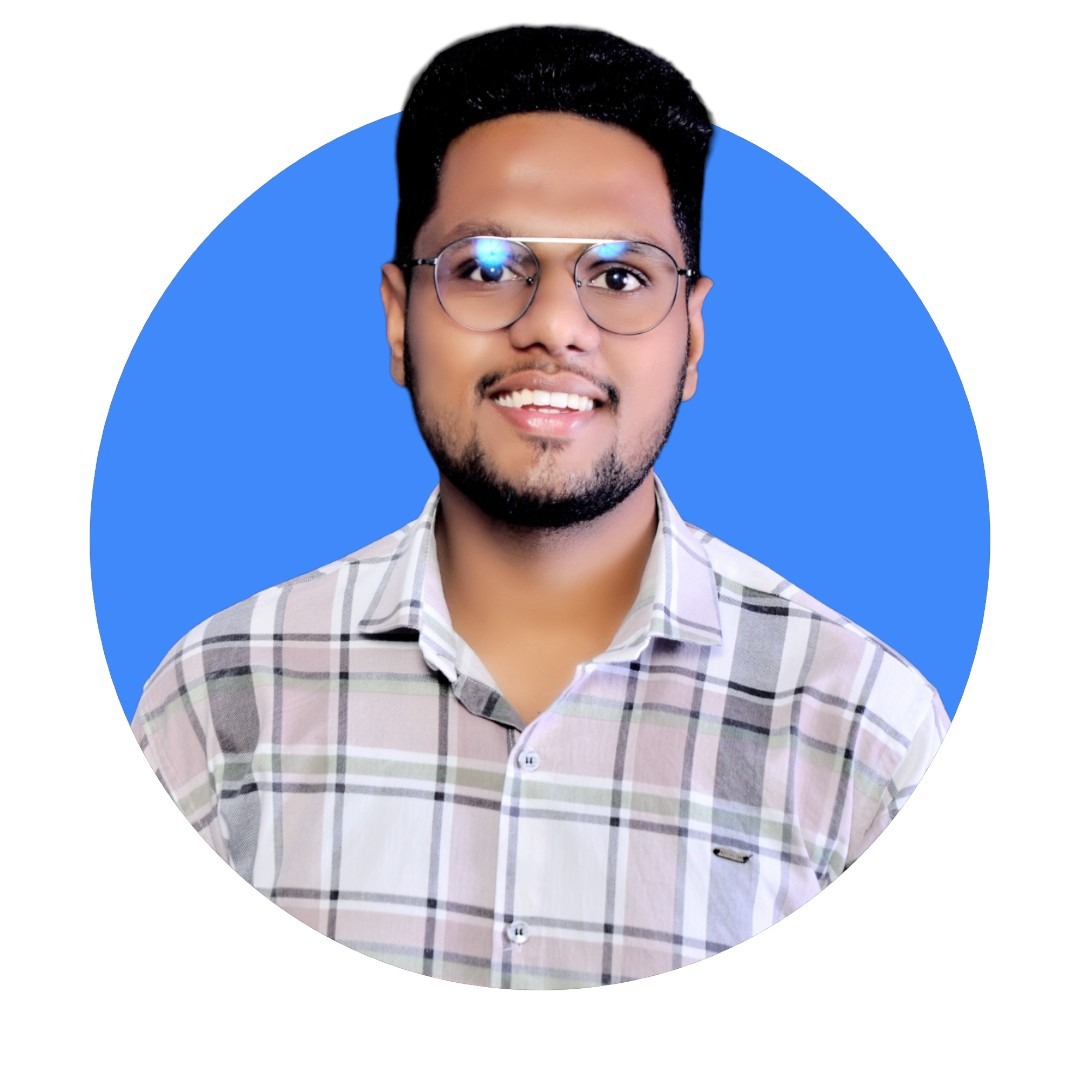
Aditya Gadhave
Aditya Gadhave
👋 Hello! I'm Aditya Gadhave, an enthusiastic Computer Engineering Undergraduate Student. My passion for technology has led me on an exciting journey where I'm honing my skills and making meaningful contributions.