Taking Screenshots Using Python & PyQt
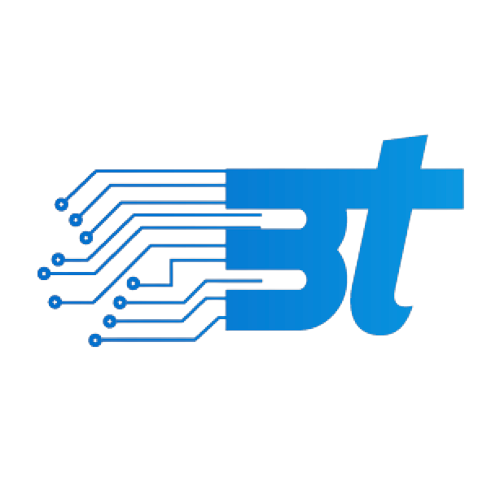

Taking screenshots is a common feature in many desktop applications, whether for capturing content, providing feedback, or documenting issues. PyQt, a set of Python bindings for the Qt libraries, allows you to create rich desktop applications with a native look and feel. In this comprehensive guide, we'll walk you through creating a PyQt application to capture and save screenshots.
Prerequisites
Before we start coding, ensure you have Python installed on your system. Additionally, you need to install PyQt5, which you can do using pip:
pip install PyQt5
Setting Up the Project
Start by creating a new Python script file, for example, screenshot_
app.py
. We will build our application step by step.
Importing Required Libraries
We need to import the essential modules from PyQt5 to create our GUI and handle the screenshot functionality.
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout, QPushButton, QLabel, QFileDialog
from PyQt5.QtGui import QPixmap, QScreen
from PyQt5.QtCore import Qt
Designing the Main Window
We'll design a simple window that includes a button to capture the screenshot and a label to display the captured image.
class ScreenshotApp(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setWindowTitle('Screenshot App')
self.setGeometry(100, 100, 800, 600)
layout = QVBoxLayout()
self.label = QLabel('Screenshot will appear here', self)
self.label.setAlignment(Qt.AlignCenter)
layout.addWidget(self.label)
self.button = QPushButton('Take Screenshot', self)
self.button.clicked.connect(self.take_screenshot)
layout.addWidget(self.button)
self.setLayout(layout)
Explanation of the UI Design
Window Title and Size: The
self.setWindowTitle
sets the title of the window, andself.setGeometry
sets the position and size of the window.Layout: We use a
QVBoxLayout
to arrange widgets vertically.Label: A
QLabel
is used to display the screenshot. It initially shows a placeholder text.Button: A
QPushButton
is used to trigger the screenshot capture. Theclicked
signal is connected to thetake_screenshot
method.
Implementing the Screenshot Functionality
Next, we'll implement the functionality to capture and save screenshots.
def take_screenshot(self):
screen = QApplication.primaryScreen()
screenshot = screen.grabWindow(0)
self.label.setPixmap(screenshot.scaled(self.label.size(), Qt.KeepAspectRatio))
# Save the screenshot
file_path, _ = QFileDialog.getSaveFileName(self, "Save Screenshot", "", "PNG Files (*.png);;All Files (*)")
if file_path:
screenshot.save(file_path, 'png')
Explanation of the Screenshot Functionality
Capture Screen:
QApplication.primaryScreen().grabWindow(0)
captures the entire screen.Display Screenshot: The captured screenshot is displayed in the
QLabel
usingsetPixmap
.Save Screenshot: A
QFileDialog
prompts the user to choose a location and filename to save the screenshot. The screenshot is saved as a PNG file.
Putting It All Together
Combine the UI design and screenshot functionality into a complete PyQt application.
class ScreenshotApp(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setWindowTitle('Screenshot App')
self.setGeometry(100, 100, 800, 600)
layout = QVBoxLayout()
self.label = QLabel('Screenshot will appear here', self)
self.label.setAlignment(Qt.AlignCenter)
layout.addWidget(self.label)
self.button = QPushButton('Take Screenshot', self)
self.button.clicked.connect(self.take_screenshot)
layout.addWidget(self.button)
self.setLayout(layout)
def take_screenshot(self):
screen = QApplication.primaryScreen()
screenshot = screen.grabWindow(0)
self.label.setPixmap(screenshot.scaled(self.label.size(), Qt.KeepAspectRatio))
# Save the screenshot
file_path, _ = QFileDialog.getSaveFileName(self, "Save Screenshot", "", "PNG Files (*.png);;All Files (*)")
if file_path:
screenshot.save(file_path, 'png')
if __name__ == "__main__":
app = QApplication(sys.argv)
window = ScreenshotApp()
window.show()
sys.exit(app.exec_())
Running the Application
Save the script and run it using Python:
python screenshot_app.py
This command opens a window with a button labeled "Take Screenshot." When you click the button, the application captures the current screen, displays the screenshot in the window, and prompts you to save the screenshot as a PNG file.
Region Capture: Allow users to select a specific region of the screen to capture.
Timestamp Naming: Automatically name the screenshot files with the current timestamp.
Image Formats: Support saving screenshots in different image formats (e.g., JPEG, BMP).
Additional Steps for macOS
On macOS, applications require explicit permission to capture the screen. To grant this permission:
Run the Application: Run your PyQt application once.
System Preferences: Go to
System Preferences
>Security & Privacy
>Privacy
tab.Screen Recording: In the left-hand sidebar, select
Screen Recording
.Add Your Application: Click the lock icon to make changes, and add your terminal or IDE to the list by clicking the
+
button and selecting the appropriate application.
Using the above I have created a tracker for our company.
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
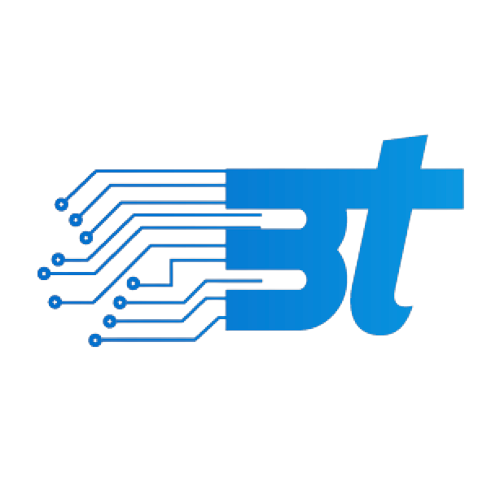
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.