How to Create an Accordion in React JS Using Tailwind CSS

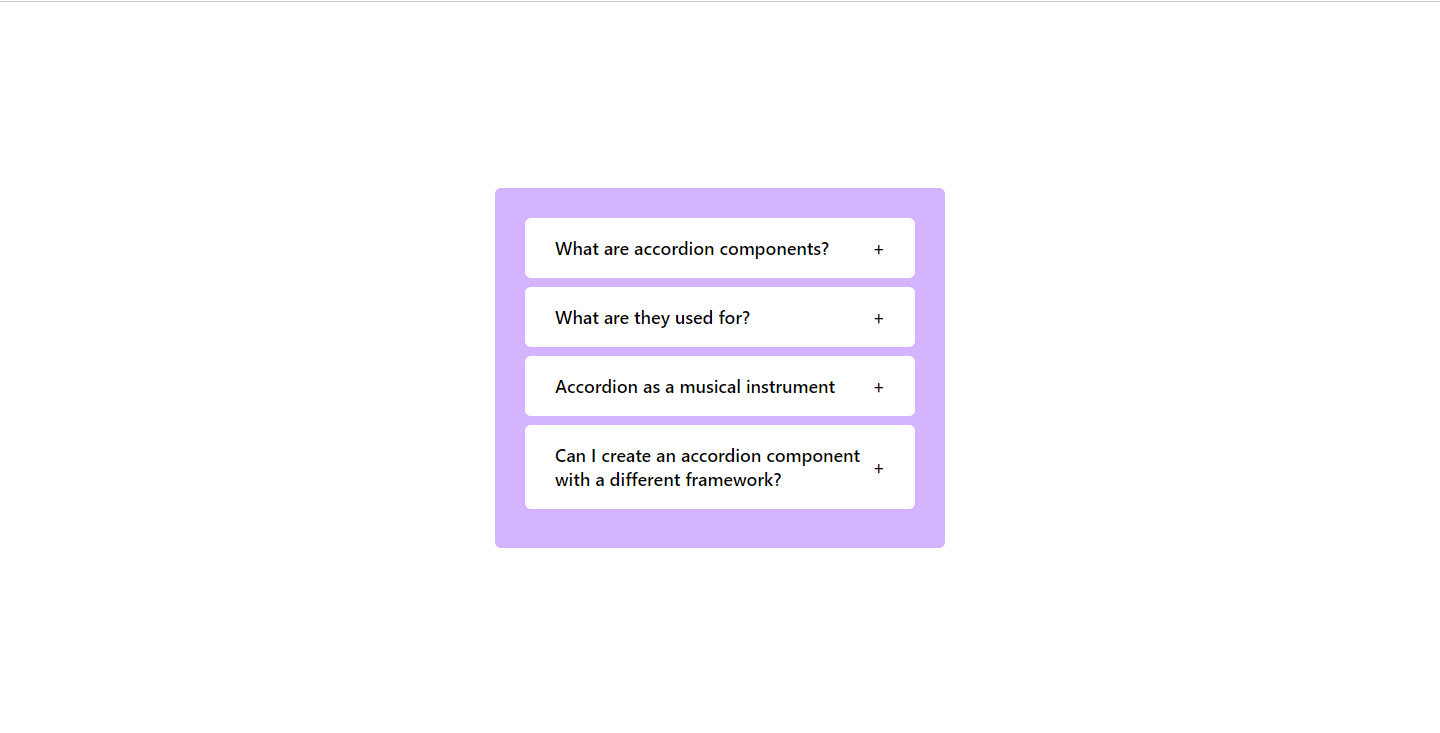
Accordion components are a popular way to organize and present content in a collapsible manner. They help save screen space and make information more accessible to users. In this blog, I'll show you how to create a simple accordion component using React.
What We'll Build
We'll create an accordion component that displays a list of questions. When a question is clicked, the corresponding answer will be revealed. We'll use some basic React concepts like state management and event handling.
Prerequisites
To follow along, you should have a basic understanding of React and how to set up a React project. If you're new to React, I recommend going through the official React documentation.
Setting Up the Project
First, create a new React project if you don't have one already. You can use Create React App for this:
npx create-react-app accordion-example
cd accordion-example
npm start
Code:
import React, { useState } from "react";
const data = [
{
id: "1",
question: "What are accordion components?",
answer:
"Accordion components are user interface elements used for organizing and presenting content in a collapsible manner. They typically consist of a header, content, and an expand/collapse action.",
},
{
id: "2",
question: "What are they used for?",
answer:
"They are commonly employed in various contexts, including FAQs, product descriptions, navigation menus, settings panels, and data tables, to save screen space and provide a structured and user-friendly interface for presenting information or options.",
},
{
id: "3",
question: "Accordion as a musical instrument",
answer:
"The accordion is a musical instrument with a keyboard and bellows. It produces sound by air passing over reeds when the player expands or compresses the bellows, used in various music genres.",
},
{
id: "4",
question: "Can I create an accordion component with a different framework?",
answer:
"Yes of course, it is very possible to create an accordion component with another framework.",
},
];
const Accordian1 = () => {
const [selected, setSelected] = useState(null);
const handleClick = (id) => {
setSelected(selected === id ? null : id);
};
return (
<div className="flex justify-center items-center h-[100vh]">
<div className="w-[600px] rounded-lg bg-purple-300 p-10">
{data && data.length > 0 ? (
data.map((item, id) => (
<div
key={id}
onClick={() => handleClick(id)}
className="flex flex-col mb-3 px-10 py-6 text-lg bg-white rounded-lg"
>
<div className="flex justify-between items-center">
<h1 className="text-2xl font-semibold">{item.question}</h1>
<p className="text-2xl font-semibold">+</p>
</div>
{selected === id && <div className="py-3">{item.answer}</div>}
</div>
))
) : (
<div>Data Not Found</div>
)}
</div>
</div>
);
};
export default Accordian1;
Understanding the Code
State Management: We use the
useState
hook to manage the state of the selected accordion item. Theselected
state variable stores the ID of the currently expanded item.Event Handling: The
handleClick
function is called when an accordion header is clicked. It updates theselected
state to either expand or collapse the content.Conditional Rendering: We conditionally render the answer for each question based on whether its ID matches the
selected
state.Styling: The component is styled using Tailwind CSS classes. You can modify these styles or use your preferred CSS framework.
Conclusion
That's it! We've built a simple accordion component in React. This component can be easily customized and extended based on your needs. Accordions are a great way to organize content and enhance the user experience on your website.
Feel free to experiment with the code and add more features like animations or different styling options. Happy coding!
Subscribe to my newsletter
Read articles from Abdul Basit Khan (Abdul Basit) directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Abdul Basit Khan (Abdul Basit)
Abdul Basit Khan (Abdul Basit)
Myself Abdul basit khan I am an undergraduate student of b-tech from Marathwada institute of technology Aurangabad.